Understanding the Pitfalls of 'instanceof' in JavaScript
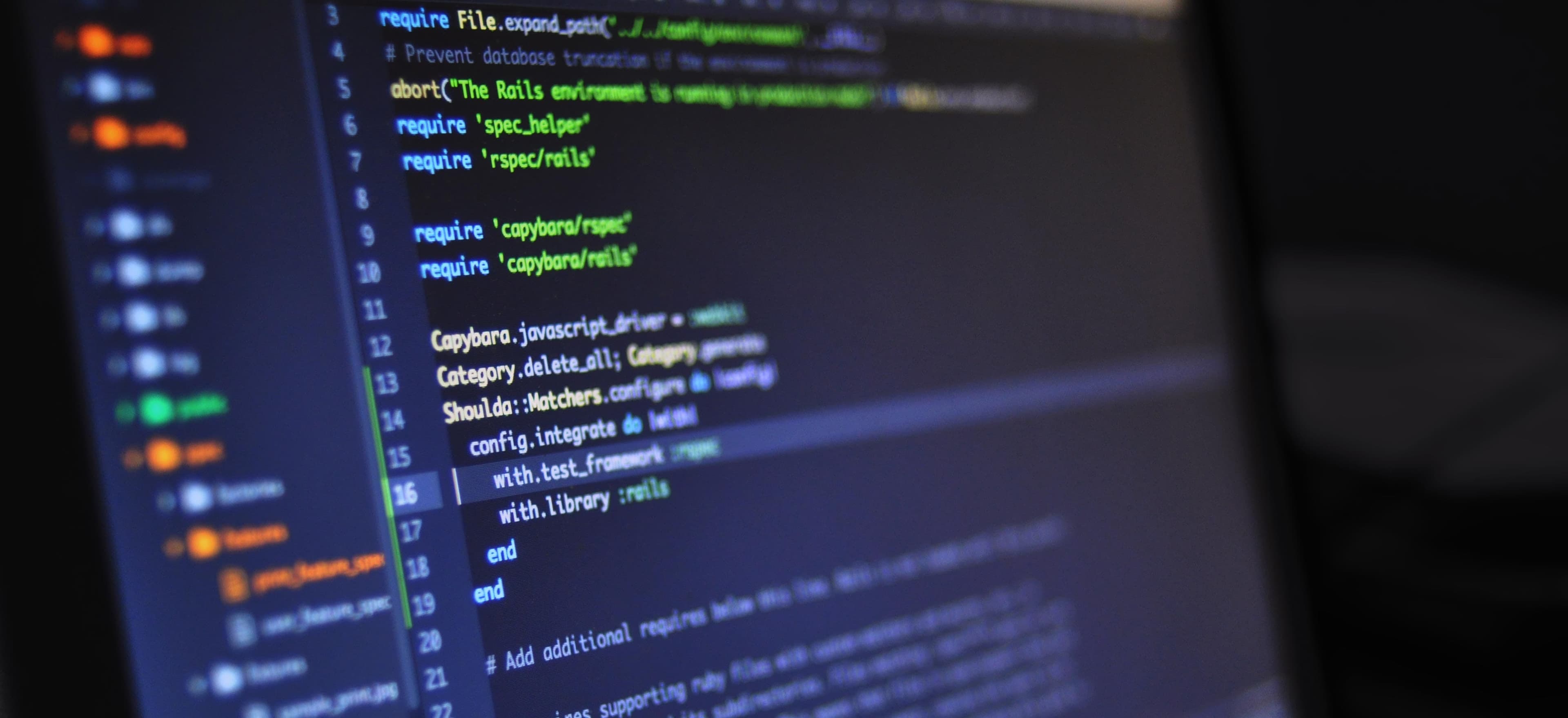
- Published on
Understanding the Pitfalls of instanceof
in JavaScript
JavaScript is a powerful and versatile language that has found its way into numerous applications, from front-end web development to server-side programming with Node.js. One of the features in JavaScript that can sometimes lead to confusion and unexpected behaviors is the instanceof
operator. In this blog post, we will delve into the intricacies of instanceof
, discuss its functionalities, and explore some common pitfalls along the way.
What is instanceof
?
The instanceof
operator in JavaScript checks if an object is an instance of a specific constructor function or class. Its syntax is straightforward:
object instanceof constructor
Here, object
can be an instance of either a built-in or user-defined object. The constructor
is the function you're checking against, which usually serves as a prototype for your objects.
Example of instanceof
Let's consider a simple example:
function Animal(name) {
this.name = name;
}
let dog = new Animal("Rex");
console.log(dog instanceof Animal); // true
In this example, dog
is an instance of Animal
, and thus dog instanceof Animal
returns true
.
Understanding the Prototype Chain
To properly grasp how instanceof
operates, it's crucial to understand the prototype chain in JavaScript. When an object is created, it inherits properties and methods from its prototype. The instanceof
operator verifies whether the prototype property of a constructor appears anywhere in the prototype chain of the object.
Prototype Chain Example
function Animal(name) {
this.name = name;
}
function Dog(name) {
Animal.call(this, name); // Call Animal constructor
}
Dog.prototype = Object.create(Animal.prototype); // Inherit from Animal
Dog.prototype.constructor = Dog;
let wolf = new Dog("Wolf");
console.log(wolf instanceof Dog); // true
console.log(wolf instanceof Animal); // true
In this case, wolf
is an instance of both Dog
and Animal
because of how the prototype chain has been set up. However, this setup can also lead to certain pitfalls.
Common Pitfalls of instanceof
Despite its usefulness, relying heavily on instanceof
can lead to numerous misconceptions and errors. Here are some common pitfalls:
1. Object Type Checking and Cross-frame Issues
One prevalent pitfall occurs when dealing with JavaScript objects that are created in different execution contexts, such as iframes. Each iframe has its own global environment, which means that even if two objects are created with the same constructor, they might not be considered the same instance.
// Frame 1
const obj1 = new SomeConstructor();
// Frame 2
const obj2 = new SomeConstructor();
console.log(obj1 instanceof SomeConstructor === obj2 instanceof SomeConstructor); // false
This behavior can lead to confusion when passing objects across different execution contexts. Always keep this in mind when using instanceof
in applications that utilize iframes or window objects.
2. Non-Function Constructors
If you mistakenly check an object against a non-function constructor, instanceof
will always return false
. For example:
let number = 42;
console.log(number instanceof Number); // false
This is because number
is a primitive type and does not inherit from Number
.
3. Modifying Prototypes
Another common pitfall arises when you dynamically alter an object's prototype. If the prototype of a constructor is changed after you've created instances, the instanceof
operator may yield unexpected results:
function Food() {}
let fruit = new Food();
Food.prototype = {}; // Changes the prototype after creating an instance.
console.log(fruit instanceof Food); // true - This still returns true.
console.log(fruit instanceof Object); // true - Still inherently an object.
let newFruit = new Food(); // A new instance with an altered prototype.
console.log(newFruit instanceof Food); // true
Changes to the prototype won't retroactively affect existing instances, but it can lead to inconsistencies in later instances.
4. Multiple Inheritance and Mixin Patterns
JavaScript does not support multiple inheritance like other languages. However, many developers attempt to mimic this through mixins. This can lead to issues when using instanceof
.
For example, consider the following setup:
function A() {}
function B() {}
Object.assign(B.prototype, A.prototype);
let bInstance = new B();
console.log(bInstance instanceof A); // false
Here, bInstance
does not recognize A
as part of its prototype chain despite having access to the properties and methods of A
.
Alternative Type Checking Methods
Given the pitfalls of instanceof
, you might consider alternative ways to check an object's type. Some methods include:
1. Using Object.prototype.toString.call()
This method allows for more precise type checking across different contexts:
function checkType(obj) {
return Object.prototype.toString.call(obj).slice(8, -1);
}
console.log(checkType([])); // Array
console.log(checkType({})); // Object
console.log(checkType(new Date())); // Date
2. Using typeof
The typeof
operator is another alternative but has its limitations, especially with complex types:
console.log(typeof 123); // "number"
console.log(typeof "Hello"); // "string"
console.log(typeof { name: "John" }); // "object"
3. Custom Type Checking
If you have specific needs, you can create your own type-checking functions to contextually ensure the types of instances you work with.
function isAnimal(obj) {
return obj instanceof Animal;
}
In Conclusion, Here is What Matters
The instanceof
operator is a powerful tool in JavaScript that can simplify type checking but comes with a plethora of pitfalls that can lead to bugs and misunderstandings. Understanding its behavior in terms of the prototype chain, iframe interactions, and modifications is vital for any JavaScript developer.
Improving your knowledge about these intricacies will not only lead to cleaner code but also help in avoiding common errors that can slow development speed and introduce complexity. Whether you decide to stick with instanceof
or explore alternatives, clarity in type checking is essential for creating robust JavaScript applications.
By taking these factors into account and being aware of potential problems, you'll be better equipped to use instanceof
effectively and over adjudge the other available options for type checking.
For further reading about JavaScript prototypes and inheritance, check out MDN Web Docs - Prototypes.
Happy coding!