Mastering Page Object Model: Common Pitfalls to Avoid
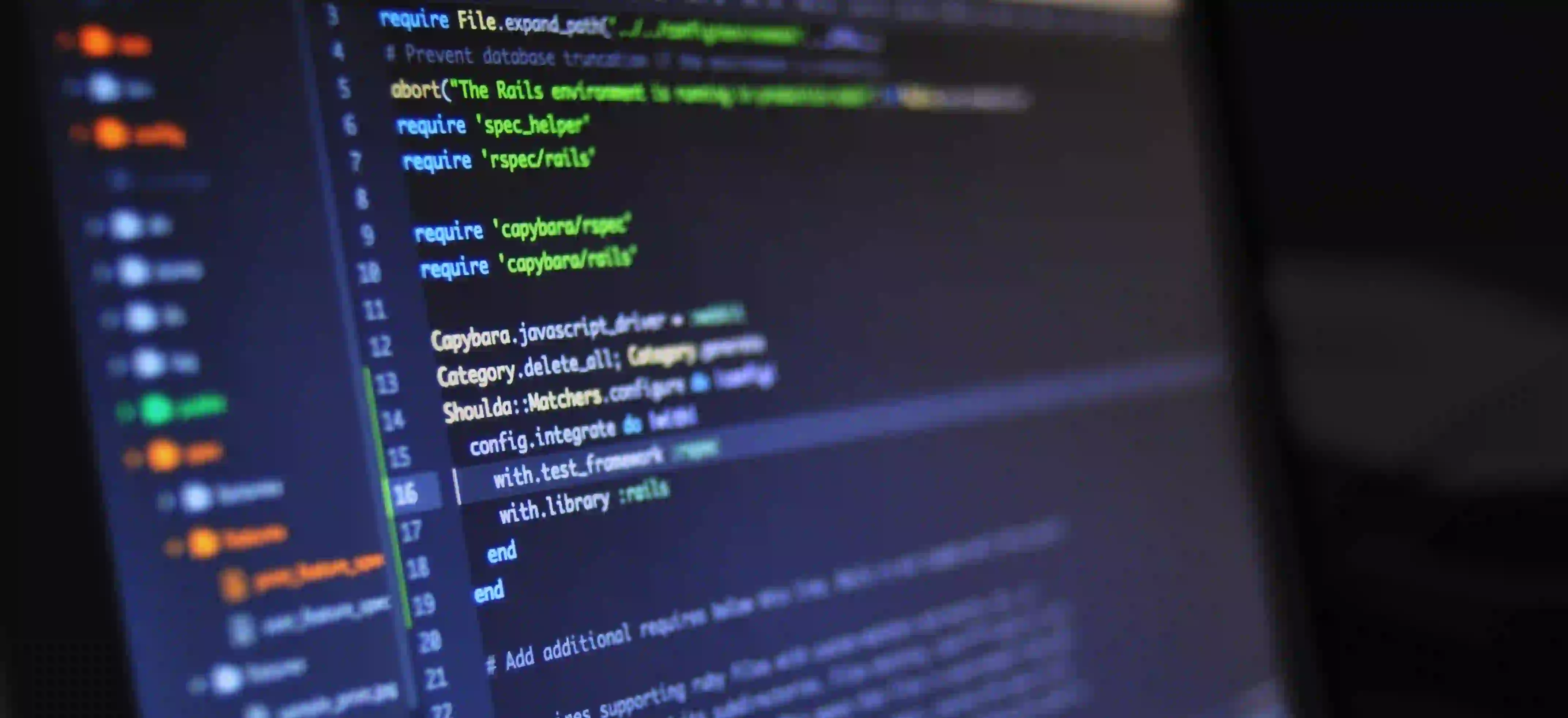
Mastering Page Object Model: Common Pitfalls to Avoid
The Page Object Model (POM) has emerged as a key design pattern in the realm of test automation. It aims to enhance maintainability, reduce code duplication, and simplify test case readability. While implementing POM can transform your test automation strategy, there are common pitfalls that testers often face. In this blog post, we will explore these pitfalls, providing practical solutions and tips to help you master POM effectively.
What is Page Object Model?
Before diving into the common pitfalls, let's define what Page Object Model is. POM is a design pattern for automating user interfaces in software testing. Essentially, a "page object" is an interface for a web page or a component, wrapping the details of that page, including its elements and methods.
Basic Structure of POM
With POM, each web page has a corresponding class that serves as the "object" for that page. Here's a simple example:
// LoginPage.java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.By;
public class LoginPage {
private WebDriver driver;
// Constructor to initialize the driver
public LoginPage(WebDriver driver) {
this.driver = driver;
}
// Locators
private By usernameField = By.id("username");
private By passwordField = By.id("password");
private By loginButton = By.id("loginBtn");
// Actions
public void enterUsername(String username) {
driver.findElement(usernameField).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(passwordField).sendKeys(password);
}
public void clickLogin() {
driver.findElement(loginButton).click();
}
}
In the above code, we encapsulate all actions related to the login page in one class. The driver’s instance is utilized to interact with the page elements, thus promoting separation of concerns.
Common Pitfalls in POM Implementation
With an understanding of the Page Object Model, let’s explore some common pitfalls and how to avoid them.
1. Overloading Page Objects
One common mistake is attempting to cram too many responsibilities into a single page object. Sometimes testers mix navigation logic, assertions, and form submissions all into one class.
Why This Matters: When you overload a page object, it becomes hard to maintain. Your class may grow to an unmanageable size, making it difficult to read and debug.
Solution: Keep your page objects focused on a single responsibility. Ideally, a page object should represent a single page or a component and expose only the associated actions. If you find that a page object is handling multiple actions from different parts of the application, consider breaking it into other relevant objects.
Example:
// Separate Page Objects for Login and Dashboard
public class DashboardPage {
private WebDriver driver;
// Constructor
public DashboardPage(WebDriver driver) {
this.driver = driver;
}
// Actions related to the Dashboard
public void logout() {
driver.findElement(By.id("logoutBtn")).click();
}
}
By keeping page objects concise, you foster a more manageable codebase.
2. Not Using Encapsulation Properly
Another pitfall occurs when the page elements are not encapsulated securely. If you expose the web elements directly, it opens the door for unintended manipulation from the tests.
Why This Matters: Poor encapsulation leads to tests that are tightly coupled with the implementation of the UI, resulting in fragile tests that break with every UI change.
Solution: Always use private access modifiers for your locators and expose public methods for interactions. This not only protects the elements but also centralizes the point of change when the UI evolves.
Example:
private By usernameField = By.id("username");
// Public method to interact with the field
public void enterUsername(String username) {
driver.findElement(usernameField).sendKeys(username);
}
3. Neglecting to Maintain Page Object Classes
Many teams adhere to the POM but neglect to maintain their page object classes. As the application grows, so should your page objects.
Why This Matters: Outdated page objects can lead to confusion, making your tests harder to read and maintain. This can render the POM ineffective and counterproductive.
Solution: Regularly review and refactor your page objects as the application changes. Conduct code reviews and maintain documentation for changes in your UI components.
4. Using Page Objects Outside Their Context
The common temptation is to use page objects not just for UI actions but also for business logic or complex test flows.
Why This Matters: This can blur the lines between test design and the application logic, making it hard to understand the intent of tests.
Solution: Let page objects handle only UI-oriented actions. For business logic, utilize service or helper classes that invoke the intended functionality, while keeping your page objects focused solely on UI interactions.
Example:
// Service Layer Class
public class UserService {
public void createUser(String username, String password) {
// logic to create a user
}
}
// In your test class
@Autowired
private UserService userService;
@Test
public void testUserLogin() {
userService.createUser("testUser", "testPass");
LoginPage loginPage = new LoginPage(driver);
loginPage.enterUsername("testUser");
loginPage.enterPassword("testPass");
loginPage.clickLogin();
}
5. Neglecting to Implement Waits
Selenium tests can fail intermittently when elements take too long to load. A common mistake is not implementing any form of waiting strategy like Implicit Waits or Explicit Waits.
Why This Matters: Neglecting waits can lead to flaky tests that pass once but fail another time due to timing issues.
Solution: Incorporate appropriate waits in your page object constructors or action methods.
Example of an Explicit Wait:
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.support.ui.ExpectedConditions;
public void clickLogin() {
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.elementToBeClickable(loginButton));
driver.findElement(loginButton).click();
}
To Wrap Things Up
Using the Page Object Model effectively can greatly enhance your test automation approach, providing cleaner and more reliable tests. However, it is essential to be mindful of common pitfalls such as overloading objects, inadequate encapsulation, and neglect in maintaining these classes.
By keeping your page objects focused, encapsulated, and updated, along with implementing appropriate waits, you can unlock the full potential of POM in your automation framework.
Further Reading
- Page Object Model in Selenium
- Effective Test Automation
Make sure to leverage these best practices, and avoid the pitfalls we’ve discussed today, to create a solid foundation for your test automation efforts using the Page Object Model. Happy testing!