Mastering Complex URL Mappings in Play Framework
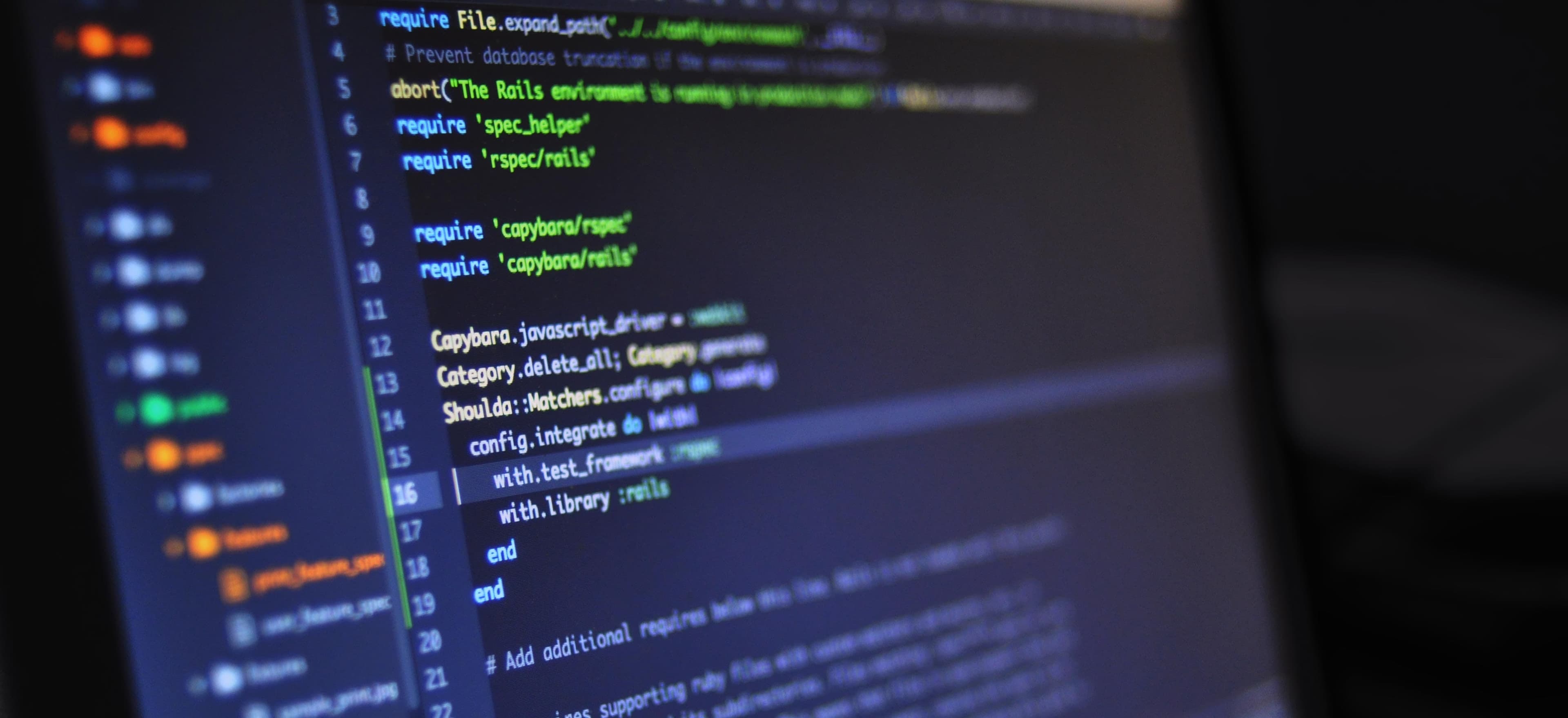
- Published on
Mastering Complex URL Mappings in Play Framework
When working with web applications, URL mappings play a crucial role in determining how users interact with your application. For developers using the Play Framework, understanding and mastering complex URL mappings can significantly enhance the routing capabilities of your app. In this post, we will delve into the intricacies of URL mappings within the Play Framework, explore best practices, and provide code snippets to illustrate key concepts.
Table of Contents
- Understanding Play Framework Routing
- Creating Basic Routes
- Implementing Path Parameters
- Using Query Parameters
- Handling Optional Parameters
- Creating Nested Routes
- Conclusion
Understanding Play Framework Routing
Routing in the Play Framework defines how incoming requests are matched to controller actions. The routing file (conf/routes
) acts as a central configuration that connects HTTP requests to their respective handler methods. This configuration is pivotal in shaping user experience and optimizing SEO.
# conf/routes
GET / controllers.HomeController.index
GET /about controllers.HomeController.about
In the above code, we map two GET requests to their respective actions in the HomeController
. This is the most fundamental aspect of routing.
Creating Basic Routes
Creating basic routes is straightforward. Each route consists of an HTTP method, a URL pattern, and an action. In our example, we have created routes for the home and about pages.
GET /products controllers.ProductController.list
GET /products/:id controllers.ProductController.show(id: Long)
Here, the :id
path parameter allows us to create a dynamic route. When a user accesses /products/1
, it maps to the show
action of the ProductController
, passing 1
as the id
.
Why Use Basic Routes?
Basic routes are essential for defining clear navigation paths within the application. They enable developers to control how users access different parts of the site, fostering an intuitive user experience while enhancing SEO capabilities.
Implementing Path Parameters
Path parameters allow you to capture variable parts of the URL. You can create dynamic and user-friendly URLs that enhance both usability and SEO.
GET /users/:username controllers.UserController.profile(username: String)
In the example above, the URL /users/johndoe
would route to the profile
action in the UserController
and would provide the username johndoe
as an argument.
Code Example
Let's see how this works in practice:
// app/controllers/UserController.scala
package controllers
import play.api.mvc._
class UserController @Inject()(cc: ControllerComponents) extends AbstractController(cc) {
def profile(username: String) = Action { implicit request =>
Ok(s"Welcome to the profile of $username")
}
}
In this action, we send a welcome message that includes the username parameter. This interaction showcases how path parameters can personalize the user experience.
Using Query Parameters
Query parameters serve as an additional layer for routing, allowing side information to be passed alongside a URL. This can be particularly useful for pagination, filtering, or searching within your application.
GET /search controllers.SearchController.results(query: String ? "")
In the route above, we define a search route that pulls a query
parameter from the URL, allowing users to search for content dynamically.
Code Example
// app/controllers/SearchController.scala
package controllers
import play.api.mvc._
class SearchController @Inject()(cc: ControllerComponents) extends AbstractController(cc) {
def results(query: String) = Action { implicit request =>
Ok(s"Search results for: $query")
}
}
In this method, the results
action handles the search query and presents a response based on user input. Using query parameters is a great way to capture additional data.
Handling Optional Parameters
Sometimes, parameters may not always be present in a URL. In such cases, handling optional parameters improves the robustness of your application.
GET /items controllers.ItemController.index(category: String ? "")
The above route accepts an optional category
parameter. If not provided, it defaults to an empty string.
Code Example
// app/controllers/ItemController.scala
package controllers
import play.api.mvc._
class ItemController @Inject()(cc: ControllerComponents) extends AbstractController(cc) {
def index(category: Option[String]) = Action { implicit request =>
val message = category match {
case Some(cat) => s"Items in category: $cat"
case None => "All items"
}
Ok(message)
}
}
Using Scala's Option
type, we elegantly handle whether a category is provided, resulting in clearer and more maintainable code.
Creating Nested Routes
For larger applications, it can be beneficial to create nested routes that represent hierarchical relationships between resources. Nesting enhances organization and clarity in URL structure.
GET /users/:userId/orders controllers.OrderController.list(userId: Long)
GET /users/:userId/orders/:orderId controllers.OrderController.show(userId: Long, orderId: Long)
With these routes, you can generate URLs that clearly represent the relationship between users and their orders.
Code Example
// app/controllers/OrderController.scala
package controllers
import play.api.mvc._
class OrderController @Inject()(cc: ControllerComponents) extends AbstractController(cc) {
def list(userId: Long) = Action { implicit request =>
Ok(s"List of orders for user: $userId")
}
def show(userId: Long, orderId: Long) = Action { implicit request =>
Ok(s"Order details for order: $orderId for user: $userId")
}
}
Nesting routes not only provides clarity but also optimizes the organization of related resources, enhancing both usability and SEO effectiveness.
Closing the Chapter
Mastering complex URL mappings in the Play Framework is essential for any web developer aiming to build scalable and user-friendly applications. By understanding how to effectively use basic routes, path parameters, query parameters, optional parameters, and nested routes, developers can create structured and meaningful URLs that significantly improve user engagement and search engine optimization.
For further reading, you might find the Play Framework Documentation beneficial, as it offers in-depth insights and advanced routing strategies. Embrace the power of routing, and witness how it can transform your web applications.
This article aimed to provide a comprehensive guide to URL mappings in the Play Framework while ensuring that it remains engaging and informative. If you have any questions or need further clarification on specific topics, feel free to leave a comment!
Checkout our other articles