Mastering JDK 9 REPL: Common Pitfalls to Avoid
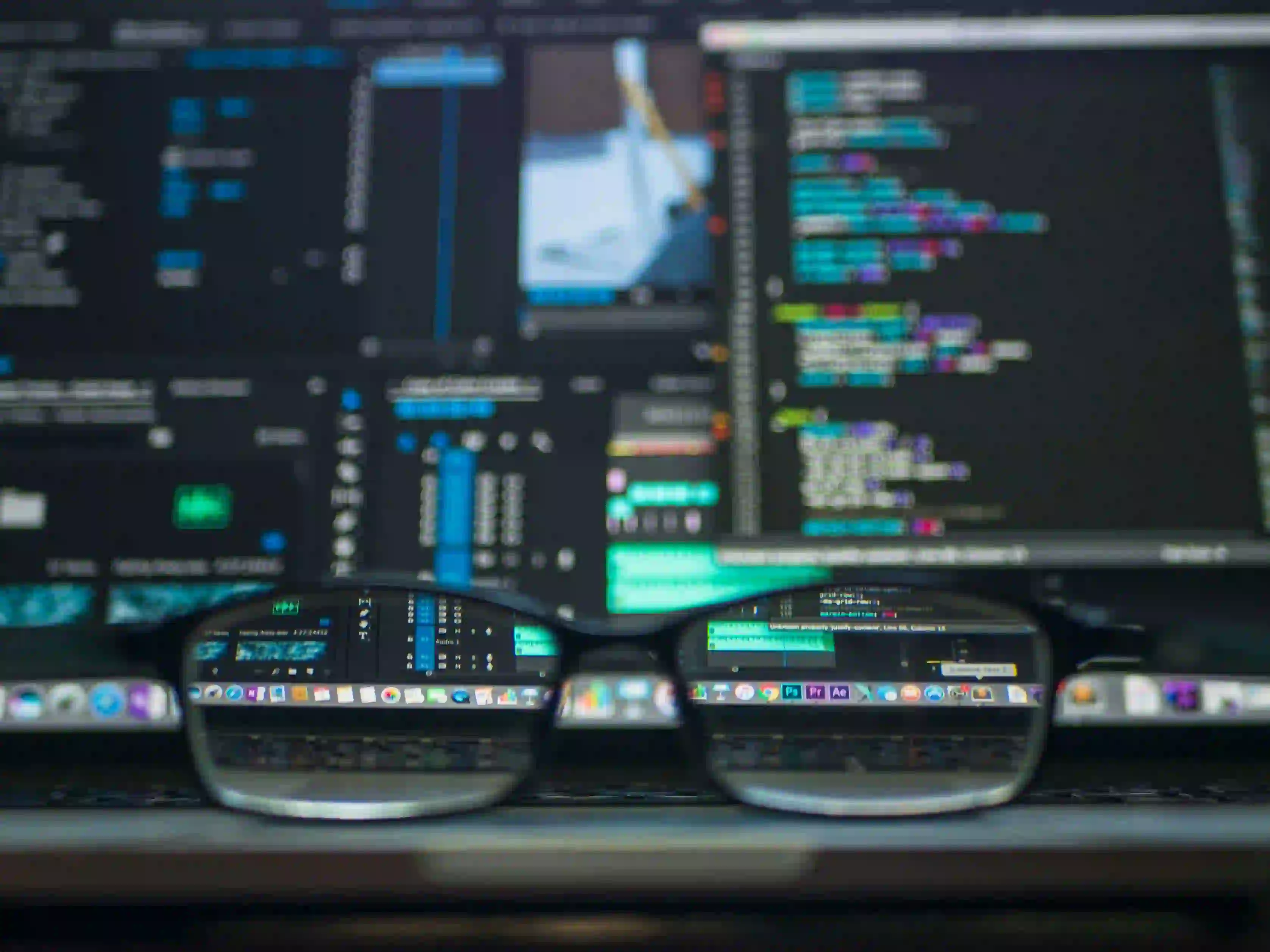
Mastering JDK 9 REPL: Common Pitfalls to Avoid
Java Development Kit (JDK) 9 introduced a significant feature: the Read-Eval-Print Loop (REPL), known as JShell. This interactive tool allows developers to execute Java code snippets, making it easier to test, debug, and learn Java's syntax and semantics without the overhead of setting up a complete project. However, when working with JShell, there are common pitfalls that both newcomers and seasoned developers can encounter. In this blog post, we will discuss those pitfalls, understand how to avoid them, and ultimately enhance your programming experience with JDK 9 REPL.
What is JShell?
Before diving into the common pitfalls, let's clarify what JShell is. JShell is an interactive command-line tool that provides a runtime for evaluating Java code snippets. It's particularly useful for:
- Testing quick code snippets.
- Experimenting with Java libraries.
- Understanding Java concepts without complex project configurations.
You can start JShell by executing the command:
jshell
Upon starting, you will obtain a prompt where you can directly input Java code.
Common Pitfalls in JShell
1. Misunderstanding Variable Scope
One of the most common misconceptions about JShell is its treatment of variable scope. Variables declared in JShell are not available outside their scope in the same way they are in a traditional Java program.
Example:
int x = 10; // Declare x
System.out.println(x); // This will output 10
If you try to declare a variable inside a block (like within a method or loop), it won't be accessible outside that block:
{ // Start of a block
int y = 20;
}
// Trying to access y here will result in an error!
System.out.println(y); // Error: cannot find symbol
Tip: Always be aware of where you declare your variables in JShell. They will be scoped similarly to how blocks function in regular Java.
2. Lack of Type Inference
While JShell supports type inference, this may lead to confusion when declaring variable types that would typically be inferred in a full class or method context. For example, in traditional Java code, you might have:
List<String> list = new ArrayList<>();
In JShell, you must explicitly declare the type if context does not provide it.
JShell Example:
var list = new ArrayList<String>(); // Correct way to declare with type
Here 'var' is a keyword that provides type inference, but if used without a clear expected type, JShell may produce an error.
Avoiding this Pitfall: When using JShell, ensure proper type declarations, especially when initializing collections or classes.
3. Forgetting to Import Classes
JShell can automatically import many of the Java standard libraries, but it does not include every class. If you are working with libraries or classes that are not automatically imported, you will receive a compilation error.
Example:
import java.util.ArrayList;
// Using ArrayList without an import will result in an error
ArrayList<String> list = new ArrayList<>();
Solution: Always check which libraries are imported and remember that you might need to import others manually. You can use the following command to see all imported classes:
/import
4. Handling Comments Inappropriately
JShell treats comments differently, especially multi-line comments (/.../). If you forget to close a multi-line comment, it will cause JShell to hang waiting for the closing annotation.
Avoid This:
/* This is an
incomplete comment
// The prompt will hang
5. Confusing JShell with a Full IDE
Another common misunderstanding is treating JShell as a fully integrated development environment (IDE). JShell lacks the comprehensive debugging, refactoring, and code navigation features found in an IDE. While it’s great for quick tests, it’s not a substitute for proper code development.
Solution: Use JShell as an adjunct to your IDE rather than a replacement. Utilize it for exploring features or libraries and then migrate the code into your IDE for further development.
6. Ignoring Equality Checks
When checking if two objects are the same, some developers use ==
instead of equals()
. Remember, ==
compares references, while equals()
assesses value equality. This differentiation can lead to unexpected results:
String a = new String("hello");
String b = new String("hello");
System.out.println(a == b); // Outputs: false
System.out.println(a.equals(b)); // Outputs: true
7. Not Using the History Feature
JShell has a built-in history feature that allows you to retrieve previous commands. Forgetting about this feature can slow down your workflow.
- To view the command history, use the command:
/vars // This displays variables
- To repeat previous commands, you can use the arrow keys to navigate.
8. Forgetting About the exit
Command
It's easy to lose track of time while experimenting in JShell, leading to multiple open sessions. Always remember to exit gracefully without leaving processes hanging.
Use the following command:
/exit
This command properly shuts down your JShell session.
The Closing Argument
Working with JShell in JDK 9 can greatly enhance your Java development experience. By avoiding these pitfalls, you can leverage its full potential for rapid testing and learning. Remember to maintain best practices by being cautious about variable scope, imports, and proper equality checks.
JShell is a handy tool, but it is essential to recognize its limitations compared to traditional IDEs. Use it in combination with your development tools for maximum efficiency.
For further reading and to enhance your Java knowledge, consider visiting:
By mastering these areas, you can improve productivity and reduce frustration while coding in Java with JShell. Happy coding!