Streamlining XML Parsing in Java: Troubles and Solutions
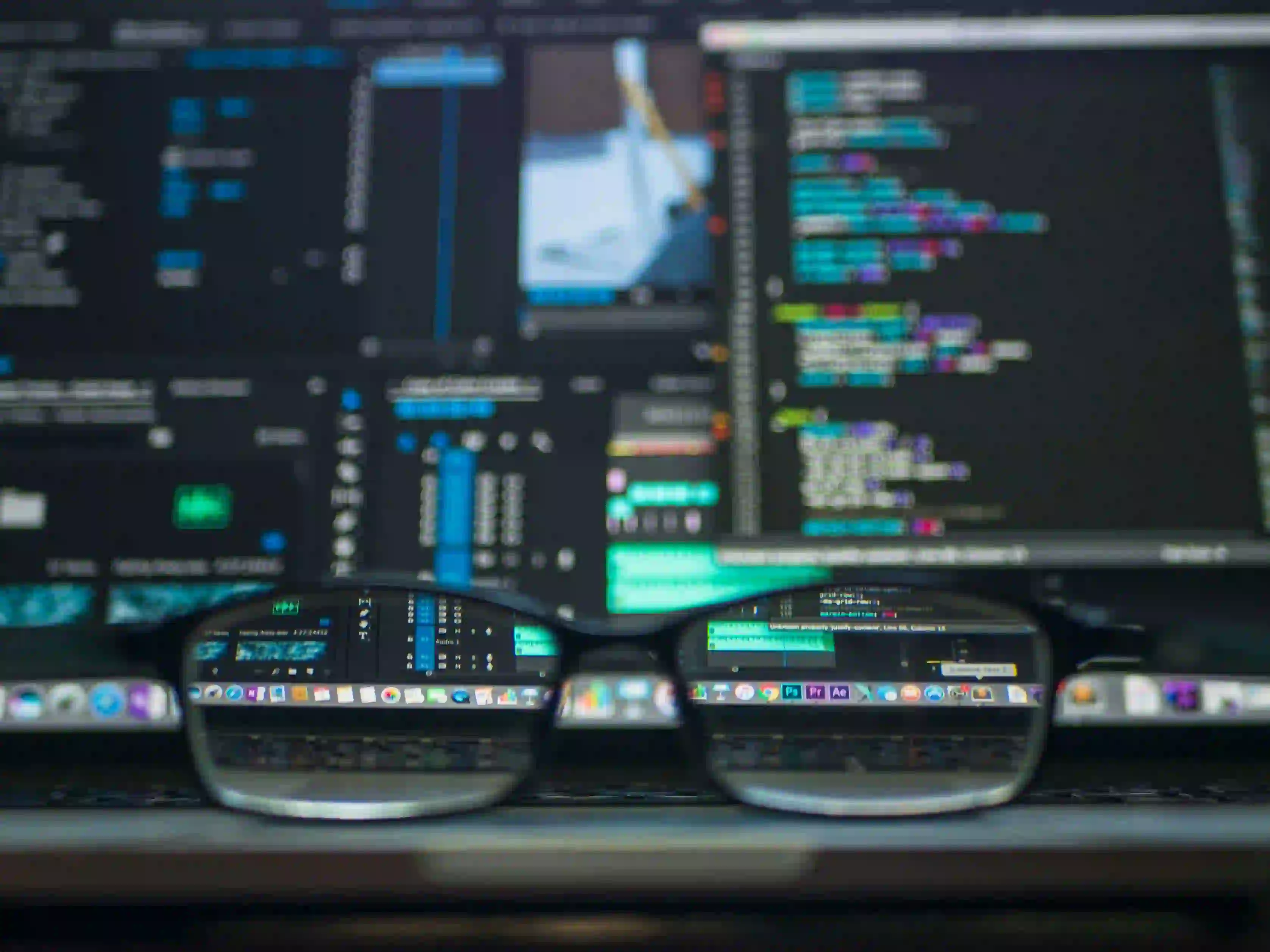
Streamlining XML Parsing in Java: Troubles and Solutions
XML (eXtensible Markup Language) is a versatile data format widely used for data interchange. Java provides a robust set of APIs for XML parsing, including DOM (Document Object Model), SAX (Simple API for XML), and StAX (Streaming API for XML). However, parsing XML can often present challenges, particularly with performance and complexity. In this blog post, we’ll explore some common troubles Java developers face when parsing XML and present practical solutions to streamline the process.
Understanding XML Parsing Methods
Before we jump into solutions, it’s essential to differentiate between the main XML parsing techniques available in Java:
-
DOM Parsing: Loads the entire XML document into memory as a tree structure. Great for small XML files but can consume a lot of memory for larger files.
-
SAX Parsing: A stream-based approach that reads XML sequentially. It doesn’t load the entire document into memory, making it more efficient for large files. However, it is less user-friendly since it doesn’t allow random access to elements.
-
StAX Parsing: Similar to SAX, it is also a stream-based API but allows for both reading (pull parsing) and writing of XML. It offers a nice balance between performance and ease of use.
Common Challenges with XML Parsing in Java
While the above APIs serve their purpose, developers often encounter several challenges:
- Memory Consumption: DOM's memory usage is a significant issue when handling large XML files.
- Sequential Access: SAX’s lack of direct access to nodes can complicate data manipulation.
- Performance Bottlenecks: Inefficient parsing logic can lead to slower applications.
By recognizing these challenges, we can address them through practical solutions.
Solutions for Effective XML Parsing
Using StAX for Efficient Parsing
As mentioned, StAX offers a more direct and controllable approach to reading XML. Here’s a simple example illustrating its usage:
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.XMLStreamReader;
import java.io.FileInputStream;
public class StAXParserExample {
public static void main(String[] args) {
try {
XMLInputFactory factory = XMLInputFactory.newInstance();
FileInputStream inputStream = new FileInputStream("example.xml");
XMLStreamReader reader = factory.createXMLStreamReader(inputStream);
while (reader.hasNext()) {
reader.next();
if (reader.isStartElement()) {
System.out.println("Start Element: " + reader.getLocalName());
}
if (reader.isCharacters()) {
System.out.println("Text: " + reader.getText().trim());
}
if (reader.isEndElement()) {
System.out.println("End Element: " + reader.getLocalName());
}
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why use StAX?
Using StAX allows us to gather data on demand rather than bulk-loading, thus overcoming the memory issues associated with DOM parsing. The above example effectively demonstrates how to read XML elements lazily and handle both start and end elements seamlessly.
Handling Large XML Files with SAX
For scenarios where XML files are particularly large and cannot fit into memory, SAX parsing is a wise choice. Below is an example of parsing XML with SAX:
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
public class SAXParserExample {
public static void main(String[] args) {
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
DefaultHandler handler = new DefaultHandler() {
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
System.out.println("Start Element: " + qName);
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException {
System.out.println("Text: " + new String(ch, start, length).trim());
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
System.out.println("End Element: " + qName);
}
};
saxParser.parse("example.xml", handler);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why opt for SAX?
SAX allows parsing large files without the need to load them entirely into memory. However, be aware of its complexity and lack of advanced querying capabilities. When you need only to read or validate vast XML data rapidly, SAX shines.
Utilizing JAXB for Unmarshalling
Another powerful approach is to use JAXB (Java Architecture for XML Binding) for marshaling and unmarshaling XML data. This method transforms XML directly into Java objects. Below is an example:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import java.io.File;
public class JAXBParserExample {
// Assuming there is a class named 'Employee' that corresponds to the XML structure
public static void main(String[] args) {
try {
File file = new File("employee.xml");
JAXBContext jaxbContext = JAXBContext.newInstance(Employee.class);
Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller();
Employee employee = (Employee) jaxbUnmarshaller.unmarshal(file);
System.out.println(employee);
} catch (JAXBException e) {
e.printStackTrace();
}
}
}
Why use JAXB?
If your XML has a predictable structure, JAXB provides a straightforward approach to parse it directly into Java objects. This leads to better type safety and keeps your code cleaner.
Performance Optimization Tips
-
Select the Right Parser: Understand your requirements before choosing between DOM, SAX, and StAX. Generally, prefer StAX or SAX for large files.
-
Streamline XML Structure: Simplifying the XML schema can significantly speed up parsing.
-
Incremental Parsing: Consider breaking the XML into chunks and processing incrementally, especially for exceedingly large datasets.
-
Profile Your Application: Use Java profiling tools to identify bottlenecks in your parsing logic. Optimize the bottleneck areas.
In Conclusion, Here is What Matters
XML parsing in Java does not have to be a cumbersome process riddled with performance woes. By understanding the strengths and weaknesses of various parsing methods—DOM, SAX, StAX, and JAXB—you can intelligently choose a strategy that meets your needs based on file size and complexity.
Explore further with resources such as XML in Java, the official Java documentation for deeper dives, and best practices.
Success in software development often hinges on choosing the right tools and approaches, and with XML parsing in Java, informed decision-making brings clarity and efficiency to your projects. Happy coding!