Overcoming Challenges in Building Scalable Java Web Services
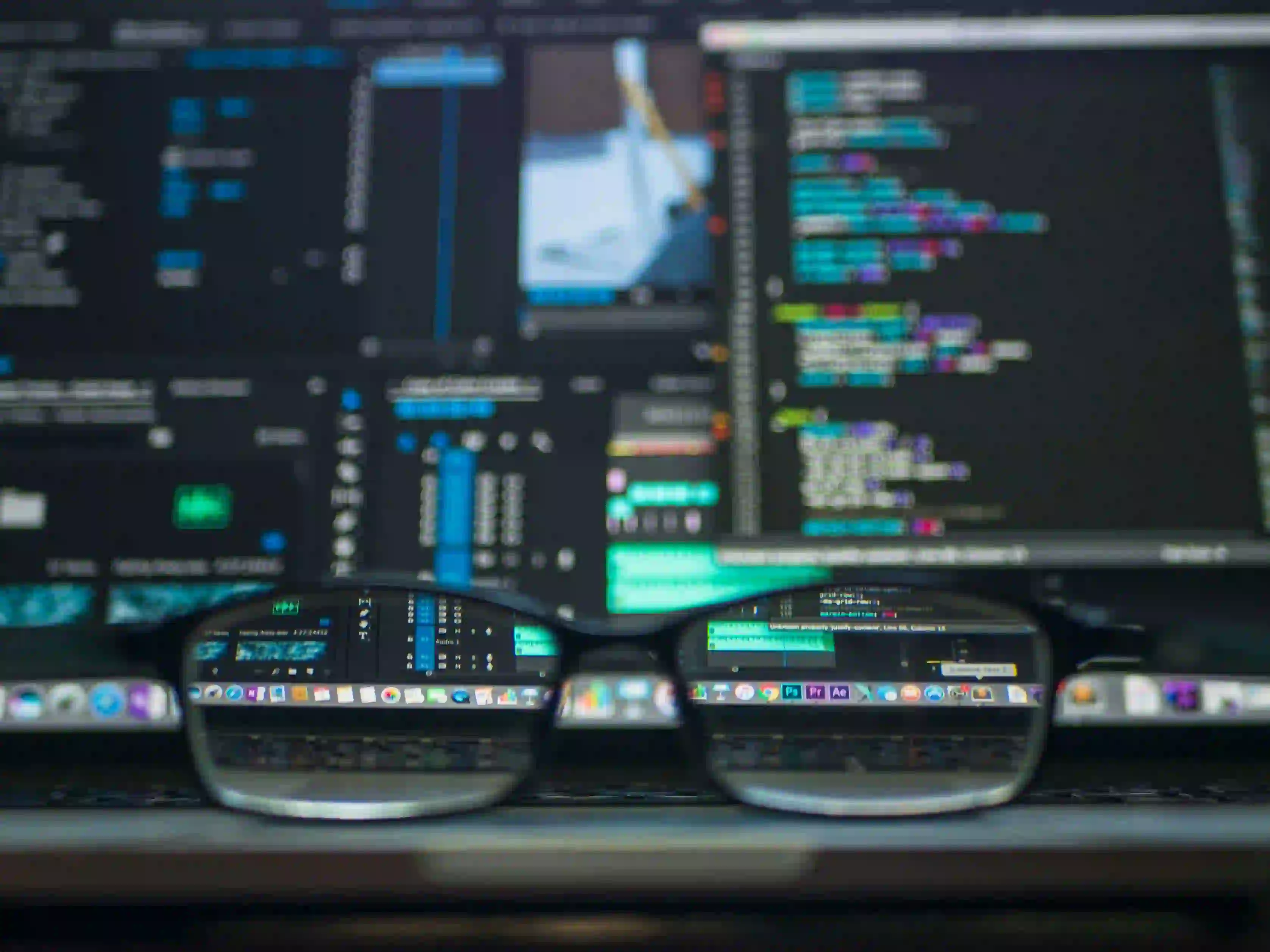
Overcoming Challenges in Building Scalable Java Web Services
Java continues to be a go-to language for developing robust, scalable web services. With its rich ecosystem and active community, Java provides developers with versatile tools that aid in building scalable applications. However, scaling a Java web service introduces several challenges that must be addressed head-on. This blog post will explore common challenges, effective strategies to overcome them, and practical tips and code snippets to aid you along the way.
Table of Contents
- Understanding Scalability
- Common Challenges
- Resource Management
- Handling Concurrency
- Load Balancing
- Data Management
- Strategies to Overcome Challenges
- Microservices Architecture
- Asynchronous Processing
- Caching Strategies
- API Gateway
- Conclusion and Best Practices
Understanding Scalability
Scalability is the capability of a system to handle a growing amount of work or its potential to accommodate growth. In the context of web services, this means being able to manage an increased load, whether in terms of users, requests, or data. Scalability can be vertical (increasing the resources of a single server) or horizontal (adding more servers).
Understanding these concepts is crucial. For an in-depth understanding of scalability in web services, check out AWS Scalability.
Common Challenges
Resource Management
One of the primary challenges in scaling Java web services is efficiently managing resources. As load increases, so do the resource demands, such as CPU, memory, and storage. Inefficient resource handling can lead to performance bottlenecks.
Handling Concurrency
Concurrency issues are an inherent challenge. Java's multithreading capabilities are powerful but can introduce complexity. Managing shared resources, avoiding race conditions, and synchronizing threads can often complicate development.
Load Balancing
As demand on your service increases, distributing that load across servers becomes necessary. An effective load-balancing mechanism is crucial for ensuring high availability and responsiveness.
Data Management
Scalability issues often stem from data handling. Rapidly increasing amounts of data require thoughtful database design, sharding strategies, and efficient querying to maintain performance.
Strategies to Overcome Challenges
Microservices Architecture
One of the most effective ways to build scalable Java web services is through a microservices architecture. Rather than a monolithic structure, a microservices approach segments your application into small, independent services.
Why Use Microservices?
- Independent Deployment: Each service can be deployed, scaled, and updated independently, reducing downtime.
- Technology Diversity: Developers can use different technologies that best fit a service's needs.
- Enhanced Resilience: If one service fails, others can continue to function, minimizing impact.
Sample Microservice Implementation
Here's a simple implementation of a microservice using Spring Boot, which allows you to build services rapidly.
@RestController
@RequestMapping("/api/greeting")
public class GreetingController {
@GetMapping("/{name}")
public Greeting greet(@PathVariable String name) {
return new Greeting("Hello, " + name + "!");
}
}
In this example, we're creating a small REST API that returns a greeting message. Spring Boot handles the underlying complexities, allowing you to focus on creating business logic.
Asynchronous Processing
Java provides several ways to handle asynchronous processing, important for improving the scalability of web services.
Why Asynchronous Processing?
- Enhanced Performance: It allows other processes to continue running while waiting for resources.
- Improved User Experience: Responsiveness can be maintained as requests are processed in the background.
Sample Asynchronous Code
Consider the use of CompletableFuture
to perform a task asynchronously:
@Service
public class UserService {
public CompletableFuture<User> findUserByIdAsync(Long id) {
return CompletableFuture.supplyAsync(() -> findUserById(id));
}
private User findUserById(Long id) {
// Simulating a delay to fetch user info from the database
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
// Handle interruption
}
return new User(id, "John Doe");
}
}
In this example, fetching the user by ID is done asynchronously, which means that the service can handle other requests while awaiting the result.
Caching Strategies
Caching is essential for scaling web services, as it dramatically reduces the latency and load on your database. It allows frequently accessed data to be stored in memory for quick access.
Why Caching?
- Faster Response Times: Reduces the need to fetch data repeatedly from the database.
- Lower Database Load: Your database can serve other requests, thus improving overall throughput.
Example of Caching
Using Spring Cache, we can easily implement caching:
@Service
public class ProductService {
@Cacheable("products")
public Product getProduct(Long id) {
// Simulate expensive database call
return findProductInDb(id);
}
}
In this example, the first time getProduct
is called with a specific ID, the product's data is fetched from the database and cached. Subsequent calls will return data from the cache, significantly enhancing performance.
API Gateway
Implementing an API Gateway can streamline various microservices, acting as a single entry point for all client requests.
Benefits of Using an API Gateway
- Simplified Client Complexity: Clients only need to communicate with one endpoint.
- Load Balancing: The gateway can intelligently distribute requests among the services.
- Cross-Cutting Concerns: It handles security, logging, and other shared concerns, reducing the burden on microservices.
Final Considerations and Best Practices
Building scalable Java web services involves understanding the core challenges such as resource management, concurrency, load balancing, and data management. Employing strategies like microservices architecture, asynchronous processing, caching, and API gateways can significantly mitigate these challenges.
Some best practices include:
- Monitoring and Logging: Continuously monitor performance metrics to identify bottlenecks.
- Testing Under Load: Simulating high-traffic scenarios can help uncover potential issues before they affect users.
- Regular Updates: Keep dependencies and libraries up to date for security and performance enhancements.
By leveraging these strategies and practices, you can effectively build Java web services that scale efficiently and remain resilient under pressure.
For more advanced techniques and practices, consider exploring Building Scalable Java Microservices with Spring Boot.
This blog post not only elucidates the challenges faced in developing scalable Java web services but also offers insightful strategies to overcome them. For any questions or further discussions, feel free to leave a comment below!