Fixing Jackson JSON Parsing: UnrecognizedFieldException Explained
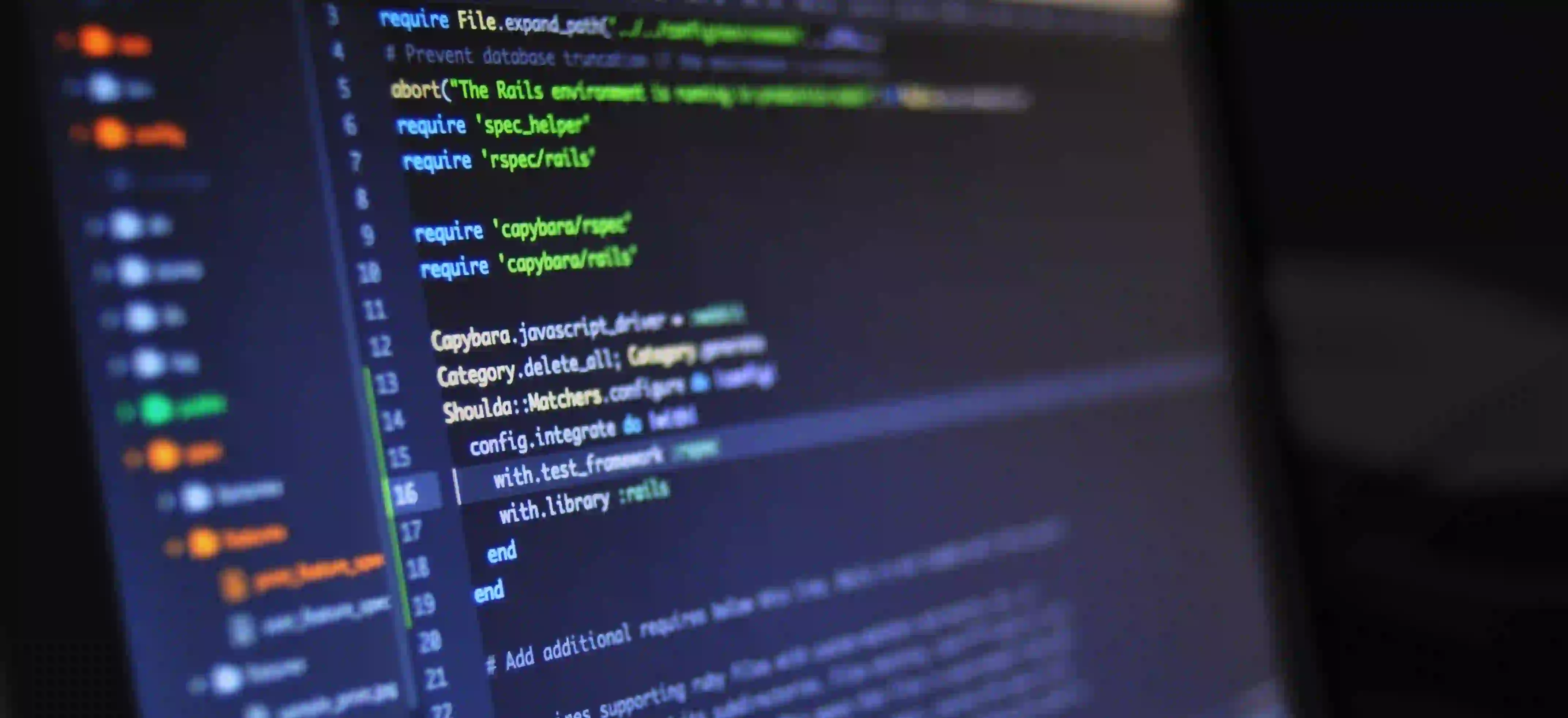
Fixing Jackson JSON Parsing: UnrecognizedFieldException Explained
Java is a robust language that often finds itself at the heart of backend development. It excels in various tasks, including data manipulation and serialization. One of the libraries that aid in this process is Jackson, which specializes in converting Java objects to JSON and vice versa. However, developers often encounter issues while using Jackson, one of the most common being the UnrecognizedFieldException
. This blog post will cover what causes this exception, how to prevent it, and how to fix it, ensuring you can navigate through your projects more efficiently.
Understanding the UnrecognizedFieldException
When you receive an UnrecognizedFieldException
, it means that Jackson has encountered a JSON field in the input that does not match any property in the Java class you are mapping these JSON fields to. This primarily happens when there's a mismatch between the JSON keys and the Java fields or when the Java class does not account for all the fields present in the incoming JSON.
Here’s the typical error you might see:
com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException: Unrecognized field "unknownField" (class com.example.MyClass), not marked as ignorable
Common Causes:
-
Field Name Mismatch: The most frequent cause is a difference between the names in the JSON and the Java object. For instance, if your JSON contains a field named
userName
, but your Java class has the fieldusername
, Jackson won't recognize it. -
Extra Fields in JSON: If the incoming JSON contains fields that don’t exist in your Java class, Jackson throws this exception unless you tell it to ignore unknown properties.
-
Missing Getters/Setters: Ensure that you have correctly defined getters and setters for the properties in your Java class. Jackson relies on these methods to access the object's fields.
-
Incorrect Field Annotations: Sometimes, annotations that control JSON serialization/deserialization could be misconfigured, leading to properties being unrecognized.
Fixing the UnrecognizedFieldException
Method 1: Ensuring Field Name Match
The simplest way to ensure compatibility is to make sure that your JSON keys match your Java object's variable names. By convention, Java uses camelCase for variable names, while JSON can use different casing.
Here is a sample of JSON data and its corresponding Java class.
Example JSON:
{
"user_name": "john_doe",
"email": "john@example.com"
}
Corresponding Java Class:
public class User {
private String userName; // Notice the camelCase
private String email;
// Getters and Setters
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
In this example, you'd encounter an UnrecognizedFieldException
because user_name
doesn't directly match userName
.
To solve this, either change your JSON structure or use annotations to handle field mapping.
Method 2: Using Jackson Annotations
You can utilize Jackson’s @JsonProperty
annotation to specify how JSON fields map to Java class fields.
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
@JsonProperty("user_name") // Maps JSON field to the Java variable
private String userName;
@JsonProperty("email")
private String email;
// Getters and Setters remain the same
}
Now, Jackson knows to convert user_name
from the JSON into the userName
variable within your User
class.
Method 3: Ignoring Unknown Properties
If you want to ignore any unknown JSON fields automatically, you can do that by using the @JsonIgnoreProperties
annotation at the class level:
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
@JsonIgnoreProperties(ignoreUnknown = true)
public class User {
private String userName;
private String email;
// Getters and Setters
}
Using this approach will allow your application to disregard any additional properties in the incoming JSON that do not match your Java class.
Method 4: ObjectMapper Configuration
You can also configure the ObjectMapper
to ignore unknown fields globally, which is useful in larger applications:
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.DeserializationFeature;
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
By setting FAIL_ON_UNKNOWN_PROPERTIES
to false
, your application will not raise an exception for any unrecognized fields across all deserialization tasks. However, this approach should be used cautiously. While it prevents runtime exceptions, it may lead to data loss where fields are inadvertently ignored.
Additional Considerations
Properly Testing Serialization/Deserialization
It's important to test your serialization and deserialization processes thoroughly. You can use JUnit for that:
import com.fasterxml.jackson.databind.ObjectMapper;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class UserTest {
ObjectMapper objectMapper = new ObjectMapper();
@Test
public void testUserDeserialization() throws Exception {
String jsonStr = "{\"user_name\": \"john_doe\", \"email\": \"john@example.com\"}";
User user = objectMapper.readValue(jsonStr, User.class);
assertEquals("john_doe", user.getUserName());
assertEquals("john@example.com", user.getEmail());
}
}
This test ensures that your Java class can handle the JSON structure correctly and will readily alert you if you need adjustments.
Key Takeaways
The UnrecognizedFieldException
in Jackson is a common issue, but fortunately, it's easy to address with the proper understanding and techniques. Always ensure field names conform to expected naming conventions, utilize Jackson’s annotations as needed, and consider global configurations judiciously.
By following the best practices outlined in this blog, you can effectively handle JSON serialization and deserialization in your Java applications, ultimately leading to a smoother development experience. For more on Jackson and JSON handling in Java, you can refer to Jackson Docs or Baeldung on Jackson.
Continue building, stay curious, and happy coding!