Common Java App Engine Issues in NetBeans: A Quick Fix Guide
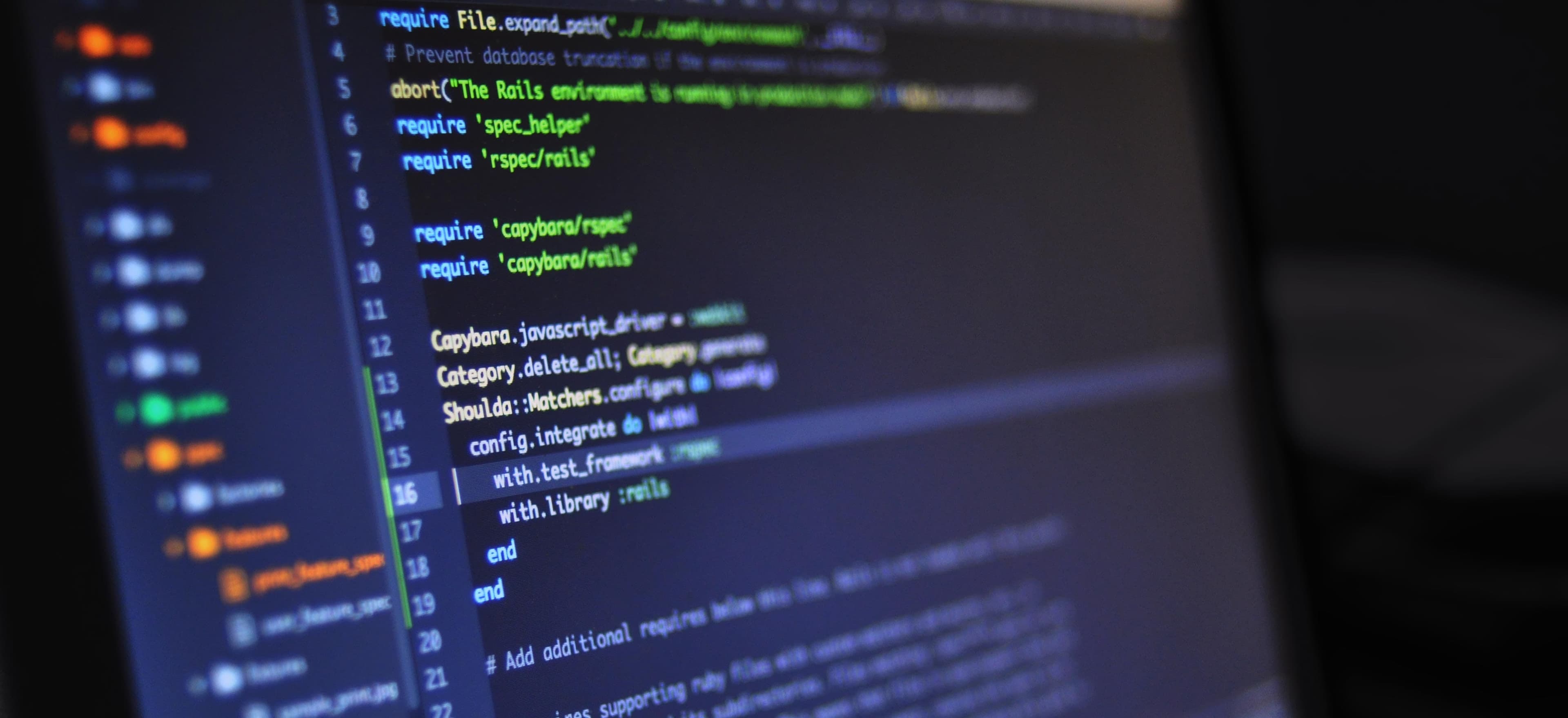
- Published on
Common Java App Engine Issues in NetBeans: A Quick Fix Guide
When working with Java on Google Cloud's App Engine, developers often rely on powerful IDEs like NetBeans for a seamless development experience. However, just like any technology stack, you may encounter common issues along the way. This guide will highlight several frequent challenges developers face when using Java App Engine in NetBeans and provide quick fixes to help you navigate these obstacles.
Prerequisites
Before diving into common issues, ensure that you have the following:
- NetBeans IDE: Updated version. You can download it from the official NetBeans site.
- Java Development Kit (JDK): Ensure JDK 8 or later is installed. The latest JDK version can be found on the Oracle website.
- Google Cloud SDK: For deploying applications. Get it here.
Issue 1: App Engine Configuration Errors
Problem
When trying to deploy, a common error is related to the appengine-web.xml
configuration file. An incorrect or missing setting can prevent deployment.
Solution
- Verify Configuration File: Check the
WEB-INF/appengine-web.xml
file for correct settings. The essential parts to focus on are:
<appengine-web-app xmlns="http://appengine.google.com/ns/1.0">
<application>your-project-id</application>
<version>1</version>
<threadsafe>true</threadsafe>
<runtime>java8</runtime>
</appengine-web-app>
- Ensure Project ID: Replace
your-project-id
with the actual project ID from your Google Cloud Console.
Why This Matters
A correctly configured appengine-web.xml
file ensures that your application is deployed correctly, with all the necessary runtime information.
Issue 2: Dependencies Not Found
Problem
If your Maven project fails to build with errors stating that some dependencies cannot be resolved, it can hinder your progress.
Solution
- Update Maven Repositories:
- Right-click on your project in NetBeans.
- Select
Reload Project
.
- Check
pom.xml
: Ensure that yourpom.xml
contains the correct dependencies. An example dependency block might look like this:
<dependencies>
<dependency>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-logging</artifactId>
<version>2.1.10</version>
</dependency>
</dependencies>
Why This Matters
Ensuring your pom.xml
is accurate and up-to-date guarantees that Maven can fetch all the required libraries, facilitating a smooth build process.
Issue 3: Local Development Environment Setup
Problem
Running your application locally may produce errors stemming from misconfigurations in your development environment.
Solution
- Check Local Development Server: Ensure that your local development server is set up correctly. Use the following command to start the local server:
gcloud app run --project=your-project-id
- Local Environment Variables: Verify if any environment variables are required for your app. You can define them in the command line or using a
.env
file.
Why This Matters
Setting up your local environment correctly allows for accurate testing and debugging before deploying to production.
Issue 4: Gradle Build Issues
Problem
If you use Gradle and encounter issues during the build process, it might result from incorrect configurations in your build.gradle
file.
Solution
- Check Your
build.gradle
: Ensure the following configuration aligns with your project’s needs:
apply plugin: 'java'
repositories {
mavenCentral()
}
dependencies {
implementation 'com.google.cloud:google-cloud-storage:2.1.5'
}
- Refresh Gradle Project: Sometimes, refreshing the project helps in resolving dependency issues.
Why This Matters
Keeping your build.gradle
file standardized allows for effective dependency management, making your build process smoother.
Issue 5: Testing and Debugging Problems
Problem
Automated tests may fail to run correctly, leading to confusion about functionality.
Solution
- JUnit Setup: Ensure you are using the correct testing framework, typically JUnit for Java projects. This may look something like:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class ExampleTest {
@Test
public void testExample() {
assertEquals("Expected Result", "Actual Result");
}
}
- Run Tests from NetBeans: Right-click on the file or test class and select
Test File
to execute the tests.
Why This Matters
Effective testing helps ensure your code runs as expected, preserving consistent application performance.
Issue 6: Deployment Failures
Problem
Sometimes the deployment may fail due to various reasons like misconfigurations or resource limits.
Solution
-
Check Deployment Logs: Utilize the Google Cloud Console to inspect logs for any messages highlighting the problem. Look specifically for messages related to instance class or memory limits.
-
Adjust Resource Settings: If resource limitation is an issue, you may want to adjust the
instance_class
in yourapp.yaml
file:
instance_class: F2
- Use Basic Tools: Familiarize yourself with the Google Cloud CLI tools to fetch deployment logs efficiently.
Why This Matters
Understanding deployment processes and tracking logs is crucial for diagnosing issues when things go wrong, ultimately ensuring a successful deployment.
Closing the Chapter
Working with Java on Google App Engine using NetBeans can present challenges, from misconfigurations to deployment failures. By recognizing common issues and employing straightforward solutions, you can streamline your development process.
For further reading, consider exploring the Google Cloud documentation and the NetBeans user guide. Keeping these resources on hand will equip you with additional knowledge and troubleshooting tips.
With patience and practice, you can effectively navigate and overcome these common issues. Happy coding!