Common SEDA Component Pitfalls in Apache Camel
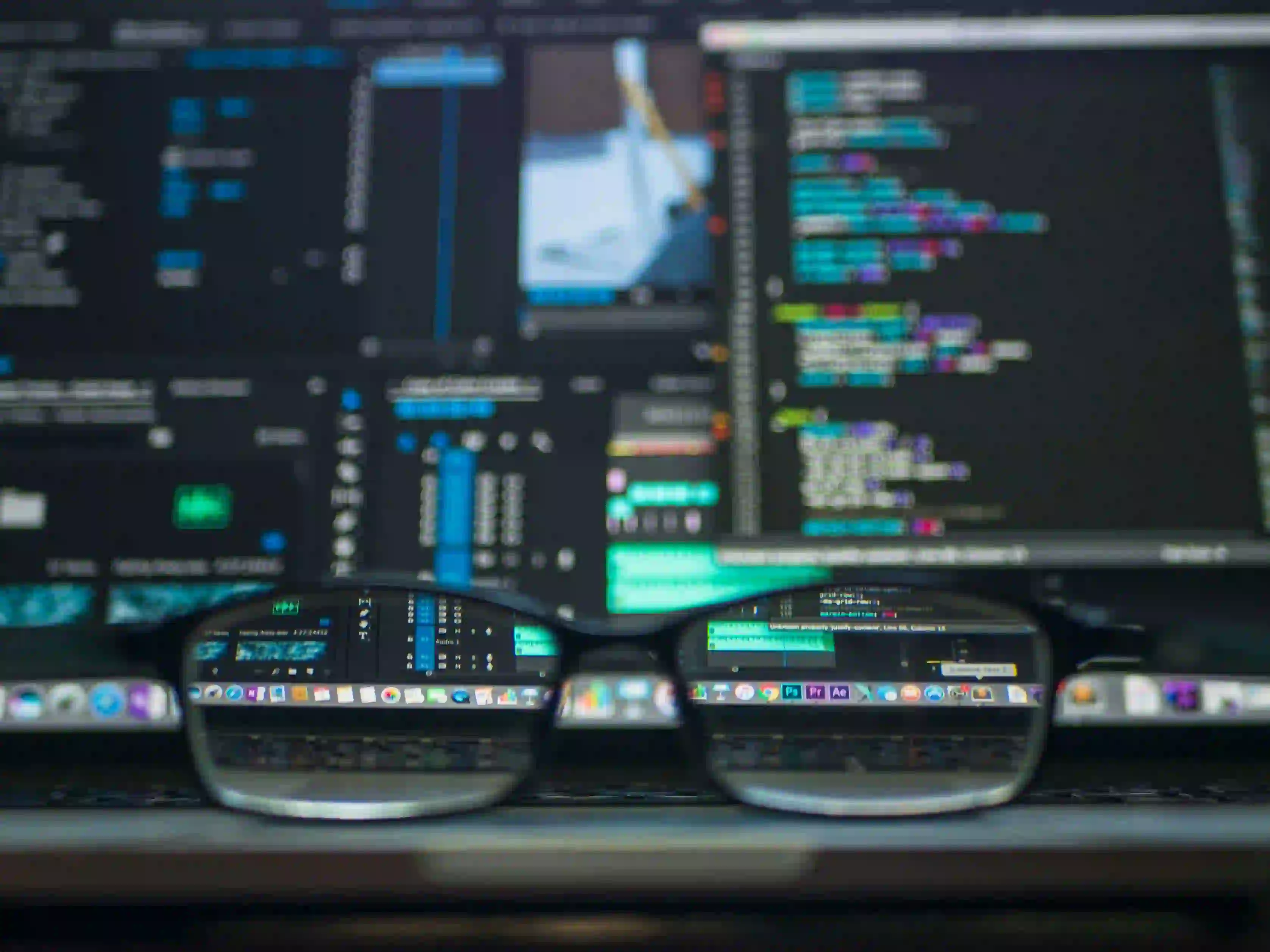
Common SEDA Component Pitfalls in Apache Camel
Apache Camel is a powerful integration framework that offers a wide range of components, one of which is SEDA (Staged Event-Driven Architecture). The SEDA component is instrumental in building event-driven applications by allowing asynchronous processing of messages. While the SEDA component brings numerous advantages, it's essential to recognize and avoid common pitfalls when using it. In this blog post, we'll detail these pitfalls while providing best practices and code examples along the way.
Understanding the SEDA Component
Before delving into the pitfalls, it's important to understand what the SEDA component does. At its core, SEDA allows you to queue messages for processing in a non-blocking, asynchronous manner. This allows the system to continue processing other tasks instead of waiting for one particular task to complete.
Basic SEDA Example
Here's a simple SEDA example that demonstrates how to set it up:
from("file:input")
.to("seda:processFile");
from("seda:processFile")
.process(exchange -> {
// Process file content
String body = exchange.getIn().getBody(String.class);
System.out.println("Processing file: " + body);
});
In this example, files from an input directory are sent to a SEDA queue named processFile
, where they are processed asynchronously. This design decouples file reading from file processing, enabling better throughput.
Team Pitfalls
Now let's look at some common pitfalls associated with the SEDA component and how to avoid them.
1. Misconfigured Queue Size
One common mistake developers make is misconfiguring the queue size. By default, SEDA creates a bounded queue with a size limit of 1000 messages. If messages exceed this limit, the producer will block or throw an exception, causing potential application failure.
Solution: Configure appropriately
from("file:input")
.to("seda:processFile?maxQueueSize=2000");
By increasing the maxQueueSize
parameter, you can accommodate more messages in the queue. However, do keep an eye on available system memory. A larger queue can lead to increased memory usage, which may result in performance degradation or crashes.
2. Ignoring Consumer Throughput
Another pitfall is assuming that a single consumer can handle the load generated by the producer. If consumers are slower than producers, the queue will rapidly fill up, leading to excessive memory consumption.
Solution: Scale Consumers
You can scale the number of consumers using the concurrentConsumers
option:
from("seda:processFile?concurrentConsumers=5")
.process(exchange -> {
// Processing logic
});
This configuration allows multiple consumers to process messages simultaneously, significantly increasing throughput and reducing chances of congestion.
3. Not Handling Exceptions Properly
Exception handling is vital in any message framework. Failing to implement proper error handling can lead to messages being lost or system stability issues.
Solution: Use Dead Letter Channel (DLC)
Integrate the Dead Letter Channel to handle failed messages gracefully.
errorHandler(deadLetterChannel("seda:errorQueue")
.maximumRedeliveries(3)
.redeliveryDelay(1000));
In this code snippet, messages that fail after three attempts will be routed to the errorQueue
, allowing administrators to inspect and address issues without affecting overall application flow.
4. Inefficient Message Size Management
While SEDA works well for many scenarios, sending excessively large messages through SEDA can hinder performance. Large messages consume more memory and take longer to process.
Solution: Chunk Your Data
Instead of sending large payloads, consider chunking the data into manageable sizes. This reduces memory overhead and leads to faster processing:
from("file:input")
.process(exchange -> {
String[] chunks = splitLargeFile(exchange.getIn().getBody(String.class));
for (String chunk : chunks) {
exchange.getContext().createProducerTemplate().sendBody("seda:processFile", chunk);
}
});
In this example, we split a large file into chunks before sending it to the SEDA queue, optimizing performance for each piece.
5. Failure to Monitor and Tune
Ignoring the performance metrics from your SEDA components can lead to unaware inefficiencies. The lack of monitoring can hide performance bottlenecks that impact the user experience.
Solution: Implement Monitoring
You can utilize tools like Hawtio, JMX, or custom logging to track SEDA performance metrics. This monitoring helps you to identify when queues start to fill up and how quickly messages are processed.
from("seda:processFile")
.to("log:processedMessages");
Adding logging for processed messages can help you track the system's performance in real time.
The Bottom Line
The SEDA component in Apache Camel, when used wisely, can enhance the robustness and scalability of your applications. However, by being aware of these common pitfalls and implementing the proposed solutions, you can reinforce your application’s performance and stability.
For a deep dive into the SEDA component and other Camel components, check out the Apache Camel Documentation and explore best practices for building scalable integration solutions.
If you're just getting started with Apache Camel, take some time to familiarize yourself with its extensive capabilities. Happy coding!