Mastering Java 8: Converting Lists to String Simplified
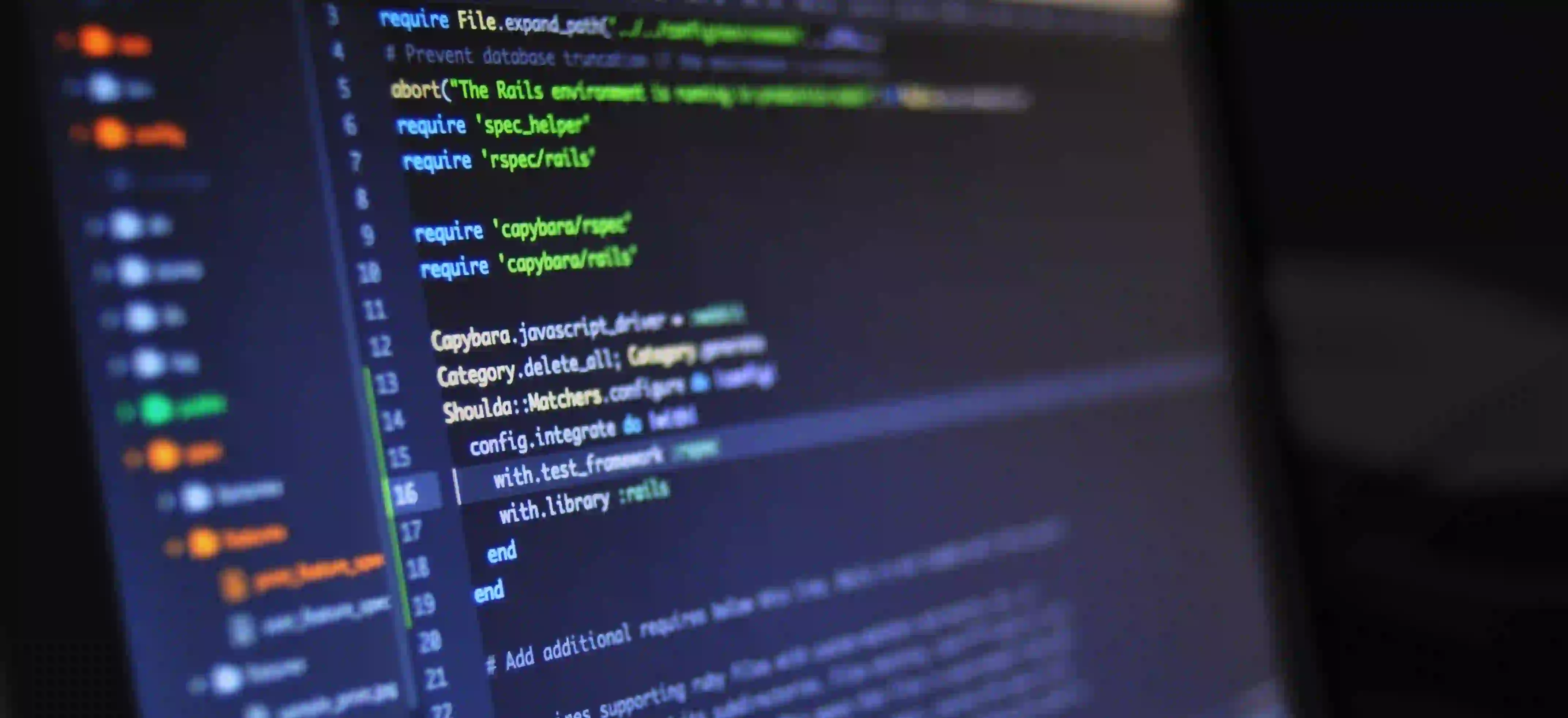
Mastering Java 8: Converting Lists to Strings Simplified
Java 8 introduced various powerful features that can significantly enhance our coding experience. One of these features is the Stream API, which provides an efficient way of manipulating collections. In this article, we will dissect how to convert lists to strings using the Stream API, focusing specifically on Java 8 and beyond. This will not only simplify your code but also make it more readable and expressive.
Table of Contents
- Understanding the List Interface
- The Need for Converting Lists to Strings
- Introduction to the Stream API
- Basic Methods for Converting Lists to Strings
- Advanced Techniques
- Performance Considerations
- Conclusion
- Further Reading
1. Understanding the List Interface
The List interface in Java represents an ordered collection of elements, allowing duplicates and maintaining the insertion order. Lists can be implemented using various classes such as ArrayList
, LinkedList
, and Vector
. Here's a simple example:
import java.util.ArrayList;
import java.util.List;
public class ListExample {
public static void main(String[] args) {
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
System.out.println(fruits);
}
}
In this example, we created a list of fruits and printed it out. However, there are times when you need the contents of a list represented as a single string.
2. The Need for Converting Lists to Strings
The conversion of lists to strings can be useful in numerous scenarios, such as:
- Logging: You might want to log a list's contents for debugging purposes.
- Display: Presenting list data in a user interface in a readable format.
- Data transfer: Converting list data to a single string for sending over a network.
3. Introduction to the Stream API
The Stream API is one of the most important abstractions introduced in Java 8. It allows for functional-style operations on streams of elements. With streams, we can process data in a more fluent and expressive manner.
Benefits of Using Streams
- Conciseness: Less boilerplate code.
- Parallel Processing: Improved performance by utilizing multicore architectures.
- Flexibility: Stream operations can be chained together.
4. Basic Methods for Converting Lists to Strings
Now, let's dig into practical examples. The simplest method to convert a list to a string involves using String.join()
, which combines elements into a single string separated by a specified delimiter.
Using String.join()
Here’s how you can do it:
import java.util.Arrays;
import java.util.List;
public class ListToString {
public static void main(String[] args) {
List<String> fruits = Arrays.asList("Apple", "Banana", "Cherry");
String result = String.join(", ", fruits);
System.out.println(result); // Output: Apple, Banana, Cherry
}
}
In this snippet, String.join(", ", fruits)
concatenates elements of the list, using ", " as the delimiter.
Advantages of String.join()
- Simplicity: Minimal lines of code needed.
- Configured Delimiters: You can easily change the delimiter based on requirements.
5. Advanced Techniques
While String.join()
is effective for simple conversions, the Stream API allows for more complex operations when needed.
Using Streams for Custom Formatting
If you require custom formatting or conditions while converting a list to a string, the Stream API is your go-to solution. Here is an example:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class AdvancedListToString {
public static void main(String[] args) {
List<String> fruits = Arrays.asList("apple", "banana", "cherry");
String result = fruits.stream()
.map(String::toUpperCase) // Convert each element to upper case
.collect(Collectors.joining(", ", "[", "]"));
System.out.println(result); // Output: [APPLE, BANANA, CHERRY]
}
}
Breakdown of the Code:
- map(String::toUpperCase): Transforms each element to its uppercase variant.
- collect(Collectors.joining(", ", "[", "]")): Joins the elements into a single string with a prefix "[" and a suffix "]".
Custom Predicate Example
Suppose you only want to include fruits containing the letter 'a':
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class FilteredListToString {
public static void main(String[] args) {
List<String> fruits = Arrays.asList("apple", "banana", "cherry");
String result = fruits.stream()
.filter(fruit -> fruit.contains("a")) // Filter by condition
.collect(Collectors.joining(", ", "{", "}")); // Format the string
System.out.println(result); // Output: {apple, banana}
}
}
6. Performance Considerations
When working with large datasets, performance issues might arise. The Stream API can process elements in parallel to speed up the operations:
String result = fruits.parallelStream()
.filter(fruit -> fruit.contains("a"))
.collect(Collectors.joining(", "));
Key Takeaway
- Use
parallelStream()
for performance optimization on large lists. - Always evaluate whether parallelism is beneficial for your specific use case.
7. Conclusion
Converting lists to strings in Java can be done quickly and efficiently, especially with the introduction of the Stream API in Java 8. Whether you prefer the simplicity of String.join()
or the expressive capability of the Stream API, both approaches can suit various needs based on complexity.
From basic string conversions to complex manipulations, Java 8's rich features enable developers to write cleaner, more maintainable code. As you embark on this journey of mastering Java, recognizing these powerful tools can elevate your programming skills.
8. Further Reading
By utilizing the tools and techniques discussed in this article, you can become adept at converting lists to strings in Java 8, thereby improving your overall ability as a Java developer. Happy coding!