Overcoming Challenges with Synchronous Requests in Spring WebClient
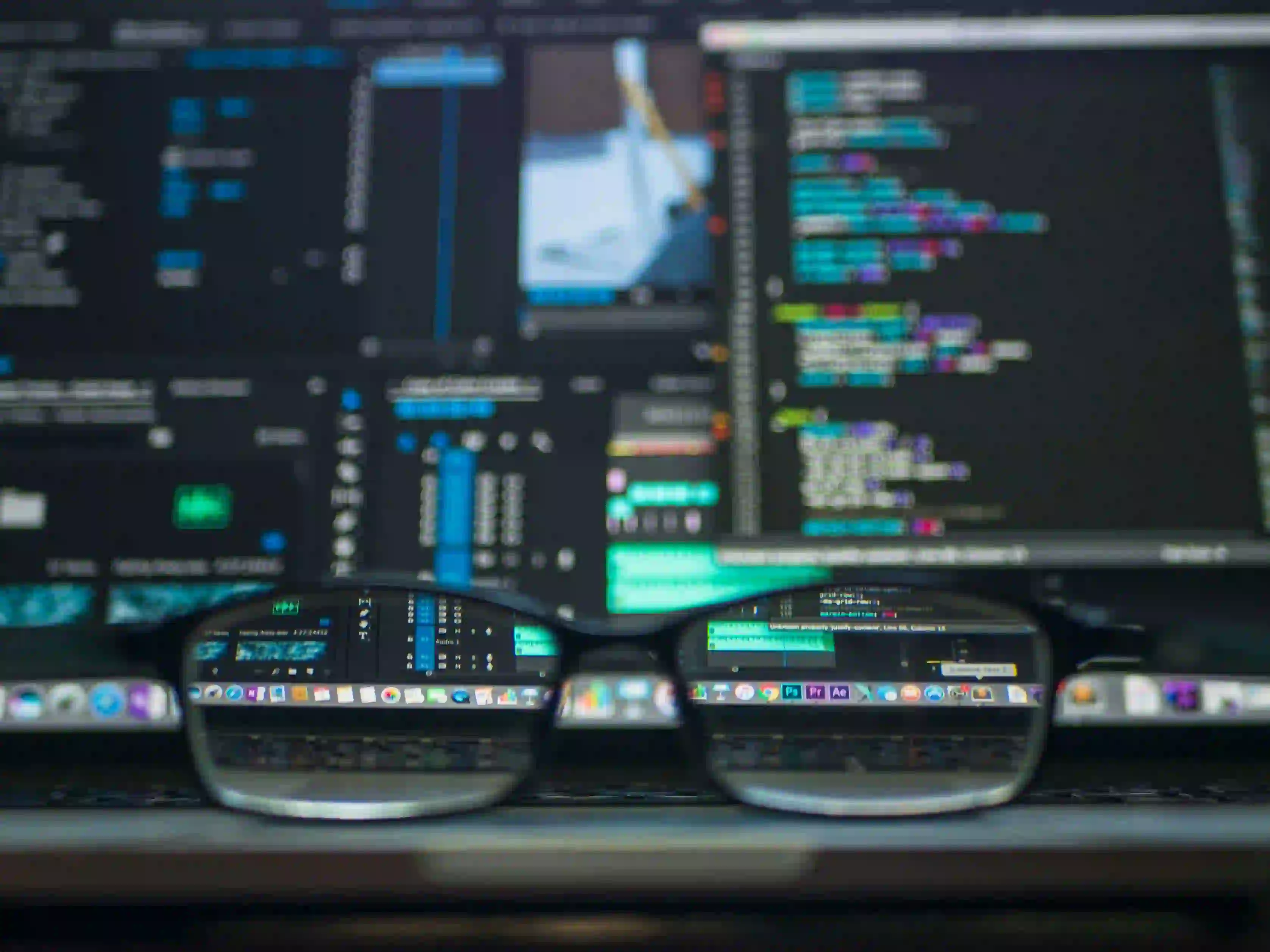
Overcoming Challenges with Synchronous Requests in Spring WebClient
Spring WebClient offers a powerful tool for making HTTP requests and is part of the Spring 5 framework. It supports both synchronous and asynchronous processing. However, the challenge arises when you try to use it in a synchronous manner, as this can lead to blocking behaviors that may not be optimal for performance. In this blog post, we’ll explore the intricacies of synchronous requests using Spring WebClient, discuss potential challenges, and provide actionable solutions.
What is Spring WebClient?
Before diving into synchronous requests, let’s quickly recap what Spring WebClient is. Spring WebClient is a non-blocking, reactive client for performing HTTP requests. It is a part of the reactive programming model introduced in Spring 5, and it allows developers to build applications that can handle many simultaneous connections without consuming many resources.
Key Features of Spring WebClient
- Non-blocking communication: Asynchronous requests using Project Reactor.
- Rich API: Supports various HTTP methods, headers, and body types.
- Customizable: Easily configurable for authentication, error handling, and request manipulation.
- Streaming support: Efficiently handle large data volumes.
Making Synchronous Requests with Spring WebClient
While Spring WebClient is primarily designed for reactive, non-blocking programming, you may find situations in which you want to make synchronous requests for simplicity or if integrating with legacy systems.
The Challenge with Synchronous Requests
Using Spring WebClient for synchronous purposes can lead to undesired blocking behavior. This defeats the purpose of using a non-blocking client and can adversely affect application performance. Here are a couple of common challenges you might face:
- Thread Blocking: When making synchronous requests, the calling thread waits for the response, which can lead to resource contention.
- Error Handling: Managing exceptions in synchronous calls can be trickier, as it often revolves around traditional try-catch blocks.
Example: Synchronous Request Using WebClient
Here’s how to make a synchronous request using Spring WebClient:
import org.springframework.web.reactive.function.client.WebClient;
import org.springframework.web.reactive.function.BodyExtractors;
import reactor.core.publisher.Mono;
public class SynchronousClient {
private final WebClient webClient;
public SynchronousClient(WebClient.Builder webClientBuilder) {
this.webClient = webClientBuilder.baseUrl("https://api.example.com").build();
}
public String fetchData() {
// Create the synchronous request
Mono<String> responseMono = webClient.get()
.uri("/data")
.retrieve()
.bodyToMono(String.class);
// Block to get the data, effectively making this synchronous
return responseMono.block();
}
}
Why is Blocking Not Ideal?
Blocking the thread with responseMono.block()
seems convenient, but it means your application will wait until the data is available. This can lead to scalability issues under high load. Instead, consider whether a non-blocking approach can work in your architecture.
Making the Best of Synchronous Approaches
While synchronous requests can be challenging, you can still implement them effectively with careful planning.
1. Connection Pooling
To resolve potential bottlenecks caused by threads waiting for responses, use connection pooling. Configure your WebClientBuilder like so:
import org.springframework.web.reactive.function.client.WebClient;
import reactor.netty.http.client.HttpClient;
import reactor.netty.resources.ConnectionProvider;
// Configure Connection Pool
ConnectionProvider provider = ConnectionProvider.builder("custom")
.maxConnections(50)
.build();
HttpClient httpClient = HttpClient.create(provider);
WebClient webClient = WebClient.builder()
.clientConnector(new ReactorClientHttpConnector(httpClient))
.baseUrl("https://api.example.com")
.build();
This approach allows multiple synchronous requests to be handled without immediately exhausting server resources.
2. Proper Error Handling
Handling errors while making synchronous requests requires awareness. Use a try-catch block effectively:
public String fetchData() {
try {
Mono<String> responseMono = webClient.get()
.uri("/data")
.retrieve()
.bodyToMono(String.class);
return responseMono.block();
} catch (Exception e) {
// Handle errors gracefully
return "Error occurred: " + e.getMessage();
}
}
3. Timeouts
Set reasonable timeouts to prevent indefinite waits that can lead to issues with application responsiveness.
webClient.get()
.uri("/data")
.retrieve()
.bodyToMono(String.class)
.timeout(Duration.ofSeconds(5)) // Set a timeout
.block();
4. Customize the WebClient
Make your WebClient instance reusable and efficient by customizing it for specific scenarios, such as adding interceptors for logging or headers.
Best Practices for Using Spring WebClient
- Use Asynchronous Patterns: Whenever possible, prefer asynchronous requests. Spring WebClient is powerful when utilized this way.
- Keep Connections Alive: Use HTTP/2 to reuse connections effectively and minimize latency.
- JSON Processing: Utilize libraries like Jackson for effective serialization/deserialization of JSON data.
- Profiling and Monitoring: Use tools to monitor your application's performance. Consider Spring Actuator for valuable insights into application metrics.
A Final Look
Spring WebClient is a powerful tool in the Spring ecosystem, but using it synchronously can lead to performance challenges. Understanding how to manage these challenges is crucial. From leveraging connection pooling to proper error handling, you can make your synchronous requests more robust and manageable.
Switching to non-blocking patterns is recommended where feasible. The benefits of asynchronous programming, including scalability and responsiveness, generally outweigh the simplicity of synchronous calls.
By implementing best practices, you can fine-tune your Spring WebClient usage and ensure that you are making the most out of this fantastic tool in building modern web applications.
For further reading, you may want to explore the official Spring WebClient Documentation to dive deeper into its capabilities.
Happy coding!