Mastering Groovy Shell: Troubleshooting Common Pitfalls
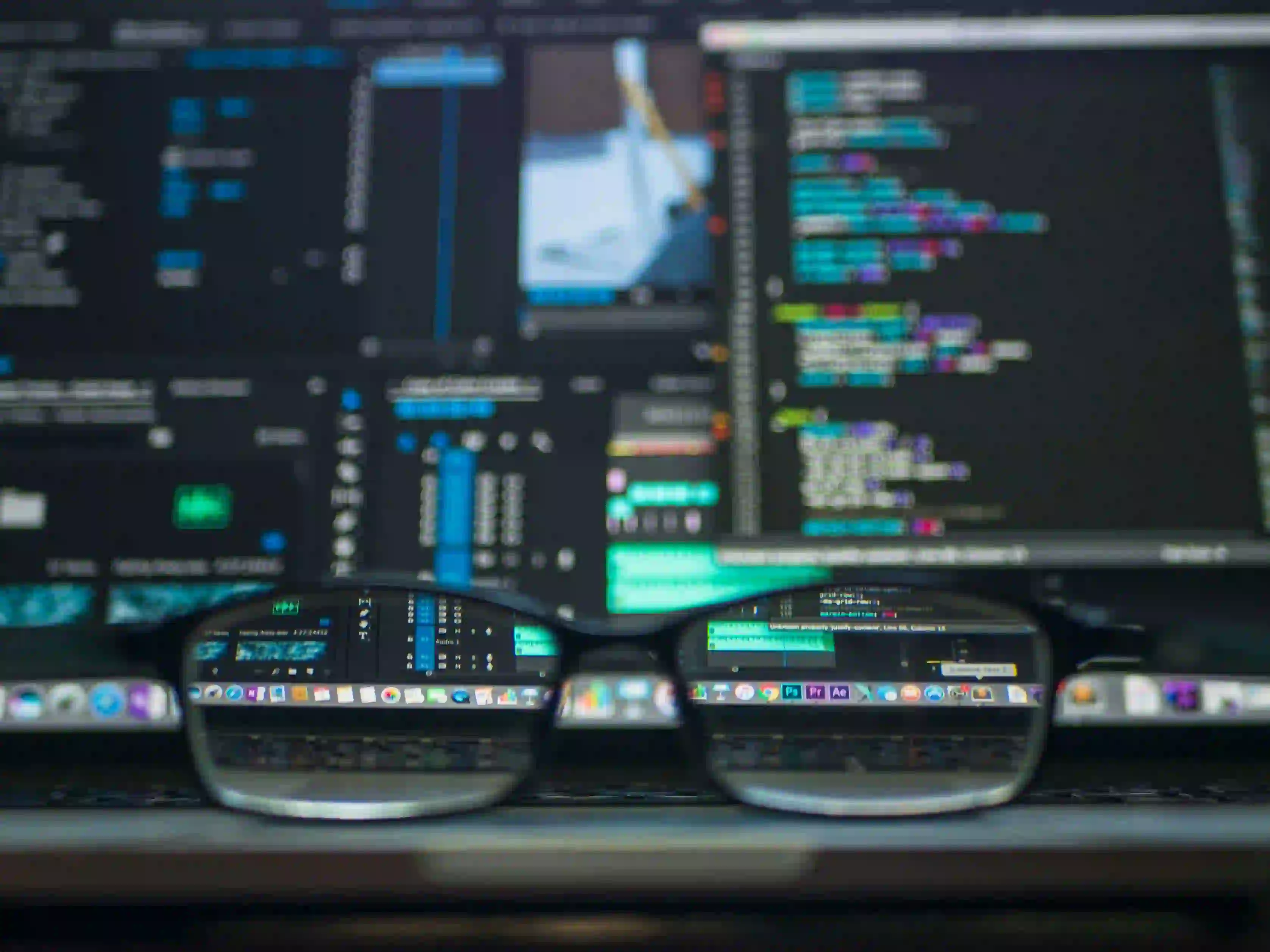
Mastering Groovy Shell: Troubleshooting Common Pitfalls
The Groovy shell, known as groovysh
, is a powerful tool that allows developers to execute Groovy code interactively. It provides a convenient way to test snippets, run scripts, and even perform complex scripting tasks. However, like any other tool, it has its fair share of pitfalls. In this blog post, we will explore common challenges encountered while using Groovy Shell and how to troubleshoot them effectively.
What is Groovy Shell?
Before diving into troubleshooting, let's briefly cover what Groovy Shell is. Groovy is a dynamic language for the Java platform, and groovysh
is its interactive command-line interface. This tool allows developers to quickly test code snippets and examine results without the need for a full-fledged development environment.
To start the Groovy Shell, ensure you have Groovy installed and simply type:
groovysh
You will be greeted with the Groovy shell prompt, ready to execute your commands.
Common Pitfalls and Troubleshooting Tips
1. ClassNotFoundException
Problem:
One of the most frequent errors encountered in Groovy Shell is ClassNotFoundException
. This typically occurs when you try to use a class that is not in the classpath.
Solution:
To resolve this, you can add jars or directories to the classpath using the --classpath
option while starting groovysh
.
groovysh --classpath /path/to/your/jarfile.jar
Additionally, remember to check if the libraries you want to use are actually available and properly referenced.
Code Snippet:
Here's an example illustrating this issue:
import com.example.MyClass
def instance = new MyClass()
println instance.someMethod()
If MyClass
is not accessible, you would get a ClassNotFoundException
. Always confirm your classpath settings to avoid this.
2. Script Execution Issues
Problem: Sometimes, scripts do not execute as expected within Groovy Shell, leading to confusion. This is often due to forgotten semicolons or incorrect syntax.
Solution: Ensure that each command or statement is properly terminated. Groovy does not require semicolons, but in a shell environment, they can be helpful for separating commands.
Code Snippet:
Let's say you have a simple script example:
def greet(name) {
"Hello, ${name}!" // Groovy’s string interpolation
}
println greet("World")
If you attempt to run multiple statements without separation, it could lead to a syntax error. Replacing the last line with:
println greet("World"); // Explicitly terminating the statement
Can help clarify your intentions.
3. Bindings and Context Issues
Problem:
Another common issue arises related to variable bindings. It is crucial to understand the scope of your variables when working within groovysh
.
Solution: To avoid variable binding issues, always declare your variables within the appropriate scope to prevent unexpected behavior.
Code Snippet:
Consider this example:
def count = 10
if (count > 5) {
def message = "Count is greater than 5"
}
println message // This would cause an error since message is not in scope
To address the variable binding:
def message
if (count > 5) {
message = "Count is greater than 5"
}
println message // Now it works!
4. Handling Closures and Methods
Problem: Another common issue is struggling with closures when it's time to call them. Closures must be defined and invoked carefully to avoid runtime errors.
Solution:
Make sure closures are defined properly and invoked with the correct context using call()
or by simply writing the closure name followed by parentheses.
Code Snippet:
Here's how you might define and use a closure:
def myClosure = { name ->
"Hello, ${name}!"
}
// Correct usage
println myClosure("Groovy")
If you forget to invoke the closure, you might wonder why nothing happens.
5. Interactive Mode and Output
Problem: While working in the interactive mode, one might find the output overwhelming if many lines are printed.
Solution:
You can control output using Groovy's built-in methods like println
, print
, or by using logging mechanisms. For a better experience, keep your outputs succinct.
Code Snippet:
You can control verbosity like this:
println "Processing data..." // Print to console
// Simulate processing
(1..5).each {
println "Processing item: ${it}"
}
This way, you’ll get a clear and concise output, avoiding clutter.
Using Resources for Further Learning
To deepen your understanding of Groovy and Groovy Shell, consider checking out these resources:
A Final Look
Mastering the Groovy Shell can significantly enhance your development productivity. By understanding common pitfalls like classpath issues, binding scope, and closure invocation, you can streamline your workflow. Remember, encountering errors is part of the process; how you handle them will determine your proficiency with Groovy Shell.
Continue exploring, experimenting, and enjoy the flexibility that Groovy offers! Happy Groovy coding!