Empowering Java Apps: Boosting Accessibility for All Users
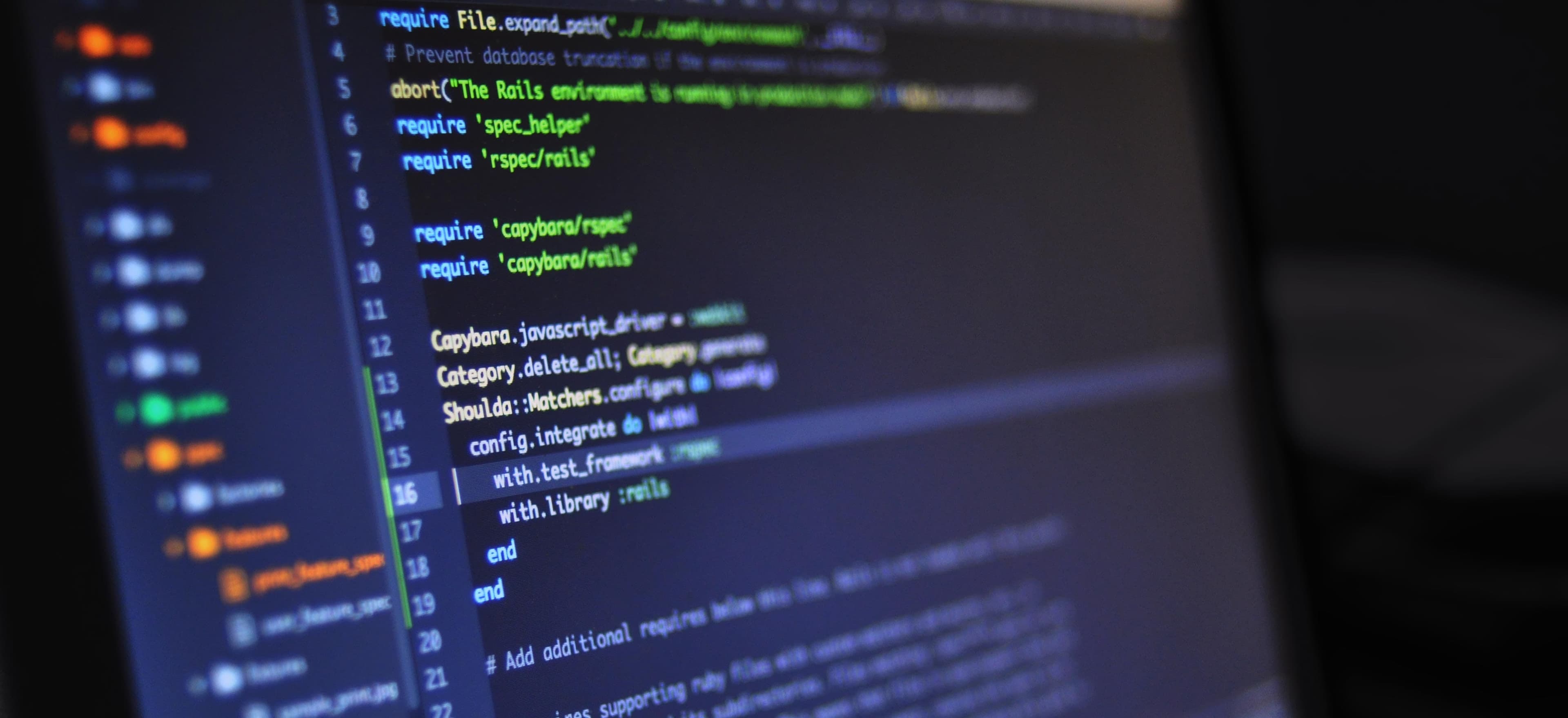
- Published on
Empowering Java Apps: Boosting Accessibility for All Users
In an increasingly digital world, ensuring that applications are accessible to everyone, including those with disabilities, is vital. Java, being one of the most versatile programming languages, provides various tools and libraries to enhance the accessibility of applications. In this blog post, we will explore how you can empower your Java applications by integrating accessibility features that cater to all users.
Understanding Web Accessibility
Before heading into the technical aspects of implementing accessibility in Java, it is crucial to understand what accessibility means. Web accessibility refers to designing websites and applications that are usable by individuals with disabilities. This includes visual impairments, hearing challenges, motor disabilities, and cognitive limitations.
According to the article Breaking Barriers: Enhancing Website Accessibility Now, web accessibility is not just a compliance issue but also a fundamental aspect of creating an inclusive environment. By making your Java applications accessible, you tap into a wider user base while enhancing the overall user experience.
Why Accessibility Matters in Java Applications
Before diving into code, let's discuss why integrating accessibility is important:
- Inclusivity: By considering accessibility, you ensure that users of diverse abilities can benefit from your application.
- Legal Compliance: Many regions have legal requirements related to accessibility that you must adhere to, minimizing legal risks.
- Better User Experience: Accessibility enhancements often lead to a more intuitive user experience, benefiting all users, not just those with disabilities.
- Broader Audience: By making your apps accessible, you expand your user base, which can lead to increased traffic and higher revenue.
Tools and Libraries for Creating Accessible Java Applications
Java offers several libraries and APIs that can help enhance accessibility in your applications. Here, we'll explore a few dominant ones:
1. Java Accessibility API
The Java Accessibility API provides interfaces and classes that make it easier to build accessible applications. It supports assistive technologies (AT), allowing tools like screen readers to interact with your application efficiently.
Here’s a simple example of making a GUI label accessible:
import javax.swing.*;
import java.awt.*;
public class AccessibleLabelExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Accessible Label Example");
JLabel label = new JLabel("Name:");
label.setDisplayedMnemonic('N'); // Set mnemonic for screen readers
label.getAccessibleContext().setAccessibleDescription("Input for the Name field"); // Description for AT
JTextField textField = new JTextField(20);
frame.setLayout(new FlowLayout());
frame.add(label);
frame.add(textField);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
}
Why This Matters:
- Mnemonic: The mnemonic allows users to quickly focus on the label with keyboard shortcuts.
- Accessible Description: Sounding out descriptions is crucial for screen-reader users, aiding their navigation through your application.
2. Swing and JavaFX Accessibility Enhancement
Swing and JavaFX are two GUI toolkits in Java. Both have methods for accessibility improvements.
JavaFX Example
Here's how you can incorporate accessibility measures in JavaFX:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class AccessibleJavaFXExample extends Application {
@Override
public void start(Stage primaryStage) {
Label label = new Label("Click the button:");
Button button = new Button("Submit");
// Add accessibility properties
button.setAccessibleRoleDescription("A button for submitting the form");
VBox vbox = new VBox(label, button);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Accessible JavaFX Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why This Matters:
- Adding an accessible role description can significantly improve the communication between your JavaFX application and the assistive technology in use.
Implementing Keyboard Navigation
Another important aspect of accessibility is ensuring that your Java application is usable via keyboard alone. This is essential for users who may have difficulty using a mouse.
Consider this simple way to navigate through components:
import javax.swing.*;
public class KeyboardNavigationExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Keyboard Navigation Example");
JButton button1 = new JButton("First Button");
JButton button2 = new JButton("Second Button");
button2.setMnemonic('S'); // Short-cut to activate the second button
frame.setLayout(new java.awt.GridLayout(0, 1));
frame.add(button1);
frame.add(button2);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
}
Why This Matters:
- Mnemonics: Assigning mnemonics allows users to navigate applications using keyboard shortcuts, crucial for users with motor disabilities.
Testing for Accessibility
After implementing accessibility features, testing them is essential:
- Automated Testing Tools: Utilize tools like WAVE and axe to audit the accessibility of your application.
- User Testing: Engage users with disabilities to test your application. Their feedback can provide invaluable insights.
The Closing Argument
Enhancing the accessibility of Java applications not only widens your audience but also fulfills moral and legal responsibilities to create an inclusive digital environment. By using the Java Accessibility API, implementing keyboard navigation, and testing your application thoroughly, you can make significant strides towards achieving this mission.
For further insights on website accessibility, you can check this resourceful article, Breaking Barriers: Enhancing Website Accessibility Now. Empower your Java apps and make them usable for everyone. With the right approach and tools, accessibility is within your reach.
Call to Action
Start implementing these strategies in your applications today! Engage with your users, prioritize their needs, and ensure that your Java applications empower everyone who wishes to access them.
This blog post serves as a guideline for developers looking to improve their Java applications' accessibility, ensuring that technology works for everyone, regardless of their abilities. Each accessible feature you add paves the way for a more inclusive digital future.
Checkout our other articles