Avoid These Java-Specific Setup Mistakes for Your Dev Machine
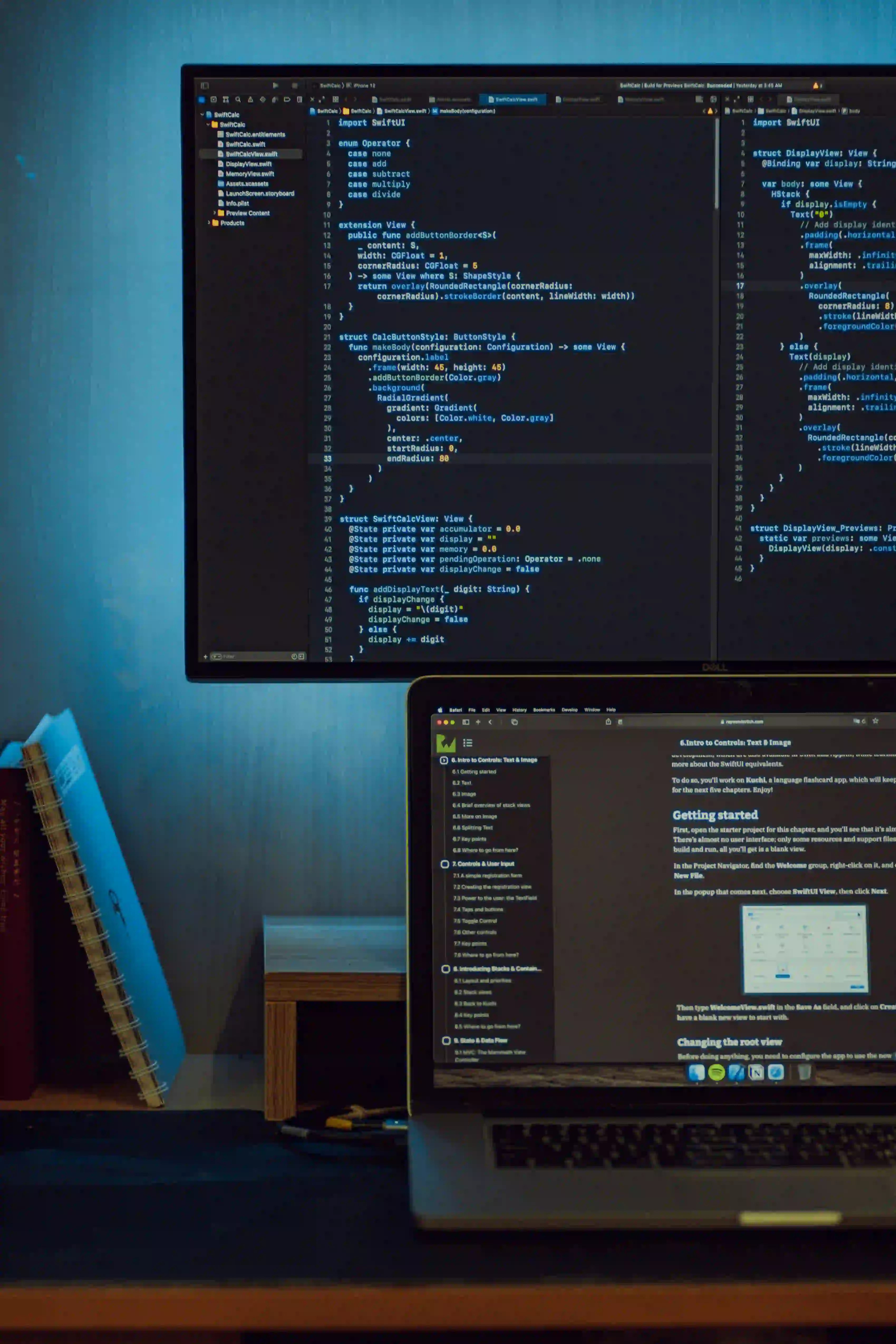
Avoid These Java-Specific Setup Mistakes for Your Dev Machine
When it comes to setting up your development machine for Java, there's a lot at stake. A well-configured environment can vastly improve your coding efficiency, while a poorly set up one can lead to frustrating errors and wasted time. In this post, we’ll explore some common mistakes specifically related to Java development. Along the way, we will also link to 5 Common Pitfalls When Setting Up Your Dev Machine for broader context.
1. Ignoring Java Version Management
Java is a versatile language that frequently updates, with new features and performance improvements rolled out constantly. One of the biggest mistakes you can make is ignoring Java version management.
Why This Matters
Different projects may depend on different versions of Java. If you're working on legacy applications, they might still rely on older versions, while new projects should incorporate the latest LTS (Long-Term Support) versions. Failing to manage your Java versions can cause compatibility issues.
Solution: Use SDKMAN!
One of the best tools for managing different versions of Java on your machine is SDKMAN! Here’s how to install it:
curl -s "https://get.sdkman.io" | bash
After you have SDKMAN! installed, you can install multiple versions with:
sdk install java <version>
sdk use java <version>
This command lets you switch between different installed versions easily.
2. Not Setting Up a Build Tool
In Java development, not using a build tool is like trying to navigate through a maze with no map. Tools such as Maven or Gradle simplify project management and ensure that your dependencies are well handled.
Why This Matters
A build tool automates tasks related to compiling your code, managing dependencies, and packaging your application. Without it, you might find yourself manually handling dependencies, which can lead to conflicts and messy configurations.
Example: Using Maven for Dependency Management
If you choose Maven, you can start a new project with:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
The pom.xml
file generated by Maven describes your project and its dependencies:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
</project>
This configuration file is powerful. By declaring your dependencies here, Maven manages their versions and downloads them automatically when you build your project.
3. Failing to Configure Your IDE Properly
A proper Integrated Development Environment (IDE) setup can significantly boost your productivity. However, many developers overlook the nuances of configuration.
Why This Matters
Java IDEs like IntelliJ IDEA, Eclipse, or NetBeans require specific configuration settings to be most effective. Poor configuration can lead to issues like slow performance, lack of syntax highlighting, or even failures to compile code.
Solution: Fine-Tuning IntelliJ IDEA
If you choose IntelliJ IDEA, here are a few configurations that can make a big difference:
-
Enable Compiler Options:
Navigate to
File -> Settings -> Build, Execution, Deployment -> Compiler
and enable "Use 'Build' instead of 'Make' on a single file". -
Set Up Code Style:
Go to
File -> Settings -> Editor -> Code Style -> Java
to adjust your formatting preferences. Consistent code style helps collaboration.
Shortcut Configuration
Additionally, familiarize yourself with the shortcut keys that can speed up your workflow dramatically.
4. Mismanaging Environment Variables
Environment variables can affect how your Java programs run. A common mistake is failing to set or misconfiguring key variables like JAVA_HOME
or PATH
.
Why This Matters
Environment variables tell your system where to look for installed Java versions and which libraries or executables to use. Misconfiguration can lead to compilation issues or runtime errors.
Example: Setting JAVA_HOME
You can set the JAVA_HOME
environment variable on a Unix-like system with:
export JAVA_HOME=/path/to/your/java
To make this change permanent, add it to your .bashrc
or .bash_profile
.
Verifying the Setup
To ensure your environment variables are correctly set, you can run:
echo $JAVA_HOME
which java
This will display the Java Home directory and the path of the java executable currently in use.
5. Neglecting Testing and Debugging Tools
Skipping proper testing and debugging setups is a mistake that can lead to hard-to-find errors. Unit testing frameworks such as JUnit and Mockito should be part of your development arsenal.
Why This Matters
Testing your code is essential for maintaining quality and performance. Java’s strong typing and static analysis features make it easier to catch errors early, but it's critical to set up your unit tests.
Example: Setting up JUnit
In Maven, you can add JUnit to your pom.xml
:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
Once added, you can create a unit test class:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class MyTest {
@Test
public void testAddition() {
assertEquals(2, 1 + 1);
}
}
Running this test ensures your addition logic functions correctly and rapidly alerts you to breaks in functionality.
Bringing It All Together
Setting up your Java development environment is crucial for productivity. By avoiding common pitfalls such as poor Java version management, neglecting build tools, misconfigured IDEs, mismanaging environment variables, and underestimating the importance of testing, you can create a setup that facilitates smooth development.
For more in-depth guidance on general setup issues, I encourage you to check out 5 Common Pitfalls When Setting Up Your Dev Machine. Embrace these best practices, and you'll be well on your way to a successful Java development experience.