Avoiding Java Development Setup Mistakes: Top Tips
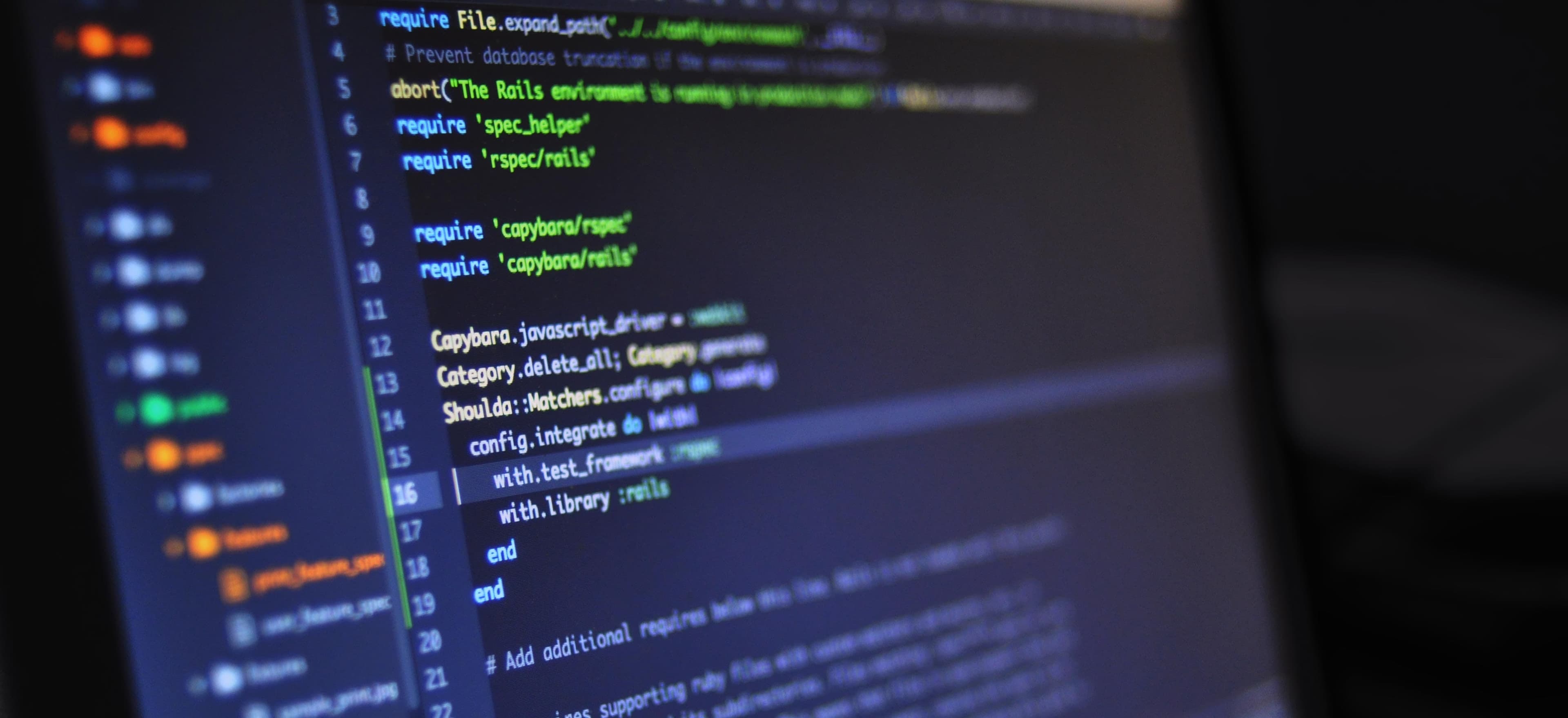
- Published on
Avoiding Java Development Setup Mistakes: Top Tips
Java is a powerful language widely used in enterprise applications, mobile development, and the Internet of Things (IoT). However, if you're just diving into Java development, you'll soon find that setting up your development environment can be a daunting task. Misconfigurations can lead to frustrating issues down the line, making the thrill of coding quickly dissipate into a sea of error messages.
In this blog post, we will discuss essential tips for a smooth Java setup, helping you avoid common pitfalls that can stall your learning curve and affect your productivity. Whether you're setting up your development environment for the first time or refining your process, this article has you covered.
Understanding Java Development Environment
Before we dive in, let’s clarify what a Java development environment entails. Typically, this involves the Java Development Kit (JDK), an Integrated Development Environment (IDE) of your choice, build tools like Maven or Gradle, and any necessary libraries or frameworks.
Tip 1: Choose the Right JDK Version
Java has multiple versions, but do you know which one to choose? As of October 2023, the most stable versions are Java 8 and Java 17, with 17 being the latest long-term support release. You may want to develop with the same version that your production environment uses to minimize compatibility issues.
# To check your Java version, run:
java -version
You can download the JDK from Oracle's website or consider OpenJDK for an open-source alternative.
Tip 2: IDE Selection and Configuration
An IDE can significantly enhance your coding experience by providing tools for debugging, code completion, and syntax highlighting. Popular options include Eclipse, IntelliJ IDEA, and NetBeans.
When setting up your IDE, consider the following:
- Install Plugins: Choose plugins that leapfrog your productivity. For example, Lombok can help ease boilerplate code in Java:
<!-- Adding Lombok in Maven -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>provided</scope>
</dependency>
- Customize Settings: Tailor features such as code formatting and autocomplete settings to match your workflow.
Tip 3: Include Version Control Early
It can be tempting to focus solely on code, but integrating version control (like Git) from the start cannot be overstated. A version control system offers substantial benefits:
- Backup: Avoid losing work through consistent commits.
- Collaboration: Share your project easily if you're working within a team.
- Branching: Experiment with features without the fear of breaking the main codebase.
Setup is straightforward:
# Initialize a new Git repository
git init
# Add files and commit
git add .
git commit -m "Initial commit"
For guidance on more advanced version control strategies, check the Git documentation.
Tip 4: Explore Build Automation Tools
Manual project compilation can quickly become tedious. Build tools like Maven and Gradle are vital for project management, dependency management, and automation of repetitive tasks.
Example with Maven:
- POM file setup:
<!-- pom.xml -->
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
</project>
- Invoke Builds:
Run the following commands to compile and package your code:
# Compile the project
mvn compile
# Package the project
mvn package
These tools take a good amount of manual effort out of the process and enable efficient management of dependencies.
Tip 5: Always Read Documentation
Documentation is your best friend. Each library, framework, and tool has its nuances, and taking the time to understand the documentation will save you hours of headaches. For instance, if you are employing Spring Boot, their official docs offer comprehensive guides for setup.
Tip 6: Set Up Your Classpath Correctly
Your classpath is crucial for Java to locate libraries and classes. Misconfiguration can lead to ClassNotFoundException
. Always verify your classpath settings in your IDE or your build tool configurations. Here’s how to check it:
# To print the current classpath, run:
echo $CLASSPATH
Tip 7: Be Wary of Environmental Issues
Sometimes, problems stem from conflicting environmental settings. Using environment variables correctly can alleviate many issues:
- JAVA_HOME: Ensure that it's pointing to your JDK installation.
- PATH: Include Java's bin directory for access to the
java
andjavac
commands.
# Set JAVA_HOME in a Unix-based system
export JAVA_HOME=/path/to/jdk
# Update PATH
export PATH=$JAVA_HOME/bin:$PATH
Tip 8: Utilize Containerization
Containerization with Docker can simplify your development environment setup, ensuring consistency in dependencies, libraries, and configurations:
- Dockerfile Example:
FROM openjdk:17-jdk-alpine
COPY . /app
WORKDIR /app
RUN ./mvnw package
CMD ["java", "-jar", "target/my-app.jar"]
- Build and Run:
# Build Docker image
docker build -t my-java-app .
# Run Docker container
docker run -p 8080:8080 my-java-app
This method provides a lightweight and isolated environment, preventing "it works on my machine" nightmares.
The Bottom Line
Setting up your Java development environment doesn’t have to be a herculean task. By understanding the various components and following the outlined tips, you can ensure a smooth and productive coding experience. Remember to revisit resources like the article on "5 Common Pitfalls When Setting Up Your Dev Machine" available at infinitejs.com/posts/common-dev-machine-pitfalls for deeper insights on avoiding common mistakes.
When you set up a strong foundation, you'll free up mental bandwidth for writing elegant, efficient code. Embrace the learning process, and before you know it, you'll be producing great Java applications. Happy coding!
Checkout our other articles