How Impure Functions Complicate Java Integration Tests
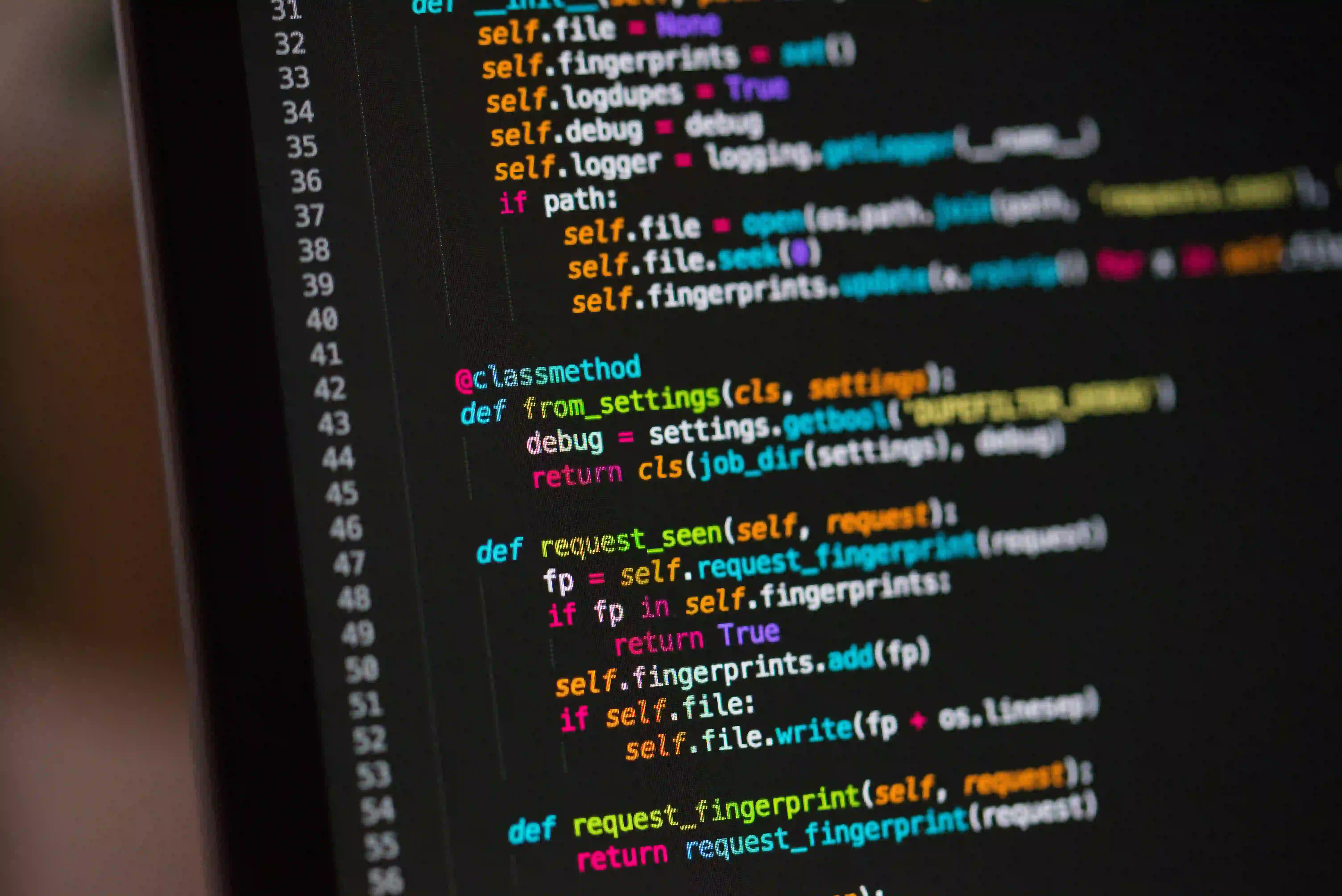
How Impure Functions Complicate Java Integration Tests
When we develop applications in Java, we typically strive for code that is both efficient and maintainable. One of the pivotal design philosophies behind writing robust code is ensuring that our functions are "pure." But, what exactly do we mean by pure functions? Why should we care, and how does this relate to integration testing in Java? In this post, we'll explore how impure functions can complicate Java integration tests and why maintaining purity in functions is crucial for healthy software architecture.
What are Pure and Impure Functions?
Pure Functions
A pure function is one where the output value is determined solely by its input values, without any observable side effects. This means that if you call a pure function with the same arguments multiple times, it will always return the same result. Here’s an example of a pure function:
public int add(int a, int b) {
return a + b;
}
Why is it pure? The function add
takes two integers as input and always returns their sum, with no side effects like modifying a global variable or interacting with I/O operations.
Impure Functions
In contrast, an impure function may produce different outputs given the same input or may introduce side effects. Consider the following function:
private int counter = 0;
public int incrementAndReturn() {
counter++;
return counter;
}
Why is it impure? Even if you call incrementAndReturn
with the same function state, it produces different output values because it modifies a global variable counter
.
The Challenges of Impure Functions in Integration Testing
1. Unpredictability
Integration tests are designed to evaluate how various modules of an application work together. When modules rely on impure functions, it can lead to unpredictable behaviors.
For instance:
public void processTransaction(double amount) {
double tax = calculateTax(amount);
updateDatabase(amount + tax);
}
If calculateTax
is an impure function that also logs information to a file or modifies global state, running processTransaction
multiple times may not yield consistent results. This unpredictability complicates testing significantly, as you cannot guarantee that the state of the application remains consistent between test runs.
2. Coupling and Complexity
Impure functions can create hidden dependencies within your code. When a function interacts with global state, such as databases or files, your tests may have to set up complex environments to ensure the correctness of each integration test.
For example:
public void saveUser(User user) {
// A side effect that writes to a database
database.insert(user);
sendWelcomeEmail(user.getEmail()); // Another side effect
}
In this case, testing saveUser
might require you to set up the database state precisely, including ensuring that emails don't flood an inbox. If you miss a setup step, the test could fail for reasons unrelated to the validity of the logic within saveUser
.
3. Difficulty in Mocking
To test integration points effectively, we often resort to mocking dependencies, especially when those dependencies have impure functions. With pure functions, mocking can be straightforward, as we expect predictable outputs.
Example of mocking a pure function:
@Test
public void testPureFunction() {
assertEquals(10, add(5, 5));
}
For impure functions, simulating their behavior while avoiding side effects becomes tedious. Consider this mocking scenario:
public class EmailService {
public void send() {
// Sends an email
}
}
If we want to test a function that depends on EmailService
, dealing with its actual email-sending behavior can lead to complications, essentially forcing you to create a dummy service for swift testing.
4. Side Effects in Tests
When testing functions with side effects, you need a way to reset state or validate the state after tests run. This requirement means that you have to enforce strict cleanup procedures in your tests.
For example:
@Test
public void testUserCreation() {
saveUser(new User("test@example.com"));
// Check if the user exists in the database
}
If saveUser
is impure and writes to the DB, you might need to ensure that you delete that user after the test runs, adding unnecessary complexity to your testing suite.
Best Practices: Strive for Purity
To alleviate some of these challenges in your Java applications, consider adopting practices that promote purity:
1. Favor Pure Functions
Whenever possible, structure your functions to be pure. This makes them easier to test and reason about. Aim to encapsulate side effects in a controlled manner.
2. Dependency Injection
Use dependency injection to manage dependencies. By doing so, you can easily swap out implementations during testing.
public class UserService {
private final EmailService emailService;
public UserService(EmailService emailService) {
this.emailService = emailService;
}
public void createUser(User user) {
// Creating user logic
emailService.sendWelcomeEmail(user.getEmail());
}
}
In this example, during testing, you could inject a mock EmailService
that simulates email sending without actually sending an email.
3. Use Mocks and Fakes Wisely
When dealing with impure functions, use mocks and fakes judiciously. Frameworks like Mockito can help simulate behavior without triggering actual side effects.
4. Test Side Effects Explicitly
If your function has side effects that actually need to be tested, ensure you have tests specifically targeting those side effects. Make it clear what state should exist after the test runs.
To Wrap Things Up
As demonstrated, impure functions can complicate Java integration tests considerably. They lead to unpredictability, increased complexity, and additional work in setting up a stable testing environment. By striving for purity in functions and adopting best practices like dependency injection and the controlled use of mocks, developers can create a more streamlined and predictable testing process.
Ultimately, the battle against impure functions is worth fighting—not only for the sake of higher quality tests but also for maintainable, robust code in the long term. For a deeper understanding of how functional purity plays a role in preventing bugs and errors, you may find the article "Why Impure Functions Can Lead to JavaScript Bugs" insightful (https://infinitejs.com/posts/why-impure-functions-lead-to-js-bugs).
Remember, developing with a mindset focused on purity can lead to cleaner, more maintainable, and testable code—an investment in the future of your software projects.