How Impure Functions Voyage Java to Unintended Bugs
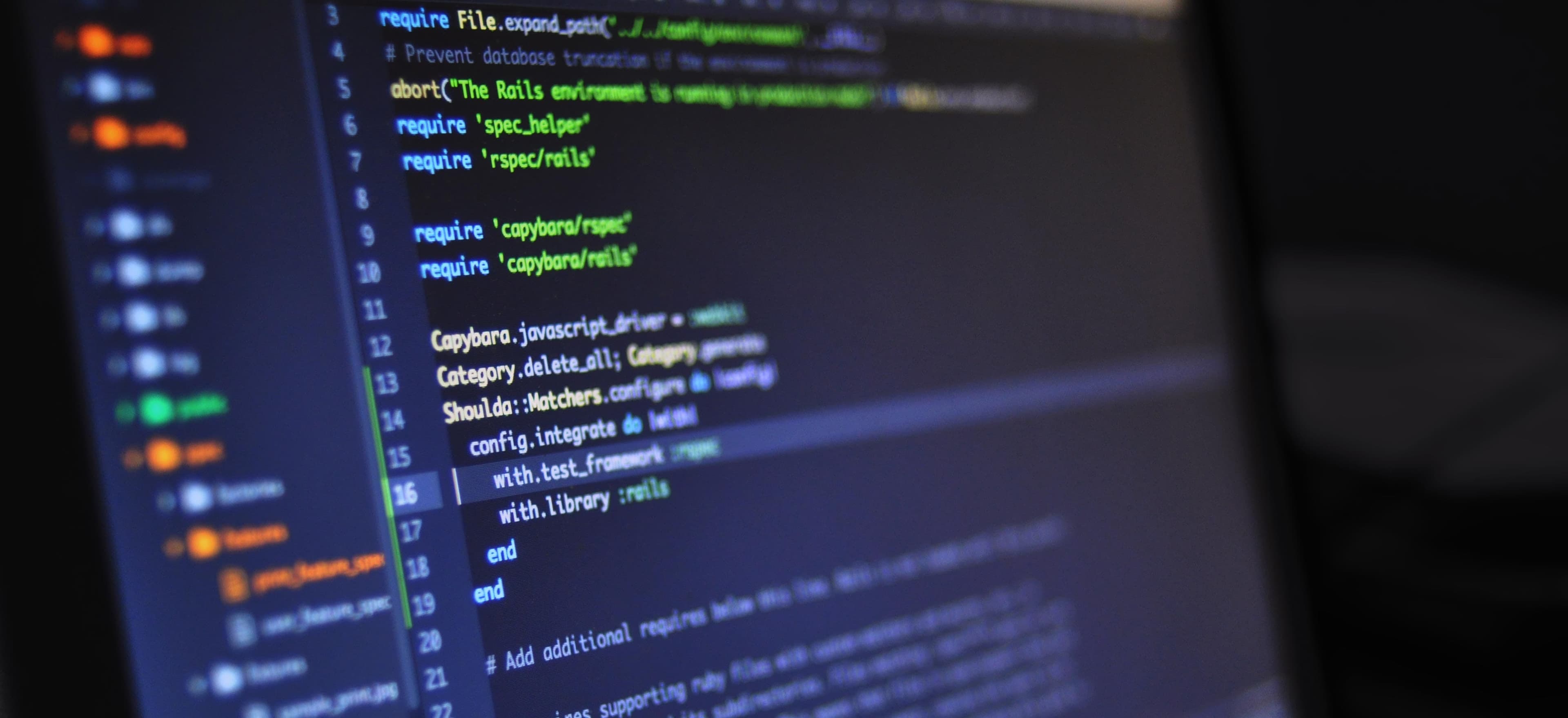
- Published on
How Impure Functions Voyage Java to Unintended Bugs
In programming, functions serve as building blocks for creating logic and behavior within software applications. However, not all functions uphold the same principles. In Java, the concept of impure functions can introduce complexities that lead to bugs and unexpected behaviors. This blog post delves into understanding impure functions, their implications in Java, and strategies for mitigating the risks they pose.
Understanding Pure vs. Impure Functions
Before we dive deeper, it's essential to clarify the difference between pure and impure functions.
-
Pure Functions: These are functions where the output value is determined only by its input values, with no side effects. This means invoking a pure function with the same arguments will always yield the same result.
public int add(int a, int b) { return a + b; }
In this example, no matter how many times you call
add(2, 3)
, it will produce the same result:5
. -
Impure Functions: Conversely, impure functions can produce different outputs even with the same inputs, or they can cause side effects, such as modifying external states or variables.
private int counter = 0; public int incrementCounter() { return ++counter; }
Here,
incrementCounter()
modifies thecounter
variable each time it is called, leading to output variability based on its state.
Understanding this fundamental differentiation is crucial, especially since impure functions can introduce unexpected behaviors and bugs into Java applications.
The Voyage of Impure Functions in Java
-
State Management Complications
Impure functions introduce complexity when managing state. State, in programming, refers to the stored information at a particular time. If different parts of our application manipulate the same state without careful coordination, it can lead to unforeseen issues.
For instance, consider a scenario where multiple threads modify shared state:
public class SharedResource { private int sharedCounter = 0; public void update() { sharedCounter++; } }
In a multithreaded environment, the above code can produce race conditions, leading to inconsistent results. One thread may update the counter just before another reads it, causing unexpected behavior or bugs.
Why is this a concern? Race conditions can happen very quickly and are often difficult to detect, making them a significant source of bugs in applications.
-
Testing and Debugging Difficulties
Impure functions are often harder to test and debug. When unit testing, developers rely on the predictability of functions.
public class User { private String name; public void setName(String name) { this.name = name; } public String getName() { // Side effect: logging the name to the console System.out.println("User's name is: " + name); return name; } }
In this example, invoking
getName()
will not only return the user’s name but also print it. This side effect complicates the testing process since your test outcomes will not solely depend on the return value but also on its interaction with the console.For effective testing, you ideally want functions like this:
public String getPureName() { return name; // Pure function with no side effects }
-
Reduced Code Reusability
Impure functions often lead to reduced code reusability. For example, if your function depends on external state, reusing that function in a different context, or different state can lead to unpredictable results.
Consider the following function that checks user privileges based on an external variable:
public boolean hasAccess(User user) { if (user.isAdmin()) { return true; } // Side effect: modifying global variable accessLogCounter++; return false; }
This function relies on the
user
object state and modifiesaccessLogCounter
, which can vary from one execution to another. A more reusable function would avoid these dependencies.
Strategies for Mitigating Impure Function Risks
Now that we understand the problems associated with impure functions, how can developers navigate these issues? Here are some strategies:
-
Adopt Functional Programming Principles
While Java is not a functional programming language, it supports several functional programming paradigms. Embrace writing pure functions as much as possible. Utilize Java’s support for lambdas and streams, which often encourage functional styles.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); List<Integer> squaredNumbers = numbers.stream() .map(n -> n * n) // Pure function .collect(Collectors.toList());
Here,
n * n
is a pure transformation, and the stream flows without side effects, contributing to clearer and more robust code. -
Isolate Side Effects
If you must use impure functions, isolate their side effects. For example, if your function logs information, consider separating the logging mechanism from business logic.
public void logUserName(User user) { System.out.println("User's name is: " + user.getName()); } public String getUserName(User user) { return user.getName(); // Pure function }
By decoupling these functions, you can test
getUserName
on its own without worrying about console output. -
Use Dependency Injection (DI)
Implement Dependency Injection to control how functions access external states. By passing dependencies through constructors, you can make your functions easier to test and manage.
public class UserService { private final Logger logger; public UserService(Logger logger) { this.logger = logger; } public String checkUserName(User user) { logger.log(user.getName()); // Logger is injected, controlling the side effect return user.getName(); } }
In this example,
UserService
doesn't handle logging directly; it relies on an injected logger. This makes testing easier since you can inject a mock logger during tests.
Final Considerations
In conclusion, while impure functions may be necessary at times, especially in complex applications, they can invite bugs and unintended behavior if not handled carefully. By understanding the differences between pure and impure functions and establishing sound coding practices, Java developers can create more maintainable, robust, and bug-free code.
As a point of reference, you may want to explore the article titled Why Impure Functions Can Lead to JavaScript Bugs for comparative insights into function purity across languages.
Remember, the voyage toward clean and maintainable code starts with embracing pure functions whenever possible. Happy coding!
Checkout our other articles