Why Native SQL in Hibernate Can Lead to Performance Issues
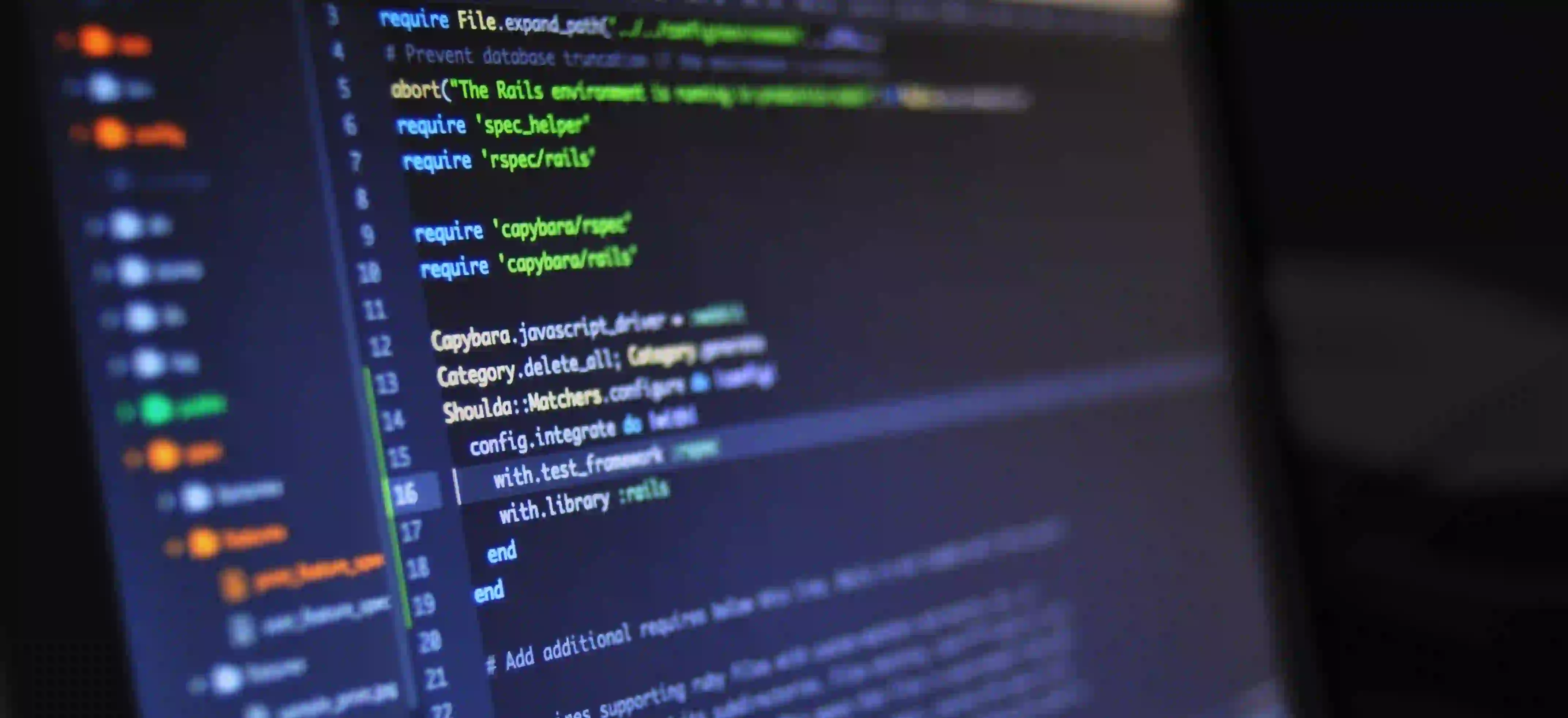
Why Native SQL in Hibernate Can Lead to Performance Issues
Hibernate has been a trusted ORM (Object-Relational Mapping) tool in Java applications for a long time. It simplifies database interactions by allowing developers to work with Java objects rather than SQL queries. However, one of the less touted features of Hibernate is its ability to execute native SQL queries directly to the database. While this capability can be tempting for specific use cases, it can also lead to performance issues if not handled carefully.
In this blog post, we will explore why using native SQL in Hibernate can compromise application performance, discussing problems like lack of abstraction, caching issues, and increased database load. We'll also provide code snippets to illustrate our points.
Understanding Hibernate and Its Architecture
Before delving into the nuances of native SQL in Hibernate, it’s essential to understand what Hibernate is and how it operates. At its core, Hibernate maps Java classes to database tables, establishing relationships and handling transactions seamlessly.
The Hibernate ORM Layers
- Session Layer: Responsible for managing the connection to the database and performing CRUD operations.
- Transaction Layer: Handles database transaction management.
- Query Layer: Supports HQL (Hibernate Query Language), Criteria API, and native SQL queries.
The beauty of Hibernate lies in this architecture, which emphasizes abstraction. When we neglect this abstraction layer by opting for native SQL, we undermine some of Hibernate's primary advantages.
Performance Issues with Native SQL
1. Lack of Optimizations
Using native SQL means you step away from HQL and the Criteria API, which come equipped with various optimization mechanisms. Hibernate’s abstraction layer automatically optimizes queries based on the underlying database and its schema.
Example of Native SQL Query:
Query query = session.createNativeQuery("SELECT * FROM users WHERE age > :age");
query.setParameter("age", 30);
List<User> users = query.getResultList();
In this example, while the query directly retrieves user data, we are missing out on HQL's potential optimizations. The Hibernate optimization engine can rewrite queries internally to improve performance, which is lost once you resort to native SQL.
2. Caching Limitations
Hibernate implements first-level and second-level caching mechanisms that significantly boost performance by reducing database load. When native SQL is employed, these caching layers are sometimes bypassed or less effective.
Consider this scenario:
User userFromDb = session.createNativeQuery("SELECT * FROM users WHERE id = :id", User.class)
.setParameter("id", 1)
.getSingleResult();
Here, if the same query is executed repeatedly, Hibernate’s first-level cache won’t leverage the previously fetched data effectively because it often associates entities based on their identifiers, not on their raw result set.
3. Increased Complexity
Native SQL queries can introduce more complex maintenance demands because they decouple queries from the Java objects they affect. For example, if you change the structure of the User
class, you must also modify every place where native SQL queries interact with this schema.
Example:
Query nativeQuery = session.createNativeQuery("SELECT username, email FROM users WHERE status = :status")
.setParameter("status", "active");
List<Object[]> result = nativeQuery.getResultList();
In this case, the result is a List
of Object[]
, representing a raw result. This makes it harder to refactor, understand, and maintain than HQL, which cleanly maps results back to the entities.
4. Manual Handling of Joins and Relationships
Hibernate excels at managing relationships (one-to-many, many-to-many, etc.) through its powerful fetching strategies. When using native SQL, developers must manually handle joins and relationships, increasing the likelihood of bugs.
For instance, the following native SQL query for a join may look simple:
String sql = "SELECT u.username, p.name FROM users u JOIN posts p ON u.id = p.user_id WHERE u.age > :age";
You have to ensure that you handle the results properly and map them back to their corresponding Java entities—a process that introduces room for human error.
5. Potential for SQL Injection
While Hibernate abstracts away many risks associated with SQL, native SQL shifts responsibility back to the developer. If you are not careful with user inputs, you could potentially open your application to SQL injection attacks.
Best practice:
String unsafeUserInput = request.getParameter("username");
Query query = session.createNativeQuery("SELECT * FROM users WHERE username = '" + unsafeUserInput + "'");
The above naive implementation poses a high risk. Always use parameterized queries to mitigate this risk.
Closing the Chapter
The temptation to use native SQL in Hibernate should be approached with caution. Although it offers a straightforward means to run queries, the downsides—including lack of optimizations, caching limitations, increased complexity, and maintenance overhead—can significantly impact performance.
Recommendations
- Leverage HQL or the Criteria API wherever possible.
- If native SQL is necessary, ensure queries are optimized and cached effectively.
- Always sanitize user inputs to prevent SQL injection vulnerabilities.
- Regularly review and refactor any native SQL queries to align them with updates in your Java entities.
By doing this, you can maintain the performance advantages that Hibernate offers, ensuring that your application runs efficiently.
For more insights on best practices in Hibernate, consider checking the official Hibernate documentation or dive deeper into ORM strategies for a well-rounded understanding.
By taking a comprehensive view of how native SQL operates within Hibernate, you position yourself to make informed decisions that benefit both your application's performance and maintainability. Remember that the key lies in balancing performance needs with effective use of the tools available to you.