Struggling with IoT Device Connectivity on Android Things?
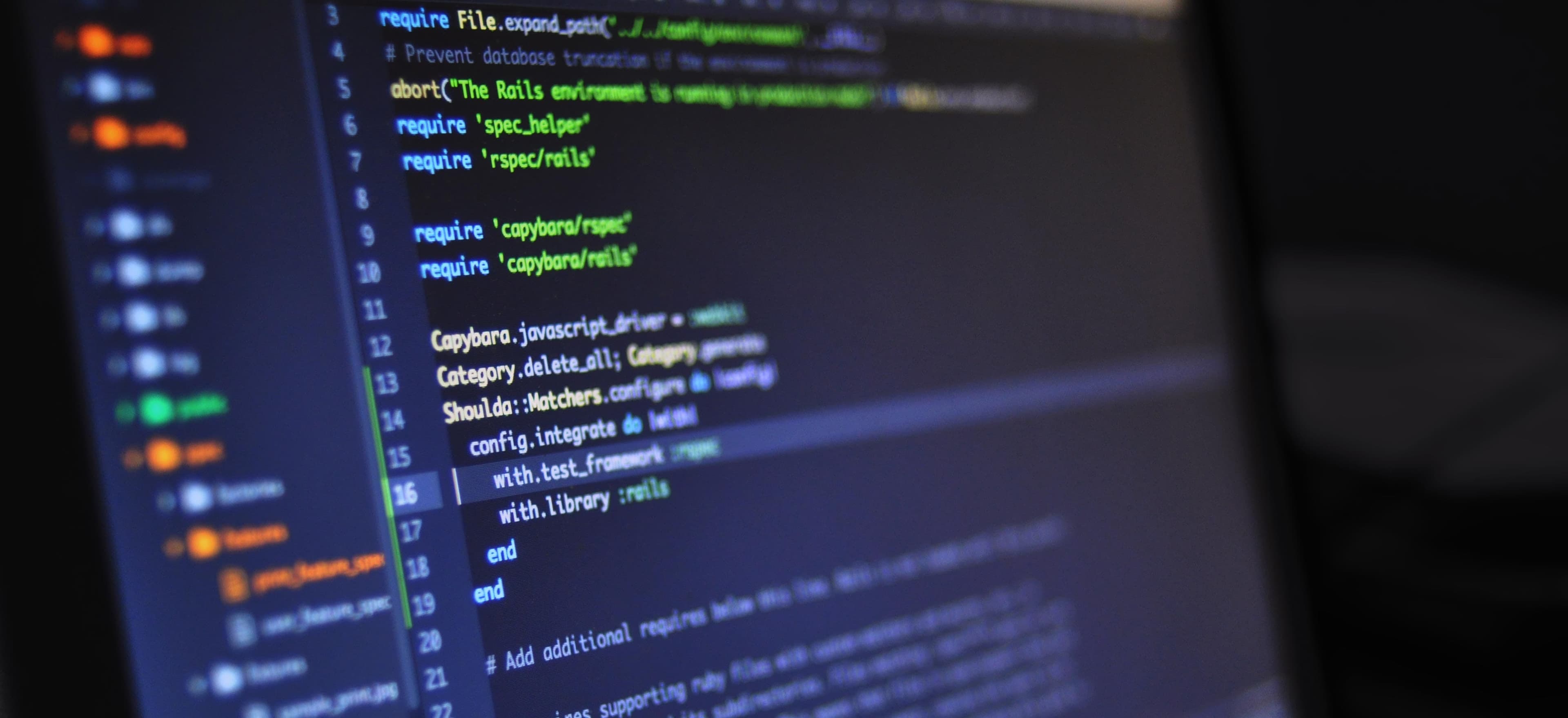
- Published on
Struggling with IoT Device Connectivity on Android Things?
As the Internet of Things (IoT) continues to grow, developers are tasked with the challenge of connecting a myriad of devices to the internet. Android Things, Google's platform designed to help developers create IoT solutions, is a powerful tool for this purpose. However, as many have experienced, working with IoT device connectivity can be challenging. In this blog post, we'll dive into key concepts, provide helpful code snippets, and give insights into best practices to ensure smoother connectivity with Android Things.
Understanding Android Things
Android Things is a version of the Android operating system designed specifically for embedded devices. It allows developers to leverage the Android ecosystem while building connected devices. While Android Things has been deprecated and received a limited update, many foundational concepts remain applicable to IoT solutions built on platforms like Raspberry Pi or other system-on-modules.
What Should You Know About IoT Device Connectivity?
-
Network Protocols: Understanding protocols such as HTTP, MQTT, and WebSocket is essential. These protocols define how devices communicate over the network.
-
Device Management: Managing how devices connect and disconnect from networks can be challenging. Ensuring a stable connection is crucial for IoT applications.
-
Security: With wireless connectivity comes potential vulnerabilities. Knowing how to secure IoT devices against unauthorized access is paramount.
Setting Up Connectivity
To establish a connection for an IoT device with Android Things, there are several general steps that need to be followed.
Step 1: Configuring Your Network
First, you want to ensure that your device can connect to the internet. This setup typically involves configuring your Wi-Fi connection. Here is how you can connect to Wi-Fi in an Android Things application:
import android.content.Context;
import android.net.wifi.WifiConfiguration;
import android.net.wifi.WifiManager;
public void connectToWifi(Context context, String ssid, String password) {
WifiManager wifiManager = (WifiManager) context.getApplicationContext().getSystemService(Context.WIFI_SERVICE);
WifiConfiguration wifiConfig = new WifiConfiguration();
wifiConfig.SSID = String.format("\"%s\"", ssid);
wifiConfig.preSharedKey = String.format("\"%s\"", password);
int netId = wifiManager.addNetwork(wifiConfig);
wifiManager.disconnect();
wifiManager.enableNetwork(netId, true);
wifiManager.reconnect();
}
Why This Code Matters: This function allows your IoT device to establish a Wi-Fi connection. With Wi-Fi being a primary IoT communication method, a robust connection setup is crucial.
Step 2: Choosing the Right Protocol for Communication
Choosing the right communication protocol is vital for the functionality and efficiency of your IoT system. For example, if you intend to send small amounts of data frequently from a sensor, MQTT (Message Queuing Telemetry Transport) is a favorable option.
Example of MQTT Implementation:
For MQTT, you'll want to use a library such as Eclipse Paho. Here's a basic structure to connect and publish data:
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
public void publishData(String broker, String topic, String payload) {
try {
MqttClient client = new MqttClient(broker, MqttClient.generateClientId());
client.connect();
MqttMessage message = new MqttMessage(payload.getBytes());
message.setQos(2); // Ensure message is delivered
client.publish(topic, message);
client.disconnect();
} catch (MqttException e) {
e.printStackTrace();
}
}
Why Use MQTT: MQTT is lightweight and designed for limited bandwidth. Its publish/subscribe model is perfect for IoT devices that communicate in real-time.
Step 3: Implementing Security Measures
Securing your data is crucial. For IoT devices, using SSL/TLS is advisable to encrypt the data being transmitted. Here's how you can set up secure MQTT connections:
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
public MqttConnectOptions createSecureOptions() {
MqttConnectOptions options = new MqttConnectOptions();
options.setCleanSession(true);
options.setConnectionTimeout(10);
options.setKeepAlive(20);
options.setUserName("username");
options.setPassword("password".toCharArray());
options.setHttpsURLConnectionFactory(new DefaultSSLSocketFactory()); // Example of SSL Socket Factory
return options;
}
The Importance of Security: Cybersecurity threats can lead to unauthorized access and data breaches. Implementing robust security measures is essential for protecting user data and maintaining trust.
Troubleshooting Connectivity Issues
Despite thorough configurations, connectivity may still falter. Here are some common issues and troubleshooting tips:
- Weak Wi-Fi Signal: Check signal strength and reposition your device if necessary.
- Misconfigured Network: Ensure you've entered the correct SSID and password.
- Firewall and Router Settings: Verify that your router is allowing communication on the required ports.
- Device Limitations: Some devices may not support all networking features. Refer to your device specifications for limitations.
Helpful Links for Additional Context
- MQTT Essentials - Learn more about MQTT and its advantages for IoT solutions.
- Android Things Documentation - Explore the official resources for Android Things, which can provide insights into device management.
The Last Word
IoT device connectivity on Android Things may be accompanied by a host of challenges—from network configurations to protocol selection and security measures. By understanding the fundamentals of connectivity, implementing the right tools, and troubleshooting effectively, developers can create robust IoT solutions.
As you continue your journey in IoT development, remember to experiment with various protocols and techniques. The world of connected devices is constantly evolving, and staying informed will ensure that your applications not only connect but thrive in a competitive landscape.
Checkout our other articles