Fixing Connection Issues Between Tomcat and Oracle DB in Docker
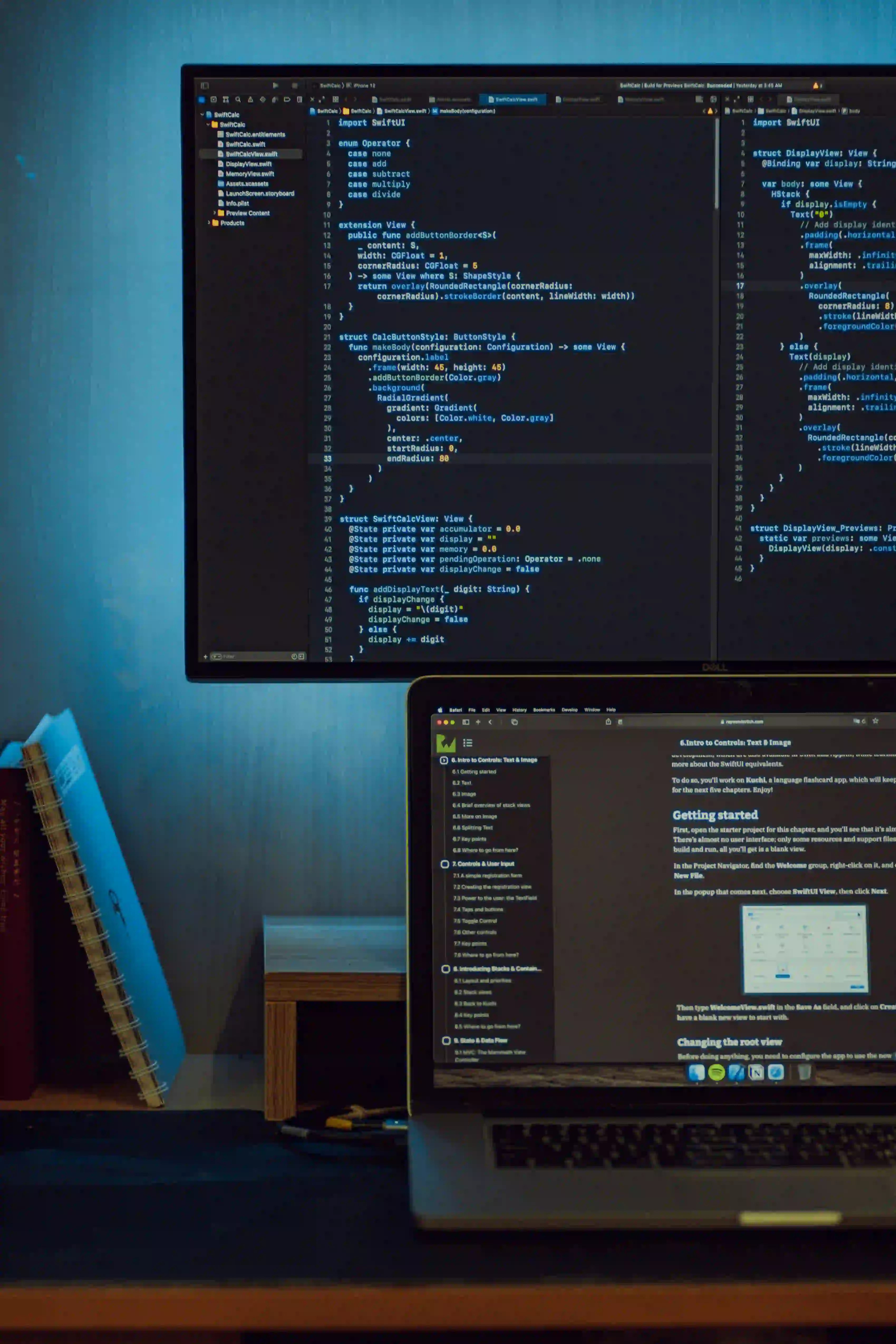
Fixing Connection Issues Between Tomcat and Oracle DB in Docker
Connecting a Tomcat server to an Oracle Database in a Dockerized environment can be challenging, especially when configurations or dependencies don't align. Whether you're developing a new application or migrating an existing one, encountering connection issues can disrupt your workflow. In this post, we will walk through common connection issues and how to resolve them effectively.
Understanding the Environment
Before diving into solutions, it’s critical to understand the components involved:
- Apache Tomcat: A widely-used application server for running Java Servlets and JSPs.
- Oracle Database: A powerful relational database management system that is widely adopted in enterprise environments.
- Docker: A platform that uses containerization to ensure your applications run reliably irrespective of the environment.
Getting these components to communicate effectively can be intricate. Let’s explore how you can troubleshoot and fix connection issues.
Common Setup for Tomcat and Oracle DB in Docker
Dockerfile Example
Let’s start with a basic Dockerfile for Tomcat:
FROM tomcat:9.0
COPY ./your-app.war /usr/local/tomcat/webapps/
COPY ./context.xml /usr/local/tomcat/conf/
Context Configuration for Oracle DB
In the context.xml
, you should configure the datasource for Oracle. Here’s how it typically looks:
<Context>
<Resource name="jdbc/OracleDB" auth="Container"
type="javax.sql.DataSource"
maxTotal="20" maxIdle="10" maxWaitMillis="-1"
username="your_username" password="your_password"
driverClassName="oracle.jdbc.driver.OracleDriver"
url="jdbc:oracle:thin:@//hostname:port/service_name"/>
</Context>
Ensure you've replaced your_username
, your_password
, hostname
, port
, and service_name
with actual values relevant to your Oracle database.
Adding JDBC Driver
Tomcat needs the Oracle JDBC driver to facilitate the connection. You can include it by adding the JAR file to the lib
directory of Tomcat:
COPY ./ojdbc8.jar /usr/local/tomcat/lib/
Connection Issues and Their Resolutions
1. Driver Class Not Found
One of the most common issues is the "Driver Class Not Found" error. This happens when Tomcat cannot find the JDBC driver.
Solution: Include the JDBC driver correctly
- Ensure the JAR file is in the
lib
directory. - Restart the Tomcat server after modifying the configuration.
2. Database URL Misconfiguration
Another frequent issue is an incorrect database URL format which may result in SQLExceptions.
Solution: Verify Connection URL
A correct connection URL should resemble:
jdbc:oracle:thin:@//your_db_host:port/service_name
Make sure the host
, port
, and service_name
are accurate and reachable from the Docker container.
3. Network Issues
When running in Docker, containers need to communicate over networks. You may encounter connection refused or timeout errors due to networking issues.
Solution: Network Configuration
Ensure you have created an appropriate Docker network and both Tomcat and Oracle containers are part of the same network. You can create a network using:
docker network create my_network
When running your containers, make sure to connect them as follows:
docker run --network=my_network --name=my_tomcat tomcat:9.0
docker run --network=my_network --name=my_oracle oracle/database:latest
4. Firewall and Security Groups
Firewalls and security groups can prevent communication between Tomcat and Oracle DB. Ensure that the relevant ports are open.
Solution: Access Control Lists
If using cloud providers or on-premise servers, check that your firewall configuration allows traffic on the database port.
5. Resource Limits in Docker
Sometimes, limits can be imposed on resources which can lead to issues in handling multiple requests.
Solution: Review Resource Allocation
You can specify resource limits in your Docker Compose file, for example:
services:
tomcat:
image: tomcat:9.0
deploy:
resources:
limits:
cpus: '0.5'
memory: 512M
Connecting Using a Simple Java Application
For further insight into how to establish a connection using Java, this simple application showcases the process.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class OracleConnectionExample {
public static void main(String[] args) {
String url = "jdbc:oracle:thin:@//host:port/service_name";
String username = "your_username";
String password = "your_password";
try (Connection conn = DriverManager.getConnection(url, username, password)) {
System.out.println("Connected to the database successfully!");
} catch (SQLException e) {
System.out.println("Connection failed");
e.printStackTrace();
}
}
}
Commentary on Code
- URL, Username, and Password: These must match the values used in your context.xml.
- DriverManager: It is used to manage the connection, ensuring that commands are executed in the right context.
- Connection Handling: The use of try-with-resources ensures that the connection is closed properly, avoiding potential memory leaks.
Testing the Connection
It’s important to test the connection rigorously. You can do this by accessing the Tomcat Manager and checking for any reported errors in the logs.
Troubleshooting with Logs
If issues persist, consult the catalina.out
log in the Tomcat container:
docker logs my_tomcat
Look for stack traces related to connection issues.
Final Thoughts
In summary, troubleshooting connection issues between Tomcat and Oracle DB in a Docker environment requires careful attention to configuration, networking, and resource management. By ensuring that your JDBC driver is included, your connection URL is correctly formatted, and your containers are networked properly, you can build a robust environment for your Java applications.
For further reading, consider checking the Oracle JDBC Documentation and the official Apache Tomcat Documentation.
By following the steps in this guide, you should be equipped to successfully connect your Tomcat instance to an Oracle database while avoiding common pitfalls. Happy coding!