Overcoming JDK 8 Javadoc Limitations for Method Listings
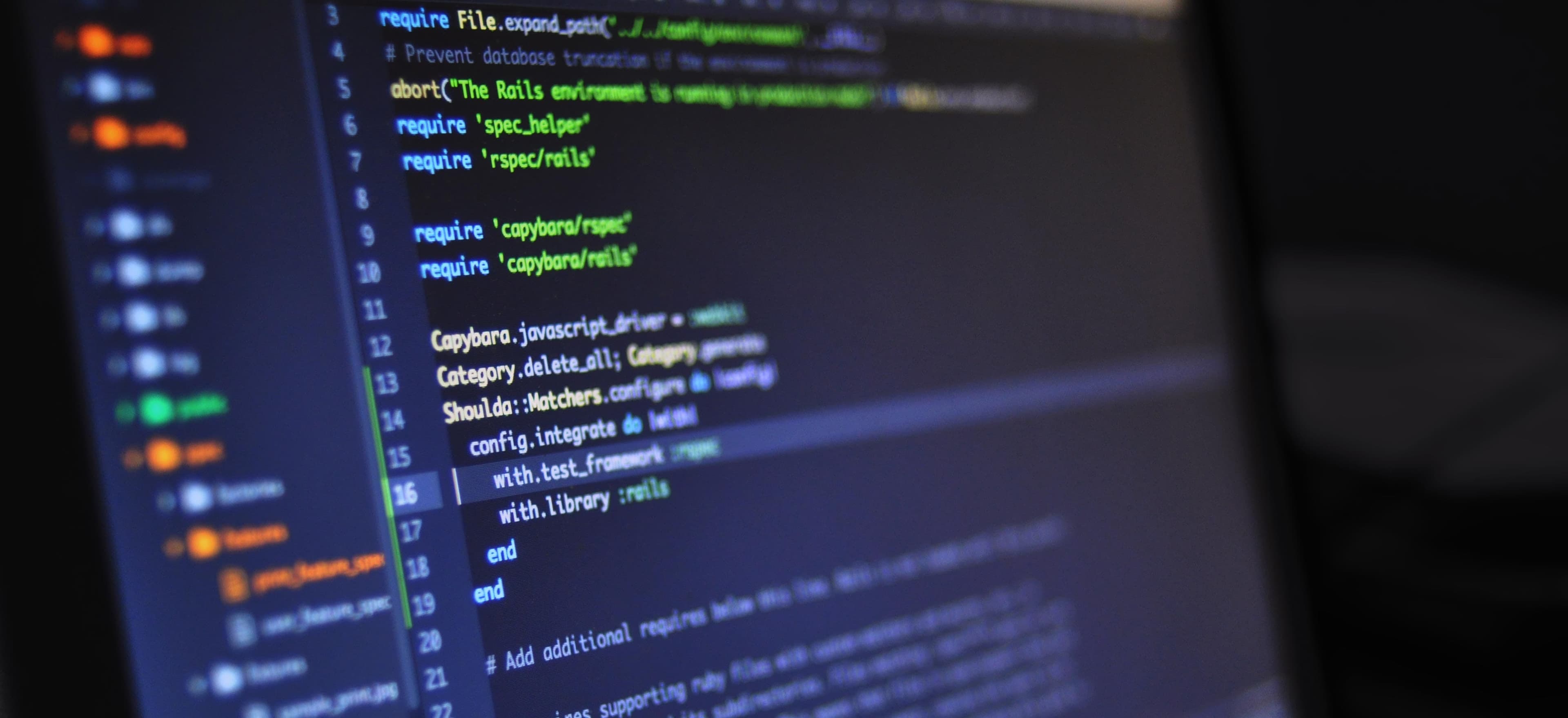
- Published on
Overcoming JDK 8 Javadoc Limitations for Method Listings
Java documentation is an integral part of software development, allowing developers to understand and utilize APIs effectively. While JDK 8 did a lot to enhance the documentation process, it also came with its limitations. One of the most notable issues is the representation of method listings in Javadocs. In this blog post, we will explore strategies for overcoming these limitations and ensuring your method listings are clear, accessible, and informative.
Understanding the Importance of Javadocs
Javadocs are HTML-based documents generated from Java source code. They provide a way for developers to understand classes, interfaces, methods, and fields. Good documentation is crucial for maintaining clear communication within development teams, especially in larger projects.
Method listings, in particular, serve as an essential guide for users to navigate through the functionality of classes. However, JDK 8 has restrictions that can make these listings less user-friendly.
Common Javadoc Limitations in JDK 8
Before diving into the solutions, it’s essential to understand the limitations of JDK 8 Javadocs. Here are a few:
-
Redundancy: JDK 8 does not effectively reuse method descriptions, leading to redundancy if multiple overloaded methods exist.
-
Lack of Hyperlinks: Some Javadocs do not automatically hyperlink parameters, return types, or exceptions thrown by methods, which hampers navigation.
-
Formatting Issues: Default formatting may not always provide the clarity that developers need, leaving room for confusion.
To illustrate these limitations, let’s consider an example below.
Example
/**
* Calculates the area of a rectangle.
*
* @param length the length of the rectangle
* @param width the width of the rectangle
* @return the area of the rectangle
*/
public double calculateArea(double length, double width) {
return length * width;
}
In the above code snippet, JDK 8 generates a straightforward documentation entry. However, it lacks contextual links to the parameters and return type, which leads to potential misunderstandings when reviewing method functionality.
Strategies for Overcoming Javadoc Limitations
There are several strategies you can implement to enhance your method listings in Javadocs. Let us outline them clearly:
1. Use @see
, @link
, and @linkplain
Utilizing these Javadoc tags offers richer connections to related classes and methods. You can improve the clarity of your documentation by providing direct links to other relevant resources.
Example:
/**
*
* @see Rectangle
* @link #calculatePerimeter(double, double)
*
* @param length the length of the rectangle
* @param width the width of the rectangle
* @return the area of the rectangle
*/
public double calculateArea(double length, double width) {
return length * width;
}
In this modified example, we introduce links to the Rectangle
class and another method, calculatePerimeter
. This empowers users with direct navigation and contextual understanding.
2. Implement Standardized Descriptions
For overloaded methods, create a standard description and leverage it across similar method signatures. This reduces redundancy.
Example:
/**
* Calculates the area of a rectangle.
*
* <p>
* This method is overloaded. Use either {@link #calculateArea(double, double)}
* for its area of the rectangle or {@link #calculateArea(Rectangle)}
* for the area derived from an object.
* </p>
*
* @param length the length of the rectangle
* @param width the width of the rectangle
* @return the area of the rectangle
*/
public double calculateArea(double length, double width) {
return length * width;
}
/**
* Calculates the area of a rectangle given a Rectangle object.
*
* @param rectangle the Rectangle object
* @return the area of the rectangle
*/
public double calculateArea(Rectangle rectangle) {
return rectangle.getLength() * rectangle.getWidth();
}
In this case, we show how to use a single description for multiple overloaded methods. This technique keeps the Javadocs less cluttered and more succinct.
3. Use HTML for Custom Formatting
Sometimes, using basic HTML can enhance readability. You can format your descriptions better and create lists or tables if needed.
Example:
/**
* Calculates the area of a rectangle.
*
* <p>
* The area can be calculated using two different methods:
* </p>
* <ul>
* <li>{@link #calculateArea(double, double)} - Pass the length and width directly.</li>
* <li>{@link #calculateArea(Rectangle)} - Pass a Rectangle object.</li>
* </ul>
*
* @param length the length of the rectangle
* @param width the width of the rectangle
* @return the area of the rectangle
*/
public double calculateArea(double length, double width) {
return length * width;
}
By implementing lists, we add clarity and make it easier for users to grasp which method to use.
4. Documentation Conventions
Adopting JQuery conventions can improve Javadocs. You might take inspiration from the Google Java Style Guide or Oracle's Java Documentation Guidelines.
These guidelines can provide valuable insights into improving your coding conventions and consequently, your Javadocs.
5. Comment Before Code
Place your Javadoc comments directly before the method declaration. This practice ensures that users see the documentation first when approaching the code.
Example:
/**
* Calculates the area of a rectangle.
* This example demonstrates the absolute simplicity of the method.
*
* @param length the length of the rectangle
* @param width the width of the rectangle
* @return the area of the rectangle
*/
public double calculateArea(double length, double width) {
return length * width;
}
Clear, direct documentation, placed judiciously, enhances usability immensely.
In Conclusion, Here is What Matters
Overcoming JDK 8 Javadoc limitations may seem daunting initially. However, by adopting clearer writing practices, utilizing links effectively, reducing redundancy, and following established documentation conventions, developers can significantly improve their method listings' quality and clarity.
Taking the time to refine your Javadocs will foster better understanding, reduce confusion, and ultimately lead to more reliable and maintainable code. Remember, well-documented code is just as essential as well-written code.
By mastering Javadoc, not only are you improving your own work, but you are contributing to an ecosystem where collaboration thrives. Happy coding!
Checkout our other articles