Troubleshooting Event Loss in Spring Boot Kafka Microservices
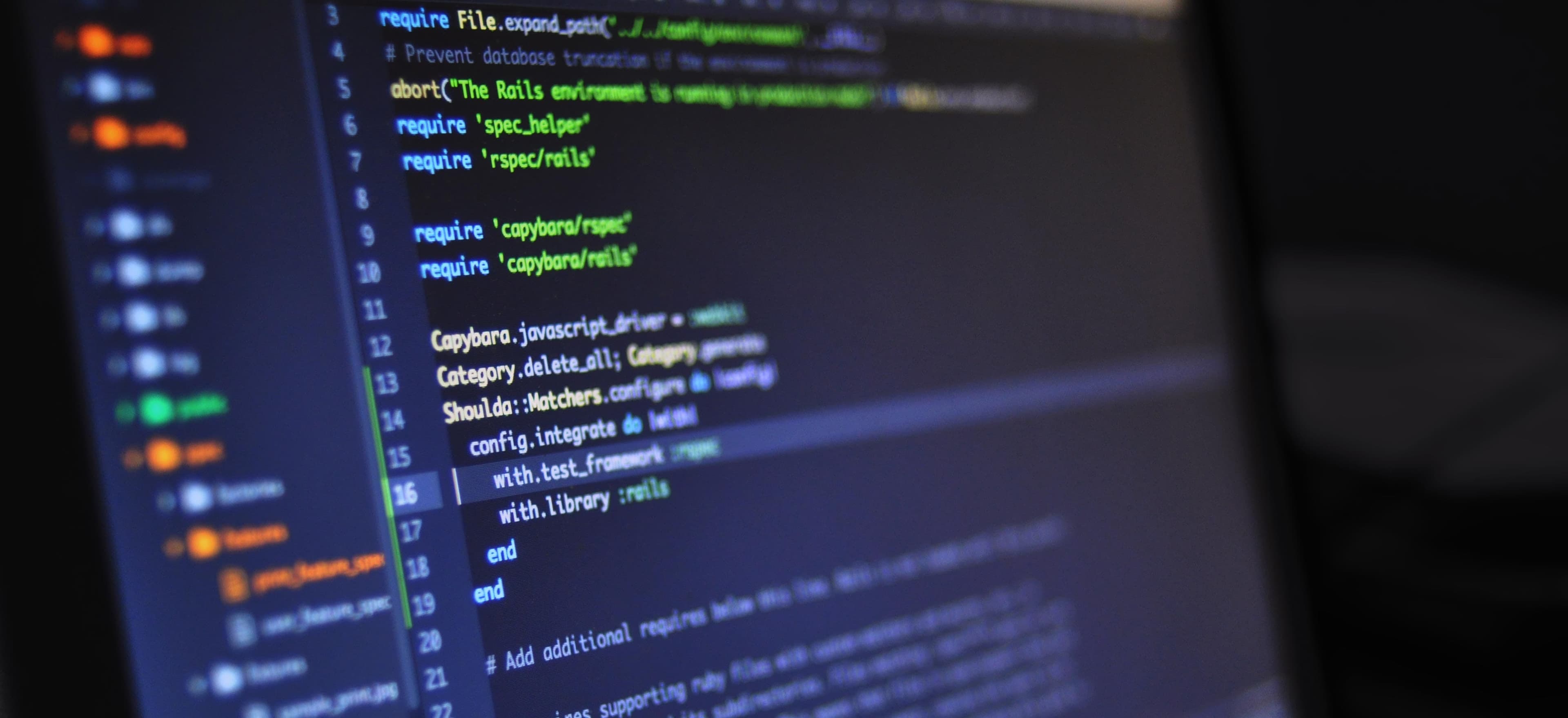
- Published on
Troubleshooting Event Loss in Spring Boot Kafka Microservices
In the world of microservices, Kafka has emerged as one of the most popular messaging systems due to its fault tolerance, scalability, and high throughput. However, even with its numerous advantages, event loss can occur, leading to significant issues for applications relying on real-time data processing. In this post, we will explore the causes of event loss in Spring Boot applications using Kafka, along with effective troubleshooting strategies.
Understanding Kafka Fundamentals
Before diving into troubleshooting, it is crucial to understand how Kafka operates under the hood. Kafka is a distributed messaging system that maintains the order of messages in a topic partition. Each message is assigned a unique offset, which is how it tracks the position of the messages in a partition.
Key Components
- Producer: Sends data to Kafka topics.
- Consumer: Reads data from Kafka topics.
- Broker: A Kafka server that stores messages.
The architecture allows for horizontal scaling, as consumers can be added to read messages concurrently across multiple partitions.
Common Causes of Event Loss
1. Misconfigured Acknowledgments
Spring Kafka allows you to configure how consumers acknowledge receipt of messages. If not configured correctly, it may result in the loss of messages.
- Auto-acknowledgment: If set to true, a consumer acknowledges the message automatically after it has been received, but before it has been processed. This can result in data loss if the application crashes before processing.
@KafkaListener(topics = "my_topic", groupId = "my_group", containerFactory = "kafkaListenerContainerFactory")
public void listen(String message) {
// Process message
}
To prevent loss, consider using manual acknowledgment. Here's how:
@KafkaListener(topics = "my_topic", groupId = "my_group")
public void listen(String message, Acknowledgment acknowledgment) {
try {
// Process message
acknowledgment.acknowledge(); // Acknowledge only after successful processing
} catch (Exception e) {
// Handle failure
}
}
2. Consumer Group Management
When multiple consumers share a consumer group, Kafka balances the load across them. If a consumer fails, the assigned partitions will be reassigned. Depending on the offset reset policy, this can result in message loss.
- Configuration: Setting the
auto.offset.reset
property to latest or earliest affects how messages are read if there are no committed offsets.
spring:
kafka:
consumer:
auto-offset-reset: earliest
Using earliest ensures that when a new consumer joins, it reads all available messages. Conversely, latest will skip uncommitted messages.
3. Message Serialization/Deserialization Issues
Messaging systems rely heavily on serialization and deserialization (SerDes) to transmit data. If there's a mismatch or error during this process, messages can be dropped.
- Example SerDes implementation:
public class MyMessage {
private String data;
// Getters and setters
}
// Custom Serializer
public class MyMessageSerializer implements Serializer<MyMessage> {
@Override
public byte[] serialize(String topic, MyMessage data) {
return data.getData().getBytes(StandardCharsets.UTF_8);
}
}
// Custom Deserializer
public class MyMessageDeserializer implements Deserializer<MyMessage> {
@Override
public MyMessage deserialize(String topic, byte[] data) {
return new MyMessage(new String(data, StandardCharsets.UTF_8));
}
}
Ensure that the producer and consumer use the same SerDes to prevent data loss due to serialization issues.
4. Deserialization Exceptions
When a message cannot be deserialized, Kafka will drop it and log an error. To mitigate this, implement error handling in your consumer.
@KafkaListener(topics = "my_topic", errorHandler = "myErrorHandler")
public void listen(MyMessage message) {
// Handle message processing
}
@Bean
public KafkaListenerErrorHandler myErrorHandler() {
return (m, e) -> {
// Log the error
};
}
5. Network Issues
Intermittent network failures between the producer/consumer and Kafka brokers can lead to dropped messages. Implementing retries or configuring timeout settings can often mitigate these issues.
spring:
kafka:
producer:
retries: 3 # Number of retry attempts
properties:
bootstrap.servers: localhost:9092
6. Unclean Leader Election
In scenarios where a Kafka broker goes down, a new leader is elected for the affected partitions. If the unclean.leader.election.enable
is set to true, a replica that does not have the latest data can become the leader, leading to potential message loss.
In your server.properties
, ensure:
unclean.leader.election.enable=false
7. Disk Issues or Log Cleanup
Finally, if Kafka brokers face disk space issues, the log retention policy can lead to deleting messages. It’s vital to monitor disk space and configure retention policies appropriately.
Best Practices to Prevent Event Loss
-
Use Transactional Producers: Utilize Kafka’s transactional capabilities to ensure that messages are sent or not at all.
kafkaTemplate.executeInTransaction(operations -> { // send messages return true; // Indicate success });
-
Monitor Offsets: Keep an eye on committed offsets and ensure they are being updated correctly without backlog.
-
Implement Retry Logic: Employ retry logic for both producer and consumer sides to account for possible failures.
-
Testing: Test the application under various failure scenarios to ensure that your implementation can handle such occurrences.
-
Read from the Right Partition: Always ensure that your consumers are correctly obtaining messages from the intended partitions to avoid processing errors.
To Wrap Things Up
In conclusion, while Kafka provides a robust framework for handling messaging in microservices, event loss can occur due to various reasons. By being aware of potential pitfalls, from configuration oversights to network issues, developers can implement strategies to prevent event loss. When combined with best practices like transactional producers and thorough testing, your Spring Boot Kafka microservices can achieve a high level of reliability.
For further reading, here are some relevant resources:
- Spring Kafka Documentation
- Kafka's Official Documentation
- Handling Events in Kafka
By adhering to the information shared in this post, you can troubleshoot and mitigate events loss effectively, ensuring your applications maintain a seamless and efficient data flow.
Happy coding!