Why Ignoring Java Coding Conventions Can Ruin Your Project
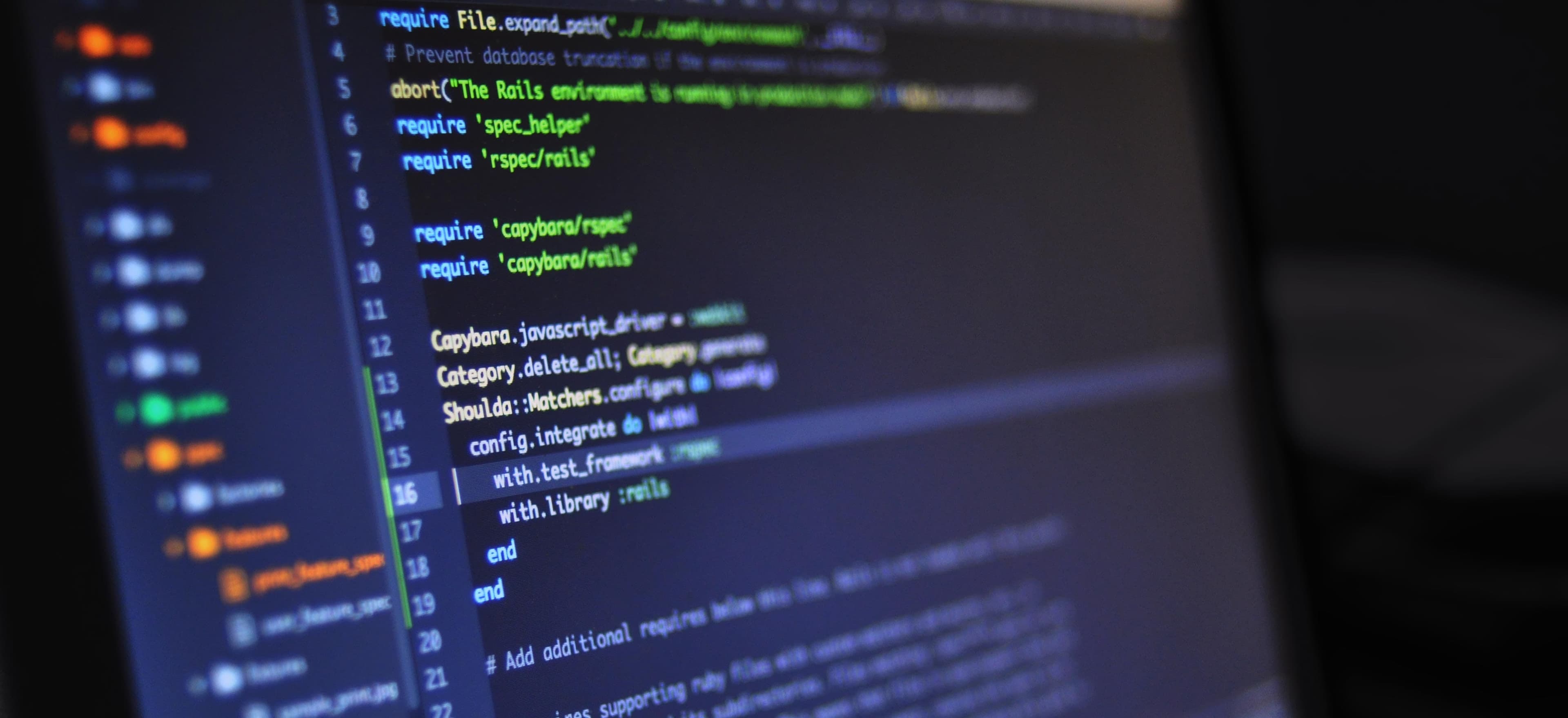
- Published on
Why Ignoring Java Coding Conventions Can Ruin Your Project
When starting a new Java project, developers often focus on implementing features, improving performance, and making their code work. While these aspects are crucial, there is one vital area that can make or break your project’s success: Java coding conventions. Ignoring these conventions can lead to a host of problems that could jeopardize the maintainability, readability, and scalability of your codebase.
In this post, we will explore the significance of adhering to Java coding conventions, the potential pitfalls of neglecting them, and some best practices to ensure your code is clean, understandable, and professional.
1. What are Java Coding Conventions?
Java coding conventions are a set of guidelines that standardize the way Java code should be written and organized. These conventions cover numerous aspects, including:
- Naming conventions: How to name classes, methods, variables, and constants.
- Code layout: Indentation levels, line spacing, and braces placement.
- Documentation: Standards for writing comments and method documentation.
The Java programming community and organizations such as Oracle have established these standards to create consistent and readable code.
For more detailed guidelines, you can explore Oracle's Coding Conventions.
2. The Importance of Consistency
Consistency is key. When multiple developers work on a project, adhering to a common set of coding conventions minimizes the cognitive load and makes it easier to read and understand each other's work.
Example: Naming Conventions
Consider the following two variable naming practices:
int numTimesClicked;
int n; // Hard to understand the purpose
In the first example, numTimesClicked
clearly conveys the variable's purpose, while n
provides no meaningful context. Using meaningful names improves code readability and helps team members quickly grasp the logic behind the code.
3. Minimized Errors and Bugs
Ignoring coding conventions can result in a rise in the number of bugs within your application. When code is improperly formatted and difficult to read, it's easy to overlook potential issues during development and debugging phases.
Example: Braces Placement
Consider the two different ways of structuring if-else statements:
if (someCondition) {
doSomething();
} else
doSomethingElse(); // No braces used here
This format can lead to confusion and mistakes, especially if you later add more lines to the else block. The proper way to structure this is:
if (someCondition) {
doSomething();
} else {
doSomethingElse(); // Braces make it clear
}
Using braces consistently, even for single statements, helps prevent bugs and improves clarity.
4. Enhancing Readability
Readable code doesn't just facilitate understanding for others; it also serves future you. Code that's easy to read will be easier to refactor, change, or debug later.
Example: Method Documentation
Using JavaDoc comments to document your methods is essential.
/**
* Calculates the area of a rectangle.
*
* @param width The width of the rectangle.
* @param height The height of the rectangle.
* @return The area of the rectangle.
*/
public double calculateArea(double width, double height) {
return width * height;
}
This documentation helps other developers and your future self understand what the method does and how to use it. Ignoring comments or writing vague documentation can lead to misunderstandings and misuse of your code.
5. Facilitating Collaboration
In a team environment, coding conventions promote effective collaboration. New team members can onboard quickly by understanding the code structure and logic without needing extensive documentation.
Example: Project Structure
Adherence to a consistent project structure is equally important. For instance, placing your classes into appropriate packages can help others to navigate the project easily.
src/
com/
yourcompany/
project/
service/
UserService.java
controller/
UserController.java
This structure organizes the project logically. New developers can instantly identify where to find services, controllers, or models associated with the project.
6. Simplifying Maintenance and Updates
The tech world is ever-evolving. As such, the need for periodic updates and maintenance of your project cannot be overstated. Well-structured code based on coding conventions allows for easier updates, modifications, and enhancements.
Example: Refactoring
Imagine you want to refactor a part of your application to improve performance. Adhering to coding conventions makes it easier to identify the specific classes or methods that need attention:
public class UserService {
public User findUserById(int id) {
// Database call to find user
}
}
If this method follows naming conventions clearly, you can easily find it to refactor and improve performance without having to sift through loads of poorly structured code.
7. Improving Code Reviews
Code reviews are a common practice in development teams. If the code adheres to established conventions, code reviews become more efficient. Reviewers can focus on logic and implementation rather than getting bogged down in formatting and style issues.
Example: Clean Code
Clean code that follows conventions can quickly be evaluated, allowing reviewers to provide valuable feedback on the core functionalities and logic rather than syntax:
public class User {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, clear getter and setter methods help reviewers see the class’s functionality effortlessly, allowing them to provide informed and constructive feedback.
Key Takeaways
Ignoring Java coding conventions can lead to readability issues, increase the likelihood of bugs, complicate maintenance, and hinder collaboration within your development team. As you embark on your next Java project, remember that the success of your project does not solely rely on functional code but also on how cleanly and consistently your code is written.
By adhering to Java coding conventions, you ensure that your code is understandable not just for you but for anyone who might work on the project in the future.
Make coding conventions an integral part of your development workflow to enhance code quality and achieve long-term project success.
For more insights on Java coding practices, you might find these resources helpful:
- Clean Code Principles
- Effective Java: A Programming Language Guide
Ensuring consistency is paramount. Commit to coding conventions; your future self and your team will thank you!