Struggling to Capture HTTP Session ID in Spring WebSocket?
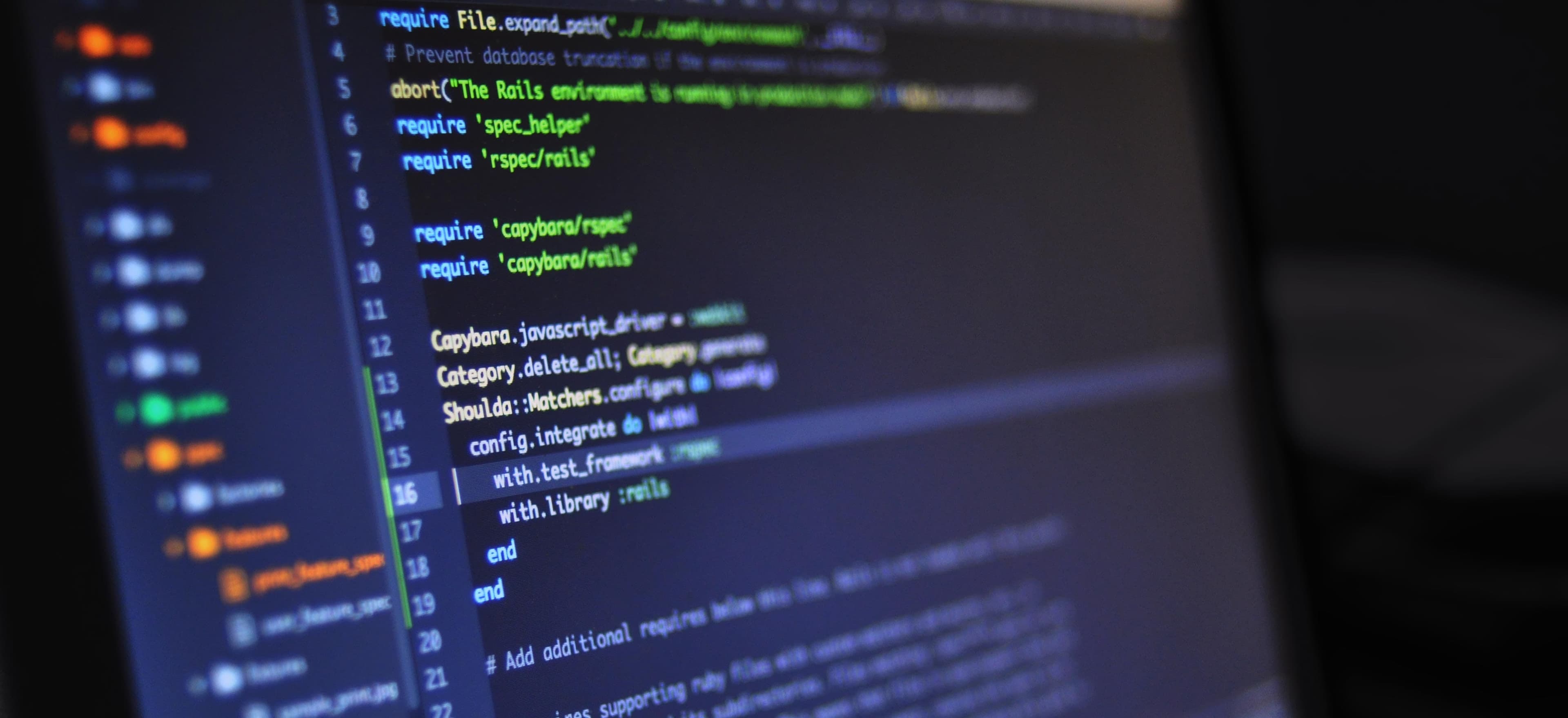
- Published on
Struggling to Capture HTTP Session ID in Spring WebSocket?
Spring WebSocket provides a powerful way to build real-time applications using the WebSocket protocol. One of the common challenges developers face when implementing this is capturing the HTTP Session ID during a WebSocket handshake. This article will guide you through the process, providing example code snippets along with explanations to clarify each step.
Understanding WebSocket Handshake
Before diving into the solution, it's important to understand the WebSocket handshake process. When a client (usually a web browser) wants to establish a connection with the server, it sends an HTTP request to upgrade the connection to WebSocket. This process is crucial for session tracking, especially when you need to tie WebSocket communications to existing HTTP sessions.
The Importance of Capturing HTTP Session ID
Capturing the HTTP Session ID is vital for scenarios where you need to maintain user-specific states during WebSocket communication. For example, if a user is logged into your application and initiates a WebSocket connection, you would want to ensure that you can track their session throughout the lifecycle of that connection. This allows you to handle messages, authentication, and user data effectively.
Steps to Capture HTTP Session ID in Spring WebSocket
1. Setup Spring WebSocket Configuration
To start, ensure that you have the necessary Spring WebSocket dependencies in your pom.xml
or build.gradle
. Here, we'll use Maven as an example:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
2. Create WebSocket Configuration Class
Next, create a configuration class that enables WebSocket support. Here's an example:
import org.springframework.context.annotation.Configuration;
import org.springframework.web.socket.config.annotation.EnableWebSocket;
import org.springframework.web.socket.config.annotation.WebSocketConfigurer;
import org.springframework.web.socket.config.annotation.WebSocketHandlerRegistry;
@Configuration
@EnableWebSocket
public class WebSocketConfig implements WebSocketConfigurer {
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) {
registry.addHandler(new ChatWebSocketHandler(), "/chat")
.setAllowedOrigins("*")
.withSockJS(); // Enable SockJS support for fallback.
}
}
In this configuration, we register a new WebSocket handler (ChatWebSocketHandler
) for the endpoint /chat
. The withSockJS()
method provides graceful degradation for browsers that do not support WebSockets.
3. Implement WebSocket Handler
Now, we need to implement the ChatWebSocketHandler
. This is where we capture the HTTP Session ID.
import org.springframework.web.socket.WebSocketSession;
import org.springframework.web.socket.handler.TextWebSocketHandler;
public class ChatWebSocketHandler extends TextWebSocketHandler {
@Override
public void afterConnectionEstablished(WebSocketSession session) throws Exception {
// Retrieve HTTP session ID
String httpSessionId = (String) session.getAttributes().get("HTTP_SESSION_ID");
System.out.println("Connected HTTP Session ID: " + httpSessionId);
// Additional logic, such as user identification can go here
}
@Override
protected void handleTextMessage(WebSocketSession session, TextMessage message) throws Exception {
// Handling incoming messages
}
}
Explanation
In the afterConnectionEstablished
method, we leverage session.getAttributes()
to fetch the HTTP Session ID captured during the handshake. The value associated with "HTTP_SESSION_ID" should be set during the handshake process. This is where a little extra work comes in.
4. Capturing the Session ID During Handshake
To bind the HTTP session with the WebSocket session, you'll need a custom HandshakeInterceptor
. Let's create one:
import org.springframework.web.socket.server.HandshakeInterceptor;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.Map;
public class HttpSessionIdHandshakeInterceptor implements HandshakeInterceptor {
@Override
public boolean beforeHandshake(HttpServletRequest request, HttpServletResponse response,
WebSocketHandler wsHandler, Map<String, Object> attributes) throws Exception {
String sessionId = request.getSession().getId();
attributes.put("HTTP_SESSION_ID", sessionId);
return true; // Returning true proceeds with the handshake
}
@Override
public void afterHandshake(HttpServletRequest request, HttpServletResponse response,
WebSocketHandler wsHandler, Exception exception) {
// Logic after the handshake. Can be left blank.
}
}
Integrating the Interceptor
Now, integrate this interceptor into the WebSocket configuration:
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) {
registry.addHandler(new ChatWebSocketHandler(), "/chat")
.setAllowedOrigins("*")
.addInterceptors(new HttpSessionIdHandshakeInterceptor())
.withSockJS();
}
5. Testing Your Implementation
You can test the implemented WebSocket functionality using a simple frontend JavaScript code snippet. Here’s how to connect to the WebSocket server:
const socket = new WebSocket("ws://localhost:8080/chat");
socket.onopen = function(event) {
console.log("WebSocket is open now.");
};
socket.onmessage = function(event) {
console.log("Message from server: ", event.data);
};
In Conclusion, Here is What Matters
Capturing the HTTP Session ID during the Spring WebSocket handshake is straightforward with proper configuration. By using a HandshakeInterceptor
, you can easily map the existing HTTP session details to the WebSocket session.
This approach not only allows you to maintain user authentication states but also provides a robust method for managing personalized interactions in real-time applications.
For a deeper dive into Spring WebSocket, check the official Spring Documentation.
If you have any questions or run into issues while implementing this, feel free to reach out through the comments below!
Happy coding!
Checkout our other articles