Autowiring Dilemma: When to Choose Manual Wiring in Spring
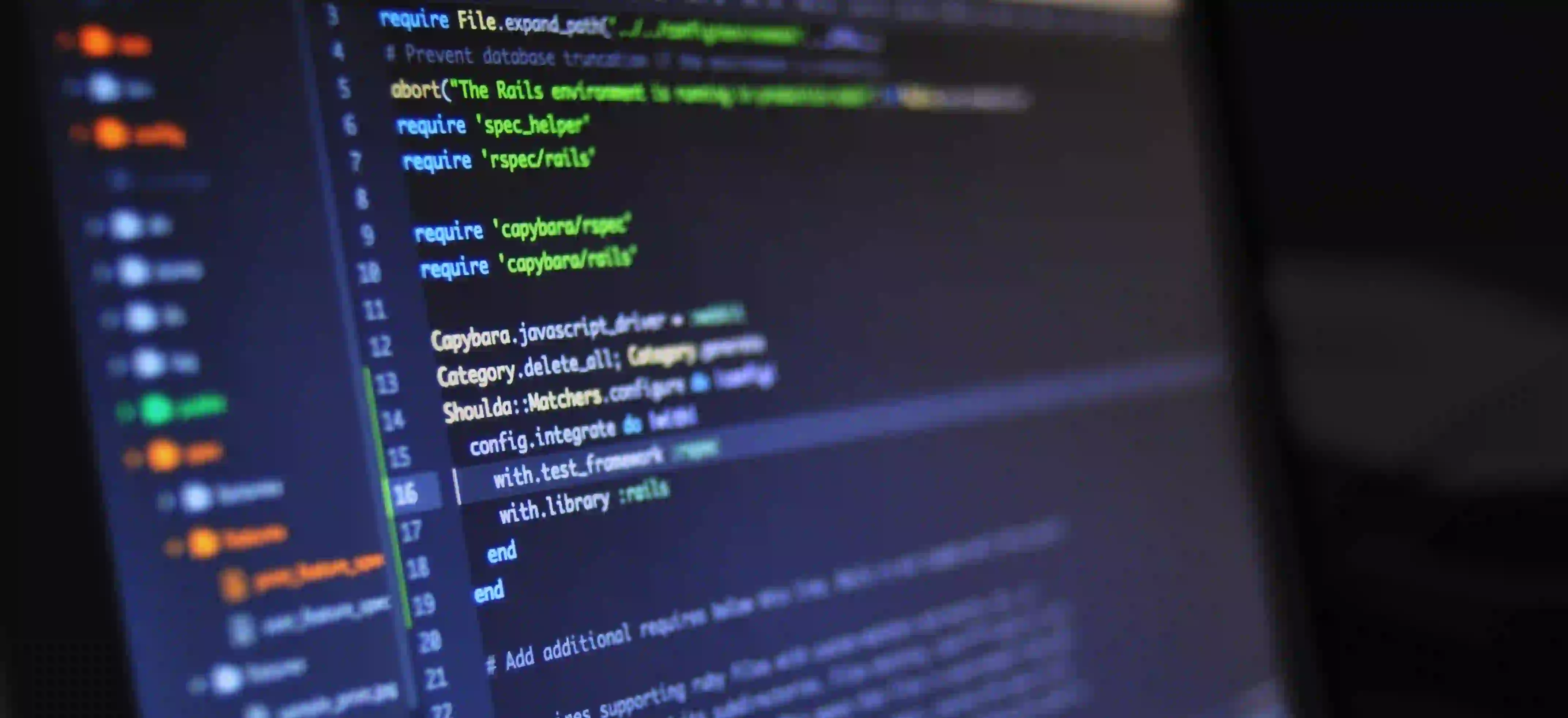
Autowiring Dilemma: When to Choose Manual Wiring in Spring
Spring Framework has transformed the way we develop Java applications. Over the years, it has introduced various features that enhance productivity and flexibility, one being Dependency Injection (DI). While Spring conveniently offers autowiring as a means to manage dependencies, there are scenarios where manual wiring could be more appropriate. This blog post delves into the autowiring dilemma and explores when to choose manual wiring in Spring.
Understanding Dependency Injection
Dependency Injection is a design pattern that allows a class to receive its dependencies from an external source rather than creating them internally. This promotes loose coupling and enhances testability. Spring supports DI through annotations like @Autowired
and XML configuration.
Autowiring in Spring
Autowiring enables Spring to automatically resolve and inject dependencies by type. Consider the following simple example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class StudentService {
private final StudentRepository studentRepository;
@Autowired
public StudentService(StudentRepository studentRepository) {
this.studentRepository = studentRepository;
}
public void printStudent() {
System.out.println(studentRepository.getStudent());
}
}
In this snippet, StudentService
has a dependency on StudentRepository
. By annotating the constructor with @Autowired
, Spring automatically injects the appropriate instance of StudentRepository
.
Advantages of Autowiring
- Conciseness: Less boilerplate code compared to manual wiring.
- Ease of management: Dependencies can easily be managed by the Spring container.
- Faster development: Reduces the complexity of handling dependencies in your application.
When to Choose Manual Wiring
Despite the advantages, autowiring is not a one-size-fits-all solution. Here are a few scenarios when manual wiring may be preferable.
1. Complex Dependency Management
In cases where the application has complex dependencies with multiple constructors or differing configurations, manual wiring offers finer control. You can explicitly define dependencies without relying on ambiguous bean types.
For instance:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public StudentRepository studentRepository() {
return new StudentRepository();
}
@Bean
public StudentService studentService() {
return new StudentService(studentRepository());
}
}
In this example, we manually wire StudentService
with StudentRepository
, giving us the authority to control the instantiation and lifecycle of these beans.
2. Required vs. Optional Dependencies
Autowiring can create ambiguity when determining whether a dependency is required or optional. You can specify optional dependencies via constructors or setter methods, but it may lead to issues when the required dependencies are not satisfied.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class StudentService {
private final StudentRepository studentRepository;
@Autowired(required = false)
public StudentService(StudentRepository studentRepository) {
this.studentRepository = studentRepository;
}
public void printStudent() {
if (studentRepository != null) {
System.out.println(studentRepository.getStudent());
} else {
System.out.println("No repository available");
}
}
}
While the code compiles, it risks running into NullPointerExceptions at runtime. Manual wiring enables developers to avoid this issue by explicitly controlling when and how dependencies are instantiated.
3. Quality of Code and Readability
While autowiring can lead to less code, it can also obscure dependencies, making the code harder to read. Manual wiring can improve clarity, thereby improving maintainability. Each dependency is explicitly defined, making the structure of your application more understandable.
4. Decoupling for Testing
Testing is an essential part of developing robust software. Autowiring can bind your classes to specific implementations, making unit tests difficult. Manual wiring allows you to replace real dependencies with mock ones during tests, enhancing isolation.
Here's an example with mock dependencies using manual wiring:
import org.junit.jupiter.api.Test;
import org.mockito.Mockito;
public class StudentServiceTest {
@Test
public void testPrintStudent() {
StudentRepository mockRepository = Mockito.mock(StudentRepository.class);
Mockito.when(mockRepository.getStudent()).thenReturn("Test Student");
StudentService studentService = new StudentService(mockRepository);
studentService.printStudent(); // Output: Test Student
}
}
This testing setup ensures that StudentService
can be tested without any reliance on actual implementations.
5. Scope Issues
Autowiring relies heavily on the Spring bean scope. If a bean's scope is not accurately defined, it can lead to unpredictable behaviors regarding the lifecycle of your objects. With manual wiring, you can determine the scope more clearly.
Consider a singleton service that holds state:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class UserSessionService {
private UserSession userSession;
@Autowired
public UserSessionService(UserSession userSession) {
this.userSession = userSession;
}
}
If UserSession
is instanciated as a prototype, multiple instances may lead to conflicting data in concurrent request scenarios. Manual wiring allows better control on session handling.
Final Thoughts
While Spring's autowiring feature brings many conveniences, there are compelling reasons to consider manual wiring. Whether handling complex dependencies, managing required versus optional setups, improving code readability, enhancing testability, or properly scoping beans, manual wiring can sometimes be the more robust solution.
Additional Resources
In the ever-evolving world of software development, being aware of these foundational choices empowers developers. Choose wisely, and tailor your dependency management strategy to fit your application's needs. Happy coding!