Optimizing Java for Massive Low-Latency Queues
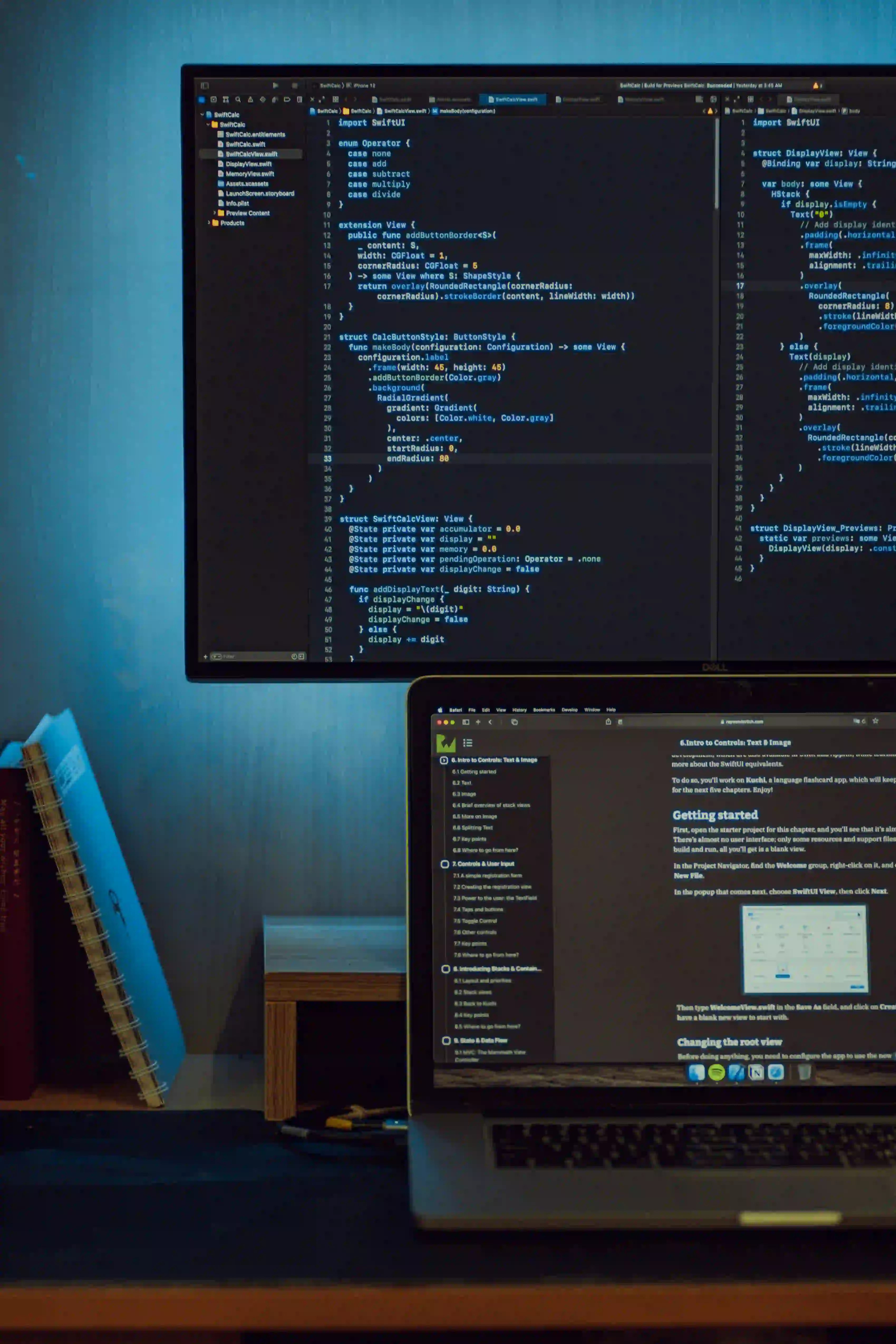
Optimizing Java for Massive Low-Latency Queues
When it comes to today's demanding applications, optimizing Java for massive low-latency queues is crucial. With the rise of real-time processing, whether you are working with financial transactions or live data streams, the efficiency of your queue can significantly influence the overall performance of your application.
In this blog post, we will discuss various strategies for optimizing Java with a focus on creating effective queues. We will explore different data structures, threading strategies, and specific Java libraries that can help you achieve the performance you desire.
Understanding Latency
Latency, in the context of Queues, can be described as the time delay before a transfer of data begins following an instruction. Low-latency response times are critical for applications requiring high throughput and real-time data processing. To optimize for low latency, understanding the factors that introduce latency is essential:
- Data Structure Overhead: Certain data structures come with inherent overheads that can slow down processing times.
- Garbage Collection: Java's automated memory management can introduce latency spikes if not properly managed.
- Thread Management: Inefficient threading can lead to contention and performance bottlenecks.
Selecting the Right Data Structure
When implementing low-latency queues, choosing the right data structure can have a substantial impact on performance. The following options are popular for low-latency queues:
ArrayDeque
ArrayDeque
is a resizable array implementation of the Deque
interface. It is often favored due to its low overhead and constant-time performance for adding and removing elements from both ends.
import java.util.ArrayDeque;
import java.util.Queue;
public class LowLatencyQueue {
public static void main(String[] args) {
Queue<Integer> queue = new ArrayDeque<>();
// Adding elements
for (int i = 0; i < 10; i++) {
queue.offer(i);
}
// Processing elements
while (!queue.isEmpty()) {
System.out.println(queue.poll());
}
}
}
Why Use ArrayDeque?
- Fast Access: Constant time for adding and removing elements.
- Minimized Overhead: Unlike linked lists, it avoids the overhead of node pointers.
- No capacity constraints: It dynamically resizes as needed.
ConcurrentLinkedQueue
For concurrent environments, ConcurrentLinkedQueue
is often the go-to option. It is a non-blocking, thread-safe implementation that uses an efficient lock-free algorithm.
import java.util.concurrent.ConcurrentLinkedQueue;
public class ConcurrentLowLatencyQueue {
public static void main(String[] args) {
ConcurrentLinkedQueue<Integer> queue = new ConcurrentLinkedQueue<>();
// Adding elements
for (int i = 0; i < 10; i++) {
queue.offer(i);
}
// Processing elements
Integer element;
while ((element = queue.poll()) != null) {
System.out.println(element);
}
}
}
Why choose ConcurrentLinkedQueue?
- Thread-Safe: Designed specifically for concurrent use.
- Lock-Free: Reduces the risk of thread contention and improves performance in multi-threaded environments.
These two data structures, ArrayDeque
for single-threaded contexts and ConcurrentLinkedQueue
for multi-threaded scenarios, can effectively optimize performance for massive low-latency queues.
Managing Garbage Collection
Garbage Collection (GC) can introduce significant latency, primarily during the Full GC phase. Here are some tips to mitigate its impact:
Use the G1 Garbage Collector
Starting with Java 9, the G1 GC is the default collector and is known for its low-pause performance. It splits the heap into regions and compacts them in a way that minimizes pauses.
You can enable it with:
-java -XX:+UseG1GC
Tuning GC Parameters: Experiment with parameters such as -XX:MaxGCPauseMillis
to specify your pause time goal.
Object Pooling
Pooling objects can significantly reduce the pressure on the Garbage Collector. Instead of creating new objects each time, reuse existing ones:
class IntegerPool {
private static final int MAX_SIZE = 1000;
private final Queue<Integer> pool = new ConcurrentLinkedQueue<>();
public Integer acquire() {
Integer value = pool.poll();
return (value != null) ? value : new Integer();
}
public void release(Integer value) {
if (pool.size() < MAX_SIZE) {
pool.offer(value);
}
}
}
Why Use Object Pools?
- Reduced GC Pressure: Less frequent creation and disposal of objects.
- Improved Throughput: Faster execution through reduced allocation overhead.
Thread Management for Low Latency
Efficient thread management is critical in a low-latency environment. The Java ForkJoinPool
and Executors.newCachedThreadPool()
can be beneficial.
ForkJoinPool
When dealing with tasks that can be broken down into subtasks, ForkJoinPool
comes into play.
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
class SumTask extends RecursiveTask<Integer> {
private final int[] nums;
private final int start, end;
public SumTask(int[] nums, int start, int end) {
this.nums = nums;
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
if (end - start <= 10) {
int sum = 0;
for (int i = start; i < end; i++) {
sum += nums[i];
}
return sum;
} else {
int mid = (start + end) / 2;
SumTask left = new SumTask(nums, start, mid);
SumTask right = new SumTask(nums, mid, end);
left.fork();
return right.compute() + left.join();
}
}
}
public class ForkJoinExample {
public static void main(String[] args) {
int[] nums = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
ForkJoinPool pool = new ForkJoinPool();
SumTask task = new SumTask(nums, 0, nums.length);
int result = pool.invoke(task);
System.out.println("Sum: " + result);
}
}
Why Use ForkJoinPool?
- Dynamic Thread Allocation: Efficiently allocates threads based on workload.
- Fine-Grained Parallelism: Splits tasks enabling better CPU utilization.
The Last Word
Optimizing Java for massive low-latency queues involves selecting appropriate data structures, managing garbage collection wisely, and leveraging effective thread management. By following the strategies outlined in this article, you can improve the performance of your applications and reduce latency.
Keep experimenting with different configurations, measure your results, and adjust according to your specific use cases. If you need further reading on this topic, consider checking resources on Java concurrency and garbage collection techniques.
By combining the power of Java with these optimization strategies, you can achieve a new level of efficiency in your applications, paving the way for robust low-latency solutions. Happy coding!