Struggling to Find a Key in LinkedHashMap? Try This!
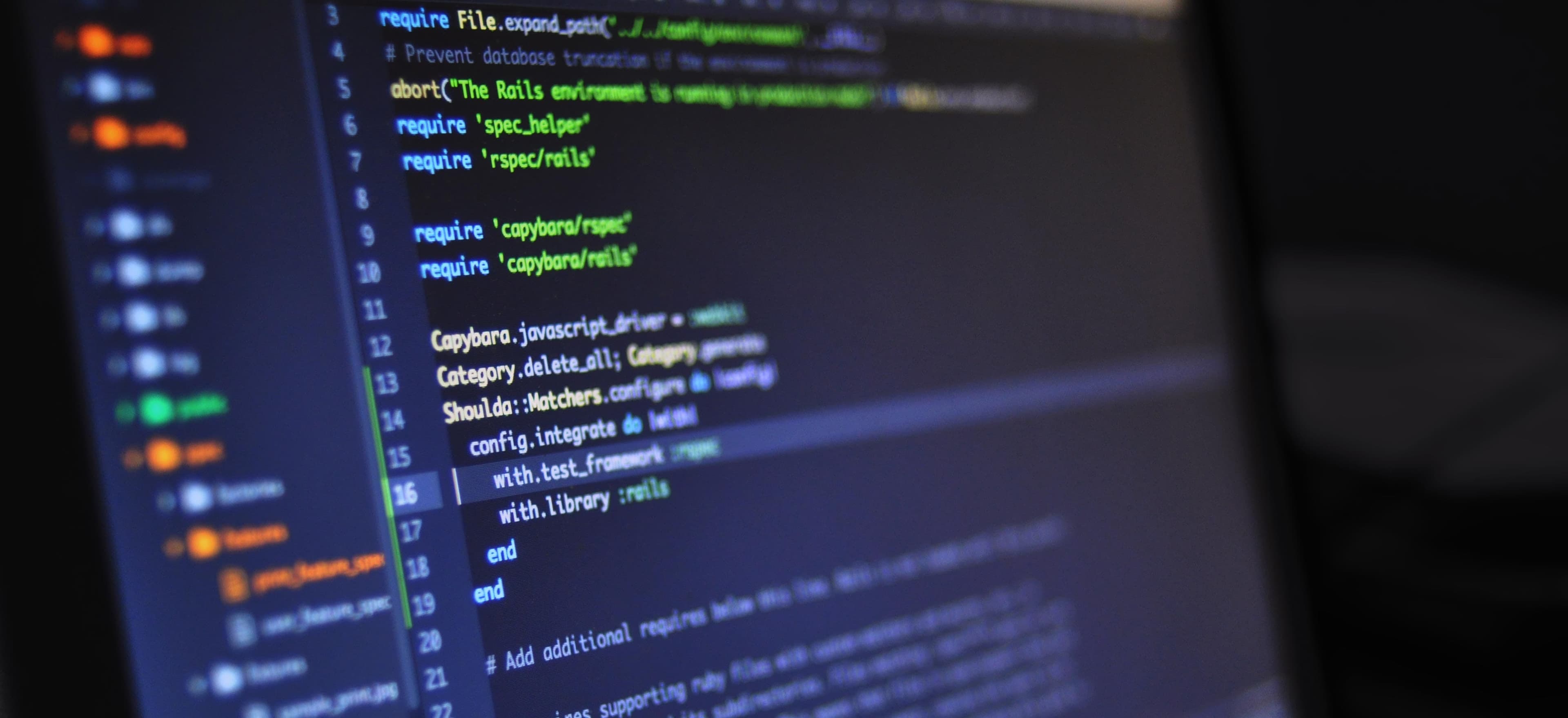
- Published on
Struggling to Find a Key in LinkedHashMap? Try This!
As Java developers, we often encounter collections that help manage our data effectively. Among these data structures, the LinkedHashMap
stands out due to its ability to maintain both insertion order and key-value mappings. In this post, we will delve into how you can efficiently find a key in a LinkedHashMap
, why this is important, and some tips on optimizing your search.
Understanding LinkedHashMap
Before we explore how to find a key in a LinkedHashMap
, let’s briefly discuss what it is and why you might choose it over other collection types.
A LinkedHashMap
is part of Java's Collections Framework. It extends HashMap
and maintains a doubly-linked list of entries. This structure allows it to remember the order of insertion. Consequently, when you iterate through a LinkedHashMap
, you will receive entries in the order they were added.
Key Characteristics:
- Maintains Insertion Order: Unlike a
HashMap
, which does not guarantee any order,LinkedHashMap
preserves the order of elements. - Performance: It provides O(1) complexity for
get()
,put()
, andremove()
operations on average, making it a great choice for performance-critical applications.
You can learn more about LinkedHashMap
here.
Why Searching for a Key Matters
When working with data, the ability to quickly locate a key can save time and resources. Whether you're managing user sessions, caching data, or tracking application states, knowing how to efficiently search will enhance your application's performance.
Example Scenario:
Imagine you are developing a web application that tracks user sessions. Using a LinkedHashMap
allows you to store session IDs as keys and user information as values. Quickly finding a session based on its ID is crucial for performance and user experience.
Finding a Key in LinkedHashMap
Searching for a key in a LinkedHashMap
is straightforward. You can use the containsKey()
method, which determines whether a specified key is present in the map.
Here is an example demonstrating how to check for a key's existence:
import java.util.LinkedHashMap;
public class KeySearchExample {
public static void main(String[] args) {
// Creating a LinkedHashMap
LinkedHashMap<String, String> userSessions = new LinkedHashMap<>();
userSessions.put("session1", "User1");
userSessions.put("session2", "User2");
userSessions.put("session3", "User3");
// Key to search for
String searchKey = "session2";
// Check if the key exists
if (userSessions.containsKey(searchKey)) {
System.out.println("Found: " + searchKey + " -> " + userSessions.get(searchKey));
} else {
System.out.println("Key not found: " + searchKey);
}
}
}
Code Commentary:
- Creating the LinkedHashMap: We first instantiate a
LinkedHashMap
and add some user session mappings. containsKey
Method: This method checks for the presence of a specific key efficiently, returningtrue
orfalse
.- Retrieving Values: If the key exists, we retrieve its associated value, showcasing how to access data from the map.
Output:
Found: session2 -> User2
Performance Considerations
While searching for a key in a LinkedHashMap
is efficient, there are some additional strategies you can consider to further improve performance:
1. Size Your Map Correctly
If you know the number of entries your LinkedHashMap
will hold, you can initialize it with a specified capacity. This minimizes the need for resizing:
int initialCapacity = 50; // Set this based on your needs
LinkedHashMap<String, String> map = new LinkedHashMap<>(initialCapacity);
2. Iterate When Necessary
If you need to perform multiple key lookups, consider iterating through your keys once instead of making multiple containsKey()
checks. This is particularly useful in a performance-critical environment.
for (String key : userSessions.keySet()) {
if (key.equals(searchKey)) {
System.out.println("Found during iteration: " + key);
}
}
3. Optimize Data Access Patterns
If you’re frequently accessing the map, consider caching data or using a secondary structure that holds the most commonly accessed keys.
Use Cases in Real-World Applications
Here we will explore a few scenarios where LinkedHashMap
shines in terms of key searching.
1. Caching
A classic use of LinkedHashMap
is to implement caching. It allows easy addition and eviction based on access patterns.
2. Maintaining State
You might use LinkedHashMap
to remember user settings across sessions. Being able to quickly find a setting based on a key ensures smooth user experience.
3. User Profiles
In large-scale applications, maintaining user profiles with the ability to search and modify data efficiently helps in providing personalized content.
The Closing Argument
Finding a key in a LinkedHashMap
may seem simple, but understanding the underlying mechanisms and performance considerations can greatly enhance your Java applications. With the proper use of containsKey()
and careful design choices, you can ensure that your applications are both efficient and scalable.
For additional reading on optimizing Java collections, you can refer to Java Collections Tutorial.
Feel free to leave your comments below if you have questions or want to share how you are using LinkedHashMap
in your projects! Happy coding!
Checkout our other articles