“Common Autowiring Pitfalls in Spring Framework”
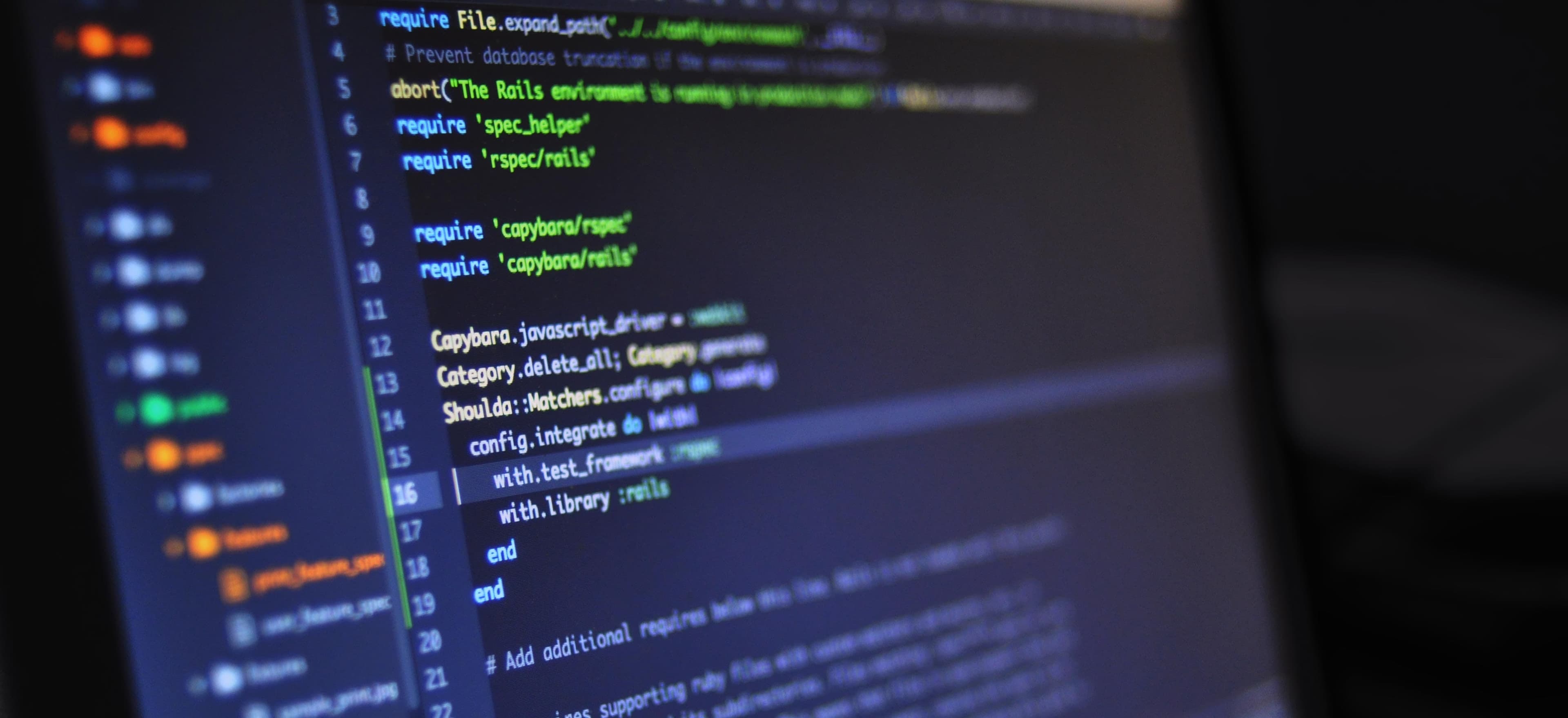
- Published on
Common Autowiring Pitfalls in Spring Framework
The Spring Framework is a powerful framework for building Java applications, renowned for its capabilities in dependency injection and aspect-oriented programming. One of the standout features of Spring is its autowiring functionality, which simplifies how beans are created and managed in your application. However, while powerful, autowiring can also lead to unexpected pitfalls if not used correctly. In this blog post, we will explore common autowiring issues you may encounter in Spring Framework and provide insight into how to avoid them.
What is Autowiring?
Before diving into the pitfalls, let’s clarify what autowiring is. In Spring, autowiring is a feature that allows the framework to automatically inject the beans into your application components without you having to explicitly declare each dependency. This can be done via annotations like @Autowired
or XML configuration.
The primary autowiring modes that Spring offers include:
- By Type: Spring looks for a bean that matches the data type of the property.
- By Name: Spring looks for a bean that matches the name of the property.
- Constructor: Spring uses the constructor to autowire dependencies.
- Required: An optional flag that determines if the dependency is mandatory.
Let’s explore common pitfalls associated with autowiring.
Autowiring Pitfall #1: Circular Dependencies
Problem Explanation
One of the most notorious issues with autowiring is circular dependencies. This occurs when Bean A depends on Bean B, while Bean B also depends on Bean A. When Spring attempts to resolve these dependencies, it can lead to a BeanCurrentlyInCreationException
.
Example Explanation
Consider the example below:
@Component
public class BeanA {
@Autowired
private BeanB beanB;
public void doSomething() {
beanB.help();
}
}
@Component
public class BeanB {
@Autowired
private BeanA beanA;
public void help() {
System.out.println("It's working...");
}
}
Solution
To resolve this, you can break the circular dependency by refactoring your beans. This often involves using interfaces or a design pattern such as Dependency Injection. For example:
@Component
public class BeanA {
private final BeanB beanB;
@Autowired
public BeanA(BeanB beanB) {
this.beanB = beanB;
}
public void doSomething() {
beanB.help();
}
}
@Component
public class BeanB {
public void help() {
System.out.println("It's working...");
}
}
Notice how we eliminated the circular reference by using constructor injection.
Autowiring Pitfall #2: Ambiguous Beans
Problem Explanation
Ambiguous beans arise when Spring encounters more than one candidate for autowiring a specific dependency. When you have two beans of the same type, Spring does not know which one to inject, resulting in a NoUniqueBeanDefinitionException
.
Example Explanation
Here’s how it can happen:
@Component
public class Car {
@Autowired
private Engine engine; // Ambiguous if there are multiple Engine beans
}
@Component
public class Engine {
// Engine implementation
}
@Component
public class V8Engine extends Engine {
// V8 implementation
}
@Component
public class V6Engine extends Engine {
// V6 implementation
}
Solution
To resolve ambiguity, you can use the @Qualifier
annotation to specify which bean to inject. Here’s how:
@Component
public class Car {
@Autowired
@Qualifier("v8Engine")
private Engine engine; // Specify explicitly
}
This forces Spring to use the v8Engine
bean, eliminating the ambiguity.
For deeper insights on autowiring in Spring, visit the official Spring Documentation.
Autowiring Pitfall #3: Missed Dependency Injection
Problem Explanation
Sometimes, you may assume that a field has been injected when it wasn’t due to incorrect configuration or the omission of the @Autowired
annotation. This can lead to a NullPointerException
at runtime.
Example Explanation
Consider the following situation:
@Component
public class UserService {
private UserRepository userRepository; // Missing @Autowired
public void createUser() {
userRepository.save(new User());
}
}
Solution
Always ensure that necessary dependencies are annotated with @Autowired
. You might also want to utilize constructor injection or setter-based injection for clarity:
@Component
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository; // Ensures the dependency is injected
}
public void createUser() {
userRepository.save(new User());
}
}
Constructor injection not only ensures your dependencies are injected but also allows for cleaner unit testing.
Autowiring Pitfall #4: Prototype Beans in Singleton Scope
Problem Explanation
Injecting a prototype bean into a singleton bean leads to unexpected behavior, where the singleton retains only a single instance of the prototype.
Example Explanation
Here's a problematic example:
@Component
public class SingletonBean {
@Autowired
private PrototypeBean prototypeBean; // Only one instance will be injected!
}
@Component
@Scope("prototype")
public class PrototypeBean {
public PrototypeBean() {
System.out.println("PrototypeBean created");
}
}
Solution
To correctly use prototype beans within singleton beans, use a @Lookup
method. Here’s how you can modify the code:
@Component
public class SingletonBean {
@Lookup
public PrototypeBean getPrototypeBean() {
return null; // Spring will override this method
}
public void doSomething() {
PrototypeBean pb = getPrototypeBean();
// Use the prototype bean
}
}
In this way, each call to getPrototypeBean()
obtains a new instance of PrototypeBean
.
A Final Look
Autowiring in Spring is a feature that can simplify your life significantly by removing boilerplate code and enhancing modularity. However, care must be taken to avoid pitfalls like circular dependencies, ambiguous beans, missed dependency injection, and improper bean scopes. By adhering to best practices such as explicit bean qualification, consistent use of the @Autowired
annotation, and appropriate scope management, you can optimize your use of Spring's autowiring capabilities effectively.
For further details regarding dependency injection in Spring, you can consult this excellent resource by Spring: Spring Dependency Injection which provides practical examples and a hands-on guide.
By being aware of these common pitfalls and understanding how to circumvent them, you enhance the maintainability and reliability of your Spring applications. Happy coding!
Checkout our other articles