Understanding the Risks of JVM Method Inlining Aggression
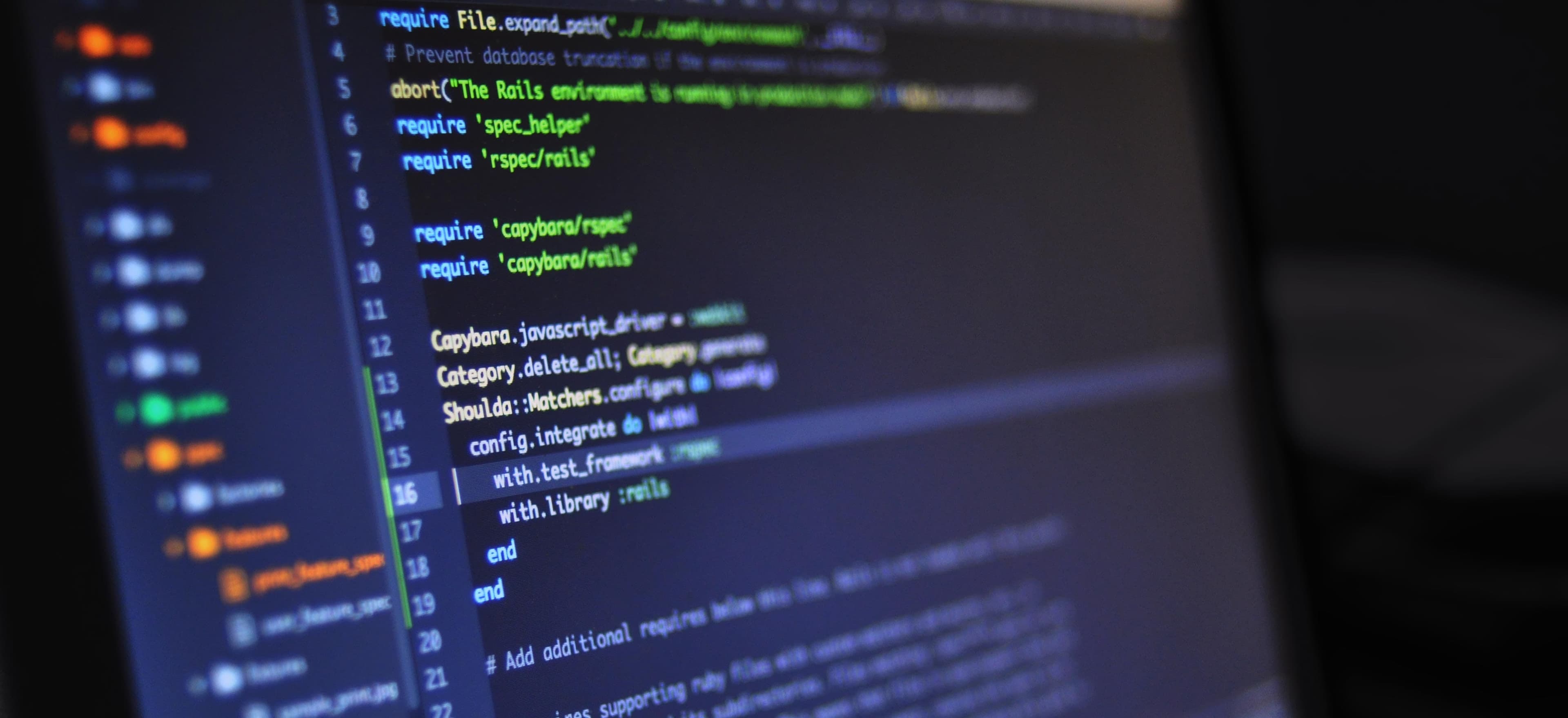
- Published on
Understanding the Risks of JVM Method Inlining Aggression
The Java Virtual Machine (JVM) optimizes code execution in numerous ways to improve performance, one of which includes method inlining. While this optimization technique can enhance performance significantly, there are risks associated with its aggressive application. Understanding these risks is crucial for Java developers who wish to write efficient and maintainable code.
What is Method Inlining?
Method inlining is a performance optimization technique where the method call is replaced with the body of the method itself. This can reduce the overhead of the call stack, eliminate certain branch instructions, and allow other optimizations to be performed on the inlined code.
Code Example:
public class InlineExample {
public int multiply(int a, int b) {
return a * b;
}
public int calculateArea(int length, int width) {
return multiply(length, width); // This method call can be inlined
}
}
In this example, if the JVM decides to inline multiply
, it might replace calculateArea
with:
public int calculateArea(int length, int width) {
return length * width; // Inlined version
}
This inlining reduces the number of method calls, thereby potentially increasing performance.
How Does the JVM Decide to Inline Methods?
The JVM uses various metrics to determine which methods to inline, including method size, frequency of invocation, and the overall impact on performance. In general, smaller methods that are called frequently are likely candidates for inlining.
The JVM employs several optimization levels—like C1 (Client Compiler) and C2 (Server Compiler)—that impact inlining decisions. The more aggressive C2 compiler can inline larger methods compared to C1, leading to better optimization at the expense of compile time and memory usage.
Advantages of Method Inlining
- Reduced Call Overhead: By eliminating method invocations, inlining reduces the call overhead often associated with methods.
- Better Optimization: When methods are inlined, the JVM can apply additional optimizations such as loop unrolling and constant propagation since it has all the code in one place.
- Increased Performance: Many applications experience faster execution times when method inlining is employed effectively, particularly in performance-critical areas.
Risks of Aggressive Method Inlining
While method inlining presents many benefits, aggressive inlining comes with notable risks:
1. Increased Binary Size
Inlining larger methods can lead to a significant increase in binary size. This means more code is loaded into memory and increased cache usage, which could lead to cache misses.
Impact: Larger binaries can lead to decreased performance due to increased memory access times.
2. Decreased Maintainability
When methods become excessively large due to aggressive inlining, it might result in code that is harder to read, understand, and maintain. This increased complexity can also introduce bugs.
Consideration: Maintainability is crucial for teams working on projects over extended periods.
3. Profiler Misleading Data
Aggressive inlining can skew profiling data. Profilers depend on method call statistics to make suggestions for optimizations. If inlining distorts this data, developers might misinterpret the effectiveness of their optimizations.
Suggestion: It's essential to regularly profile your application with different configurations to identify how inlining impacts performance.
4. Loss of Dynamic Method Resolution
Java supports dynamic method resolution through polymorphism. Excessive inlining can hinder this feature since the JVM might decide to inline a method that should have been dispatched dynamically.
Example:
class Base {
public void show() {
System.out.println("Base show");
}
}
class Derived extends Base {
public void show() {
System.out.println("Derived show");
}
}
class Test {
public void display(Base obj) {
obj.show(); // May miss the derived implementation if inlined aggressively
}
}
In the above example, if the show
method in display
is inlined, the JVM may not correctly resolve which version to execute at runtime, potentially leading to application logic errors.
5. JIT Compiler Pressure
The Just-In-Time (JIT) compiler must balance between performance gains and memory efficiency. When inlining creates excessive overhead, it can strain JIT compiler resources, leading to slower runtime performance.
6. Increase in Compilation Time
Aggressive inlining can lead to increased compilation times while the JIT compiler analyzes and prepares code paths. This could negatively impact performance during the startup phase of applications.
Best Practices for Managing Inlining Risks
-
Understand Your Application's Profile: Regularly use profilers to analyze method call frequencies and execution paths to better understand the impact of inlining.
-
Monitor Performance Metrics: Keep an eye on binary size, cache performance, and overall runtime efficiency after applying aggressive inlining tactics.
-
Use the -XX:InlineSmallCode=0 flag: To disable inlining for small methods if you encounter issues related to large binary size.
-
Limit the Size of Methods: Aim for smaller methods during development. This can lead to better maintainability and less aggressive inlining by the JVM.
-
Test with Different JVM Settings: Experiment with compiler flags to find the optimal balance for your specific application. Use options like
-XX:TieredStopAtLevel
to halt compilation after certain steps and analyze its performance impact. -
Get Familiar with Profiling Tools: Tools like VisualVM, YourKit, and Java Mission Control can help in profiling JVM performance and tracking method inlining impact.
Closing Remarks: A Balanced Approach
While method inlining is an effective optimization, it's essential to recognize and understand the associated risks. An aggressive approach can yield benefits, but it may also lead to potential pitfalls in maintainability, complexity, and performance.
Developers should focus on maintaining a balance. Profiling, monitoring, and adhering to best practices around method design will help ensure that your application remains both performant and maintainable.
For more in-depth information on JVM performance, visit Oracle's Java Performance Tuning Guide. Additionally, exploring resources like Java Performance Tuning by Jack Shirazi will enhance your understanding of these advanced concepts.
By leveraging the right strategies and understanding the underlying mechanics, you can harness the power of method inlining while mitigating its risks effectively.
Checkout our other articles