Mastering Word Count in Java with Lambdas and ForkJoin
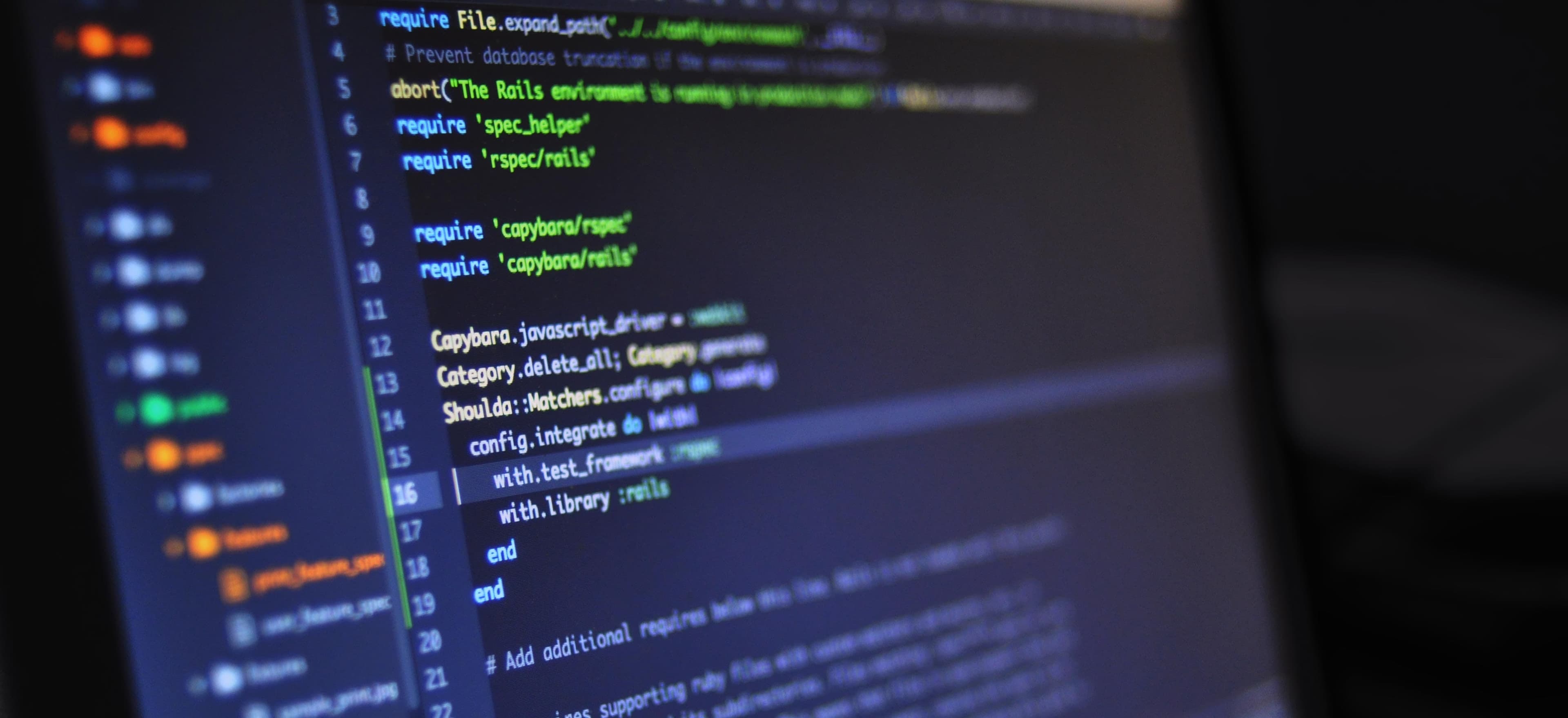
- Published on
Mastering Word Count in Java with Lambdas and ForkJoin
In today's world, data processing is ubiquitous, and efficient handling of large datasets has become crucial. One fundamental task in data processing is counting words. This blog post will guide you through achieving word count in Java using modern features such as Lambdas and the Fork/Join Framework. By the end of this article, you will have a deeper understanding of these concepts, and how to leverage them for efficient and scalable programming.
A Quick Look to Word Counting
Counting words is conceptually simple: you take a block of text and determine how many words it contains. However, when dealing with large texts or multiple files, the computational complexity grows. This is where parallel processing can play a crucial role.
The Concept of Lambdas
Java introduced lambda expressions in Java 8, which allow you to pass functionality as parameters to methods, mainly used for defining the behavior of functional interfaces. They can make your code more concise and readable.
The Fork/Join Framework
The Fork/Join Framework is designed to take advantage of multiple processors, enabling parallel computation. It splits a task into smaller subtasks, processes them independently, and then combines the results.
Setting Up Your Java Environment
Before we dive into the code, make sure you are using Java 8 or higher. The examples provided in this article will utilize Java’s built-in capabilities for threading and functional programming.
Implementing Word Count with Lambdas
Let’s start with a simple example of counting words using a straightforward approach without parallelism.
Traditional Word Count
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class WordCount {
public static void main(String[] args) {
String filePath = "path/to/textfile.txt";
try {
List<String> lines = Files.readAllLines(Paths.get(filePath));
long wordCount = lines.stream()
.flatMap(line -> Stream.of(line.split("\\W+")))
.filter(word -> !word.isEmpty())
.count();
System.out.println("Total Words: " + wordCount);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explanation of the Code
-
Reading Lines: We utilize
Files.readAllLines()
to read the lines from the file. This method collects all lines into aList<String>
. -
Creating a Stream: We create a stream of lines and then use
flatMap
to split each line into words based on a regular expression that matches non-word characters. -
Filtering and Counting: After flattening the stream, we filter out empty strings and finally count the words using
count()
.
This approach is simple, but not optimal for very large files or multiple files.
Upgrading to Fork/Join
Next, let’s enhance our solution to handle larger texts using Java's Fork/Join framework. We'll be creating a separate task for each line of text.
Fork/Join Implementation
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class ForkJoinWordCount extends RecursiveTask<Long> {
private static final int THRESHOLD = 10;
private final List<String> lines;
public ForkJoinWordCount(List<String> lines) {
this.lines = lines;
}
@Override
protected Long compute() {
if (lines.size() <= THRESHOLD) {
return lines.stream()
.flatMap(line -> Stream.of(line.split("\\W+")))
.filter(word -> !word.isEmpty())
.count();
} else {
int mid = lines.size() / 2;
ForkJoinWordCount leftTask = new ForkJoinWordCount(lines.subList(0, mid));
ForkJoinWordCount rightTask = new ForkJoinWordCount(lines.subList(mid, lines.size()));
leftTask.fork(); // Fork the left task
long rightResult = rightTask.compute(); // Compute the right task
long leftResult = leftTask.join(); // Join the result of the left task
return leftResult + rightResult; // Combine results
}
}
public static void main(String[] args) {
String filePath = "path/to/textfile.txt";
try {
List<String> lines = Files.readAllLines(Paths.get(filePath));
ForkJoinPool pool = new ForkJoinPool();
ForkJoinWordCount task = new ForkJoinWordCount(lines);
long wordCount = pool.invoke(task);
System.out.println("Total Words: " + wordCount);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explanation of the Fork/Join Code
-
RecursiveTask: We extend
RecursiveTask<Long>
which allows us to return a result (the word count in our case). -
Threshold: If the number of lines is less than or equal to the threshold (10), the task processes it using streams. This reduces overhead for small tasks.
-
Recursive Division: If the number of lines exceeds the threshold, the method splits the list of lines in half, creating two subtasks.
-
Forking and Joining: Using
fork()
initiates the left task, andcompute()
is called on the right task. Finally,join()
retrieves the result of the left task, and both results are combined. -
Parallel Execution: A
ForkJoinPool
is used to invoke the resulting task.
Closing Remarks
In this blog post, we've explored how to perform word counting in Java, starting with a simple linear approach and progressing towards leveraging the power of parallel processing with the Fork/Join framework.
Utilizing these concepts, especially in large data processing scenarios, can greatly enhance performance and efficiency. You can find further insights into Java concurrency in the official Java Documentation.
Final Thoughts
The use of lambdas and parallel processing in Java helps you write clean, efficient, and scalable programs. Whether you are counting words or performing more complex data analyses, these strategies will serve as invaluable tools in your Java programming arsenal.
Don’t forget to explore these concepts further and see how they can be applied in your springboards into other areas of programming!
Checkout our other articles