Overcoming Common Pitfalls in JAX-RS Context Management
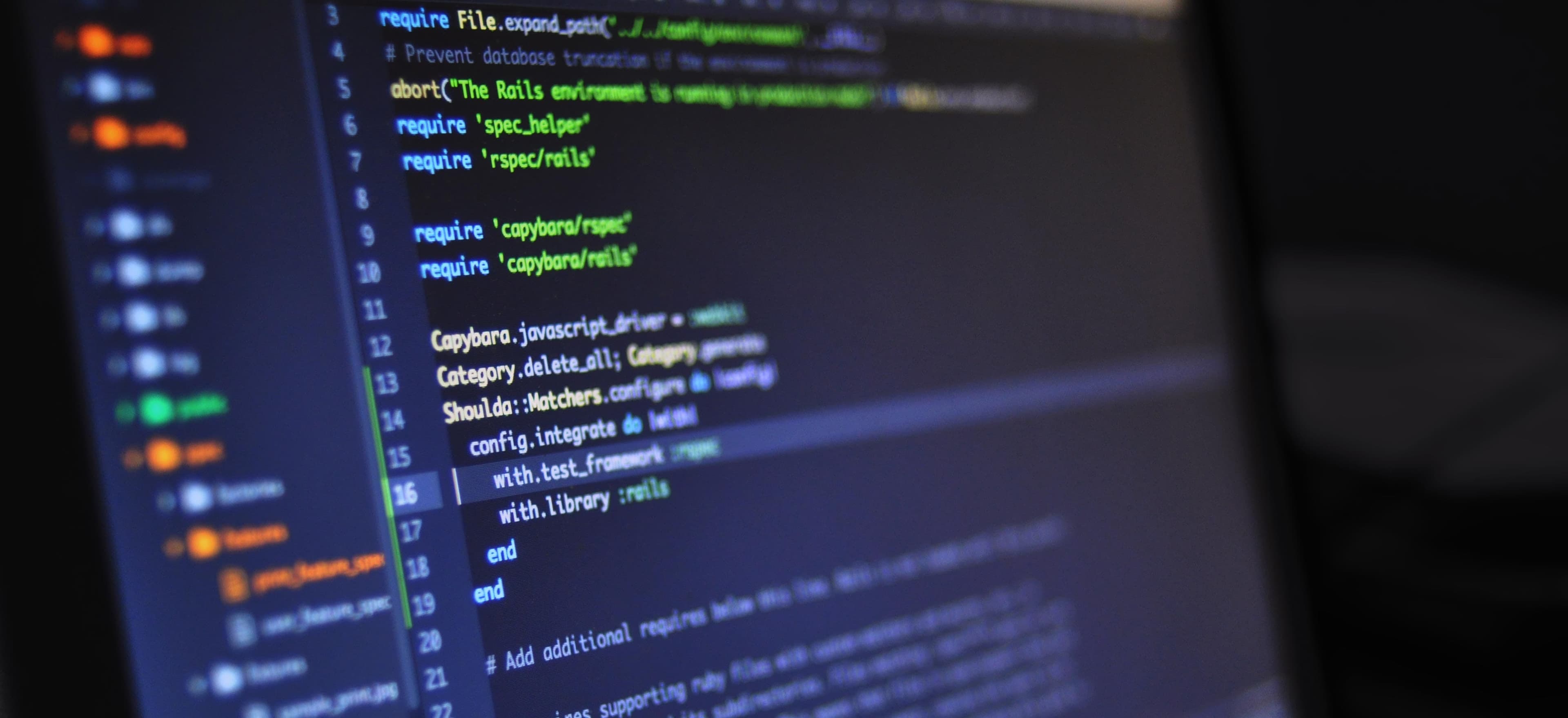
- Published on
Overcoming Common Pitfalls in JAX-RS Context Management
Java API for RESTful Web Services (JAX-RS) enables the development of server-side applications. While its intuitive design offers developers flexibility, it comes with its own set of challenges, particularly around context management. Understanding the context in which your REST services operate is crucial for ensuring performance, scalability, and reliability. In this blog post, we will dissect common pitfalls in JAX-RS context management and illustrate effective strategies to overcome them.
Understanding Context Management in JAX-RS
JAX-RS defines several context types to facilitate operations between client requests and server responses. These contexts include requests, responses, and application-level contexts. Proper management of these contexts is vital for executing clean code and maximizing efficiency.
What is Context in JAX-RS?
A context in JAX-RS refers to the state and data associated with the request and response cycle. JAX-RS provides context types via dependency injection, allowing developers to access crucial aspects such as metadata, resources, and any associated state. Key context types include:
- RequestContext: Information related to the client request.
- ResponseContext: Data pertaining to the service's response.
- Injectable services such as
UriInfo
,SecurityContext
, andApplicationContext
.
Common Pitfalls in JAX-RS Context Management
1. Misunderstanding of the Lifecycle
JAX-RS is built on the Java EE model, where understanding the lifecycle of request and response is critical. A common pitfall is assuming that singleton beans (or objects) will maintain state across requests.
Solution: Whenever possible, use request-scoped or conversation-scoped beans. They exist only as long as they are needed — particularly within the context of handling an HTTP request.
Code Example:
@Singleton
public class MySingletonBean {
// Singleton beans are shared across requests
private String sharedData;
public String serveData() {
return sharedData;
}
}
@RequestScoped
public class MyRequestBean {
private String requestData;
public String serveRequestData() {
return requestData;
}
}
Why use request-scoped beans? In this example, MyRequestBean
offers a clean slate for each HTTP request, whereas MySingletonBean
can induce shared state issues across different requests.
2. Ignoring Thread Safety
With multiple threads handling JAX-RS requests, it's imperative to consider thread safety. Manipulating shared resources without proper synchronization can lead to data corruption and race conditions.
Solution: Always use thread-safe data structures or synchronize access to mutable shared resources.
Code Example:
private final Map<String, String> sharedMap = new ConcurrentHashMap<>();
public void safeUpdate(String key, String value) {
synchronized (this) {
sharedMap.put(key, value);
}
}
Why synchronize? Using ConcurrentHashMap
allows for better performance in multi-threaded environments, but explicit synchronization guarantees thread safety when performing compound actions.
3. Neglecting Exception Handling
Failure to manage exceptions effectively can disrupt service and lead to unexpected behavior. In JAX-RS, unhandled exceptions may propagate and result in internal server errors, confusing both developers and users.
Solution: Implement a global exception handler to ensure all exceptions are handled uniformly and efficiently.
Code Example:
@Provider
public class CustomExceptionMapper implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("Custom error: " + exception.getMessage()).build();
}
}
Why use Custom Exception Mappers? This approach allows you to centralize and customize error-handling logic throughout your JAX-RS application, improving maintainability and user experience.
Leveraging Built-in Contexts
4. Underutilizing Context Interfaces
Many developers overlook the serving of built-in JAX-RS context interfaces such as UriInfo
and SecurityContext
. These elements provide valuable information that can enhance your service's functionality.
Solution: Take advantage of these contexts to optimize routing behavior, enhance security, and adapt your services for various conditions based on incoming requests.
Code Example:
@Context
private UriInfo uriInfo;
@Path("/resource")
public Response getResource() {
String path = uriInfo.getPath(); // Fetch the request path
// Additional logic to handle the request using the path
return Response.ok().entity("Path requested: " + path).build();
}
Why utilize UriInfo
? Using contextual information like UriInfo
allows developers to build RESTful services that are more aware of their HTTP context, improving response handling and enrich user interaction.
5. Failing to Release Resources
Over time, failing to manage resources like database connections and file handles can lead to memory leaks and increased latency, severely impairing your application's performance.
Solution: Properly release resources when they are no longer needed, often achieved through try-with-resources statements or relying on container-managed resources.
Code Example:
@Singleton
public class DatabaseManager {
private Connection connection;
@PostConstruct
public void init() {
// Initialize database connection
}
public void performDatabaseOperation() {
try (PreparedStatement stmt = connection.prepareStatement("SQL_STATEMENT")) {
// Execute operations...
} catch (SQLException e) {
// Handle exceptions
}
}
@PreDestroy
public void cleanup() {
if(connection != null) {
connection.close();
}
}
}
Why use try-with-resources? This pattern ensures PreparedStatement
is automatically closed after use, minimizing resource leaks while executing database operations.
Best Practices for Context Management
6. Use CDI for Better Management
Context and Dependency Injection (CDI) can significantly improve your context management in JAX-RS. By allowing you to engage with lifecycles more effectively, CDI offers cleaner and more maintainable code.
7. Configuration and Properties Management
Centralizing configurations can ease context management. Use properties files for storing external configurations which can be injected as needed.
8. Testing and Documentation
Lastly, always ensure to have comprehensive documentation and unit tests for your service methods. This ensures the durability of context management practices over iterations of code updates.
Bringing It All Together
Context management in JAX-RS applications can pose several challenges, but understanding and employing effective strategies mitigates these pitfalls significantly. By promoting the use of request-scoped resources, prioritizing thread safety, and leveraging built-in context interfaces like UriInfo
and SecurityContext
, developers can create robust JAX-RS applications.
By practicing error-handling strategies through custom exception mappers and ensuring resource management is a priority, you can ensure your application is resilient and efficient. For more insights on JAX-RS and RESTful services, you may want to check JAX-RS Official Documentation, and for best practices on service implementation, consider reviewing RESTful API Design by Matthias Biehl.
By cultivating these practices, you not only avoid common pitfalls but also lead your projects towards long-term success. Happy coding!
Checkout our other articles