Common Gson Pitfalls in Android Development and How to Fix Them
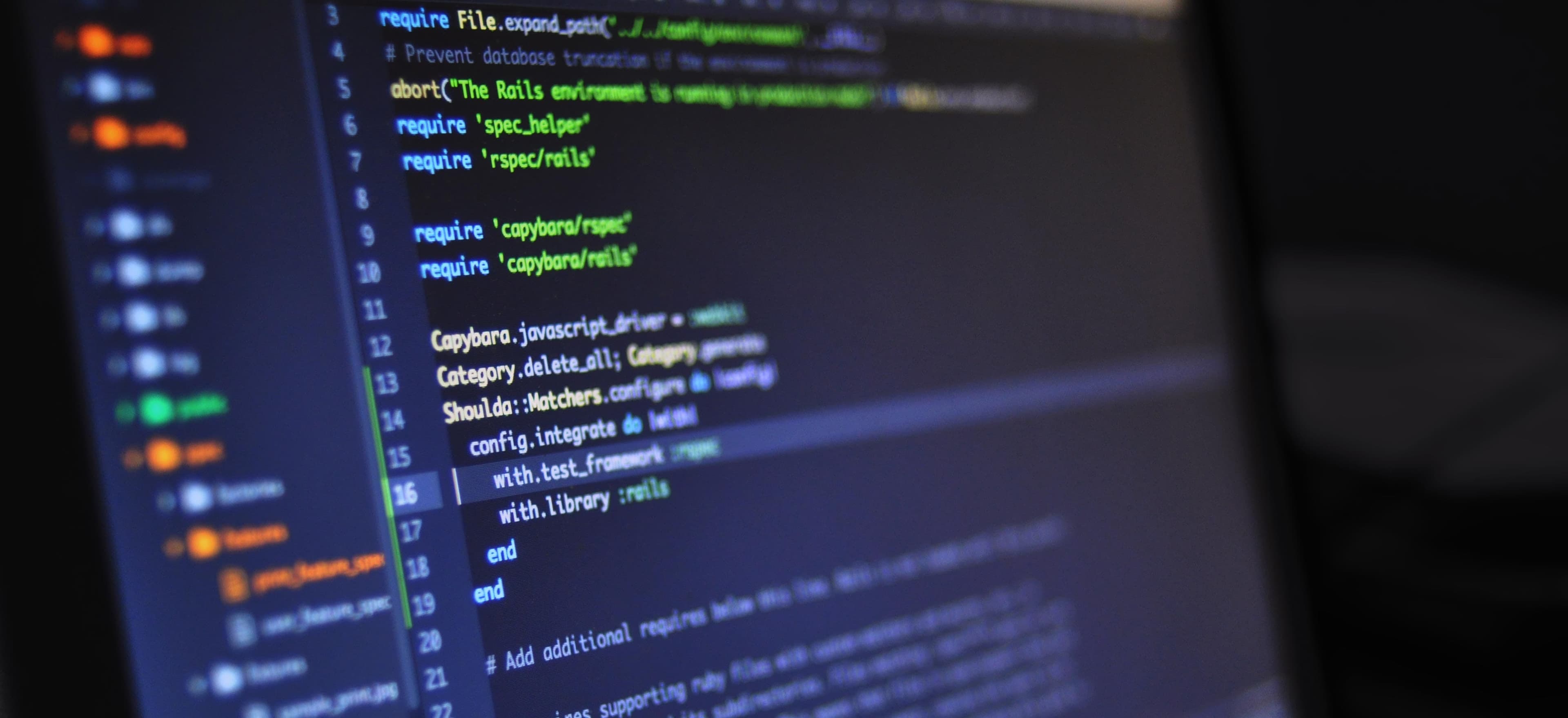
- Published on
Common Gson Pitfalls in Android Development and How to Fix Them
When developing Android applications, JSON parsing is an integral part of managing data communication with web services. Gson, Google's library for converting Java Objects into JSON and vice versa, is a popular choice among developers. However, like any tool, it comes with its own set of common pitfalls. In this blog post, we will explore these pitfalls and how to fix them to make your Android development experience smoother and more efficient.
Table of Contents
Understanding Gson
Before diving into common pitfalls, let’s quickly understand what Gson is and why it's vital for Android development. Gson is a Java library that can be used to convert Java objects to their JSON representation and vice versa. It works seamlessly with objects and arrays and can handle complex data structures with custom rules for serialization and deserialization.
Here’s a simple example of how to convert a Java object to JSON using Gson:
Gson gson = new Gson();
MyClass myObject = new MyClass("Hello, World!", 123);
String jsonString = gson.toJson(myObject);
In this example, MyClass
is converted into a JSON string which can then be sent over the network or stored locally.
Common Pitfalls
1. Using Incorrect Field Names
One of the most straightforward pitfalls is using incorrect field names. JSON is case-sensitive, so any mismatch in the Java field names and the JSON keys could lead to null values.
class User {
String name;
int age;
}
If your JSON looks like this:
{
"Name": "John",
"Age": 30
}
You might end up with null values when converting to the User
class.
2. Improper Handling of Nested Objects
When dealing with nested JSON objects, developers often overlook the necessity of creating corresponding classes.
{
"user": {
"name": "John",
"age": 30
}
}
If the User
class is not nested inside another class, the deserialization will fail.
3. List and Collection Mismanagement
Using arrays or lists can also lead to issues. If you attempt to deserialize a JSON array directly into a standard Java List without proper type specifications, you will encounter problems.
{
"users": [
{
"name": "John",
"age": 30
},
{
"name": "Doe",
"age": 25
}
]
}
Mismanaging the conversion could result in a class cast exception.
4. Using Wrong Custom Deserializers
Gson allows for custom deserializers, but using them incorrectly can lead to more complex issues. Incorrect handling of your custom logic can cause exceptions or incorrect data.
class CustomDeserializer implements JsonDeserializer<MyClass> {
@Override
public MyClass deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) {
// custom deserialization logic
}
}
If your logic doesn’t align with the JSON structure, it may lead to runtime exceptions.
How to Fix Common Pitfalls
1. Ensure Field Name Matching
The most effective way to ensure your JSON keys and Java field names align is through annotations:
class User {
@SerializedName("Name")
String name;
@SerializedName("Age")
int age;
}
By using @SerializedName
, you can map JSON keys with different casing or naming to your Java fields, eliminating potential null values.
2. Create Appropriate Classes for Nested Objects
Always create corresponding classes for nested JSON structures. Here’s how you can handle our earlier user
JSON structure:
class User {
String name;
int age;
}
class Response {
User user;
}
This ensures proper deserialization of the nested object.
3. Manage Lists Properly
When deserializing lists, ensure you leverage Generic types:
Type userListType = new TypeToken<List<User>>() {}.getType();
List<User> userList = gson.fromJson(jsonString, userListType);
By defining TypeToken
, Gson can accurately interpret the JSON array and convert it into a list of User
objects.
4. Use Custom Deserialization Wisely
When utilizing custom deserializers, ensure your logic matches the JSON structure you expect. Here’s an example:
class CustomDeserializer implements JsonDeserializer<MyClass> {
@Override
public MyClass deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) {
JsonObject jsonObject = json.getAsJsonObject();
return new MyClass(jsonObject.get("name").getAsString(), jsonObject.get("age").getAsInt());
}
}
In this example, we are converting the JSON data into the required object safely.
My Closing Thoughts on the Matter
Gson is an incredibly valuable tool in Android development with powerful capabilities for handling JSON. However, as highlighted, it comes with its pitfalls. By being mindful of field name mismatches, nested object requirements, proper list management, and the application of custom deserializers, developers can avoid these common traps.
For more in-depth knowledge about Gson, you may check out the official Gson documentation or learn more about its performance optimizations.
Navigating these challenges may require some effort, but with proper understanding and careful implementation, you can ensure smooth data handling in your Android applications. Happy coding!
Checkout our other articles