Debugging Java: Tackling the Challenges of Mutation Testing
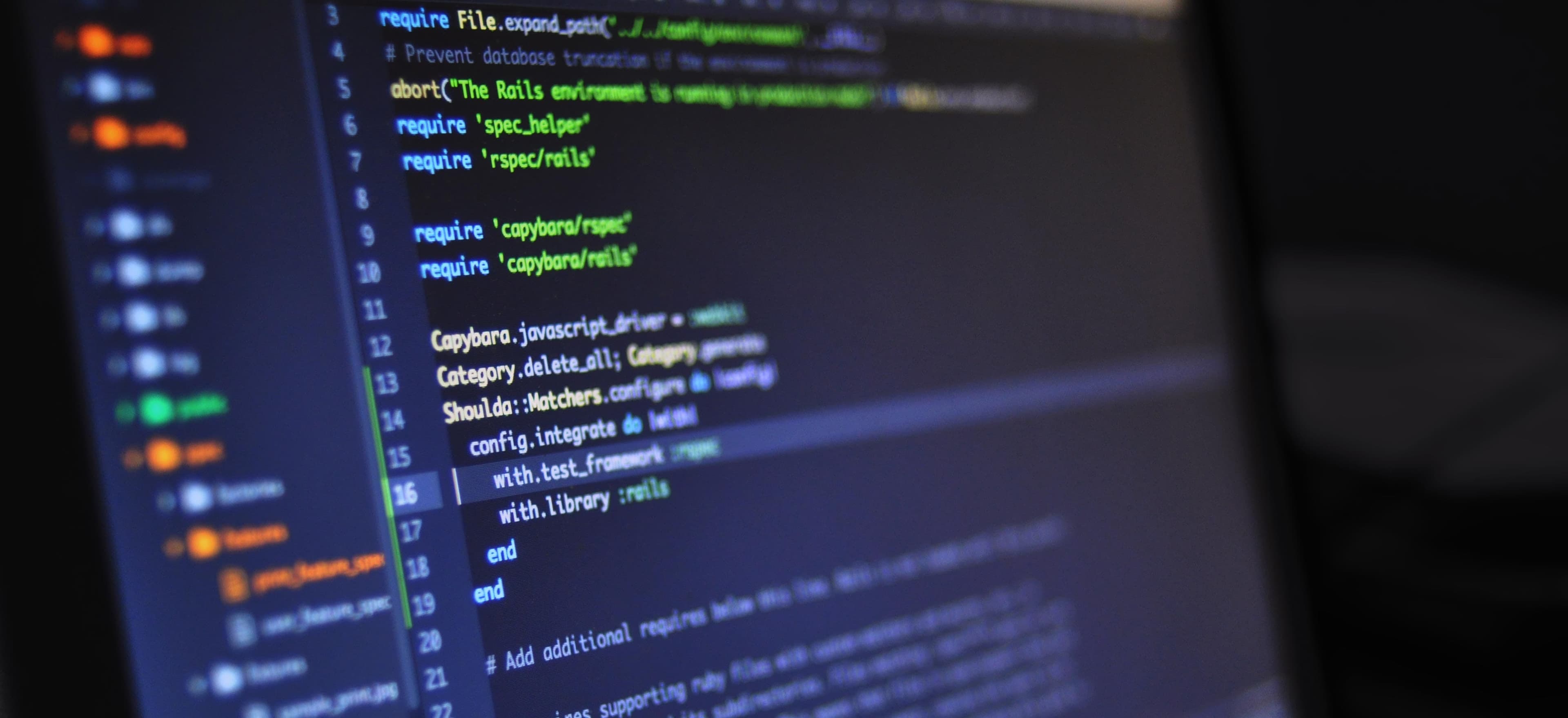
- Published on
Debugging Java: Tackling the Challenges of Mutation Testing
Mutation testing is an advanced technique used primarily for evaluating test suites in Java and other programming languages. It is an essential part of software development, especially in creating robust, reliable, and maintainable applications. However, debugging in the context of mutation testing presents unique challenges. In this blog post, we will explore the ins and outs of mutation testing, how to effectively debug it, and best practices for leveraging this powerful testing technique in Java.
Understanding Mutation Testing
What is Mutation Testing?
Mutation testing involves purposely introducing small changes (mutations) to a program's source code to assess the effectiveness of test cases. The goal is to ensure that existing tests can detect these mutations, thereby confirming that the tests are effective and cover various scenarios.
Why Use Mutation Testing?
- Improves Test Quality: Mutation tests identify dead tests—those that do not effectively check the program's outcomes.
- Encourages Better Testing Practices: Developers are prompted to think critically about their tests, potentially leading to more comprehensive test cases.
- Enhances Code Coverage: While traditional coverage metrics can be misleading, mutation testing provides a more reliable indication of test suite effectiveness.
The Challenges of Mutation Testing
Despite its benefits, mutation testing comes with challenges.
1. Performance Issues
Mutation testing can be resource-intensive. When you mutate a program, the entire suite of tests has to run repeatedly for each mutation. This can lead to long execution times.
2. Complexity in Mutation Operators
Choosing the right mutation operators is crucial. Each operator modifies the code in a specific way. Understanding these operators and how they affect program behavior is essential for effective debugging.
3. False Positives and Negatives
A well-written test suite can show “survivor” mutants (mutations that the tests failed to detect) that are not necessarily indicative of test suite inadequacy. This can lead to confusion and misinterpretation of results.
4. Debugging Introduced Mutations
Debugging can become complicated when you have to deal with the original code and mutated versions simultaneously. Understanding why a particular test failed requires a careful analysis of both versions.
Techniques for Successful Mutation Testing in Java
1. Select Appropriate Tools
Various tools facilitate mutation testing in Java, including:
- PIT (PIT Mutation Testing): A popular choice that integrates well with existing testing ecosystems. It provides a comprehensive report on mutation results.
- JMutate: An older tool, but still useful for educational purposes.
Tool selection is essential for effective mutation testing, as it greatly affects the ease of debugging.
2. Use Incremental Mutation Testing
Instead of applying mutations to the entire codebase at once, focus on incrementally mutating specific modules or methods. This approach will reduce execution time and make debugging more manageable.
3. Focus on High-Risk Areas
Start by focusing your mutation tests on high-risk areas of your codebase—modules that are set to change frequently or those with complex logic. This targeted approach saves time and maximizes the effectiveness of your tests.
4. Analyze Mutation Reports
Tools like PIT provide detailed mutation reports that highlight which tests detected mutations and which did not. Pay attention to these reports to understand your test suite's weaknesses.
5. Debugging Techniques
When you encounter mutations that are not caught by your tests, take the following debugging steps:
- Trace: Use Java's built-in debugging tools to step through code execution and understand how inputs are processed.
- Log: Introduce logging to track variable states at crucial points in execution. This helps illuminate where your tests may be falling short.
Example of Mutation Testing in Java
Here’s a simple Java method and how you'd set up a basic mutation test.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Mutation Operator: Replace the '+' operator with a '-', creating a mutant:
public int add(int a, int b) {
return a - b; // Introduction of mutation
}
Running Tests:
You might have a test defined like this:
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
private Calculator calculator = new Calculator();
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3)); // This test should catch the mutant
}
}
Why: If the add
method has been mutated to use subtraction, then this test should fail. If it passes, you must revise your test to improve its coverage and effectiveness.
Best Practices for Mutation Testing
- Combine Mutation Testing with Other Techniques: Complement mutation testing with traditional unit tests and code reviews for a more robust testing strategy.
- Educate Your Team: Ensure everyone involved in writing tests understands the goals and complexities of mutation testing.
- Refactor Tests Regularly: As your application evolves, regularly revisit and refactor your tests to keep them relevant and effective.
Final Considerations
Mutation testing is an invaluable strategy for ensuring the robustness of your Java applications. Although it brings challenges, implementing the right techniques can greatly enhance your debugging efforts and application reliability.
By understanding the fundamentals of mutation testing, selecting appropriate tools, and employing smart debugging strategies, you can create a more reliable and efficient testing ecosystem. Remember, the goal is not just to catch mutations but to improve your overall testing practices and deliver high-quality software.
For further insights into mutation testing, you can check out the official [PIT documentation](https://pitest.org/)
or explore the concept of [test-driven development](https://en.wikipedia.org/wiki/Test-driven_development)
to complement your mutation testing approach.
Embrace the challenge of mutation testing, and you will significantly enhance your code's resilience against future changes and bugs. Happy coding!
Checkout our other articles