Debugging Java Apps: Top Tools for Common Pitfalls
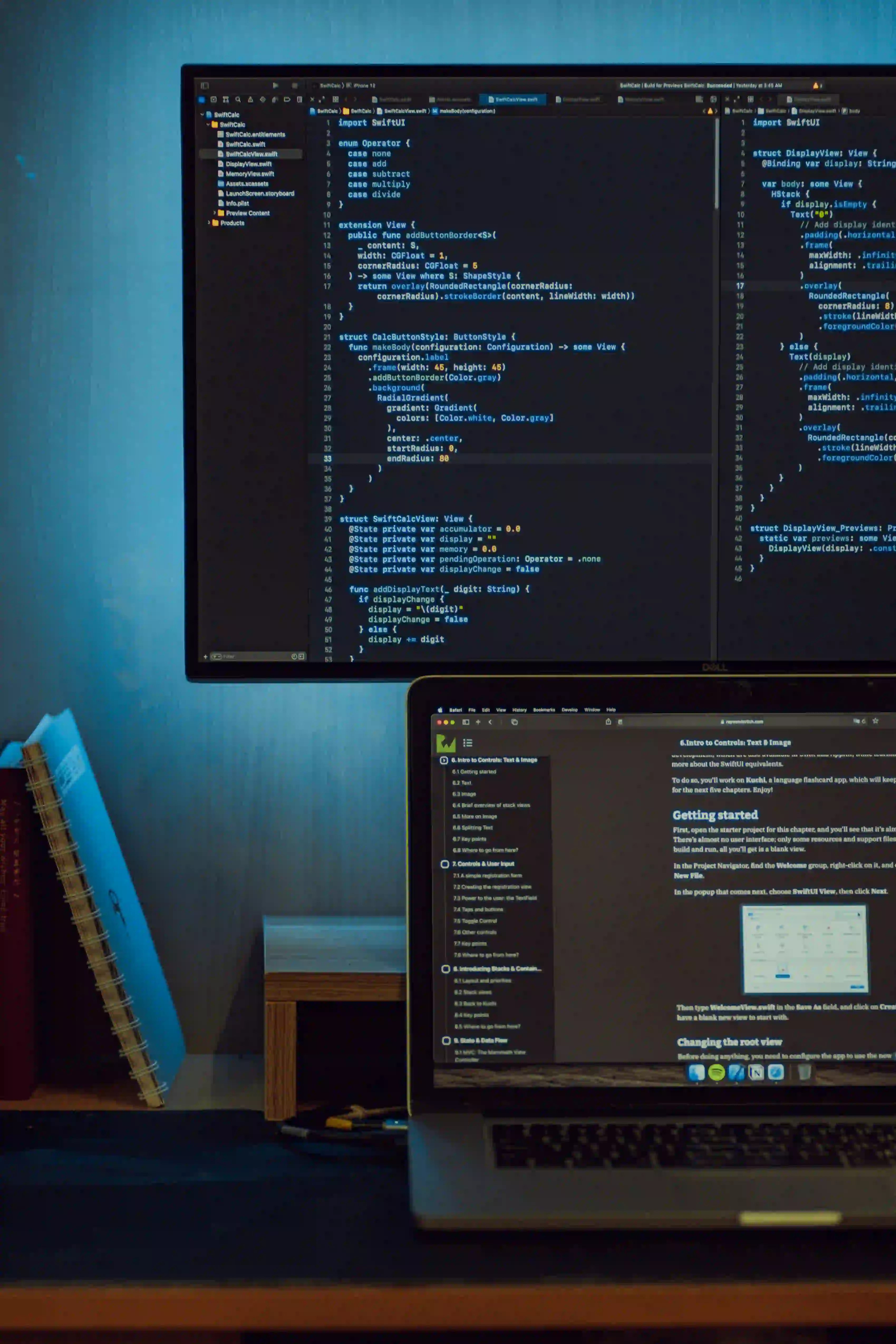
Debugging Java Apps: Top Tools for Common Pitfalls
Debugging is a crucial part of software development, especially when working with Java applications. Whether you’re a seasoned developer or a newbie, knowing how to effectively debug your code will save you time and frustration. In this blog post, we will explore some of the best tools and practices for debugging Java applications, alongside common pitfalls you may encounter.
Understanding Debugging in Java
Before diving into tools, it’s important to understand what debugging actually involves. Debugging is a systematic process of detecting and removing errors, bugs, and issues from your application. In Java, where the underlying architecture involves both the Java Virtual Machine (JVM) and the language's rich standard library, this can be a challenging task.
Common Java Pitfalls
-
Null Pointer Exceptions: This is one of the most common and frustrating errors you'll face. It occurs when trying to access an object that is null.
-
ArrayIndexOutOfBoundsExceptions: Occurs when trying to access an index that is outside the bounds of an array.
-
Concurrency Issues: Multithreading can lead to subtle bugs like race conditions and deadlocks.
-
ClassCastExceptions: Happens when trying to cast an object to a subclass of which it is not an instance.
Top Debugging Tools for Java
Let's explore some of the most effective tools for debugging Java applications.
1. IntelliJ IDEA Debugger
Introduction
IntelliJ IDEA is one of the most popular Integrated Development Environments (IDEs) for Java development. It features a built-in debugger that helps to run the application step by step, inspect variables, set breakpoints, and evaluate expressions.
How to Use
-
Setting Breakpoints: You can set breakpoints in your code by clicking in the gutter next to the line numbers.
-
Running in Debug Mode: Run your application in debug mode, and it will stop at your breakpoints.
-
Inspect Variables: Hover over variables to see their current values, or use the 'Variables' panel to view all variables in the current scope.
Why Choose IntelliJ?
IntelliJ’s intelligent code analysis helps you find potential bugs before you even run the program. It allows for a smooth transition between writing code and debugging it.
public class NullPointerExample {
public static void main(String[] args) {
String str = null;
System.out.println(str.length()); // This will throw NullPointerException
}
}
In the code above, if you run it, you'll encounter a NullPointerException
.
2. Eclipse Debugger
Introduction
Eclipse is another widely-used IDE, known for its rich plugin ecosystem. The Eclipse debugger offers similar functionality to IntelliJ, allowing you to debug applications efficiently.
How to Use
-
Breakpoint Management: Just like in IntelliJ, you can set breakpoints by double-clicking on the left margin.
-
Step Over/Into/Out: These options in the toolbar allow you to navigate through the code effectively.
Why Choose Eclipse?
Many developers prefer Eclipse due to its extensive libraries and plugins. It can be highly customized to fit your workflow.
public class ArrayIndexOutOfBoundsExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]); // This will throw ArrayIndexOutOfBoundsException
}
}
Running this code will result in an ArrayIndexOutOfBoundsException
, helping you realize the importance of checking array lengths before accessing.
3. JDB (Java Debugger)
Introduction
JDB is a command-line debugger for Java programs. Although it may not have the graphical interface that IDEs offer, it’s a powerful tool for debugging Java applications.
How to Use
-
Run with JDB: Compile your Java files and run JDB with the command
jdb MyProgram
. -
Using Breakpoints: You can implement breakpoints in JDB with the command
stop at ClassName:lineNumber
.
Why Choose JDB?
JDB is lightweight and can be used in environments where IDEs are not available. It’s also scriptable, providing greater flexibility.
public class ConcurrencyExample {
private static int counter = 0;
public static void main(String[] args) {
Runnable task = () -> {
for (int i = 0; i < 1000; i++) {
counter++;
}
};
Thread thread1 = new Thread(task);
Thread thread2 = new Thread(task);
thread1.start();
thread2.start();
}
}
This code demonstrates a classic concurrency issue where two threads manipulate a shared variable. Debugging this can help you uncover race conditions.
4. VisualVM
Introduction
VisualVM is a monitoring, troubleshooting, and profiling tool for Java applications. It provides a visual interface that allows developers to monitor performance, memory usage, and thread activity.
How to Use
-
Monitoring Memory: Track memory usage over time to identify leaks.
-
Thread Analysis: View the state of threads and analyze the stack traces for deadlocks.
Why Choose VisualVM?
VisualVM is incredibly helpful for performance tuning. It helps you identify bottlenecks in your application.
5. Logging Frameworks
Logging is an essential aspect of debugging. Using proper logging can help you identify issues without stepping through code.
Apache Log4j
How to Use
- Add Dependency: Include Log4j in your project.
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
- Log Messages: Use
Logger
to log messages at various severity levels.
import org.apache.log4j.Logger;
public class LoggingExample {
private static final Logger logger = Logger.getLogger(LoggingExample.class);
public static void main(String[] args) {
logger.info("Application started");
logger.warn("This is a warning");
}
}
Logging allows you to trace what’s happening in your application, making it easier to identify issues.
Tips for Effective Debugging
-
Reproduce the Error: Always try to reproduce the error consistently before debugging it.
-
Use Version Control: This allows you to track changes and understand what could have caused a bug.
-
Incremental Testing: Break your code into smaller, testable parts. It’s easier to find a bug in a small piece of code than in a large one.
-
Pair Programming: Sometimes, having another set of eyes on your code can help identify issues you might’ve overlooked.
-
Stay Updated: Keep learning about new tools and techniques. The Java ecosystem is always evolving, and so should your skill set.
Closing the Chapter
Debugging is an essential skill in a developer's toolkit, especially in Java programming. With the right tools and practices, you can confidently navigate through errors and improve the reliability of your applications. Embrace tools like IntelliJ IDEA, Eclipse, JDB, and VisualVM, and leverage logging frameworks to streamline your debugging process.
Keep experimenting and refining your debugging strategies—over time, you'll become more adept at identifying and fixing issues quickly.
For further reading, check out Java Debugging Basics and Best Practices for Java Logging.
Happy debugging!