Mastering Messaging: Overcoming Streaming Data Challenges
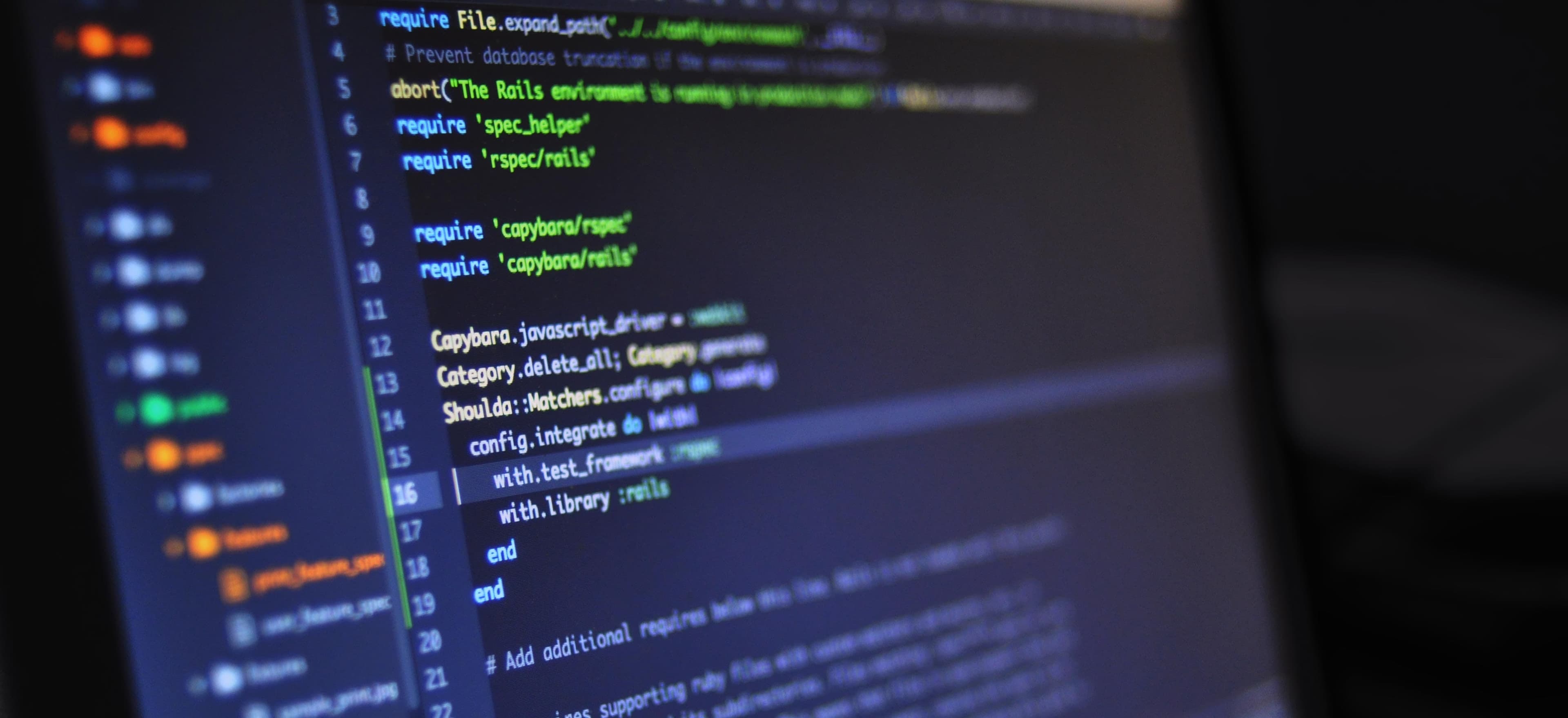
- Published on
Mastering Messaging: Overcoming Streaming Data Challenges
The rapid rise of data streaming technology has revolutionized the way businesses operate. From real-time analytics to responsive application designs, streaming data is at the heart of modern decision-making. However, along with its advantages comes a set of challenges that developers must master to fully leverage its potential. In this blog post, we'll explore common streaming data challenges and how to overcome them, specifically focusing on messaging systems in Java.
What is Streaming Data?
Streaming data refers to continuous flows of data generated from various sources, such as sensors, logs, or user interactions, that need to be processed in real time. Unlike batch processing, which handles data at rest, streaming data enables the immediate action or analytics on incoming data.
The Importance of Messaging
At the core of streaming data architectures lies messaging systems. These platforms facilitate the transfer of data between producers and consumers, making it easier to process and react to events as they happen. Java, a popular programming language, offers a robust ecosystem for managing messaging. Kafka, RabbitMQ, and ActiveMQ are just a few examples of messaging systems often used with Java.
Challenges of Streaming Data
As much as streaming data empowers real-time capabilities, several challenges can arise. Here’s a closer look at some of these obstacles and ways to tackle them.
1. High Volume of Data
The Challenge
One of the biggest challenges in streaming data is managing the sheer volume of data generated every second. Systems can become overwhelmed, affecting processing speed and data integrity.
Solution
To tackle high data volume, implement:
- Partitioning & Sharding: Distributing data across multiple processing units or physical storage can ensure no single resource becomes a bottleneck.
- Backpressure: Use backpressure mechanisms to regulate the flow of data based on the consumer's ability to process it.
Example in Java
Here’s how to implement backpressure in a Kafka producer:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import java.util.Properties;
public class BackpressureExample {
private final KafkaProducer<String, String> producer;
public BackpressureExample(Properties properties) {
this.producer = new KafkaProducer<>(properties);
}
public void send(String topic, String message) {
try {
producer.send(new ProducerRecord<>(topic, message), (metadata, exception) -> {
if (exception != null) {
// Handle exception (e.g., log it or retry)
} else {
// Record sent successfully
}
});
} catch (Exception e) {
// Apply backpressure logic, e.g. sleep for a while before retrying
try {
Thread.sleep(100); // wait for 100ms before retry
} catch (InterruptedException interrupted) {
Thread.currentThread().interrupt();
}
}
}
}
2. Data Latency
The Challenge
Another significant challenge is latency, which affects the speed of data delivery. It can arise from network delays, processing bottlenecks, or inefficient data architectures.
Solution
To reduce latency, consider:
- Optimizing Serialization: Use lightweight serialization formats like Avro or Protocol Buffers to reduce the size and processing time.
- In-memory Data Processing: Leverage in-memory databases or data grids like Apache Ignite or Hazelcast for real-time processing.
3. Data Consistency
The Challenge
Ensuring consistency in a distributed streaming system can be complex. Data may arrive out of order, or there may be duplicates or missing messages.
Solution
Implementing exactly-once processing and strong coordination between producers and consumers can help. Tools like Kafka Streams support exactly-once semantics.
Example in Java
A simplified example of using Kafka Streams for exactly-once processing:
import org.apache.kafka.streams.KafkaStreams;
import org.apache.kafka.streams.StreamsBuilder;
import org.apache.kafka.streams.StreamsConfig;
import java.util.Properties;
public class ExactlyOnceExample {
public static void main(String[] args) {
Properties props = new Properties();
props.put(StreamsConfig.APPLICATION_ID_CONFIG, "exactly-once-application");
props.put(StreamsConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
props.put(StreamsConfig.PROCESSING_GUarantee_CONFIG, StreamsConfig.EXACTLY_ONCE);
StreamsBuilder builder = new StreamsBuilder();
// Define your stream processing topology here
KafkaStreams streams = new KafkaStreams(builder.build(), props);
streams.start();
}
}
4. Scalability
The Challenge
As data volumes grow, systems must scale to accommodate increased loads. Designing for scalability is essential and can become tricky.
Solution
- Horizontal Scaling: Scale the number of consumer instances based on the load. Messaging systems like Kafka already support consumer group scaling.
- Auto-scaling: Use orchestration tools like Kubernetes to automatically manage resource allocation as demand grows.
5. Monitoring and Debugging
The Challenge
Monitoring streaming applications can be soft-spoken compared to traditional applications because issues may need to be identified in real-time.
Solution
Integrating monitoring tools can greatly enhance observability. Solutions include:
- Distributed Tracing Tools: Tools like OpenTelemetry can help you understand performance issues in real time.
- Logging: Use structured logging to ensure logs provide necessary context, making it easier to debug issues.
A Final Look
Streaming data has the power to transform how we interact with information. However, it comes with its set of challenges, from managing data volume to ensuring consistency. Java's robust ecosystem offers numerous tools and libraries to help developers overcome these challenges effectively.
By implementing strategies such as partitioning data, optimizing serialization, and using modern monitoring tools, you can enhance your messaging systems and ensure your applications are truly responsive to real-time data streams.
For further reading on streaming technologies, consider these resources:
Mastering these concepts not only improves your data processing capabilities but also places your organization at the forefront of harnessing the power of streaming data. As you venture further into this dynamic realm, keep innovating and pushing the boundaries. Your real-time data strategy will dictate your success.
Checkout our other articles