Mastering EDT: Troubleshooting invokeAndBlock Challenges
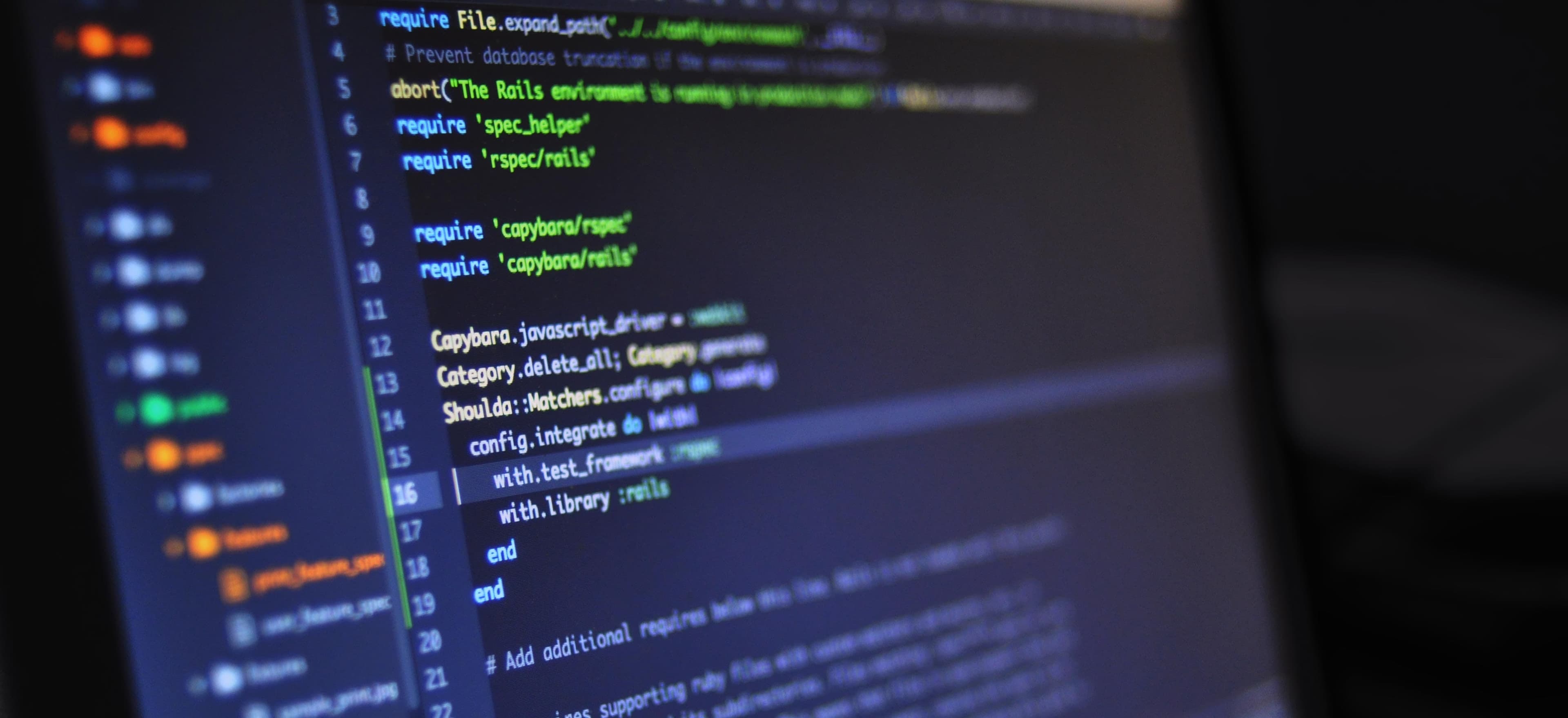
- Published on
Mastering EDT: Troubleshooting invokeAndBlock Challenges in Java Swing
Java's Swing framework has been a mainstay for creating rich desktop user interfaces. At the heart of Swing's multithreaded designs is the concept of the Event Dispatch Thread (EDT). However, developers often face challenges when trying to manipulate GUI components from threads other than the EDT. This blog aims to demystify the invokeAndBlock
challenges and provide practical strategies for efficient GUI programming in Java Swing.
Understanding the Event Dispatch Thread (EDT)
The Event Dispatch Thread (EDT) is a special thread in Java Swing responsible for handling all the events and updating the GUI components. This design ensures that the user interface remains responsive and performs better by managing events in a single thread without the risk of multithreading issues like deadlocks or races on GUI state.
Why Use EDT?
- Responsiveness: Operations that take a lot of time can block the user interface. By processing events on the EDT, the UI remains responsive to user actions.
- Thread Safety: Swing components cannot be safely accessed from outside the EDT. Accessing UI elements from other threads can lead to unpredictable behavior.
The Challenges of Using invokeAndBlock
The invokeAndBlock
method is designed to allow background threads to execute code on the EDT while blocking the calling thread until the action is complete. However, there are pitfalls associated with its use that can lead to a deadlocked application if not handled properly.
Here’s a simple illustration:
// A simple runnable to be executed on the EDT
Runnable updateUI = new Runnable() {
public void run() {
// Update the UI component here
myLabel.setText("Updated from background thread");
}
};
// Imagine this being executed in a worker thread
SwingUtilities.invokeAndWait(updateUI);
The example above submits an update to the myLabel
UI component. However, if the invokeAndWait
is called from a callback that is already running on the EDT, it can lead to a deadlock.
Common Pitfalls
- Calling from the EDT: If
invokeAndWait
is called from code that is already running on the EDT, it will block indefinitely, leading to deadlock. - Long-Running Tasks: If the task being run on the EDT is lengthy, it will freeze the UI while waiting for the task to complete.
- Unhandled Exceptions: If any exception is thrown during the execution of the Runnable, it may terminate the EDT.
Practical Solutions to Avoid Deadlocks
1. Use invokeLater
Instead of invokeAndWait
If you do not need to block the calling thread and can execute code asynchronously, using invokeLater
is a better choice. It schedules the Runnable for execution on the EDT without blocking the calling thread.
// A simple runnable to be executed on the EDT
Runnable updateUI = new Runnable() {
public void run() {
// Update the UI component here
myLabel.setText("Updated immediately from background thread");
}
};
// Invokes the runnable without blocking
SwingUtilities.invokeLater(updateUI);
2. Background Processing with SwingWorker
For long-running tasks, consider using SwingWorker
. This class allows you to run background tasks and safely update your UI in an efficient way without blocking the EDT.
SwingWorker<Void, String> worker = new SwingWorker<Void, String>() {
@Override
protected Void doInBackground() throws Exception {
// Perform long-running background operations
for (int i = 0; i < 10; i++) {
// Simulate work
Thread.sleep(1000);
publish("Count: " + i);
}
return null;
}
@Override
protected void process(List<String> chunks) {
// Update GUI components
for (String text : chunks) {
myLabel.setText(text);
}
}
@Override
protected void done() {
// Finish work, update final states
myLabel.setText("Done!");
}
};
// Start the SwingWorker
worker.execute();
In this example, the doInBackground
method runs the long task, while the process
method safely updates the UI at intervals.
3. Exception Handling
Always handle exceptions when using invokeAndWait
and invokeLater
. Consider catching exceptions in your Runnable to avoid leaving the EDT in an unexpected state.
Runnable updateUI = new Runnable() {
public void run() {
try {
myLabel.setText("Updating from background thread");
} catch (Exception e) {
e.printStackTrace(); // Handle exception appropriately
}
}
};
SwingUtilities.invokeLater(updateUI);
Wrapping Up
Mastering the Event Dispatch Thread (EDT) is crucial for effective Java GUI programming. By knowing when to use invokeAndBlock
versus invokeLater
, you can prevent deadlocks and ensure your Swing applications remain responsive. Embrace the power of SwingWorker
for managing background tasks efficiently and never forget to incorporate proper exception handling to maintain the integrity of your applications.
For further reading on Swing’s threading model, check out the official documentation here.
Understanding and following these guidelines will enhance your programming skill in Java and allow you to create smoother and more effective GUI applications. Happy coding!