Eliminate BOM Characters for Clean File Formatting
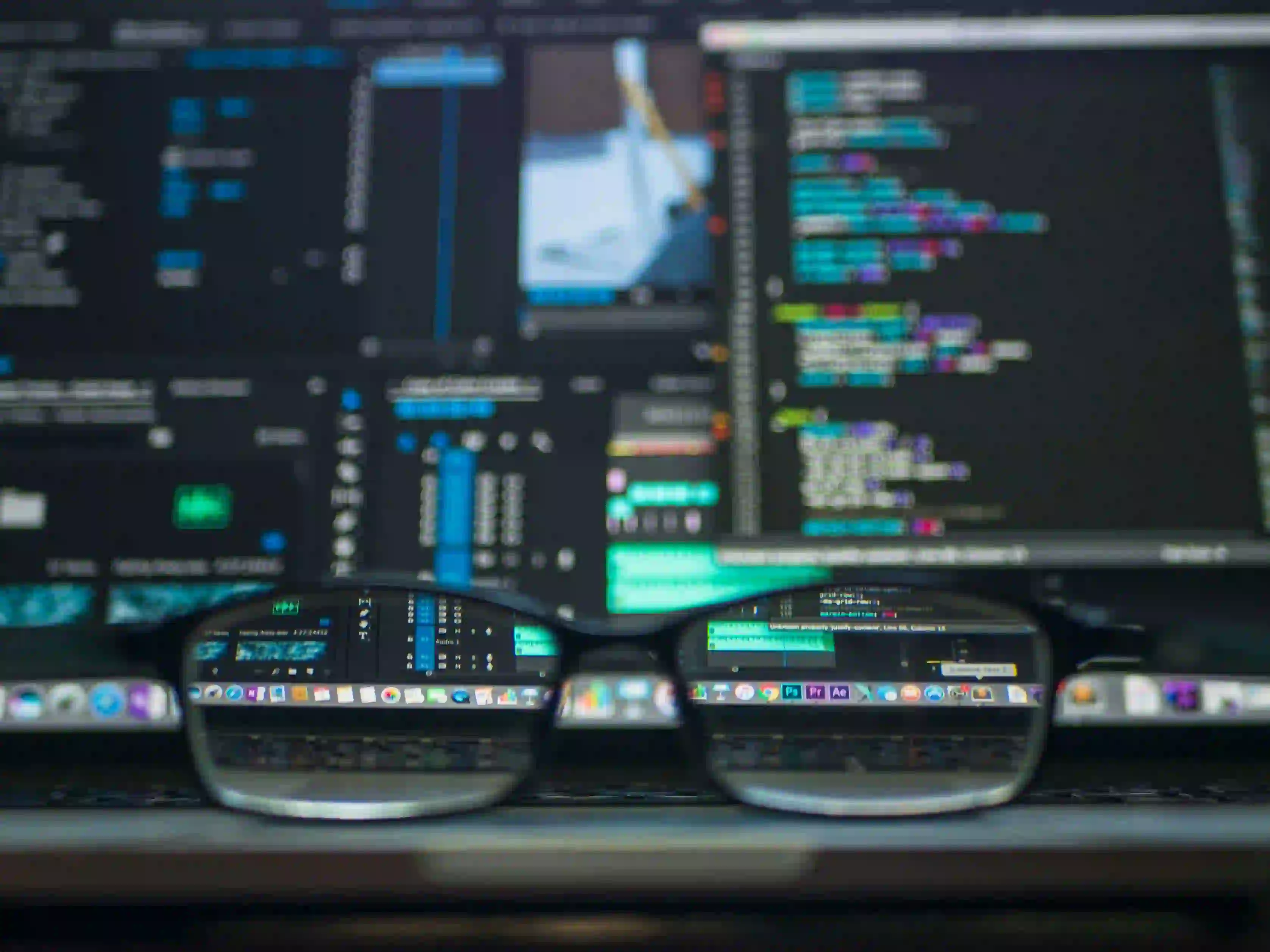
Eliminate BOM Characters for Clean File Formatting in Java
When dealing with text files in Java, particularly those containing Unicode characters, you may encounter Byte Order Mark (BOM) characters. These invisible characters can cause issues such as incorrect file reading, unexpected formatting in outputs, and challenges in text file comparisons. In this blog post, we’ll explore what BOM characters are, how they can affect your Java applications, and provide code examples to effectively eliminate them for cleaner file formatting.
What is a BOM Character?
The Byte Order Mark (BOM) is a Unicode character (U+FEFF) used to denote the endianness of a text file or stream. It is primarily found in UTF-16 and UTF-32 encoded files, but UTF-8 can also incorporate it. While it serves a purpose in determining byte order, it can lead to unintended consequences in text processing.
Issues Caused by BOM Characters
- Parsing Errors: When reading a BOM-laden file, programs may introduce unexpected characters in their output.
- Comparative Inconsistencies: Two seemingly identical strings may differ because one contains a BOM.
- Data Storage Complications: Saving data generated from a file with BOM may lead to corruptions in data processing systems expecting a clean input.
Detecting BOM in Files
Before we can eliminate BOM characters, we need to detect whether they exist in a file. Typically, a BOM will appear at the beginning of the file. Here’s a short Java method utilizing InputStream
to check for BOM:
import java.io.FileInputStream;
import java.io.IOException;
public class BOMDetector {
public static boolean hasBOM(String filePath) throws IOException {
try (FileInputStream fis = new FileInputStream(filePath)) {
byte[] bom = new byte[3];
fis.read(bom);
// Check for UTF-8 BOM
return (bom[0] == (byte) 0xEF && bom[1] == (byte) 0xBB && bom[2] == (byte) 0xBF);
}
}
public static void main(String[] args) {
try {
String filePath = "path/to/your/file.txt";
if (hasBOM(filePath)) {
System.out.println("File contains BOM.");
} else {
System.out.println("File does not contain BOM.");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Code Explanation
- FileInputStream: We use this to read the file as a stream of bytes.
- Byte Array: A byte array is created to read the first three bytes, allowing us to compare them to the expected BOM values.
- Condition Check: If the first three bytes match the UTF-8 BOM, we return true.
Removing BOM Characters from Files
Once a BOM character is detected, we can remove it by rewriting the file without the BOM. Here’s how to do that in Java:
import java.io.*;
public class BOMRemover {
public static void removeBOMFromFile(String filePath) throws IOException {
File file = new File(filePath);
String newFilePath = file.getAbsolutePath() + ".clean";
try (BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(file), "UTF-8"));
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(newFilePath), "UTF-8"))) {
String line;
// Read and write the file line by line
reader.mark(3);
if (reader.read() == -1) {
return; // Empty file
}
reader.reset(); // Go back to the start
int firstByte = reader.read();
int secondByte = reader.read();
int thirdByte = reader.read();
if (!(firstByte == 0xEF && secondByte == 0xBB && thirdByte == 0xBF)) {
writer.write((char)firstByte);
}
// Write the rest of the file
while ((line = reader.readLine()) != null) {
writer.write(line);
writer.newLine();
}
}
System.out.println("BOM removed. Clean file created at: " + newFilePath);
}
public static void main(String[] args) {
try {
String filePath = "path/to/your/file.txt";
removeBOMFromFile(filePath);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Code Explanation
- Input and Output Stream:
BufferedReader
andBufferedWriter
are used to read and write the file efficiently. - Encoding: We specify "UTF-8" to handle the text correctly.
- Mark and Reset Method:
- mark(3): Mark the current position before reading 3 bytes.
- reset(): Go back to the last marked position for further checks.
- Byte Comparison: After checking for the BOM, the code only writes non-BOM first bytes and continues to write the rest of the lines.
A Final Look
Removing BOM characters is essential for maintaining clean file formatting, especially in applications designed for text processing. BOM characters can lead to a series of issues down the line, from errors in file parsing to complications in data storage. By using Java's file handling capabilities, we can efficiently detect and eliminate BOM characters, ensuring our applications behave predictably.
For more information on handling files in Java, check out the Java File I/O documentation. If you encounter issues related to character encoding, the Java Character Encoding guide will be a helpful resource.
By following the approaches outlined above, you can guarantee cleaner, BOM-free text files that are ready for straightforward processing in any application. Happy coding!