Solving Common Pitfalls in JUnit 5 Parameterized Tests
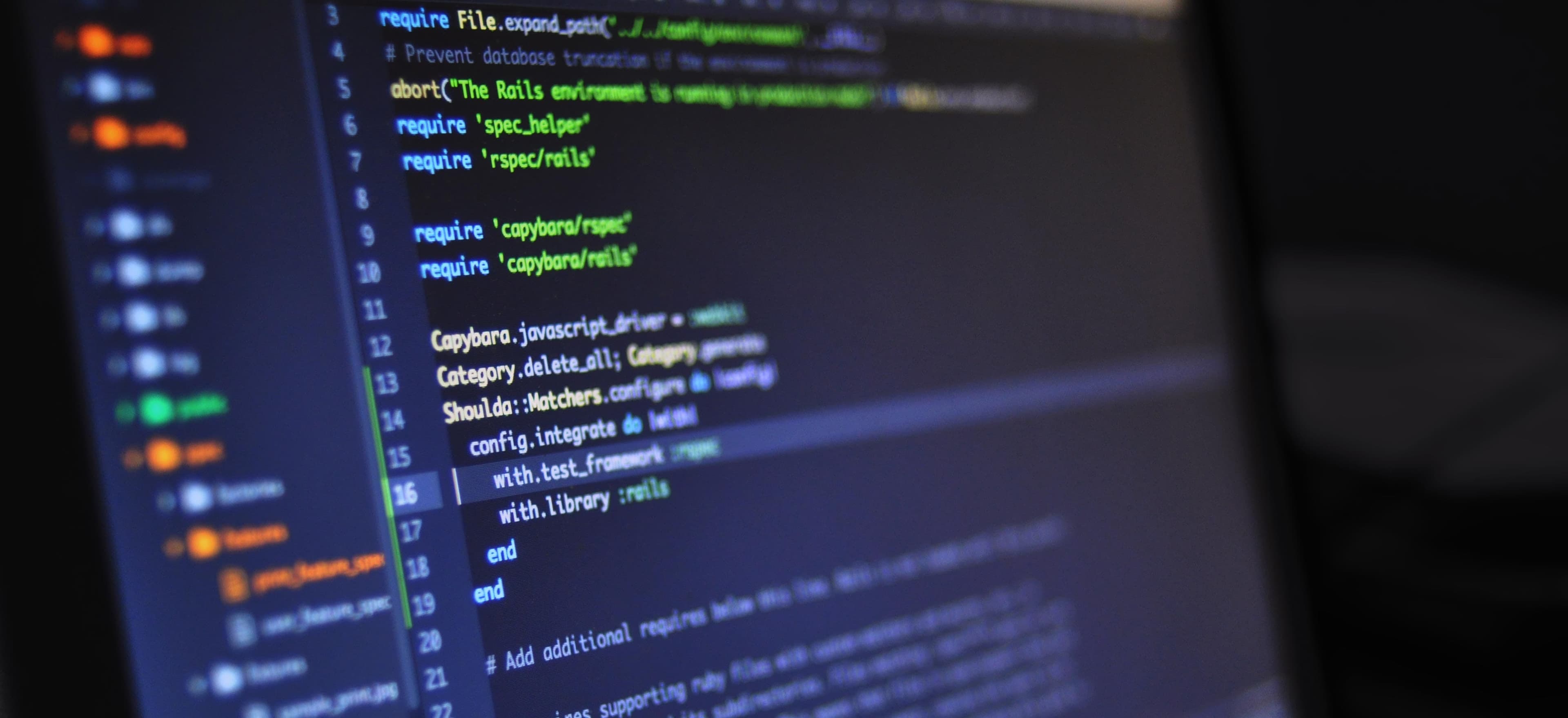
- Published on
Solving Common Pitfalls in JUnit 5 Parameterized Tests
JUnit 5 has revolutionized how we write tests in Java, especially when it comes to Parameterized Tests. If you're coming from JUnit 4, you might find the new capabilities profound yet sometimes a little overwhelming. This blog post delves into some common pitfalls with JUnit 5 Parameterized Tests and provides practical solutions to help you write robust tests effectively.
What Are Parameterized Tests?
Parameterized Tests allow developers to run the same test multiple times with different arguments. This capability is invaluable when you want to assert the behavior of a method under various conditions without writing multiple test cases.
Why Use Parameterized Tests?
- DRY Principle: Avoid code duplication by reusing test logic for different inputs.
- Clearer Tests: It makes the intention of tests clearer, helping future developers understand the use cases being asserted.
- Maintainability: Adding more test cases becomes easier. Without parameterized tests, you would need to create numerous methods for each scenario.
Pitfall 1: Incorrect Annotations
One of the first things that can trip up a developer new to JUnit 5 is not using the correct annotations. The key annotations for Parameterized Tests are:
@ParameterizedTest
@MethodSource
,@CsvSource
, or@ValueSource
Example of Correct Annotated Parameterized Test
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
public class MathUtilsTest {
@ParameterizedTest
@ValueSource(ints = {1, 2, 3, 4, 5})
void testIsPositive(int number) {
assertTrue(MathUtils.isPositive(number), "Number should be positive");
}
}
class MathUtils {
static boolean isPositive(int number) {
return number > 0;
}
}
Commentary
In this example, @ValueSource
is used to provide a list of integers to the testIsPositive
method. Each integer is tested against the assertion, demonstrating how verbose test structures can be effectively simplified.
Pitfall 2: Misunderstanding the Parameter Types
JUnit 5 allows multiple types of parameters, but developers often confuse these types. For instance, using @ValueSource
only works with primitive types, String
, or Class
. If your test logic requires more complex inputs, using @MethodSource
or @CsvSource
is crucial.
Example of Method Source
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.MethodSource;
import java.util.stream.Stream;
public class StringUtilsTest {
@ParameterizedTest
@MethodSource("provideStringsForIsBlank")
void testIsBlank(String input, boolean expected) {
assertEquals(expected, StringUtils.isBlank(input));
}
static Stream<org.junit.jupiter.params.provider.Arguments> provideStringsForIsBlank() {
return Stream.of(
Arguments.of("", true),
Arguments.of(" ", true),
Arguments.of("not blank", false)
);
}
}
class StringUtils {
static boolean isBlank(String str) {
return str == null || str.trim().isEmpty();
}
}
Commentary
Here, we use @MethodSource
which allows us to use a method that returns a stream of Arguments
. This can include multiple parameters, showcasing the flexibility of JUnit 5's parameterized tests.
Pitfall 3: Forgetting to Import Required Packages
It sounds trivial, but it's easy to overlook necessary imports, especially when focusing on the test logic. Be sure you have the right imports:
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
import static org.junit.jupiter.api.Assertions.*;
Make sure your IDE is set up to help you manage these imports, or create a test template to help maintain consistency.
Pitfall 4: Selecting Inappropriate Test Data
Choosing the right test data is crucial. Inappropriate or poorly representative data can lead to false positives or negatives. Always aim to cover both boundaries and edge cases.
Example of Comprehensive Test Data
@ParameterizedTest
@CsvSource({
"John, 25, true",
"Jane, -5, false",
"Alex, 0, false"
})
void testIsAdult(String name, int age, boolean expected) {
assertEquals(expected, AgeUtils.isAdult(name, age));
}
Commentary
Here, we utilize @CsvSource
to cover a range of ages. Each test case spans typical values, edge conditions (like 0), and invalid data (-5). This enhances test coverage, ensuring your method behaves correctly across diverse scenarios.
Pitfall 5: Not Handling Exceptions
JUnit 5 Parameterized Tests can generate exceptions, and failing to account for this can lead to test failures that provide little context. It is crucial to include failure messages in assertions or use assertThrows
for exceptions as needed.
Example with Exception Assertions
@ParameterizedTest
@ValueSource(ints = {-1, -10})
void testShouldThrowExceptionForNegative(int input) {
assertThrows(IllegalArgumentException.class, () -> {
AgeUtils.checkAge(input);
});
}
Commentary
By including assertThrows
, we clearly specify the expected behavior when wrong data is supplied. The failure message can enhance debuggability, making it easier to identify issues.
Pitfall 6: Poor Naming Conventions
Test names should be descriptive to enhance readability and maintenance. JUnit 5 allows you to use argument names in test output, so capitalizing on this offers more clarity.
Proper Naming Example
@ParameterizedTest(name = "Input: {0}, Expected: {1}")
@CsvSource({
"John, true",
"Jane, false"
})
void testIsUserValid(String username, boolean expected) {
assertEquals(expected, UserUtils.isValid(username));
}
Commentary
The name
parameter in the @ParameterizedTest
annotation creates a meaningful output during test runs. This feature enhances clarity, helping you quickly identify which input values triggered failures.
Closing the Chapter
JUnit 5 Parameterized Tests are an extraordinary asset for writing efficient and maintainable tests. By being aware of these common pitfalls and observing best practices like proper annotations, selecting appropriate test data, and crafting descriptive test names, you can leverage the full potential of JUnit 5.
For further reading, check out the JUnit 5 User Guide and explore more advanced topics such as conditional execution or custom argument providers.
Remember, testing is more than just a requirement—it's a commitment to producing reliable and robust software. Happy testing!