Maximize Productivity: Troubleshooting Ant Build Tags
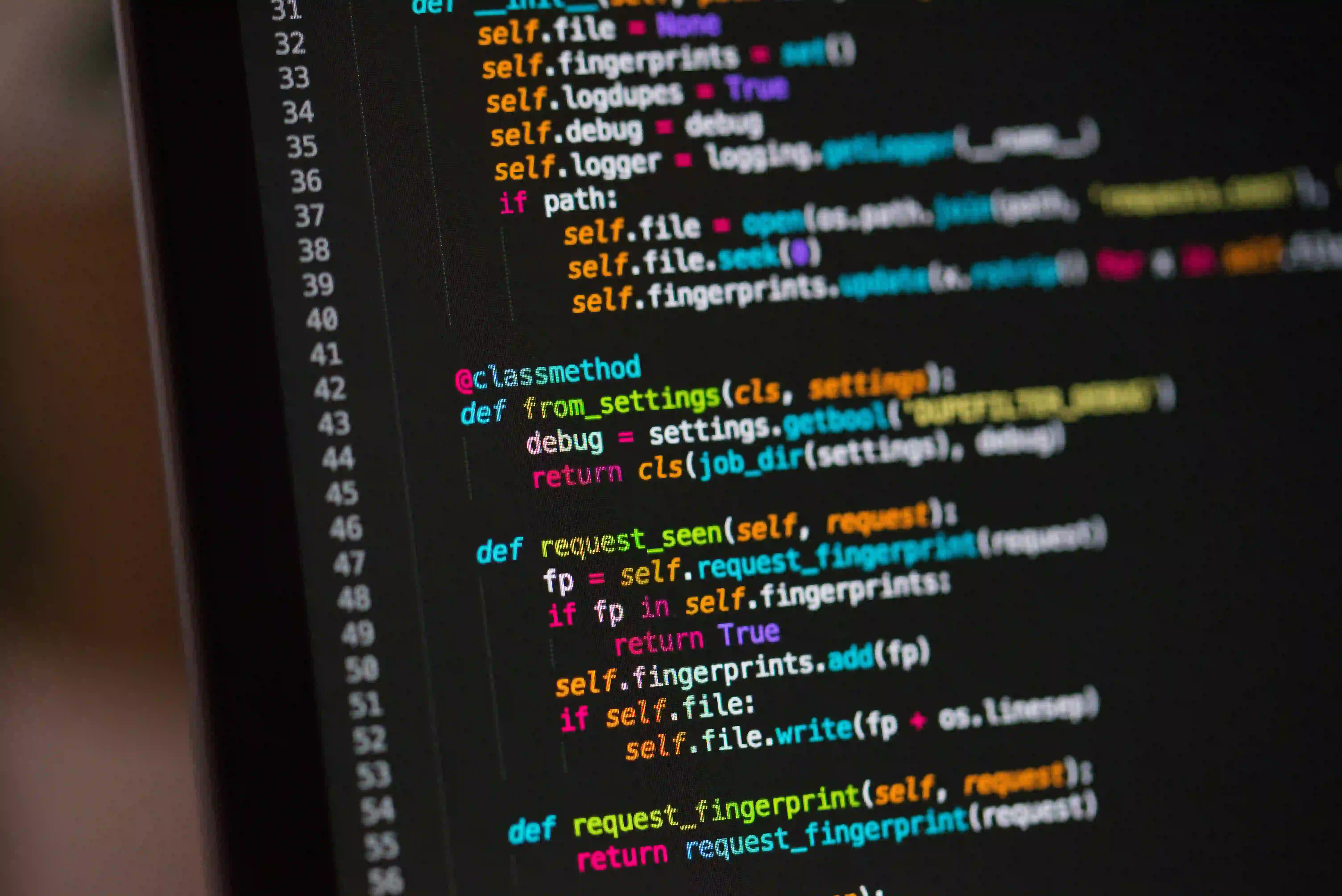
Maximize Productivity: Troubleshooting Ant Build Tags
Ant is a powerful build tool for Java projects, enabling streamlined builds and efficient project management. However, even the most seasoned developers can encounter pitfalls that can delay productivity. One common source of confusion lies in the use of Ant build tags. This blog post will delve into what Ant build tags are, why they matter, and how to troubleshoot issues effectively.
Understanding Ant Build Tags
Ant uses tags defined in XML to orchestrate the build process. Each tag represents a specific task, such as compiling source code, copying files, or creating archives. Understanding these tags is essential because they form the backbone of the build configuration.
Common Ant Tags
Here are some of the commonly used Ant build tags:
-
project: Defines the project and its attributes, such as name, default target, and base directory.
πsnippet.txt<project name="MyProject" default="compile" basedir=".">
-
target: Specifies a collection of tasks to execute. Targets can depend on other targets.
πsnippet.txt<target name="compile"> <javac srcdir="src" destdir="bin"/> </target>
-
task: Represents the actual work to be done, using predefined tasks like
javac
,jar
, andcopy
. -
property: Enables the definition of variables within your Ant build scripts.
πsnippet.txt<property name="src.dir" value="src"/>
Inevitably, when working with Ant build tags, problems can arise. Let's explore some common issues and how to troubleshoot them.
Troubleshooting Common Ant Build Tag Issues
Issue 1: Target Not Found
One of the most frequent problems with Ant scripts is the dreaded "target not found" error. This indicates an attempt to call a target that doesn't exist.
Solution
-
Check the Target Name: Double-check for typos in your command line or XML file.
π§snippet.shant compile # Ensure you are calling the correct target name
-
Inspect the Build File: Look at your
build.xml
to confirm that the target is appropriately defined. -
Review Dependencies: If a target depends on another, ensure all dependent targets are defined correctly.
Example
Hereβs a simple build.xml
file illustrating the correct definition of targets:
<project name="MyProject" default="all">
<target name="clean">
<delete dir="bin"/>
</target>
<target name="compile">
<javac srcdir="src" destdir="bin"/>
</target>
<target name="all" depends="clean, compile"/>
</project>
In this example, invoking ant all
will run clean
first, followed by compile
. Ensure such dependencies exist before executing your targets.
Issue 2: Property Not Set
If a property is referenced but not defined, Ant will report an error indicating that the property does not exist.
Solution
- Define All Properties: Ensure all properties are defined at the start of your build script.
<property name="src.dir" value="src"/>
-
Check for Typos: Verify that the property name is correctly referenced.
-
Use Default Values: If necessary, provide default values in your build file or from the command line.
Example
Hereβs how to effectively set and use properties:
<project name="MyProject" default="compile">
<property name="src.dir" value="src"/>
<property name="bin.dir" value="bin"/>
<target name="compile">
<javac srcdir="${src.dir}" destdir="${bin.dir}"/>
</target>
</project>
In this case, ${src.dir}
and ${bin.dir}
are used to improve maintainability.
Issue 3: Classpath Issues
Classpath problems can arise during the compilation step, often leading to a frustrating "cannot find symbol" error.
Solution
- Set Classpath Correctly: Ensure your
javac
invocation within the target includes the correct classpath.
<javac srcdir="${src.dir}" destdir="${bin.dir}" classpath="lib/*"/>
- Check JAR Dependencies: Ensure all necessary JAR files are present in the specified libraries directory.
Example
Here's how to set up a classpath for third-party libraries:
<project name="MyProject" default="compile">
<property name="lib.dir" value="lib"/>
<target name="compile">
<javac srcdir="${src.dir}" destdir="${bin.dir}" classpath="${lib.dir}/*"/>
</target>
</project>
By listing your libraries in the lib
directory, Ant will include them in the classpath during builds.
Issue 4: Permissions Errors
Sometimes, permission issues can prevent tasks from executing correctly. This usually happens when Ant does not have permission to read, write, or execute necessary files or directories.
Solution
-
Check Directory Permissions: Use commands such as
ls -l
on Unix-based systems to verify that your user account has the necessary permissions. -
Run as Administrator: On Windows, consider running the command prompt as an administrator when executing Ant commands.
Example
If access is denied on the bin
directory, ensure that the owner and the permissions are set correctly:
chmod 755 bin
By adjusting directory permissions, you can often solve permission-related build issues.
To Wrap Things Up
Troubleshooting Ant build tags is crucial for maintaining productivity. By understanding common errors and their solutions, you can minimize disruptions during the build process.
Want to dive deeper into Ant build scripts? Consider visiting the Apache Ant Manual for comprehensive documentation. Familiarizing yourself with these tools and techniques will enhance your command over Ant, helping you work more efficiently with Java projects.
Now, go ahead and tackle those build tags with confidence!