Mastering Java Packages: Common Function Pitfalls Explained
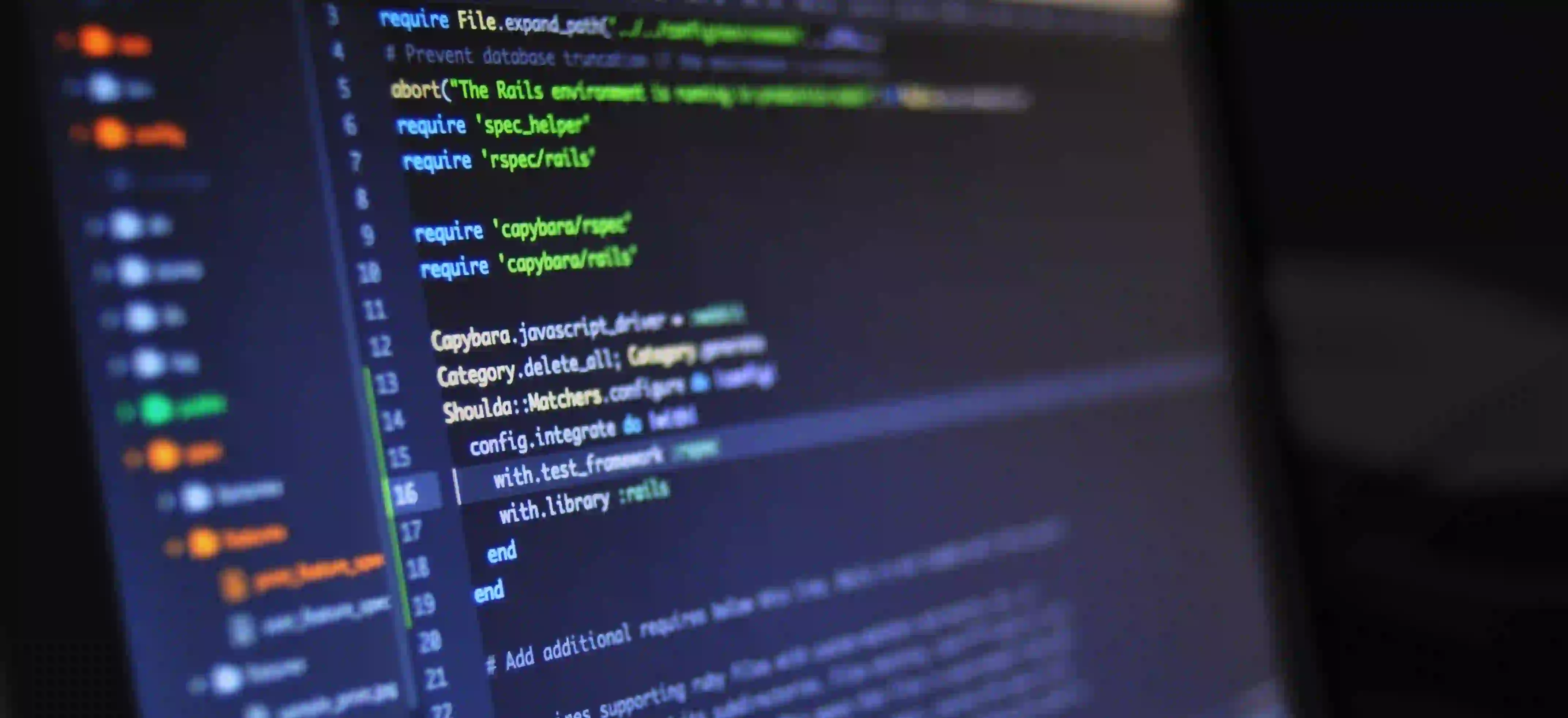
Mastering Java Packages: Common Function Pitfalls Explained
Java is a powerful and versatile programming language that is widely used for building robust applications. One of the key features of Java is its package system, which provides an organized way to group related classes and interfaces. While this organization simplifies code management, it also introduces potential pitfalls that developers must navigate. In this blog post, we will uncover common function pitfalls you may encounter while using Java packages and provide you with strategies to avoid these pitfalls effectively.
What Are Java Packages?
Java packages are essentially namespaces that help to organize classes and interfaces. Think of a package as a folder in your file system. In Java, packages help:
- Prevent naming conflicts: Two classes with the same name can exist in different packages without interfering with each other.
- Control access: You can control visibility and access of classes, making your code more secure and easier to manage.
- Organize files: Packages make it easier to locate a particular class within a complex application.
A typical Java package declaration looks like this:
package com.example.myapp;
public class MyClass {
// class implementation
}
Defining Package Structure
The package structure should ideally reflect the organization of the project. A common convention is to use reverse domain names. This helps in avoiding name collisions with other projects.
For example, if your domain is example.com
, your package might look like:
com
βββ example
βββ myapp
βββ models
βββ controllers
βββ services
This hierarchical organization assists in maintaining a clean and intuitive codebase. However, challenges arise when classes and interfaces become too intertwined across different packages.
Common Function Pitfalls in Java Packages
Even with the structured approach offered by Java packages, developers often face challenges. Let's explore some common pitfalls and how to avoid them.
1. Inconsistent Imports
One frequent issue is inconsistent import statements, which can lead to confusion about which class or interface is being referenced. In Java, if a class is imported incorrectly, it can result in ClassNotFound
exceptions or ambiguities.
Example of Inconsistent Imports:
import com.example.utilities.StringUtils;
import com.example.helpers.StringUtils; // Conflict: two classes with the same name
public class MyApp {
public void process() {
StringUtils util = new StringUtils();
// Action here
}
}
Solution: Use fully qualified names when thereβs a chance of conflict or only import classes that are directly needed.
2. Accessibility Issues
Access modifiers in Java packages can be tricky. Classes in different packages may not have the access required to communicate with each other, especially when using the default (package-private) access modifier.
Example:
// Inside com.example.models
class User {
void displayInfo() {
// implementation
}
}
// Inside com.example.controllers
public class UserController {
User user = new User(); // This will cause a compilation error
}
Solution: Identify when classes need to interact across packages and declare them public
if access is necessary for functionality. Additionally, use interfaces to decouple package dependencies.
3. Overuse of Static Imports
While static imports can make the code shorter and sometimes more readable, they can also lead to less clarity.
import static java.lang.Math.*;
public class Calculator {
public double calculateCircleArea(double radius) {
return PI * pow(radius, 2); // Less clear than Math.PI and Math.pow
}
}
Solution: Use static imports judiciously. Whenever clarity is paramount, prefer using the class name to maintain readability.
4. Package-Private Classes
Using package-private (default) access that prevents classes from being visible to other packages can lead to maintenance headaches. For instance, if you later realize you need to use a certain class in another package, it can become cumbersome.
Example:
// This class is not visible outside its package
class HiddenService {
public void serve() {
// implementation
}
}
Solution: Evaluate if classes need wider accessibility early in the design process. If a class may need to be used across multiple packages, declare it public
from the start.
5. Circular Dependencies
A more complex issue arises when two packages depend on each other, creating circular dependencies. This can lead to class loading problems and makes your architecture difficult to understand.
Example:
// Package A
package com.example.a;
public class A {
B b = new B(); // Package B is somehow tied here
}
// Package B
package com.example.b;
public class B {
A a = new A(); // Circular dependency issue
}
Solution: Carefully design the architecture of your application. Use a service-oriented approach if necessary and ensure that packages are loosely coupled.
Best Practices for Managing Java Packages
To avoid these pitfalls, here are some best practices for managing your Java packages effectively:
-
Maintain a Consistent Package Naming Convention: Follow a clear and consistent naming convention to make your codebase navigable.
-
Limit Access Modifier Scope: Use public access only when necessary. Default (package-private) can still be useful for restricting access within a specific module.
-
Utilize Refactoring Tools: Modern IDEs like IntelliJ IDEA and Eclipse offer refactoring tools to help manage and organize packages effectively, reducing errors with imports.
-
Implement Interface Segregation: Use interfaces to allow multiple packages to interact seamlessly without creating tight coupling.
-
Code Reviews: Conduct regular code reviews focused on package structure and access modifiers. Encourage peers to flag potential pitfalls and inconsistencies.
In Conclusion, Here is What Matters
Java packages play an essential role in organizing your application and ensuring maintainability. However, it's crucial to recognize the common pitfalls that can arise and develop strategies to avoid them. By adhering to best practices and fostering clear communication about package structures and dependencies, you can create a more robust and scalable Java application.
Remember, efficient management of Java packages not only improves your application's architecture but also enhances team collaboration in the long run. If you want to dive deeper into Java packages, consider reading the Java Documentation.
Feel empowered to structure your packages wisely and eliminate the pitfalls; doing so is one step towards mastering Java!