Handling File Events: Java 7 WatchService Pitfalls
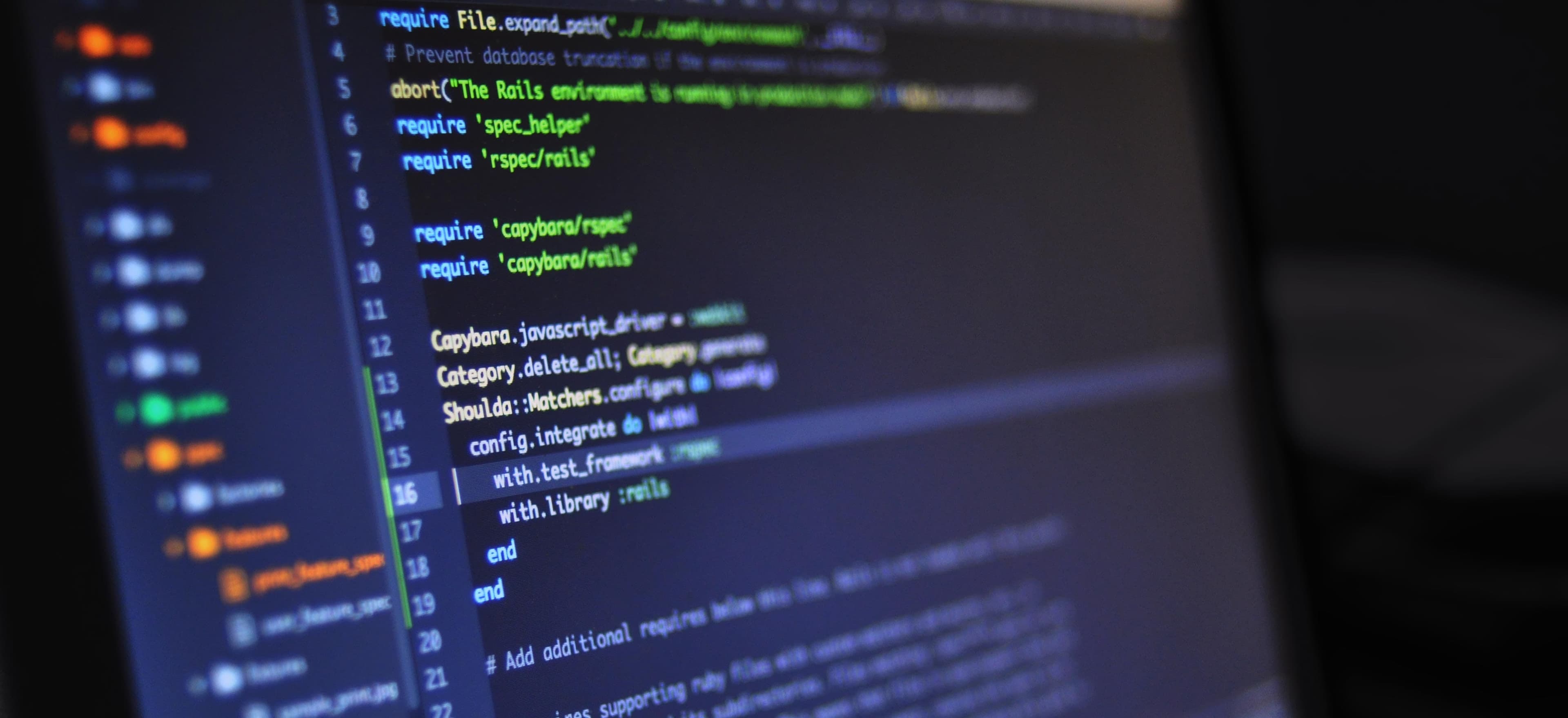
- Published on
Handling File Events: Java 7 WatchService Pitfalls
In Java, the WatchService API introduced in Java 7 provides a robust way to monitor file system changes. While it offers a powerful mechanism for tracking events such as file creation, deletion, and modification, there are pitfalls that can arise when using it. In this blog post, we'll explore these pitfalls, provide exemplary code snippets, and discuss best practices for effectively handling file events.
Understanding the WatchService API
The WatchService API allows developers to register a directory and listen for events triggered by changes in that directory. It is part of the java.nio.file
package and improves the performance and usability of file I/O operations.
Basic Setup
To get started with the WatchService, you'll need to set up a directory watcher. Here's a brief snippet that initializes a WatchService to monitor a directory for file creation events:
import java.nio.file.*;
public class DirectoryWatcher {
public static void main(String[] args) {
try {
// Get the default WatchService
WatchService watchService = FileSystems.getDefault().newWatchService();
// Register the directory to watch
Path path = Paths.get("path/to/your/directory");
path.register(watchService, StandardWatchEventKinds.ENTRY_CREATE);
System.out.println("Watching directory: " + path);
// Poll for events
while (true) {
WatchKey key = watchService.take(); // Blocking call
for (WatchEvent<?> event : key.pollEvents()) {
WatchEvent.Kind<?> kind = event.kind();
if (kind == StandardWatchEventKinds.OVERFLOW) {
continue; // Overflow occurred, skip this event.
}
// Context contains the file name
Path fileName = (Path) event.context();
System.out.println("New file created: " + fileName);
}
key.reset(); // Reset the key to receive further events
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
- WatchService Creation: The
FileSystems.getDefault().newWatchService()
method initializes a new WatchService instance. - Directory Registration: The
path.register()
method is used to register the directory to listen to specific events; in this case, file creation events. - Polling for Events: The while loop keeps the service running and polls for events. The
watchService.take()
call is a blocking operation that waits until an event occurs.
Pitfall #1: Buffer Overflow
A significant caveat is that, under heavy load, the WatchService may experience buffer overflow. When this occurs, events can be lost. Thus, you must check for the OVERFLOW
event and handle it appropriately.
if (kind == StandardWatchEventKinds.OVERFLOW) {
System.err.println("Event overflow occurred, some events may not have been processed.");
continue; // Skip this event, since we can't rely on it
}
Pitfall #2: Using a Blocking Approach
While the above example uses a blocking approach with watchService.take()
, this may cause problems in scenarios where you need a non-blocking method or more flexibility. Instead, consider using a separate thread to handle events or using the poll()
method.
Here's an updated version employing a polling mechanism:
while(true) {
WatchKey key = watchService.poll(); // Non-blocking
if (key != null) {
for (WatchEvent<?> event : key.pollEvents()) {
WatchEvent.Kind<?> kind = event.kind();
if (kind != StandardWatchEventKinds.OVERFLOW) {
Path fileName = (Path) event.context();
System.out.println("Event detected: " + kind + " on file: " + fileName);
}
}
key.reset();
}
// Additional logic can be placed here to avoid busy-waiting
}
This polling approach permits other operations to be executed while waiting for file system events, thus increasing the agility and performance of your application.
Pitfall #3: Directory Deletions
When a directory being watched is deleted or moved, the WatchService stops generating events for it. If your application logic relies on continuous monitoring, ensure you have contingency handling for such scenarios.
public void handleDirectoryDeletion(Path path) {
if (!Files.exists(path)) {
System.err.println("Watched directory has been deleted or moved: " + path);
// Consider re-registering or exiting gracefully
}
}
Pitfall #4: Event Order
The order of events is not guaranteed. If several events occur quickly, you may find them in a different order than they were processed. This can complicate certain use cases where the order is critical, such as when two files depend on each other.
It is crucial to manage your logic accordingly. One way of handling this is to introduce event queuing or maintain a stateful pattern so you can order the events logically based on timestamps.
Best Practices for Using WatchService
- Separate Threads: Always handle file events in a separate thread to keep your main application responsive.
- Event Queueing: Implement an event queue to manage incoming events effectively and to process them in sequence.
- Exception Handling: Make sure to implement robust error handling to catch and manage exceptions effectively.
- Performance Monitoring: Regularly monitor the performance implications of your WatchService usages, especially under high loads.
To Wrap Things Up
While the Java 7 WatchService API provides a powerful means to handle file system events, understanding its pitfalls and implementing best practices can mitigate potential issues. By integrating effective error handling, leveraging separate threads, and considering performance implications, you can build a robust file monitoring solution that caters to your application's needs.
For more information about the WatchService API, you can refer to the Java Documentation.
By employing the strategies outlined in this article, you can become adept at handling file events in Java while avoiding common pitfalls. Happy coding!
Checkout our other articles