Mastering JPA: Overcoming the N+1 Select Dilemma
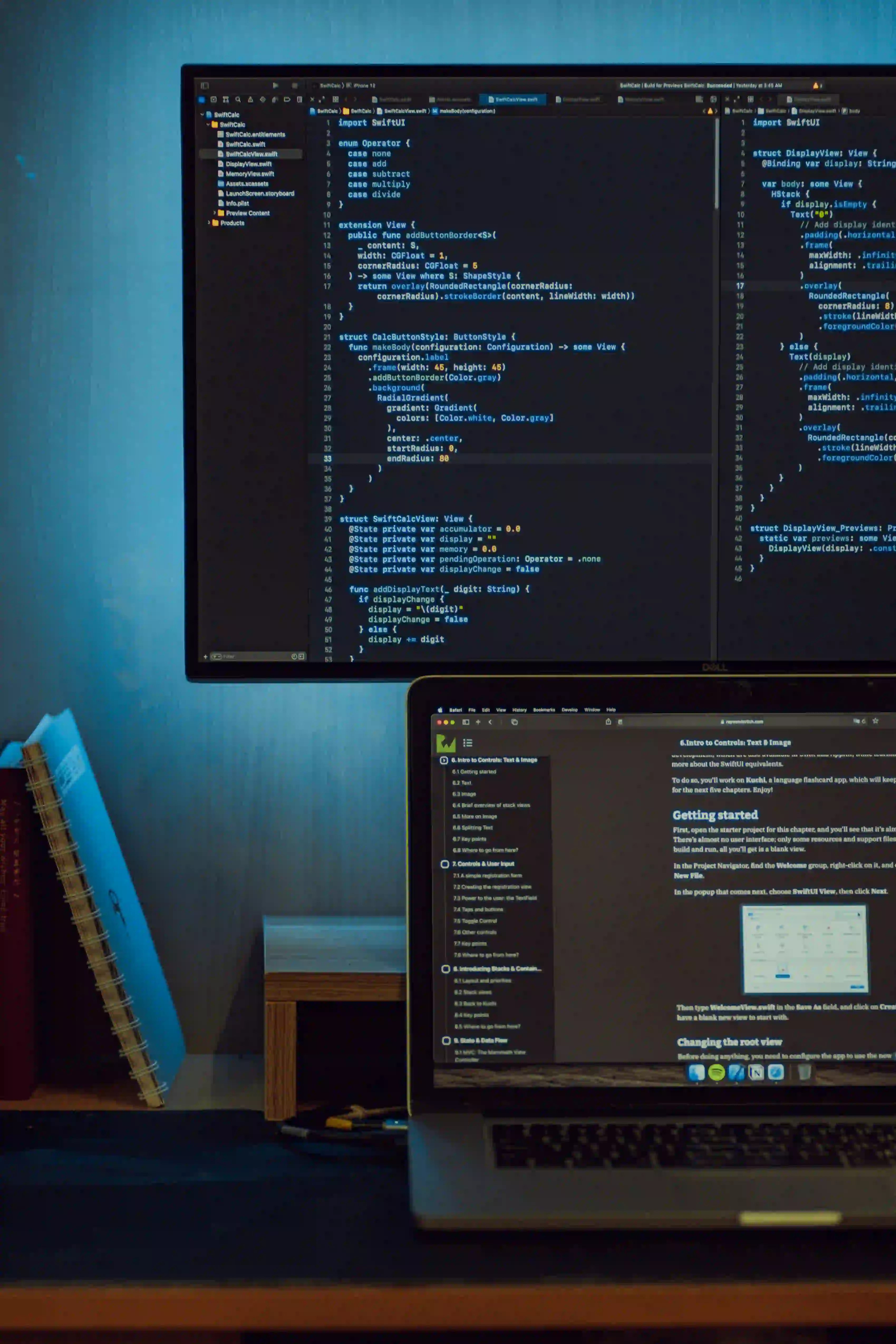
Mastering JPA: Overcoming the N+1 Select Dilemma
When working with Java Persistence API (JPA), one of the most prevalent issues developers encounter is the notorious N+1 Select problem. This issue leads to ineffective database access patterns, causing performance issues that can degrade the user experience. In this blog post, we'll delve into the N+1 Select issue, understand its implications, and explore various strategies to overcome it using JPA efficiently.
Understanding the N+1 Select Problem
What is N+1 Select?
The N+1 Select problem occurs when an application executes one query to retrieve a list of entities and then executes additional queries to retrieve related entities for each of those records. For example, consider an application that retrieves a list of Authors
and their associated Books
.
In this case:
- The first query retrieves all
Authors
, which is 1 query (N=1). - For each
Author
, an additional query retrieves theirBooks
, resulting in N additional queries (where N is the number of authors).
This means that if you have, say, 10 authors, the total number of SQL queries executed would be 1 (for authors) + 10 (for books) = 11 queries in total.
Example Scenario
Let’s illustrate this with a sample code snippet. Consider the following JPA entity relationships:
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author")
private List<Book> books;
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "author_id")
private Author author;
}
Now, when we try to load authors and their books:
public List<Author> findAllAuthors() {
return entityManager.createQuery("SELECT a FROM Author a", Author.class).getResultList();
}
The aforementioned query results in the N+1 problem if you iterate over authors to get their books:
List<Author> authors = findAllAuthors();
for (Author author : authors) {
System.out.println(author.getBooks().size()); // This triggers another SQL query for each author
}
As the application grows, this can lead to performance bottlenecks and strain on database resources.
Strategies to Overcome the N+1 Select Problem
Now that we understand the N+1 Select Problem, let's explore ways to mitigate it.
1. Eager Fetching
Eager fetching allows you to specify that related entities should be loaded at the same time as the primary entity. This can be done using the fetch
attribute on your @OneToMany
annotation:
@OneToMany(mappedBy = "author", fetch = FetchType.EAGER)
private List<Book> books;
With eager fetching, when you retrieve authors, the books will be loaded in a single query, which makes the database access more efficient.
Pros and Cons
- Pros: Reduces the number of SQL queries; better performance for read-heavy operations where relationships are frequently accessed together.
- Cons: It can lead to loading too much unnecessary data (potentially causing memory issues) if not handled carefully, especially in large datasets.
2. Fetch Joins
Fetch joins are a more controlled approach to load related entities. You can combine the fetching of entities into a single query using JPQL:
public List<Author> findAllAuthorsWithBooks() {
return entityManager.createQuery(
"SELECT a FROM Author a JOIN FETCH a.books", Author.class
).getResultList();
}
This query will bring authors and their books back in just one SQL query, significantly improving performance.
Why Use Fetch Joins?
Using fetch joins allows you to selectively load relationships based on your specific needs, making it easier to manage memory and workload on your database.
3. Batch Fetching
Batch fetching allows you to load entities efficiently in batches instead of one at a time. You can use @BatchSize
annotation on your entity:
@Entity
public class Author {
...
@BatchSize(size = 10)
public List<Book> getBooks() {
return books;
}
}
This method avoids the N+1 query problem by increasing the number of Books
fetched in a single database call when navigating the relationship.
4. Pagination
When working with large datasets, consider pagination. JPA provides built-in support for pagination through the setFirstResult()
and setMaxResults()
methods.
public List<Author> findPaginatedAuthors(int pageNumber, int pageSize) {
return entityManager.createQuery("SELECT a FROM Author a", Author.class)
.setFirstResult(pageNumber * pageSize)
.setMaxResults(pageSize)
.getResultList();
}
This approach helps split the dataset into manageable chunks, reducing the load on your database.
5. DTO Projections
Instead of loading entire entities, you can create Data Transfer Objects (DTOs) that contain only the fields you need. This limits the amount of data fetched and can reduce the number of queries executed.
Example DTO:
public class AuthorDTO {
private Long id;
private String name;
private List<String> bookTitles;
public AuthorDTO(Long id, String name, List<String> bookTitles) {
this.id = id;
this.name = name;
this.bookTitles = bookTitles;
}
}
Using JPQL to populate DTOs:
public List<AuthorDTO> findAuthorDTOs() {
return entityManager.createQuery(
"SELECT new AuthorDTO(a.id, a.name, b.title) FROM Author a JOIN a.books b", AuthorDTO.class
).getResultList();
}
Lessons Learned
The N+1 Select Problem can be a significant hurdle for JPA developers, resulting in performance issues that can impact user experience and resource utilization. Understanding how this issue arises is the first step to addressing it effectively.
By employing strategies such as eager fetching, fetch joins, batch fetching, pagination, and using DTO projections, you can significantly reduce the overhead of database calls and improve performance in your applications. Always analyze the specific needs of your application and data access patterns to determine the best strategy for your use case.
For further reading, consider checking out resources such as:
By mastering these techniques, you'll not only enhance the efficiency of your application but also bolster its scalability for future growth. Happy coding!