Common Pitfalls in Securing Spring Boot with Okta
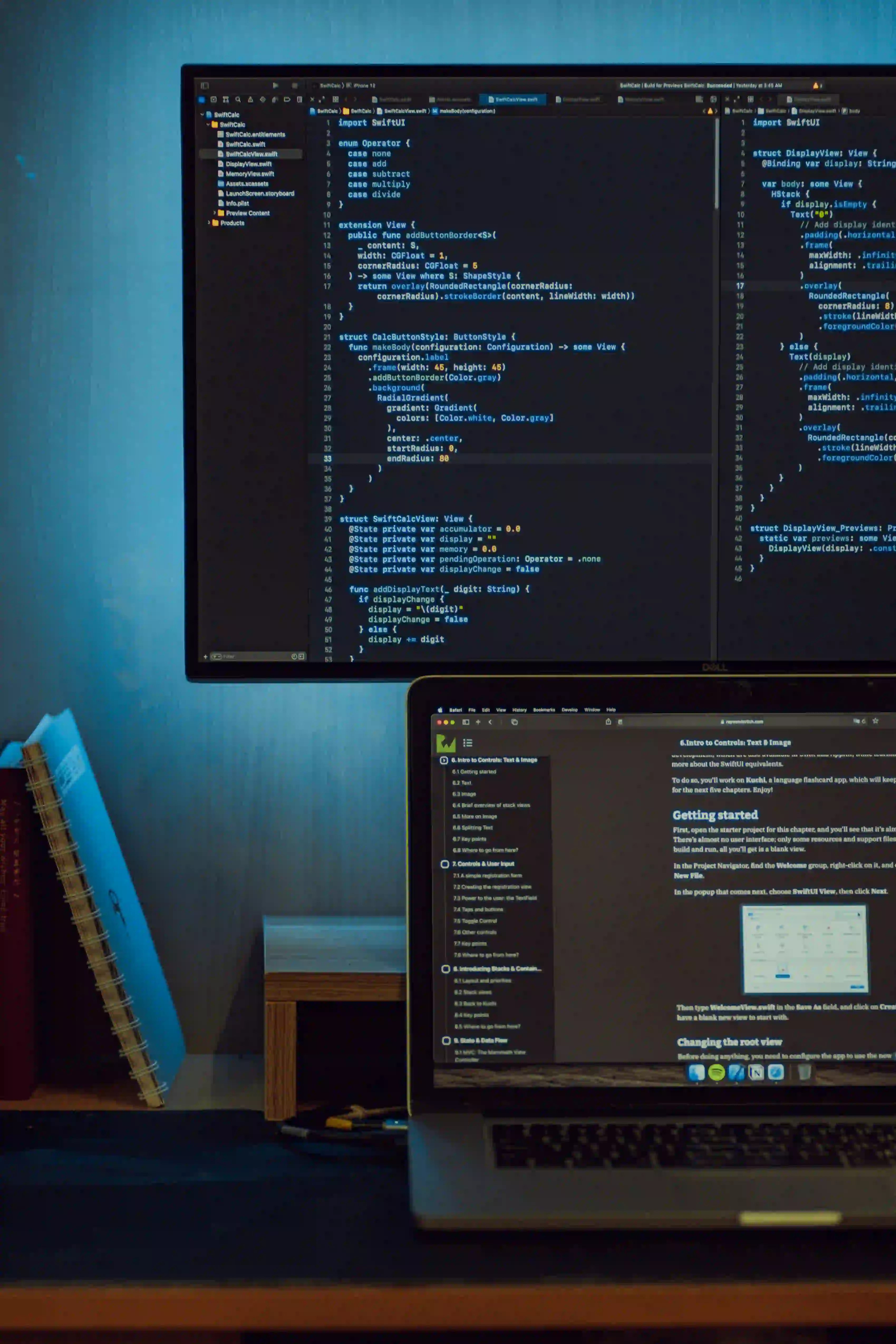
Common Pitfalls in Securing Spring Boot with Okta
Security is a critical aspect of any web application. Spring Boot simplifies the process of developing applications while also providing robust security features. Integrating with identity providers like Okta enhances security but carries its own set of challenges. In this blog post, we will explore common pitfalls when securing a Spring Boot application with Okta and provide best practices to avoid them.
Table of Contents
- Understanding Spring Boot and Okta
- Key Pitfalls to Avoid
- Misconfiguration
- Ignoring Security Annotations
- Insufficient Token Validation
- Overlooking Logout Functionality
- Best Practices for Securing Spring Boot with Okta
- Conclusion
Understanding Spring Boot and Okta
Spring Boot is a powerful framework that enables rapid application development with minimal configuration. It allows developers to build standalone applications that "just run". Meanwhile, Okta is a leading identity and access management provider offering a robust API for managing authentication and authorization.
When you combine these two technologies, you get a powerful setup for securing your applications. However, improper implementation can lead to vulnerabilities and misconfigurations that could compromise the application's integrity.
Key Pitfalls to Avoid
1. Misconfiguration
One of the most common pitfalls is misconfiguring the OAuth2 settings in Spring Boot. This can lead to failures in redirect URI settings, client IDs, and secret keys.
Sample Configuration
spring:
security:
oauth2:
client:
registration:
okta:
client-id: YOUR_CLIENT_ID
client-secret: YOUR_CLIENT_SECRET
scope: openid, profile, email
redirect-uri: "{baseUrl}/login/oauth2/code/{registrationId}"
provider:
okta:
authorization-uri: https://{yourOktaDomain}/oauth2/default/v1/authorize
token-uri: https://{yourOktaDomain}/oauth2/default/v1/token
user-info-uri: https://{yourOktaDomain}/oauth2/default/v1/userinfo
Why correct configuration matters: This configuration dictates how your application communicates with Okta. Failing to enter the right URIs or client details can prevent your application from authenticating users properly.
2. Ignoring Security Annotations
Spring Security provides powerful annotations like @PreAuthorize
and @Secured
to control access at the method level. Ignoring these can lead to overexposed endpoints.
Example of Security Annotations
@RestController
@RequestMapping("/api/user")
public class UserController {
@GetMapping("/profile")
@PreAuthorize("hasAuthority('SCOPE_profile')")
public ResponseEntity<UserProfile> getUserProfile() {
// Business logic to retrieve user profile
return ResponseEntity.ok(userProfile);
}
}
Why use security annotations: They ensure that only authorized users can access sensitive data and functionalities. Neglecting to apply these annotations can expose your application's API endpoints to unauthorized access.
3. Insufficient Token Validation
A common oversight in many implementations is failing to validate the JWT (JSON Web Token) correctly. Without rigorous token validation, you leave your application vulnerable to attacks.
Token Validation Example
@Bean
public JwtDecoder jwtDecoder() {
return JwtDecoders.fromOidcIssuerLocation("https://{yourOktaDomain}/oauth2/default");
}
Why stringent token validation is critical: Validating the token ensures that it hasn't expired or been tampered with. It’s essential for maintaining the application’s integrity and trustworthiness.
4. Overlooking Logout Functionality
Implementing authentication is only half the battle. Logging users out securely is equally important. A poorly implemented logout can expose the system to session fixation attacks.
Logout Implementation
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.logout()
.logoutSuccessUrl("{baseUrl}/")
.clearAuthentication(true)
.invalidateHttpSession(true);
}
Why proper logout mechanisms matter: Securely handling logout is crucial in preventing unauthorized users from reusing an existing session. This is a common oversight that can lead to serious security vulnerabilities.
Best Practices for Securing Spring Boot with Okta
To further reinforce security, here are additional best practices:
-
Use Environment Variables for Sensitive Configuration: Store sensitive information like client secrets in environment variables to prevent it from being hard-coded. This enhances security by ensuring that secrets are not exposed in source code.
-
Keep Dependencies Updated: Regularly update your Spring and Okta dependencies to protect against known vulnerabilities.
-
Implement Rate Limiting: Employ rate limiting on your authentication endpoints to mitigate brute-force attacks.
-
Monitor and Log Access: Use monitoring tools to log access to your application. Analyzing these logs can help detect anomalies and potential intrusions.
-
Regularly Review Security Policies: Periodically review and update security policies to adapt to emerging threats and vulnerabilities.
Key Takeaways
Securing a Spring Boot application with Okta involves understanding potential pitfalls and implementing best practices. Misconfiguration, ignoring security annotations, insufficient token validation, and overlooking logout functionalities are just a few common mistakes developers make. By paying attention to these areas and following recommended practices, you can safeguard your application effectively.
For more detailed guidance, consider checking the official documentation for Spring Security and Okta. Remember that security is not a one-time task but an ongoing commitment. Regularly revisiting your security posture can help mitigate risks and keep your application secure.
By following the insights shared in this article, you will not only improve your application’s security but also gain confidence in deploying robust and secure applications to production. Happy coding!