Why Java Serialization Can Break Your Application
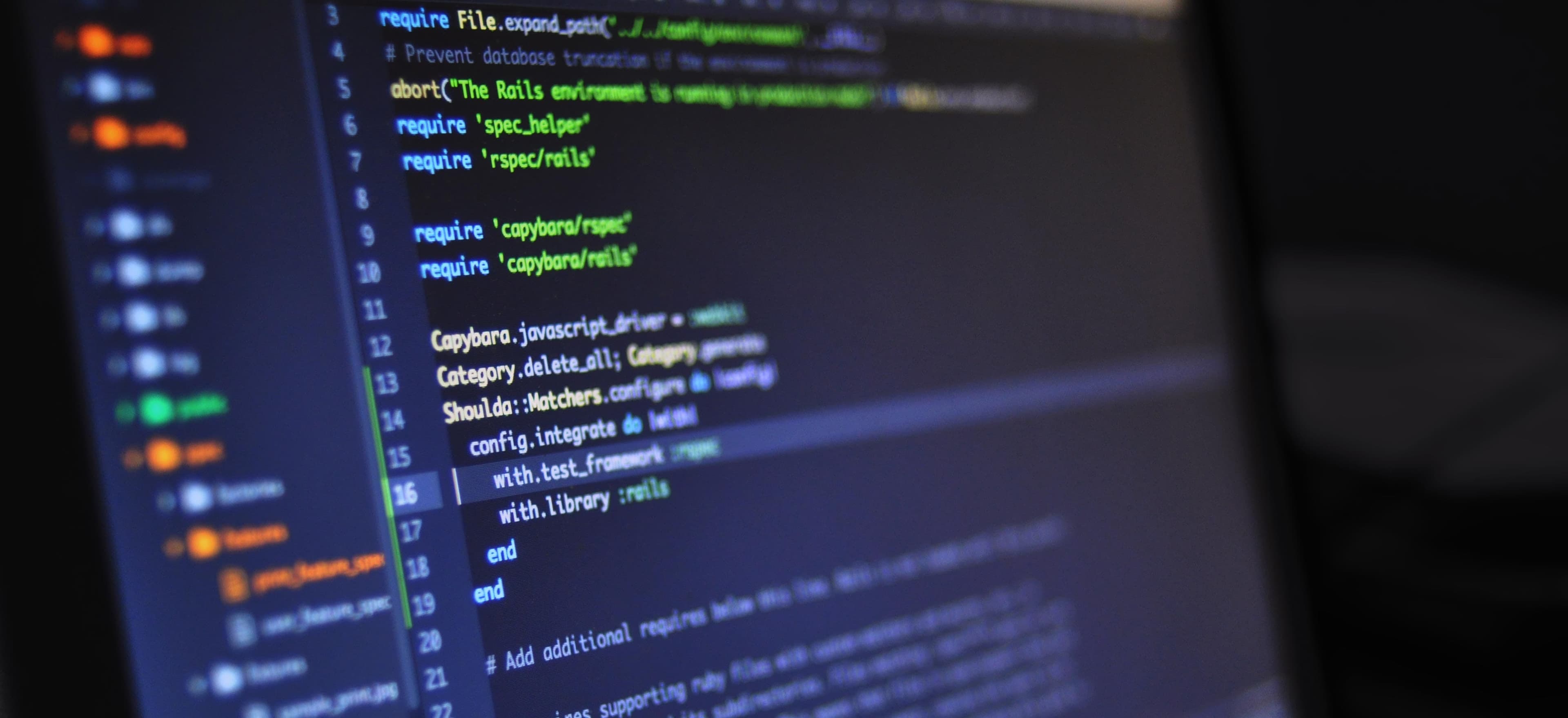
- Published on
Why Java Serialization Can Break Your Application
Java serialization is a powerful mechanism that allows you to convert an object into a byte stream, making it easy to save objects to files, send them over a network, or store them in databases. However, while serialization can be straightforward and quite useful, it also has drawbacks that can lead to application issues when mismanaged. This blog post will give you insights into why Java serialization can break your application, and how you can mitigate the risks.
Understanding Serialization
Serialization in Java is performed using the Serializable
interface. When a class implements this interface, it can be converted into a byte stream. Here's a simple example:
import java.io.*;
class User implements Serializable {
private String username;
private int age;
public User(String username, int age) {
this.username = username;
this.age = age;
}
@Override
public String toString() {
return "User{" +
"username='" + username + '\'' +
", age=" + age +
'}';
}
}
In this example, the User
class is marked as Serializable
, allowing instances of it to be serialized.
Why Use Serialization?
The primary reasons for using serialization include:
- Persistence: Storing the state of an object for later use.
- Communication: Transmitting objects over the network.
- Data Exchange: Sending data between different parts of an application or between different applications.
Despite these advantages, serialization can quickly become a source of problems.
Common Pitfalls of Serialization
1. Versioning Issues
One of the most significant pitfalls of serialization is versioning. If your object structure changes, you may run into InvalidClassException
. This issue often arises when a class’s serialVersionUID does not match that of a serialized object's version.
To mitigate this, define a unique serialVersionUID
in your class:
private static final long serialVersionUID = 1L; // Always increase this if you change the class!
If you need to make backward-compatible changes, consider adding new fields and providing default values for them.
2. Security Risks
Serialization can introduce security vulnerabilities, especially if untrusted data is deserialized. A malicious actor could craft a stream that, when deserialized, executes unwanted code. To prevent this, consider using a safer serialization mechanism, such as JSON or XML, rather than Java serialization.
3. Increased Memory Usage
Serialized objects are typically larger than their in-memory counterparts. This increase is due to the additional information added to the serialized stream. To reduce memory overhead, consider using efficient serialization libraries like Kryo or FST.
4. Performance Issues
Serialization can be slow, especially when dealing with large object graphs. If performance is a critical aspect of your application, it might be worthwhile to evaluate other serialization options that can achieve better throughput.
5. Serialization of Non-Serializable Fields
When a class has fields that do not implement Serializable
, it can result in a NotSerializableException
. To avoid this, mark those non-serializable fields as transient
.
class User implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
private transient String password; // This field will not be serialized
// Constructor and methods
}
6. Complex Object Graphs
When you serialize objects that contain references to other objects, you risk creating complex object graphs, which can lead to unexpected behavior during deserialization. If possible, simplify the structure of your objects, or flatten them when necessary.
Alternatives to Java Serialization
Java serialization may not always be the best option for your application. Here are some alternatives you can explore:
- JSON Serialization: Libraries like Jackson provide an easy way to serialize and deserialize objects to and from JSON.
import com.fasterxml.jackson.databind.ObjectMapper;
User user = new User("john_doe", 25);
ObjectMapper objectMapper = new ObjectMapper();
String jsonString = objectMapper.writeValueAsString(user);
User deserializedUser = objectMapper.readValue(jsonString, User.class);
-
Protocol Buffers: Google’s Protocol Buffers offer a more efficient binary serialization method that can be particularly beneficial for performance.
-
Kryo: As mentioned earlier, Kryo is an efficient binary serialization framework. It can serialize and deserialize more quickly than the default Java serialization.
Best Practices for Safe Serialization
To ensure that your application remains robust while using serialization, consider the following best practices:
-
Always declare a serialVersionUID: This avoids versioning issues that can arise when class definitions change.
-
Use transient for non-serializable fields: This keeps problems at bay when your class contains fields that shouldn’t be serialized.
-
Consider using custom serialization methods: Implement
writeObject
andreadObject
methods to control the serialization process better.
private void writeObject(ObjectOutputStream out) throws IOException {
out.defaultWriteObject(); // Write default fields
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject(); // Read default fields
}
-
Evaluate alternative serialization frameworks: Depending on your project needs, other serialization methods may be more appropriate.
-
Implement thorough security checks: Always validate incoming serialized data to protect against potential attacks.
Lessons Learned
Java serialization offers many conveniences but also comes with a fair share of pitfalls that can break your application. Understanding the challenges related to versioning, security, performance, and non-serializable fields is crucial for crafting robust applications. By following best practices and considering alternative serialization methods, you can harness the benefits of serialization without falling prey to its common dangers.
For further reading on Java serialization, consider checking the official Java documentation for a deeper dive into the topic.
By being forewarned and adopting a proactive approach, you can utilize serialization effectively while avoiding potential application breakdowns.
Feel free to share your thoughts or questions regarding serialization in Java in the comments below!