Understanding Apache Shiro's Authentication Pitfalls
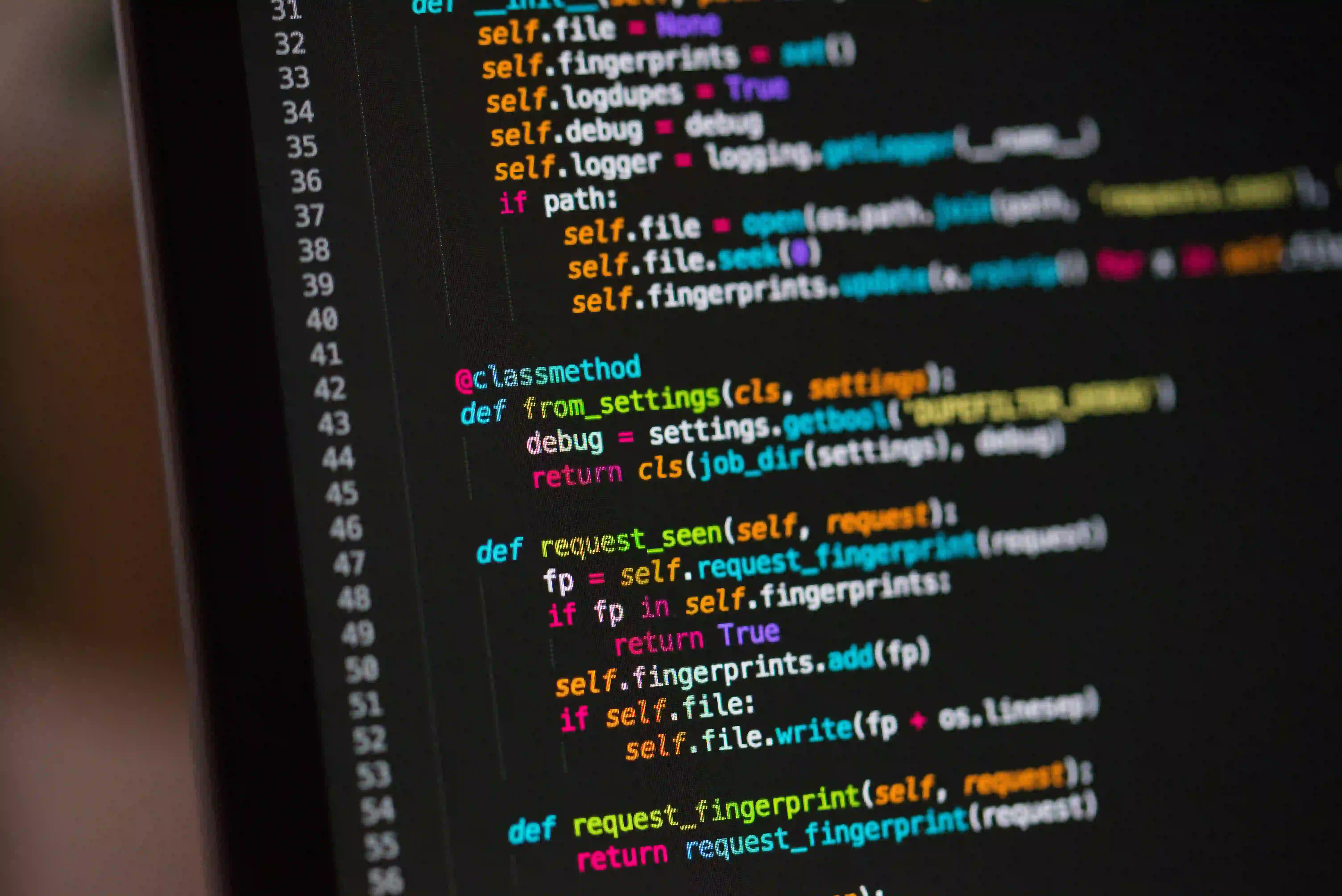
Understanding Apache Shiro's Authentication Pitfalls
In the realm of Java application security, Apache Shiro stands out as a powerful framework that simplifies authentication, authorization, cryptography, and session management. However, while Shiro is designed to be user-friendly, it is not devoid of pitfalls—especially concerning authentication. In this post, we will explore these pitfalls systematically and provide actionable insights to ensure secure authentication practices with Apache Shiro.
What is Apache Shiro?
Apache Shiro is a versatile Java security framework that aims to handle authentication, authorization, session management, and cryptography. Unlike other security frameworks that tend to be complicated, Shiro is designed with simplicity in mind, promoting easy integration into any Java application. However, with simplicity comes the responsibility of understanding its limitations and potential issues, especially in authentication.
The Importance of Authentication
Authentication is the process of verifying the identity of a user. In simpler terms, it ensures that the user claiming to be someone is indeed who they say they are. This process is vital for maintaining the integrity and security of an application, particularly in today's digital landscape, where unauthorized access can lead to significant data breaches and security issues.
Common Authentication Pitfalls in Apache Shiro
1. Weak Password Policies
One of the most common pitfalls is not enforcing a robust password policy. It's tempting to allow users to set weak passwords due to ease of use, but this approach can lead to security vulnerabilities.
Solution: Enforce Strong Passwords
public class PasswordValidator {
private static final Pattern STRONG_PASSWORD_PATTERN =
Pattern.compile("(?=.*[0-9])(?=.*[a-z])(?=.*[A-Z]).{8,}");
public boolean isValid(String password) {
return STRONG_PASSWORD_PATTERN.matcher(password).matches();
}
}
In this snippet, the PasswordValidator
enforces a strong password policy. A strong password must contain at least one number, one uppercase letter, one lowercase letter, and be at least eight characters long. This simple validation reduces the likelihood of brute-force attacks.
2. Insecure Storage of User Credentials
Storing user credentials insecurely is a grave mistake. It is essential to hash passwords before saving them in a database. Storing plain-text passwords opens doors to hackers if a data breach occurs.
Solution: Use Secure Hashing Algorithms
import org.apache.shiro.crypto.hash.SimpleHash;
public String hashPassword(String plainPassword, String salt) {
return new SimpleHash("SHA-256", plainPassword, salt, 1024).toHex();
}
Here, the hashPassword
method uses the SHA-256 hashing algorithm to securely hash the password. The salt
provides an extra layer of security by ensuring that even identical passwords result in different hashes. Additionally, the 1024
iterations increase the hashing time, making it harder for attackers to use brute-force methods.
3. Improper Use of Sessions
Another potential pitfall is the improper handling of sessions. If not managed correctly, attacks such as session fixation or session hijacking can occur.
Solution: Implement Proper Session Management
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.session.Session;
public void manageSession() {
Session session = SecurityUtils.getSubject().getSession();
session.setTimeout(1800000); // Set session timeout to 30 minutes
}
This session management code snippet ensures that sessions time out after 30 minutes of inactivity. Furthermore, continuously monitoring session activity can prevent unauthorized usage.
4. Lack of Multi-Factor Authentication (MFA)
With the rise of cyber threats, multi-factor authentication has become crucial. Relying solely on passwords increases risks significantly.
Solution: Implement MFA Integrating MFA with Apache Shiro may not be straightforward since Shiro does not provide built-in support for it. You can implement it by combining Shiro with other libraries, such as Spring Security or by using APIs that provide such capabilities. This way, you can alert users to verify a one-time code sent via SMS or email during a login attempt.
5. Not Using Secure Protocols
Sending sensitive data over insecure channels is a significant vulnerability. Avoid using HTTP for authentication as it exposes credentials to interception.
Solution: Use HTTPS Make sure that your web application enforces HTTPS for all traffic.
<Connector protocol="HTTP/1.1"
port="8080"
redirectPort="8443"
secure="true"
scheme="https"
sslRedirect="true" />
This configuration snippet from a Tomcat server's server.xml
file redirects HTTP traffic to HTTPS, adding an essential layer of security for your authentication process.
6. Inadequate Logging and Monitoring
Without effective logging and monitoring, suspicious activities can go unnoticed. This lack of oversight can hinder your response to potential security breaches.
Solution: Enable Detailed Logging Shiro provides a robust logging mechanism that can be easily configured for monitoring authentication attempts and failures.
log4j.logger.org.apache.shiro=DEBUG
Enabling detailed logging for Shiro can help detect unwanted authentication attempts. Additionally, integrate with tools like ELK (Elasticsearch, Logstash, and Kibana) or Splunk for real-time monitoring and alerting.
Best Practices for Secure Authentication with Apache Shiro
-
Mandatory Password Policies: Always enforce strong password policies. Use libraries like JPasswordStrengthValidator to automate this.
-
Secure Password Storage: Always store passwords using strong, salted hashes.
-
Session Management: Regularly review and manage user sessions effectively. Use Shiro's session management features.
-
Implement MFA: Look for reliable third-party APIs to add multi-factor authentication to your application.
-
Use HTTPS: Always direct traffic over HTTPS to secure sensitive information during transmission.
-
Enable Monitoring and Logging: Implement a logging system and regularly review logs to detect and analyze suspicious activities.
-
Stay Updated: Keep your Shiro and other dependencies updated to leverage the latest security enhancements.
The Bottom Line
Apache Shiro is an incredibly powerful tool for managing authentication in Java applications, but it comes with challenges. By understanding and addressing common pitfalls, developers can ensure that their applications are secure against unauthorized access.
Security is an ongoing endeavor—stay informed and proactive, and you can safeguard your applications against evolving threats. For thorough documentation and advanced configuration options, be sure to check out the official Apache Shiro documentation.
By following these best practices and focusing on security, you can leverage the full potential of Apache Shiro while keeping your application safe and secure.