Common Pitfalls When Migrating Spring Apps to JUnit 5
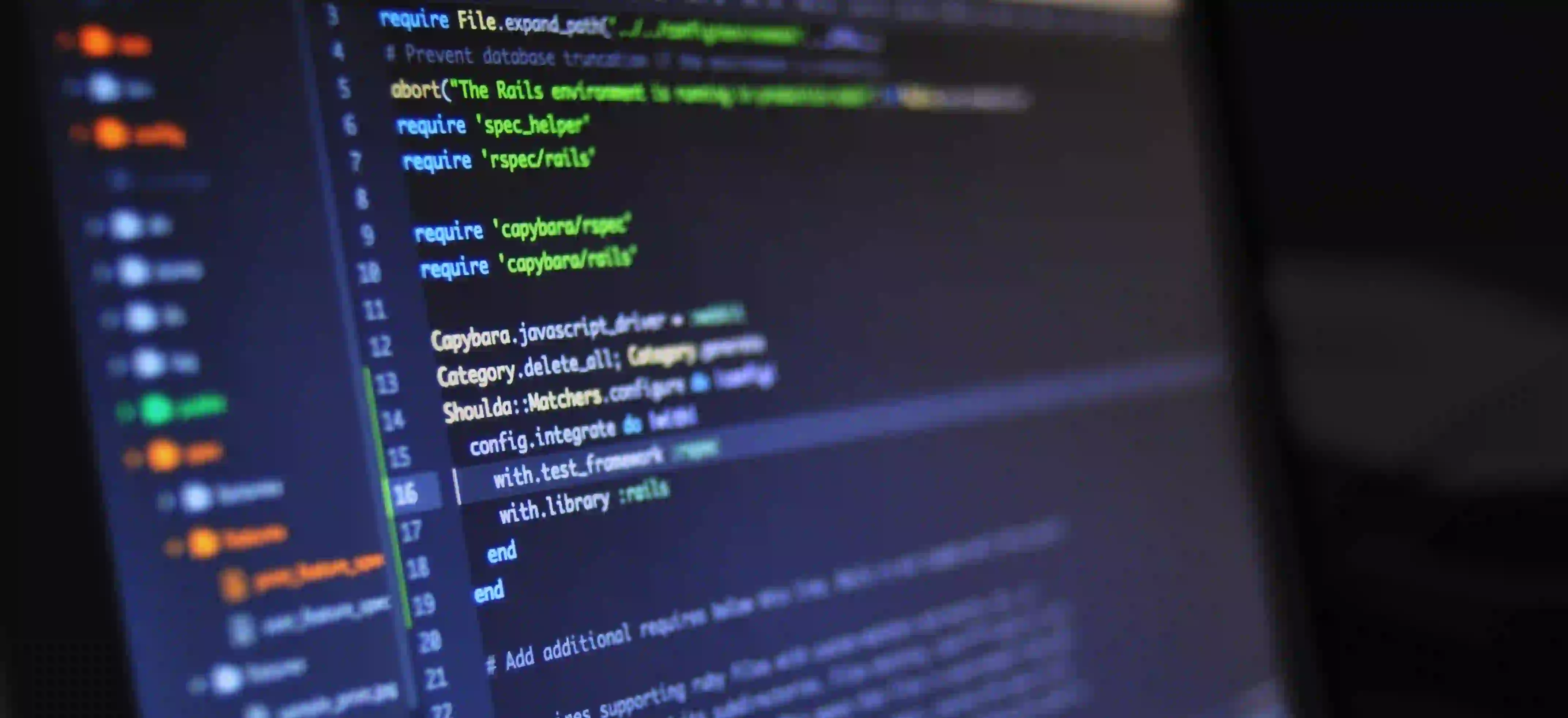
Common Pitfalls When Migrating Spring Apps to JUnit 5
Migrating your existing Spring applications from JUnit 4 to JUnit 5 can seem daunting. However, this transition opens the door to advanced features like improved extensibility, better support for Java 8+, and a more modern programming model. In this post, we’ll examine some common pitfalls you might encounter during this migration, and provide tips on how to avoid them.
Understanding JUnit 5
Before diving into the common pitfalls, let’s take a moment to understand JUnit 5. JUnit 5 is made up of three sub-projects:
- JUnit Platform: The foundation that allows for launching tests from various tools or environments.
- JUnit Jupiter: The programming model and extension model for writing tests in JUnit 5.
- JUnit Vintage: A component that provides support for running JUnit 4 tests on the JUnit 5 platform.
Migrating to JUnit 5 isn’t just about updating dependencies; it requires understanding the underlying architecture.
1. Misunderstanding the Annotations
One of the most common pitfalls is misunderstanding the differences between JUnit 4 and JUnit 5 annotations.
JUnit 4 Annotations:
import org.junit.Test;
public class MyTest {
@Test
public void testSomething() {
// test code
}
}
JUnit 5 Equivalent:
import org.junit.jupiter.api.Test;
public class MyTest {
@Test
void testSomething() {
// test code
}
}
The Why
JUnit 5 has moved some annotations under the org.junit.jupiter.api
package, while others have been completely replaced (e.g., @Before
, @BeforeClass
, @After
, @AfterClass
are replaced by @BeforeEach
, @BeforeAll
, @AfterEach
, and @AfterAll
respectively). Ensure that you replace all annotations accordingly, or your tests might not run as expected.
2. Ignoring Dependency Management
When incorporating JUnit 5, it's crucial to ensure your dependencies are properly defined. Often, developers simply replace the JUnit 4 dependency with JUnit 5 and move on.
Example Maven Dependency
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
The Why
JUnit 5 libraries are modular. You need to include additional dependencies if you're using features like parameterized tests or the vintage engine for backward compatibility. Check the JUnit 5 User Guide for comprehensive details on dependencies.
3. Mixing JUnit 4 and JUnit 5
Many developers try to run both versions concurrently during their transition. This often leads to confusion and unintended test behaviors.
The Why
While JUnit Vintage provides compatibility for running JUnit 4 tests, mixing annotations and constructs can lead to unexpected outcomes. It’s advisable to isolate tests and run them in different test suites during migration.
4. Lack of Extension Awareness
JUnit 5 introduces a powerful extension model that replaces RunWith
, allowing for greater flexibility. However, many developers overlook this capability while migrating.
Example Usage
import org.junit.jupiter.api.extension.ExtendWith;
@ExtendWith(MyCustomExtension.class)
public class MyTest {
@Test
void testWithExtension() {
// test code
}
}
The Why
Custom extensions can drastically reduce boilerplate code, improve test organization, and facilitate features like conditional execution and shared context. Make sure to leverage these offerings during your transition.
5. Failing to Update Test Lifecycle Management
JUnit 5 has refined its lifecycle model. Methods running before and after tests operate at different levels, specifically designed for more granular control.
Example of Lifecycle Management
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.AfterEach;
public class MyTest {
@BeforeEach
void setUp() {
// setup code
}
@AfterEach
void tearDown() {
// cleanup code
}
@Test
void testSomething() {
// test code
}
}
The Why
Utilizing @BeforeEach
and @AfterEach
provides a clearer understanding of when setup and teardown occur. Ensure you replace @BeforeClass
and @AfterClass
with @BeforeAll
and @AfterAll
when appropriate, and take note that these methods must be static
.
6. Not Utilizing Parameterized Tests
JUnit 5 offers an enhanced parameterized test mechanism, allowing for easy testing of multiple sets of parameters.
Example of Parameterized Test
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
public class MyTest {
@ParameterizedTest
@ValueSource(strings = {"Hello", "World"})
void testWithStringParam(String param) {
// test code with param
}
}
The Why
Utilizing parameterized tests simplifies writing repeated code tests, thus streamlining maintenance and improving readability.
7. Overlooking Migration Tools
There are automated tools available to make the transition easier, such as JUnit 5 Migration Tool, which can identify JUnit 4 tests and suggest changes.
The Why
Utilizing tools can save time and prevent errors. Relying on manual migration can introduce bugs that may take longer to resolve than if you used automated tools.
8. Not Testing the Migrated Tests
Lastly, an often underestimated stage in migration is the post-migration testing phase. While it’s essential to update tests, it’s equally crucial to verify that they still function as intended.
The Why
The act of migrating can introduce subtle issues that might not be immediately apparent. Conduct regression testing to validate that the behavior of your tests is consistent with expectations.
Final Considerations
Migrating your Spring applications from JUnit 4 to JUnit 5 can provide significant advantages, but it’s not without its pitfalls. By being aware of the common mistakes outlined in this blog post, you can navigate the migration process more smoothly.
Ensure you understand the differences in annotations, dependency management, and lifecycle handling. Take advantage of JUnit 5’s modern features, and approach the migration with a plan. Make use of automated tools, and never skip out on testing post-migration.
With these tips and a methodical approach, you'll be able to successfully migrate your Spring apps with minimal friction and maximal efficiency. Happy testing!
Further Reading
By understanding and avoiding these common pitfalls, your transition to JUnit 5 will not only be efficient but also enhance your testing capabilities in the long run.