Common Pitfalls in Service Testing with Docker Containers
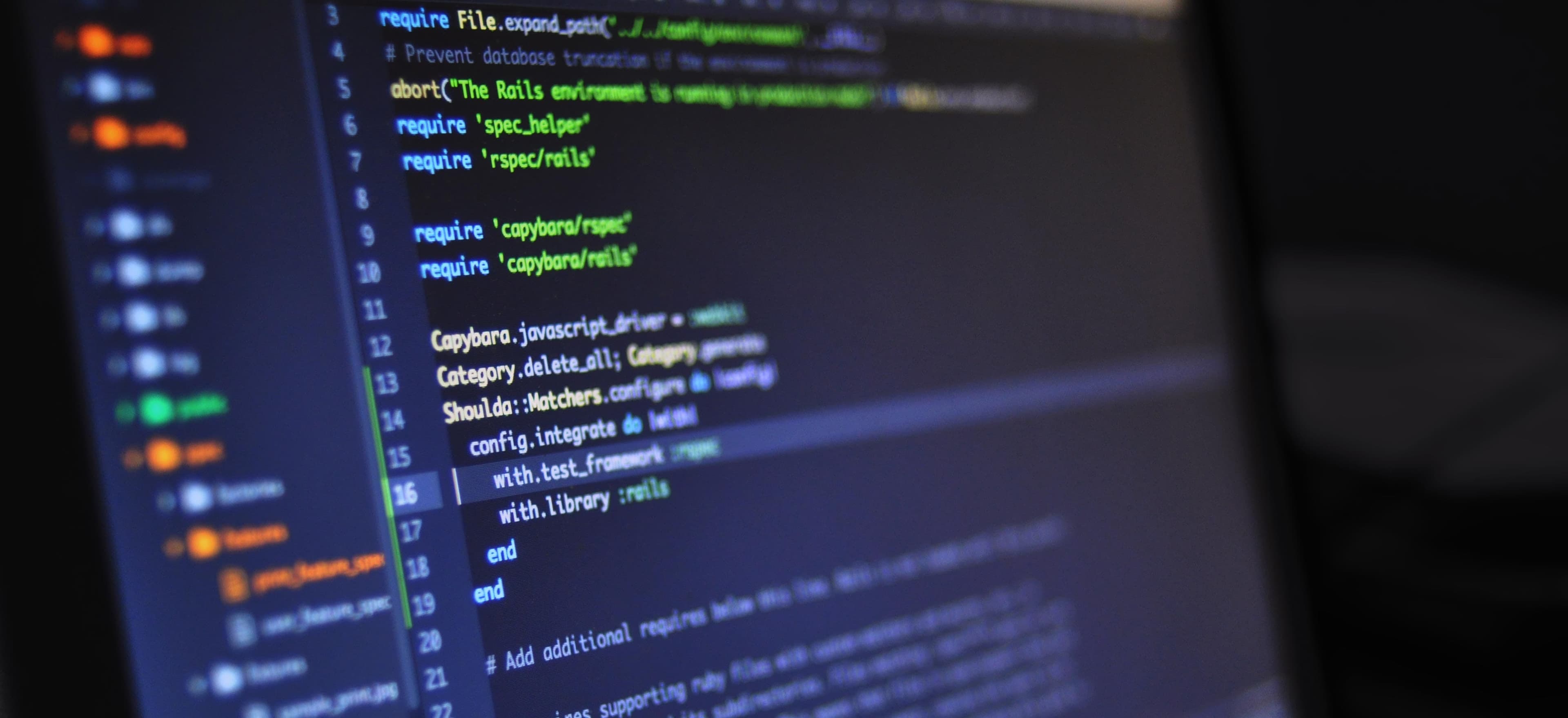
- Published on
Common Pitfalls in Service Testing with Docker Containers
Docker has revolutionized the way we build, run, and test applications. For service-oriented architectures, specifically, Docker containers provide isolation, portability, and scalability. However, as with any technology, there are common pitfalls that developers encounter, especially during service testing. This blog post aims to shed light on these pitfalls and provide actionable solutions to ensure successful service testing in Docker containers.
Understanding Docker Containers in the Context of Service Testing
Before diving into the pitfalls, let’s clarify what Docker containers are. Docker is a platform that allows developers to package applications (along with their dependencies) into standardized units called containers. These containers can be run consistently on any environment that supports Docker, be it a developer’s local machine or a production server.
For service testing, Docker containers can simulate the environment in which the service would run in production, which is beneficial for testing reliability, scalability, and performance. However, without proper understanding and planning, testing in Docker can lead to some common issues.
Common Pitfalls in Service Testing with Docker Containers
1. Ignoring Container Isolation
Docker containers are designed to be isolated from each other. However, developers sometimes inadvertently allow services to depend on the host machine’s configuration or other containers without proper management.
Solution: Always use Docker Compose or Kubernetes for orchestrating your services. Define each service with its required dependencies to ensure that tests are run in a consistent environment. Here’s an example docker-compose.yml
file:
version: '3.8'
services:
web:
image: my-web-app
build: .
ports:
- "5000:5000"
depends_on:
- db
db:
image: postgres
environment:
POSTGRES_DB: mydatabase
POSTGRES_USER: user
POSTGRES_PASSWORD: password
volumes:
- pg_data:/var/lib/postgresql/data
volumes:
pg_data:
Why this works: This structure ensures that your web app is tested alongside a dedicated database instance, eliminating reliance on external systems.
2. Overlooking Configuration Variability
Another common oversight is hardcoding configurations directly into the service code. This leads to problems when the environment changes or when running tests with different configurations.
Solution: Utilize environment variables to manage configurations. Use a .env
file in conjunction with Docker Compose:
DATABASE_URL=postgres://user:password@db/mydatabase
Why this matters: By using environment variables for configuration, you can easily switch settings for development, testing, and production environments without changing the codebase.
3. Neglecting Network Configuration
Networking can be complex in Docker, and incorrectly configured networks can lead to failures in service communication during tests. It's crucial that services can discover and communicate with one another.
Solution: Define networks in your docker-compose.yml
file to ensure that services can see and talk to each other.
networks:
app-network:
services:
web:
networks:
- app-network
db:
networks:
- app-network
Why this improves testing: This will create consistent communication pathways between services, mimicking production environments more closely.
4. Not Cleaning Up After Tests
Failing to clean up containers after tests can lead to resource exhaustion and unwanted states in subsequent tests. This can seriously hinder test reliability.
Solution: Use Docker's built-in features to ensure cleanup. Incorporate cleanup commands in your test scripts.
docker-compose down --volumes
Why this is important: It ensures that each test starts from a clean slate, reducing the chance of flaky tests due to leftover artifacts from previous runs.
5. Using Outdated Images
Using outdated base images or service images can lead to security vulnerabilities and compatibility issues.
Solution: Regularly update your Docker images and ensure you're using official or trusted sources for base images. For example, in your Dockerfile
, specify a tag that reflects the version you need:
FROM python:3.9-slim
Why this protects your application: Specifying versions helps you maintain stability while regularly checking for updates to ensure the latest patches and features are included.
6. Inadequate Logging and Monitoring
When your services are running in isolation within containers, debugging becomes more challenging. Without proper logging, identifying issues that arise during tests can prove difficult.
Solution: Implement effective logging mechanisms to capture and analyze logs during your tests. Redirect logs to standard output to take advantage of Docker's logging capabilities:
CMD ["python", "app.py"]
With proper configuration in the Docker Compose file, logs can be easily accessed by running:
docker-compose logs
Why logging is essential: It allows for real-time troubleshooting of issues, providing visibility into the application's behavior during testing.
7. Failing to Conduct Resource Testing
Containers can result in resource over-utilization, leading to performance bottlenecks if not tested properly.
Solution: Use tools such as Apache JMeter or Locust.io to simulate load tests on your containerized services.
Here’s an example of a basic Locust setup for load testing:
from locust import HttpUser, task
class WebUser(HttpUser):
@task
def load_test(self):
self.client.get("/api/data")
Why extensive resource testing is crucial: It helps ensure that your application can handle expected traffic levels, providing insight into how well containerized services perform under load.
8. Not Using the Right Testing Frameworks
Not utilizing the right frameworks can lead to ineffective testing. While it’s tempting to rely on simple assertions, proper unit, integration, and end-to-end testing frameworks are essential for quality assurance.
Solution: Implement testing frameworks like JUnit for Java-based services or Mocha for Node.js services. Here's an example using JUnit in a Spring Boot application:
@RunWith(SpringRunner.class)
@SpringBootTest(webEnvironment = WebEnvironment.RANDOM_PORT)
public class MyServiceTests {
@Autowired
private MyService myService;
@Test
public void contextLoads() {
assertNotNull(myService);
}
}
Why using frameworks matters: They provide structured testing environments, enabling more comprehensive test coverage and improved bug detection.
Closing Remarks
Testing services within Docker containers can yield reliable and scalable applications if conducted correctly. By avoiding these common pitfalls and implementing the suggested solutions, you can enhance your service testing strategy, ensuring your containers are efficient, reliable, and maintainable.
For further reading on Docker and service testing, check out Docker's official documentation and The Twelve-Factor App, which provides best practices for building software-as-a-service apps.
Stay tuned for more insights on containerization and best practices in service-oriented architectures!