Overcoming Common Pitfalls in Custom Thread Pool Executors
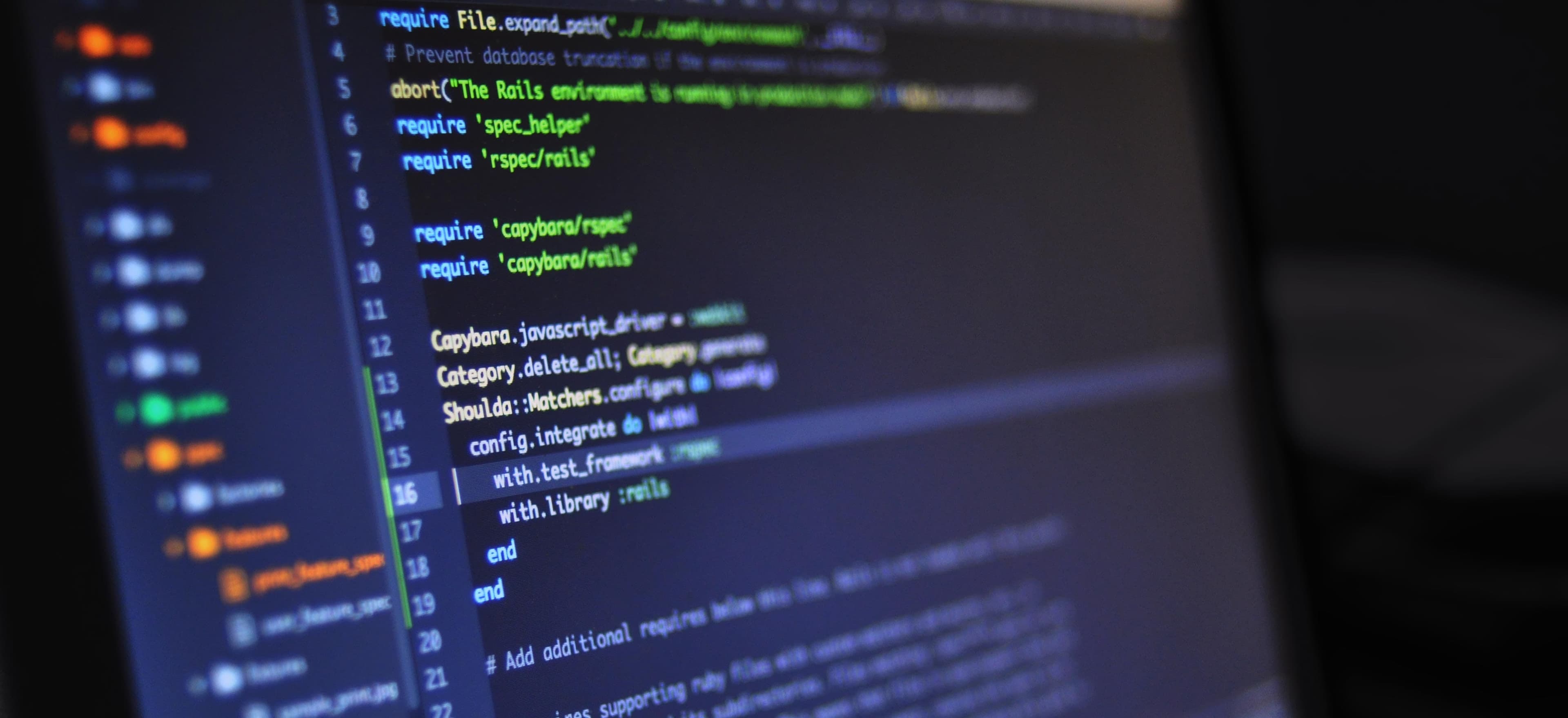
- Published on
Overcoming Common Pitfalls in Custom Thread Pool Executors
Thread pools are powerful constructs in Java that facilitate the management of multiple threads while optimizing resource use. However, building a custom thread pool executor can introduce various pitfalls that could compromise an application's performance and reliability. In this blog post, we will explore common challenges faced when creating custom thread pool executors, discuss best practices to navigate these hurdles, and provide code examples to illustrate each point.
Understanding ThreadPoolExecutor
Before delving deeper, it’s important to grasp the essence of ThreadPoolExecutor
. This class in Java’s java.util.concurrent
package provides a flexible framework for managing a pool of threads that can execute tasks asynchronously.
Here’s a basic initializer for ThreadPoolExecutor
:
import java.util.concurrent.Executors;
import java.util.concurrent.ThreadPoolExecutor;
public class SimpleExecutor {
public static void main(String[] args) {
ThreadPoolExecutor executor = (ThreadPoolExecutor) Executors.newFixedThreadPool(5);
// Execute a runnable task
executor.execute(() -> System.out.println("Task executed"));
// Properly shutdown executor
executor.shutdown();
}
}
In this example, we create a fixed-size thread pool with five threads. Each task executed by the pool will use these threads, ensuring efficient resource management.
Common Pitfalls When Implementing Custom Executors
While ThreadPoolExecutor
simplifies thread management, custom implementations may lead to several potential issues. Let’s discuss a few common pitfalls and how to work around them.
1. Failure to Handle InterruptedException
When the executing task is interrupted, it throws an InterruptedException
. Failing to handle this exception can lead to unnoticed task cancellations or thread leaks.
Solution: Always handle interruption properly by resetting the interrupt flag after catching InterruptedException
.
public void executeWithExceptionHandling(Runnable task) {
try {
task.run();
} catch (InterruptedException e) {
// Restore the interrupt flag
Thread.currentThread().interrupt();
System.out.println("Task was interrupted");
}
}
In this snippet, restoring the interrupt status ensures that other components in your application that rely on thread interruption are informed.
2. Not Shutting Down the Executor
Failing to shutdown the executor properly can lead to memory leaks and performance issues. Each thread that remains alive consumes resources, and tasks that are not completed can lead to incomplete operations.
Solution: Always invoke shutdown()
or shutdownNow()
on your executor.
executor.shutdown();
try {
if (!executor.awaitTermination(60, TimeUnit.SECONDS)) {
executor.shutdownNow();
}
} catch (InterruptedException ex) {
executor.shutdownNow();
Thread.currentThread().interrupt();
}
Using awaitTermination
allows you to gracefully wait for existing tasks to finish, enhancing performance and reliability.
3. Starting Too Many Threads
Creating too many concurrent threads can overwhelm system resources, leading to excessive context switching, high CPU usage, and slowing down the application.
Solution: Use bounded queues to limit the number of concurrent tasks. This controls the number of tasks that can wait in the queue.
import java.util.concurrent.ArrayBlockingQueue;
ThreadPoolExecutor executor = new ThreadPoolExecutor(
5, // core pool size
10, // maximum pool size
60L, // keep-alive time
TimeUnit.SECONDS,
new ArrayBlockingQueue<>(100) // bounded queue
);
In the above example, we set a maximum pool size of ten, with a blocking queue size of 100. This allows your application to maintain efficiency while avoiding overload.
4. Ignoring Thread Safety
Non-thread-safe operations can lead to data corruption when multiple threads attempt to modify shared data concurrently. You should ensure all shared resources are managed safely across threads.
Solution: Utilize synchronization mechanisms, like synchronized
blocks or concurrent collections such as ConcurrentHashMap
.
private final ConcurrentHashMap<String, Integer> taskResults = new ConcurrentHashMap<>();
public void executeTask(String taskName) {
executor.execute(() -> {
// Process the task
taskResults.put(taskName, performTask(taskName));
});
}
Using a ConcurrentHashMap
here allows multiple threads to update it without causing a race condition.
5. Not Considering Exception Handling in Tasks
When a task throws an unchecked exception, it can terminate your executor prematurely, especially if it’s not properly handled.
Solution: Catch exceptions within the run
method of your tasks to ensure that exceptions do not propagate up to the executor.
executor.execute(() -> {
try {
// Execute task
performCriticalOperation();
} catch (RuntimeException e) {
System.err.println("Task failed: " + e.getMessage());
}
});
This implementation ensures exceptions are logged or managed without bringing down your pools, thereby maintaining the application's resilience.
Best Practices for Custom Thread Pools
Now that we have identified common pitfalls and their solutions, let’s quickly summarize best practices to follow when working with custom ThreadPoolExecutor
implementations:
- Define Bounded Queues - Limit the number of tasks waiting to be executed.
- Handle InterruptedException - Restore interrupt status for graceful shutdown.
- Use Shutdown Methods - Always shut down executors to avoid resource leaks.
- Ensure Thread Safety - Make use of concurrent collections and synchronization techniques.
- Implement Robust Exception Handling - Capture exceptions within tasks to prevent executor failures.
Closing the Chapter
Creating a custom thread pool executor in Java can be both beneficial and complex. Understanding and overcoming the common pitfalls mentioned above will help you build a more robust and performant application. Adhering to best practices ensures efficient task management, reduces the risk of resource leaks, and ultimately leads to a smoother user experience.
For additional reading on concurrency in Java, you may refer to Java Concurrency in Practice or check out the Java Documentation for ThreadPoolExecutor.
With these insights, you are now equipped to create custom thread pool executors that harness the power of concurrency in your applications! Happy coding!
Checkout our other articles