Mastering Event Handling in Spring 3.x for Smooth Integration
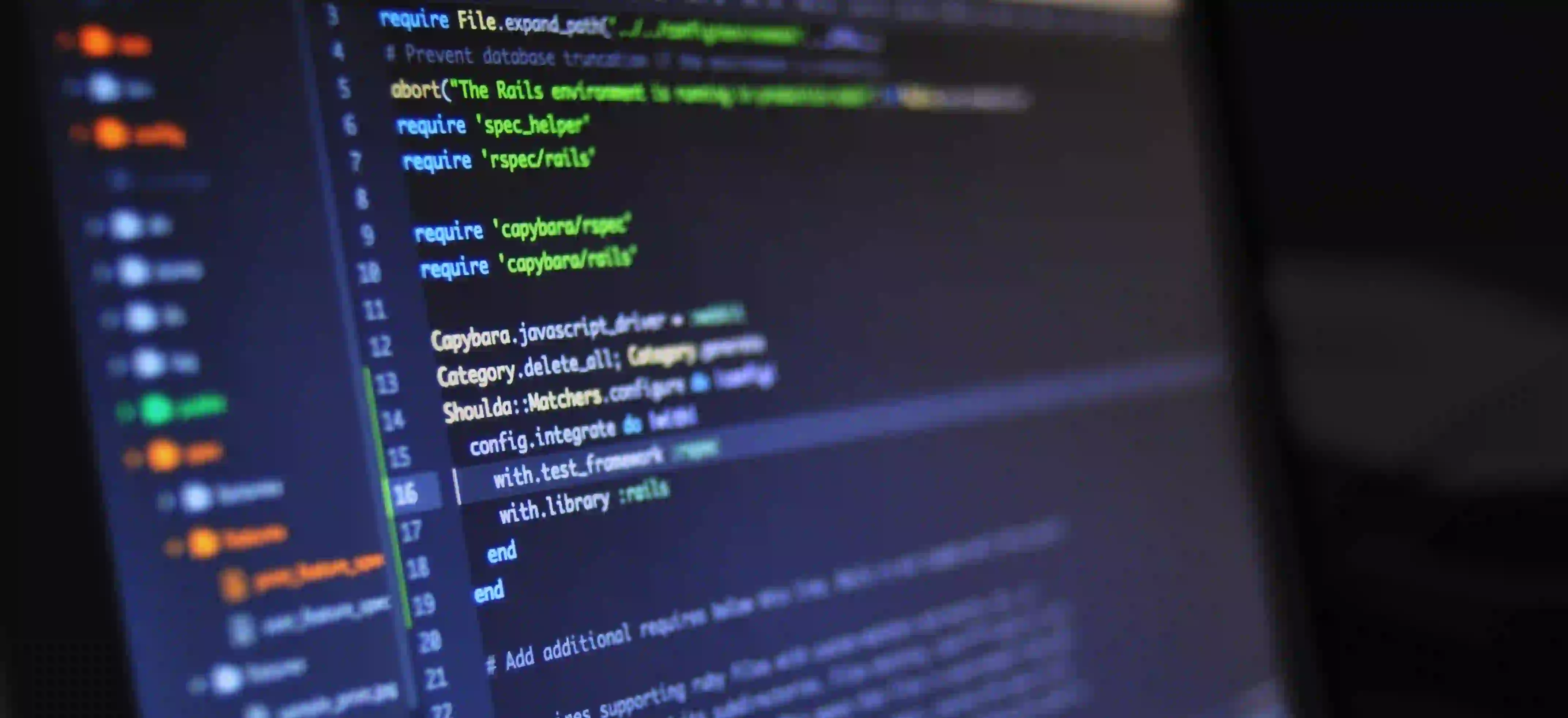
Mastering Event Handling in Spring 3.x for Smooth Integration
Spring Framework is renowned for its robust and flexible architecture. One of the critical components of this architecture is event handling. Effective event handling allows for decoupled components, which is the hallmark of a well-designed application.
In this article, we will explore event handling in Spring 3.x. We’ll cover its purpose, how it functions, and provide practical code examples. Let's dive in!
What is Event Handling?
Event handling is the process of responding to events—significant actions taken by users or the system itself. It allows your application to execute specific code when particular actions occur. In Spring, an event handling mechanism is built into the framework, allowing developers to listen to and publish events efficiently.
Why Use Spring Event Handling?
-
Decoupling Components: Events separate the sender and receiver. This is crucial for keeping code organized and maintainable.
-
Asynchronous Processing: Events can be processed asynchronously, which helps in improving application performance.
-
Improved Testability: By decoupling components, it becomes easier to test each piece in isolation.
Understanding the Event Model in Spring
Spring employs an event-driven model based on the ApplicationEvent
class. The process involves two main types of participants:
- Event Publisher: The component that publishes or fires events.
- Event Listener: The component that listens for and handles those events.
Key Components
-
ApplicationEvent: This is the base class for all events. When you want to create a custom event, you extend this class.
-
ApplicationListener: This interface allows you to define how to respond to events of the specified type.
-
ApplicationEventPublisher: This interface exposes the method to publish an event.
Creating and Publishing Custom Events
To illustrate how event handling works, let's create a simple example of a user registration system.
Step 1: Define the Custom Event
First, we will create a custom event extending ApplicationEvent
.
import org.springframework.context.ApplicationEvent;
public class UserRegisteredEvent extends ApplicationEvent {
private String username;
private String email;
public UserRegisteredEvent(Object source, String username, String email) {
super(source);
this.username = username;
this.email = email;
}
public String getUsername() {
return username;
}
public String getEmail() {
return email;
}
}
Why: By extending ApplicationEvent
, we create a new event type that carries additional data (username and email) related to the user's registration.
Step 2: Create the Event Publisher
Next, we need a class to publish this event.
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class UserRegistrationService {
@Autowired
private ApplicationEventPublisher eventPublisher;
public void registerUser(String username, String email) {
System.out.println("Registering user: " + username);
// Publish the user registration event
UserRegisteredEvent event = new UserRegisteredEvent(this, username, email);
eventPublisher.publishEvent(event);
}
}
Why: The UserRegistrationService
class manages user registration. It publishes UserRegisteredEvent
when a user registers, triggering any applicable listeners.
Step 3: Create the Event Listener
Now, we’ll create a listener that reacts to the event.
import org.springframework.context.ApplicationListener;
import org.springframework.stereotype.Component;
@Component
public class UserRegisteredListener implements ApplicationListener<UserRegisteredEvent> {
@Override
public void onApplicationEvent(UserRegisteredEvent event) {
System.out.println("User registered: " + event.getUsername());
// Additional logic like sending a welcome email could be added here
}
}
Why: The UserRegisteredListener
class implements ApplicationListener
, which specifies how it responds to UserRegisteredEvent
events.
Step 4: Configuration
Next, ensure that component scanning is enabled in your Spring configuration. This allows Spring to detect your components automatically.
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan("your.package.name")
public class AppConfig {
}
Why: This configuration enables Spring to find and register our components, including event publishers and listeners.
The Closing Argument
With this setup, whenever a user registers through the registerUser
method, a UserRegisteredEvent
is published. Any components listening for that event (like UserRegisteredListener
) will react accordingly, showing an elegant, decoupled design.
Further Enhancements
While this simple example illustrates the essentials of event handling in Spring, several enhancements can be made:
- Asynchronous Listening: Use
@Async
annotation on your event listener methods for non-blocking calls. - Event Filtering: Control which events to handle by filtering them in your listeners.
- Multiple Listeners: You can have multiple listeners for a single event, each handling different concerns.
Resources for Further Reading
- Spring Framework Documentation
- Spring Events in Action
Now that you've got a solid understanding of event handling in Spring 3.x, you can apply it to your projects for smoother integration and cleaner architecture. Happy coding!