Troubleshooting Common H2 Database Issues in Spring Boot
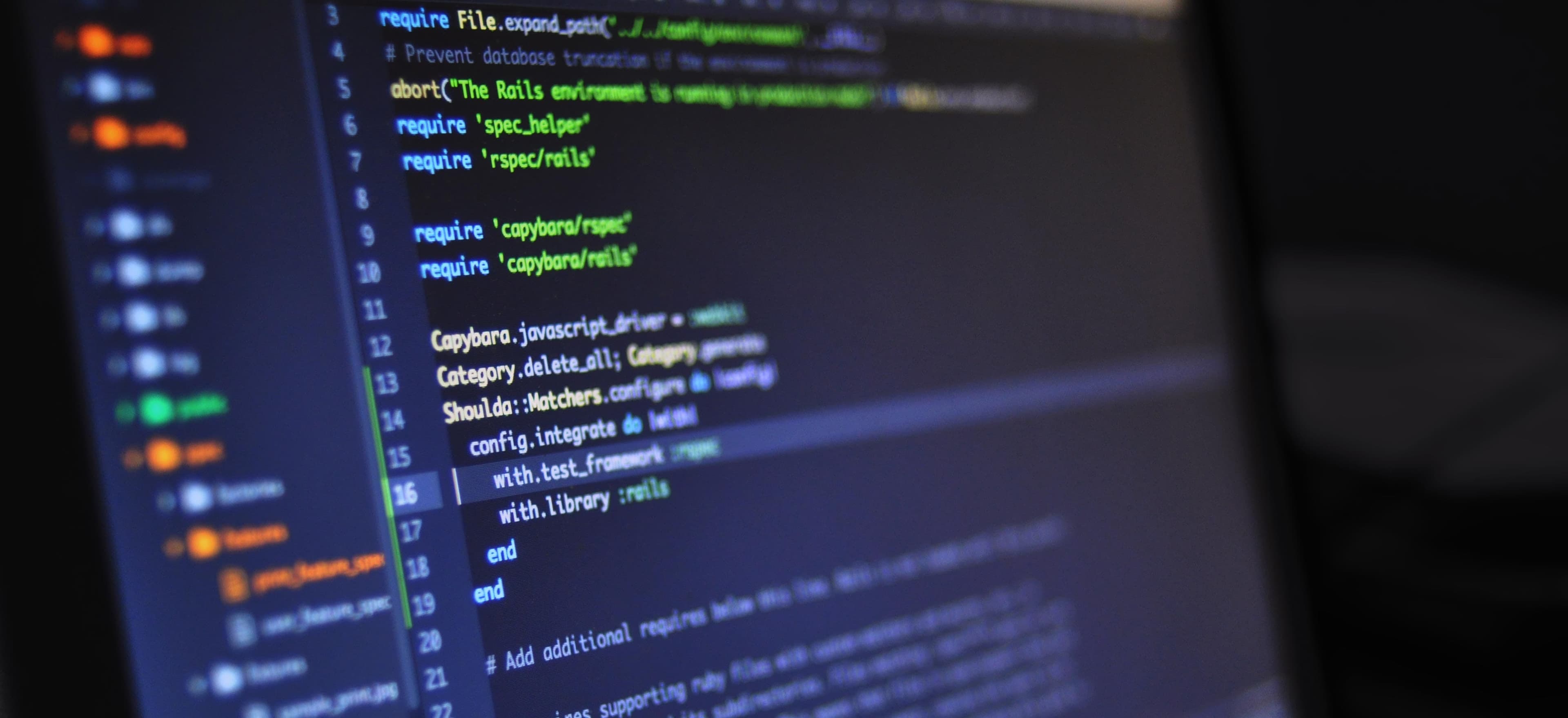
- Published on
Troubleshooting Common H2 Database Issues in Spring Boot
Spring Boot is known for its rapid development capabilities and the ease with which developers can set up applications. One of the reasons behind its popularity is the integration of an in-memory database like H2. However, despite its ease of use, developers often encounter issues when working with H2 databases in Spring Boot applications.
In this post, we'll explore some common hurdles developers face while integrating H2 with Spring Boot, how to troubleshoot them, and best practices to ensure smooth operations.
Understanding H2 Database
H2 is an open-source lightweight relational database written in Java. It's often used in development and testing environments because of its simplicity and the ability to run in memory. A few reasons why developers prefer H2 with Spring Boot include:
- No installation required: H2 runs as a Java application, meaning there's no complex installation process.
- In-memory operations: Speed up testing by using an in-memory database that resets every time the application is restarted.
- Compatibility with standard SQL: It supports standard SQL syntax, making it easy for developers familiarized with SQL to adapt.
Setting Up H2 with Spring Boot
Before diving into troubleshooting, ensure your H2 database is correctly set up in your Spring Boot application. Below is a simple setup.
Step 1: Add H2 Dependency
Include the following dependency in your pom.xml
if using Maven:
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
For Gradle, you’d include:
implementation 'com.h2database:h2'
Step 2: Configure H2
Add the following properties to your application.properties
or application.yml
file:
# Enable H2 console
spring.h2.console.enabled=true
# Database URL
spring.datasource.url=jdbc:h2:mem:testdb
# Username and password
spring.datasource.username=sa
spring.datasource.password=
# Hibernate auto DDL
spring.jpa.hibernate.ddl-auto=create
These configurations will ensure that H2 is set up correctly within your Spring Boot application.
Common H2 Database Issues
The following sections examine frequent issues encountered when using H2 databases with Spring Boot, along with their troubleshooting techniques.
1. H2 Console Not Accessible
Problem: You cannot access the H2 Console, which is a web-based GUI for managing the database.
Solution:
-
Ensure that the H2 console is enabled in your
application.properties
:spring.h2.console.enabled=true
-
Restart your Spring Boot application. You can access the console at
http://localhost:8080/h2-console
. -
If you receive an "HTTP Error 404" when trying to access it, check if you have the correct port. By default, Spring Boot runs on port 8080.
2. Database Connection Issues
Problem: You encounter a Cannot open database
error when your application attempts to connect to the H2 database.
Solution:
-
Verify your database URL, username, and password in your
application.properties
:spring.datasource.url=jdbc:h2:mem:testdb spring.datasource.username=sa spring.datasource.password=
-
Ensure you are correctly using the Go option to connect to the database in the H2 console. For example, use the following URL:
jdbc:h2:mem:testdb
This URL means you are using an in-memory database named testdb
, and will vanish when the application shuts down.
3. Data Persistence Issues
Problem: Changes made to the database do not persist after the application restarts.
Solution:
-
Remember that the default H2 database is in-memory, meaning any data is lost after the application stops. If you want persistence across sessions, you should use a file-based database:
spring.datasource.url=jdbc:h2:file:./data/testdb
This change will store the database in ./data/testdb
on your file system.
4. Compatibility Issues with SQL Queries
Problem: SQL queries that work on other databases throw an error in H2.
Solution:
-
Review the SQL syntax. H2 does support standard SQL, but there are some discrepancies, especially with constraints and datatypes.
-
Use the H2 documentation to check for supported syntax and features H2 Documentation.
5. No Tables Created in Database
Problem: Running your application doesn't create any database tables.
Solution:
- Verify that
spring.jpa.hibernate.ddl-auto=create
is set. This property allows Hibernate to automatically create tables based on your JPA entities.
If you don't have any JPA entities defined or if there are issues with annotations, Hibernate won't create any tables.
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and Setters
}
This ensures that a users
table will be created when the application first runs.
Best Practices for Using H2 with Spring Boot
-
Use Profiles: If you are using H2 for development, consider leveraging Spring Profiles to switch between H2 and your production database seamlessly. This approach allows you to maintain different database configurations.
-
Consistent Environment: Always try to replicate your development and production environments as closely as possible to avoid subtle database-related bugs.
-
Monitor Performance: While H2 is excellent for development and testing, always verify your application's performance in production with the actual database you intend to use.
-
Backup Data: If you're using file-based storage, ensure you have a backup plan in place and regularly back up your database, especially in a testing environment.
Final Considerations
H2 can be a game-changer for Spring Boot applications, especially in development and testing phases. However, as with any technology, it brings its own set of challenges. By understanding common problems and employing effective troubleshooting techniques, you can harness its full potential without having to spend hours deciphering complications.
Always ensure your configuration is correct, double-check your SQL queries for compatibility, and keep your application properties organized. With these practices in place, you can work with H2 confidently.
If you've faced unique issues or overcome challenges, feel free to share your experiences in the comments below. Happy coding!
This blog post should provide clarity not only to beginner developers but also to seasoned programmers looking to hone their skills in integrating H2 with Spring Boot. For more advanced use cases and configurations, check out the official Spring Boot Documentation or explore Baeldung's Guide on Spring Data JPA for deeper insights.
Checkout our other articles